Apollo & Dionysus#
The cycle completes. The new order stands victorious, the dust of battle settles, and history rewrites itself in the language of certainty. What was once a radical shift, a knife’s edge moment, now ossifies into law, into custom, into an unquestioned truth. The victors, once revolutionaries, now fashion themselves as the rightful inheritors of destiny. The past is erased, the purge forgotten, and what remains is the new equilibrium: 5/95, the illusion of permanence restored.
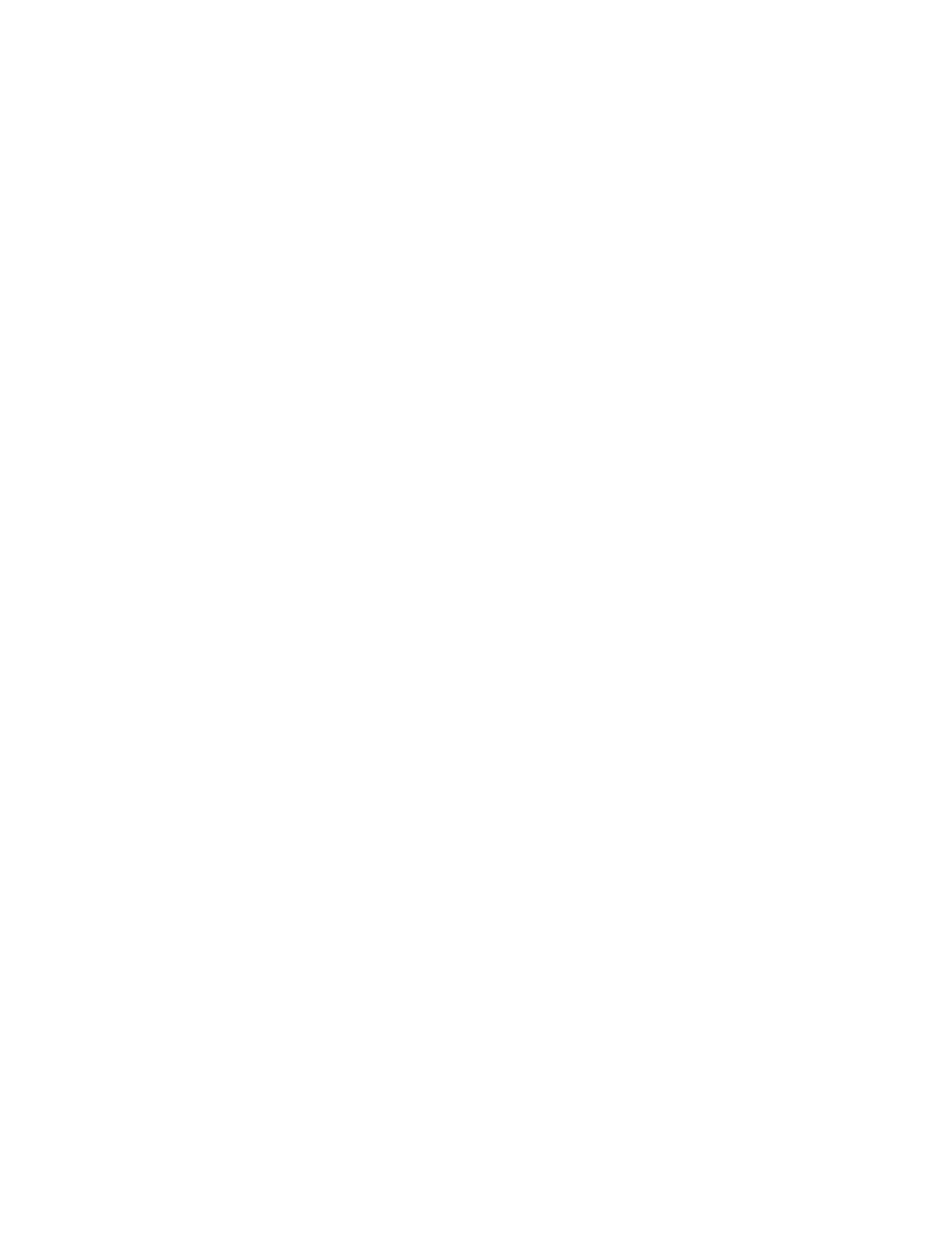
Fig. 20 Trump—Flanked By Larry Ellison, Sam Altman, & Masayoshi Son—Announces Project Stargate. President Trump, flanked by top tech executives and AI experts, announces a major new AI initiative called Project Stargate. Our App provides infrastructure that connects this to the academic medicines workflows#
Yet, as Theseus proclaims his triumph in A Midsummer Night’s Dream, there is something unsettling beneath the surface. He wooed Hippolyta with his sword, won her through violence, and yet now speaks as if harmony were always inevitable. The irony is profound—power does not transition cleanly, it devours and absorbs, turning conquest into celebration. Those who remember the prior instability are now few, their voices scattered like remnants of an old dialect no longer spoken. It is not that the revolution was a lie; it was simply one compression among many, destined to be re-compressed.
Richard III’s tragedy lies in his belief that monarchy, once seized, is permanent. But kings, like all structures, live within the churn. Heirship at 95/5 feels absolute until it isn’t. The Wars of the Roses, the French Revolution, the October Revolution—all followed the same script, each seemingly unique, yet reducible to the same mathematical inevitability: the Monarch’s Certainty, undone by Genius, leading to Chaos, resolved by Blood, and resting again in Stability. Until the cycle begins anew.
This is the anxiety that haunts empire. It is why the colonial office feared a new Christ arising in Zululand. It is why Rome fixated on a carpenter’s son in a backwater province. The deeper terror is not rebellion but reinvention—the realization that today’s permanence is only tomorrow’s prelude. If 95/5 were truly unbreakable, history would be over. But history does not stop. It only waits for the next compression.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network fractal
def define_layers():
return {
'World': ['Cosmos-Entropy', 'Planet-Tempered', 'Life-Needs', 'Ecosystem-Costs', 'Generative-Means', 'Cartel-Ends', ], # Polytheism, Olympus, Kingdom
'Perception': ['Perception-Ledger'], # God, Judgement Day, Key
'Agency': ['Open-Nomiddleman', 'Closed-Trusted'], # Evil & Good
'Generative': ['Ratio-Weaponized', 'Competition-Tokenized', 'Odds-Monopolized'], # Dynamics, Compromises
'Physical': ['Volatile-Revolutionary', 'Unveiled-Resentment', 'Freedom-Dance in Chains', 'Exuberant-Jubilee', 'Stable-Conservative'] # Values
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Perception-Ledger'],
'paleturquoise': ['Cartel-Ends', 'Closed-Trusted', 'Odds-Monopolized', 'Stable-Conservative'],
'lightgreen': ['Generative-Means', 'Competition-Tokenized', 'Exuberant-Jubilee', 'Freedom-Dance in Chains', 'Unveiled-Resentment'],
'lightsalmon': [
'Life-Needs', 'Ecosystem-Costs', 'Open-Nomiddleman', # Ecosystem = Red Queen = Prometheus = Sacrifice
'Ratio-Weaponized', 'Volatile-Revolutionary'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Inversion as Transformation", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
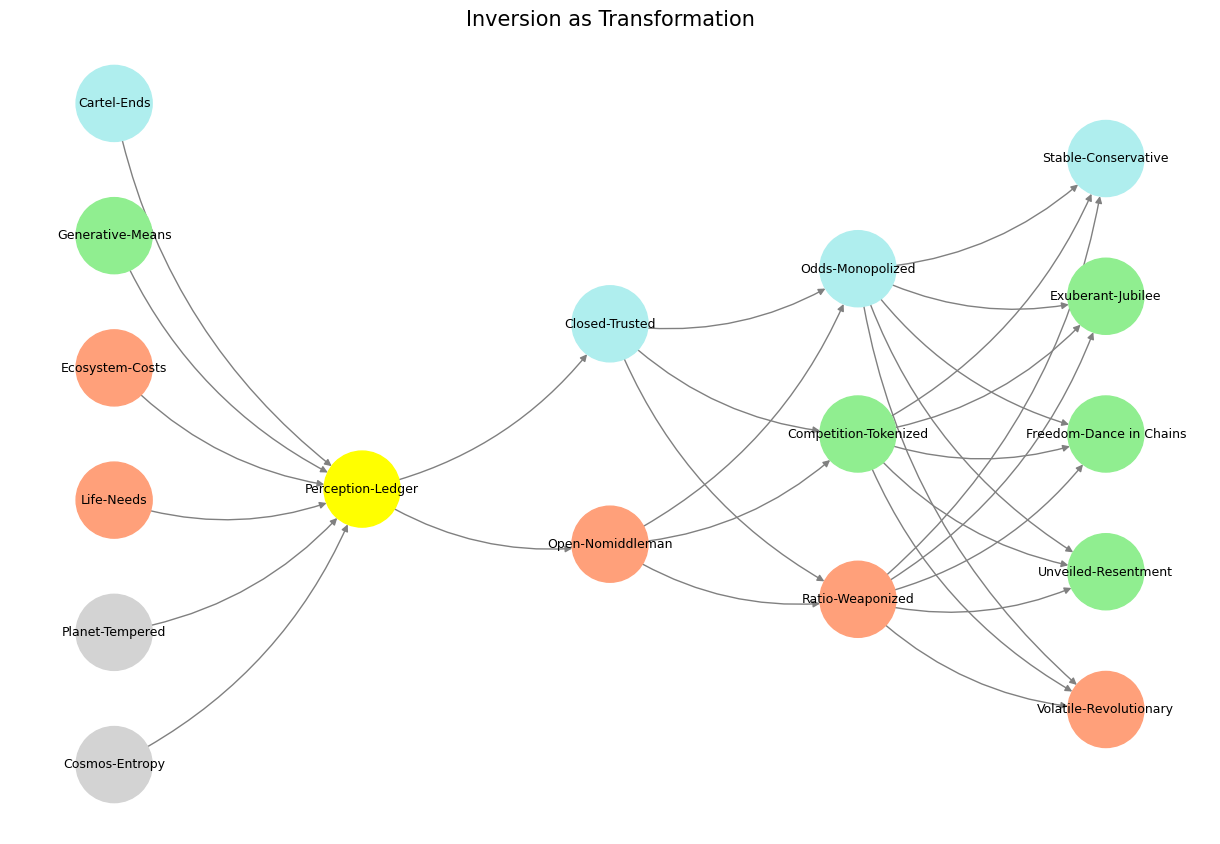
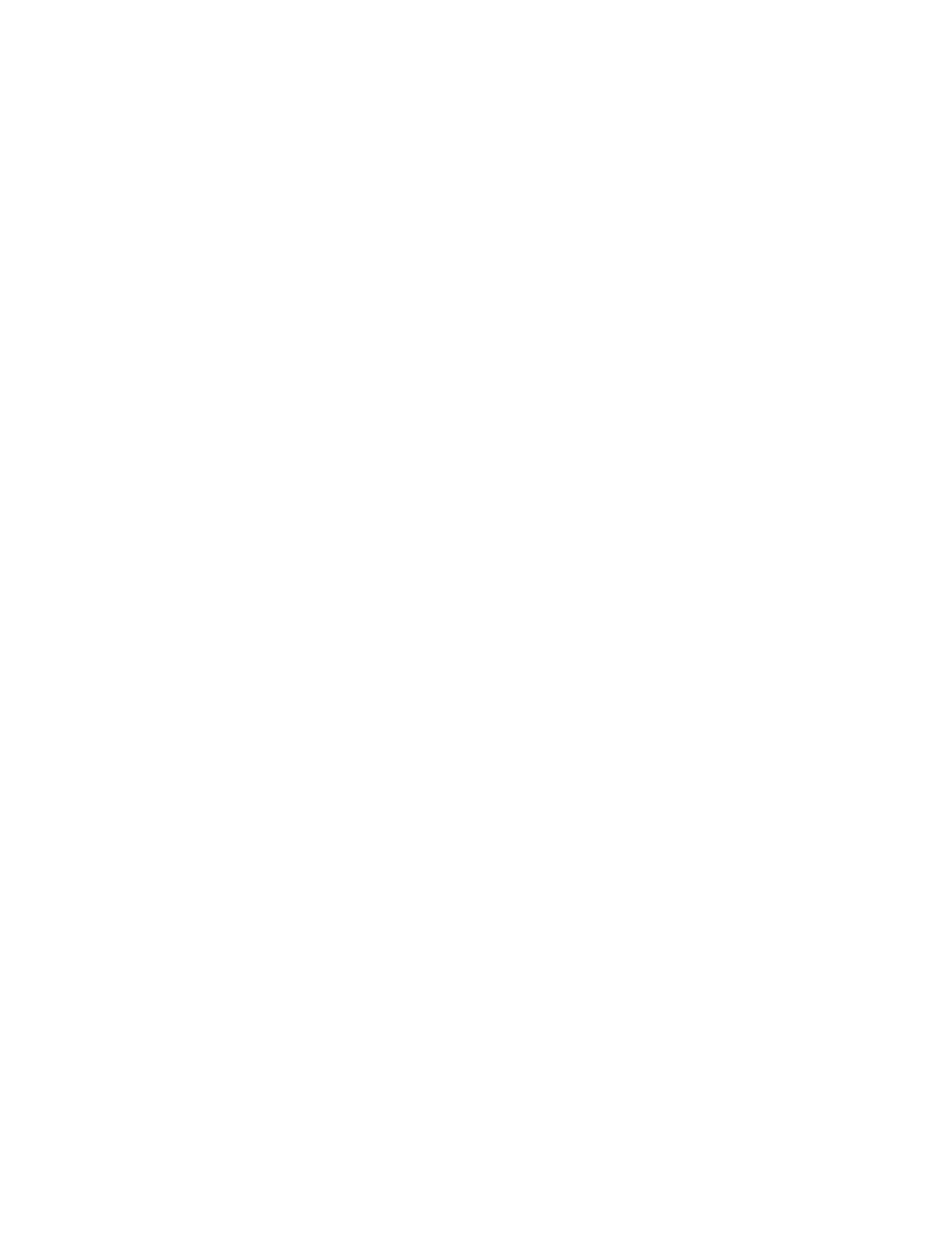
Fig. 21 Change of Guards. In Grand Illusion, Renoir was dealing the final blow to the Ancién Régime. And in Rules of the Game, he was hinting at another change of guards, from agentic mankind to one in a mutualistic bind with machines (unsupervised pianos & supervised airplanes). How priscient!#