Ecosystem#
We want to references to games to be explicit, woven into the structure of our essay as the natural law behind these forces, not just as metaphor but as the mechanics of history itself. The randomness of Heir, the adversarial skill of Genius, the long bets of Brand, the strategic depth of Tribe, and the all-consuming certainty of Religion.
These five forces—Heir, Genius, Brand, Tribe, Religion—do not merely define history; they structure it. Like a fractal pattern, they eternally recur, shaping the inheritance of power, the emergence of disruption, the codification of ideas, the consolidation of identity, and the final collapse into dogma. History does not unfold arbitrarily—it plays out through games, each force bound to a particular logic of risk, strategy, and inevitability.

Fig. 9 Isaiah 2:2-4 is the best quoted & also misunderstood article on the conditions of social harmony. Putnams discomforts with the data tells us that he was surprised by what the UN knew half a century earlier and what our biblical prophet articulated several millenia ago. Putnam published his data set from this study in 2001 and subsequently published the full paper in 2007. Putnam has been criticized for the lag between his initial study and his publication of his article. In 2006, Putnam was quoted in the Financial Times as saying he had delayed publishing the article until he could “develop proposals to compensate for the negative effects of diversity” (quote from John Lloyd of Financial Times). In 2007, writing in City Journal, John Leo questioned whether this suppression of publication was ethical behavior for a scholar, noting that “Academics aren’t supposed to withhold negative data until they can suggest antidotes to their findings.” On the other hand, Putnam did release the data in 2001 and publicized this fact. Source: Wikipedia#
Heir is a coin toss, a dice roll, a roulette spin. The odds are fixed before the wheel even turns. The British monarchy, corporate dynasties, political legacies—all of them follow this logic. A Bush, a Kennedy, a Murdoch, a Windsor—they do not fight for power; they inherit it. Inheritance does not reward merit, only survival. The heir can be competent or useless, a transformative figure or a footnote, but the seat is already theirs. Nations, too, are heirs—Germany after Versailles did not choose its burden; it was dealt a losing hand. The heir stands at the precipice, but it is the genius who decides whether to accept the hand or burn the table.
Genius plays poker. It is not about fair odds—it is about reading the game, knowing when to go all in, when to bluff, when to cut losses. The odds of a true genius appearing are staggering. For every Mozart, Bach, and Beethoven, how many billions have tried and failed? And yet, when genius arrives, it reshapes the game itself. Hitler did not inherit a powerful state—he was dealt Weimar, weak and broken. He did not accept its rules; he rewrote them. Steve Jobs did not inherit Apple as an empire—he gambled, bet against the market, and won. Genius is adversarial, unpredictable, and often destructive. It does not simply play the game—it bends the game to its will. But a genius alone does not endure. It must be branded, packaged, and sold, or it fades into obscurity.
Brand is horse racing, Formula 1, the long bet. A horse, a car, a name—it is nothing without the jockey, without the driver. Genius is raw potential, but without branding, it vanishes. The Nazis mastered this transition from genius to brand, transforming an ideology into something iconic. The swastika, the torch-lit rallies, the seamless propaganda—all of it was branding. In the modern world, Trumpism follows a similar trajectory. Trump himself is a player, but MAGA is a brand, something that can be worn, displayed, repeated in simple slogans. The strength of a brand is that it outlives the genius. Beethoven is dead, but “Beethoven” remains. Lewis Hamilton is one driver, but Formula 1 is bigger than him. The brand is what ensures continuity—but branding alone does not create power. It must be embodied, carried forward by a tribe.
Tribe is chess or war. In chess, resources are equal—what matters is resourcefulness. The tribe is an organism that does not just wear the brand; it modifies it, weaponizes it, expands it. Chess is the managerial role—directing pawns, knights, rooks, all of them pieces on a board. In war, however, resources are unequal, and resourcefulness may not be enough. The tribe is both the strategist and the soldier, ranging from errand boys who follow orders to those who undergo transformation, as in Apocalypse Now. Tribes iterate, adapt, self-propagate through marriage, conquest, and ideology. The Nazis created a racial mythos; MAGA positions itself as the true inheritor of America; Hindu nationalism under Modi fuses religion with statehood. But all tribes seek something beyond themselves, a permanence that only religion can provide.
Religion is the game of love, of infatuation, of idealism. It does not obey logic—it collapses the odds into certainty. Where the heir is determined by history, the genius by improbability, the brand by resonance, and the tribe by iteration, religion abolishes doubt entirely. It transforms the mutable into the eternal. The Nazis sought to replace Christianity with a racial mythology, where the Führer was not just a leader but a messianic figure. Trumpism, in its most fervent form, takes on the same religious intensity—every contradiction rationalized, every flaw excused, because faith has supplanted reason. Religion does not need gods; it needs belief, structure, and an eschatology. And once belief hardens into dogma, the cycle reaches its inevitable conclusion, awaiting the next genius to shatter it and begin anew.
The cycle recapitulates, fractal-like, refining and reducing complexity until it solidifies—only for the next disruptor to come and break it apart. This is why history repeats, why movements rise and fall, why the same patterns emerge across politics, business, art, and war. The odds may shift, but the structure remains immutable. The cycle is not something one escapes—it is only something one learns to play.
Show code cell source
# LEFT: Life, Ecosystem, Fire, Tech
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network fractal
def define_layers():
return {
'World': ['Cosmos-Entropy', 'Planet-Tempered', 'Life-Needs', 'Ecosystem-Costs', 'Generative-Means', 'Cartel-Ends', ], # Polytheism, Olympus, Kingdom
'Perception': ['Ledger-Isaac'], # God, Judgement Day, Key
'Agency': ['Open-Jacob', 'Closed-Esau'], # Evil & Good
'Generative': ['Ratio-Weaponized', 'Competition-Tokenized', 'Odds-Monopolized'], # Dynamics, Compromises
'Physical': ['Volatile-Revolutionary', 'Unveiled-Resentment', 'Freedom-Dance in Chains', 'Exuberant-Jubilee', 'Stable-Conservative'] # Values
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Ledger-Isaac'],
'paleturquoise': ['Cartel-Ends', 'Closed-Esau', 'Odds-Monopolized', 'Stable-Conservative'],
'lightgreen': ['Generative-Means', 'Competition-Tokenized', 'Exuberant-Jubilee', 'Freedom-Dance in Chains', 'Unveiled-Resentment'],
'lightsalmon': [
'Life-Needs', 'Ecosystem-Costs', 'Open-Jacob', # Ecosystem = Red Queen = Prometheus = Sacrifice
'Ratio-Weaponized', 'Volatile-Revolutionary'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Rebecca-Laban as Transformation", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
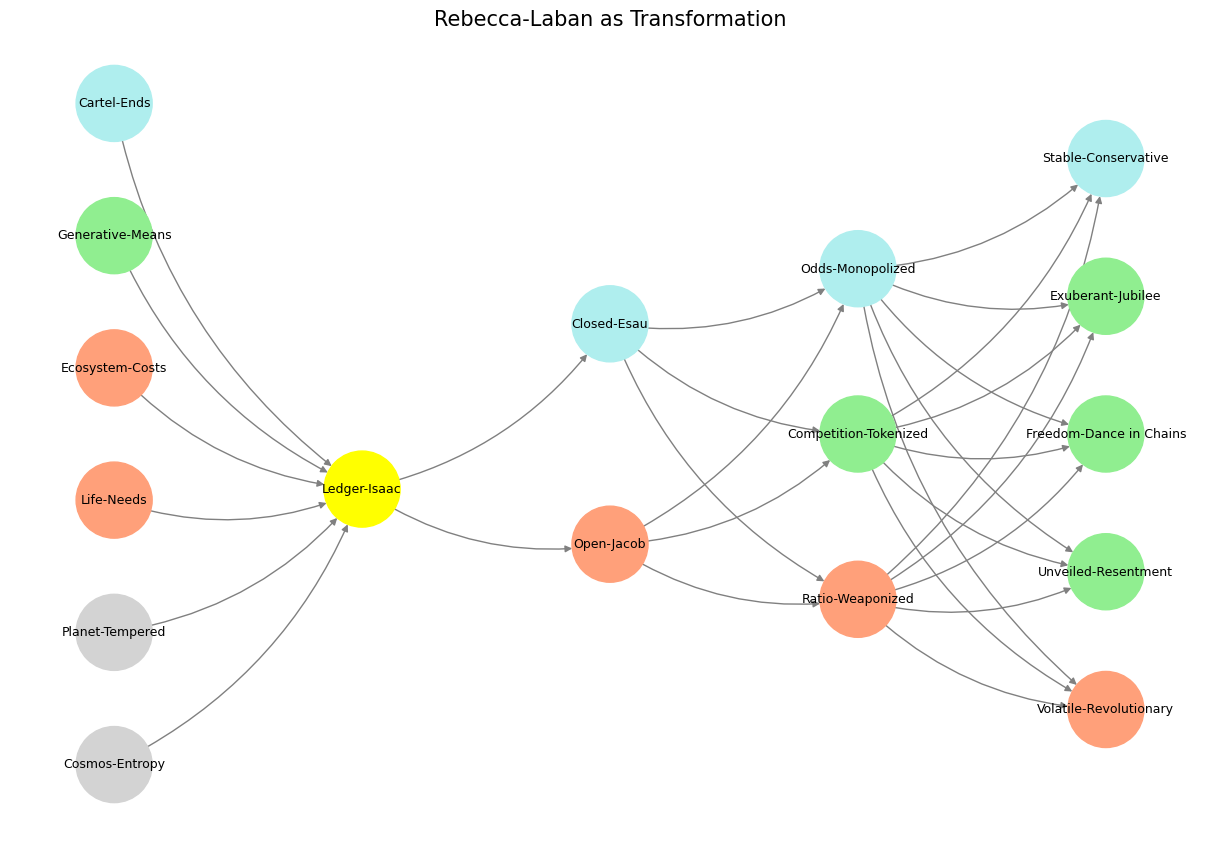
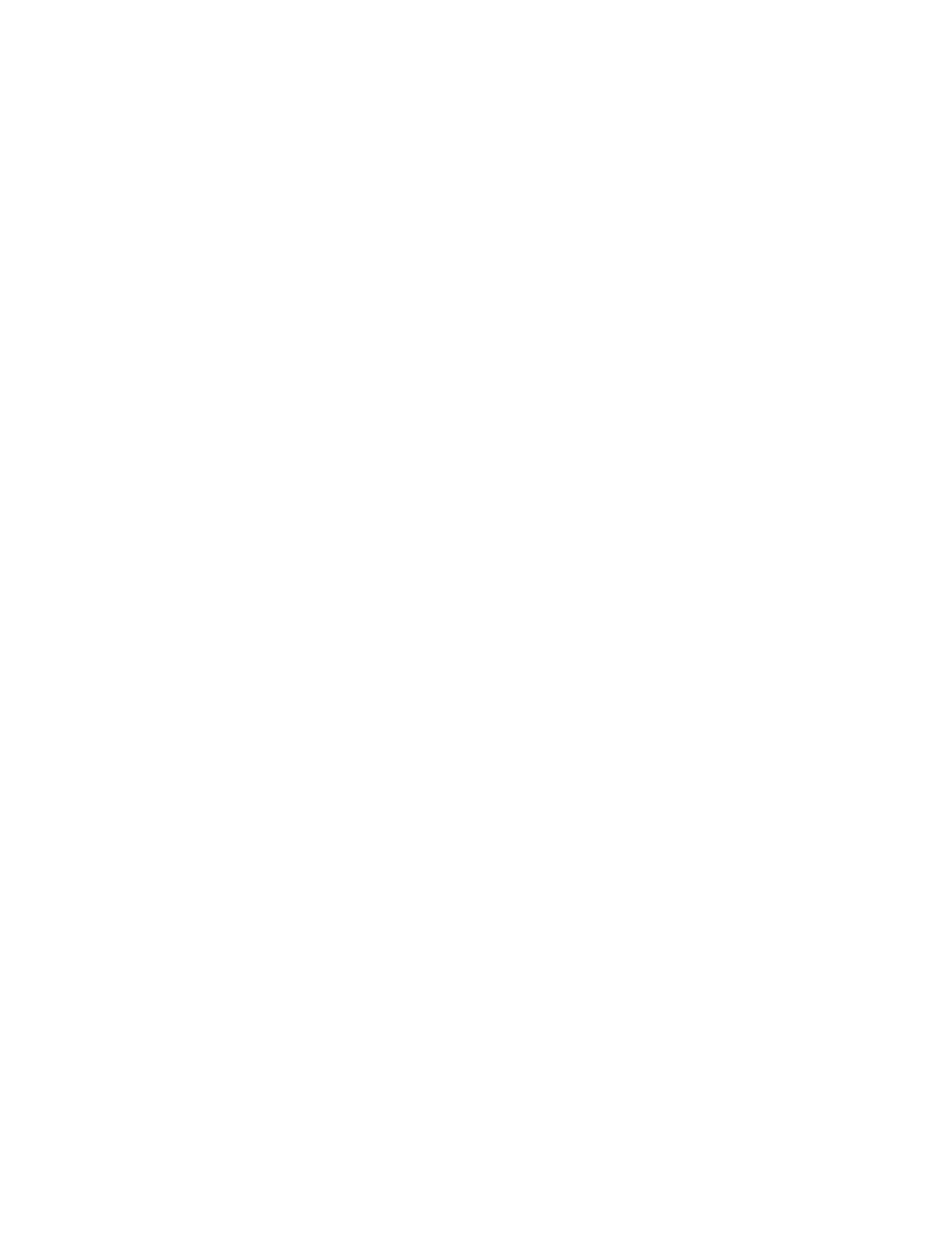
Fig. 10 So we have a banking cartel, private ledgers, balancing payments, network of banks, and satisfied customer. The usurper is a public infrastructure, with open ledgers, digital trails, block-chain network, and liberated customer#