Risk#
Proposal for Aligning Efforts on NIH Grant Projects
Dear XXX,
I’ve been reflecting on our overlapping research interests and the dynamics between the NIH grants you’re mentoring. While I initially perceived a potential conflict of interest, I believe there’s a unique opportunity here to transform it into a productive collaboration that benefits everyone involved.
Would it make sense for us to explore a coordinated approach to authorship and contributions across these projects? For example, we could streamline co-authorship by clarifying roles—such as co-first or co-senior author arrangements—and aligning on shared goals. This approach could not only resolve competition but also enhance the visibility and impact of each project.
Additionally, I’d like to propose integrating my app into the projects as a shared resource. This could serve as a unifying element, offering consistent data workflows and potentially opening up access to the datasets that have been challenging to work with independently. By pooling efforts, I’m confident we could increase productivity and ensure the best possible outcomes for both grants.
Let me know if you think this is worth discussing further. I’m happy to propose a time to meet or adapt to your availability.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Unicameralism'],
'Input': ['Commons', 'Lords'],
'Hidden': [
'Risk',
'Oxbridge',
'Peers',
],
'Output': ['Anarchy', 'Discord', 'Honors', 'Unity', 'Monarchy', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Unicameralism':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Lords':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Peers':
return 'paleturquoise'
elif node == 'Oxbridge':
return 'lightgreen'
elif node == 'Risk':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Monarchy':
return 'paleturquoise'
elif node in ['Unity', 'Honors', 'Discord']:
return 'lightgreen'
elif node == 'Anarchy':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Monarchy (Great Britain) vs. Anarchy (United Kingdom)")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
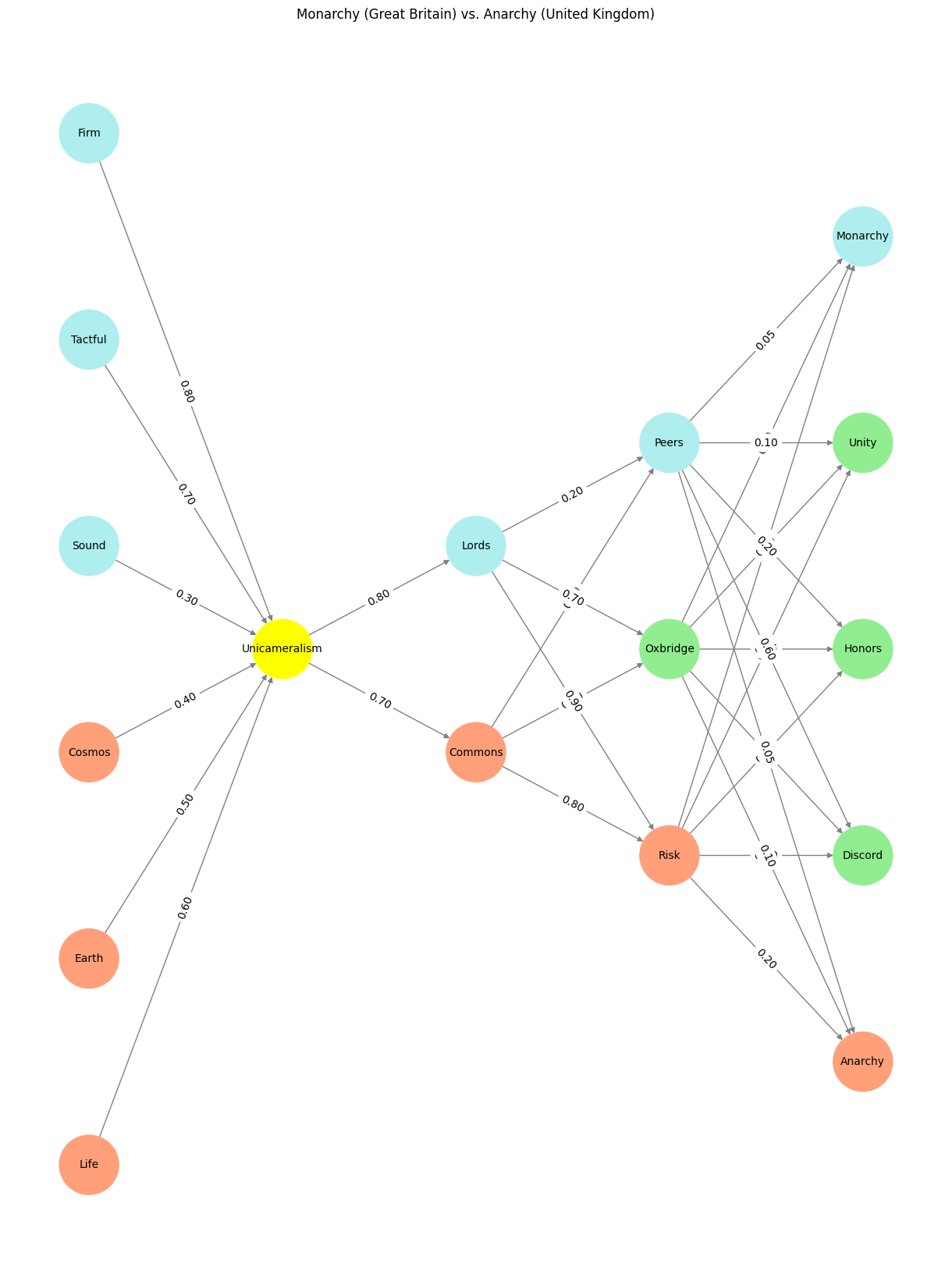
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Ideals'],
'Input': ['America', 'Britain'],
'Hidden': [
'Independence',
'FVEY',
'Colonial',
],
'Output': ['Uncertainty', 'Dissonance', 'Alliances', 'Harmony', 'Stability', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Ideals':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Britain':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Colonial':
return 'paleturquoise'
elif node == 'FVEY':
return 'lightgreen'
elif node == 'Independence':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Stability':
return 'paleturquoise'
elif node in ['Harmony', 'Alliances', 'Dissonance']:
return 'lightgreen'
elif node == 'Uncertainty':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Let There Be Light: Genesis 1:3")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
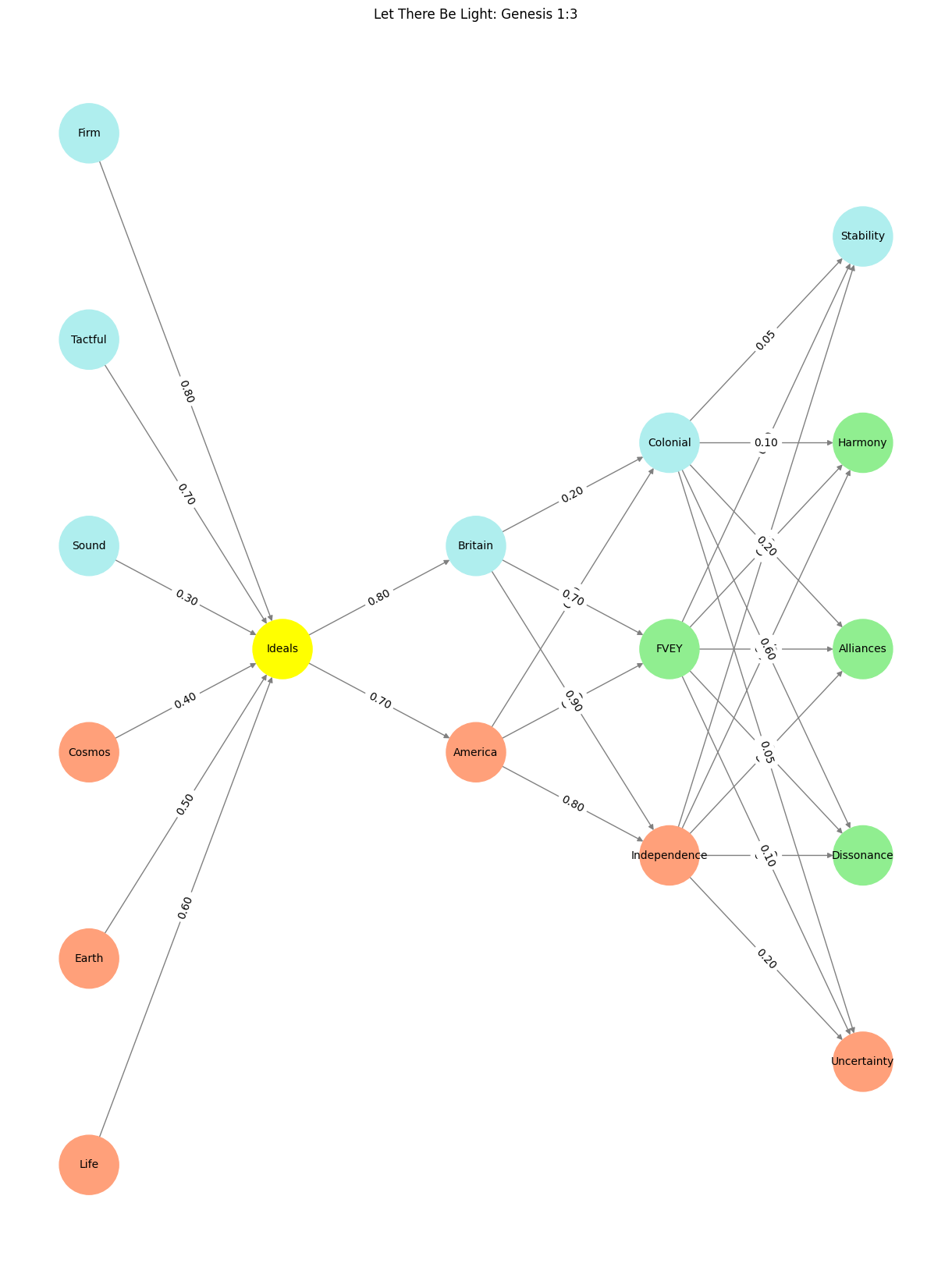
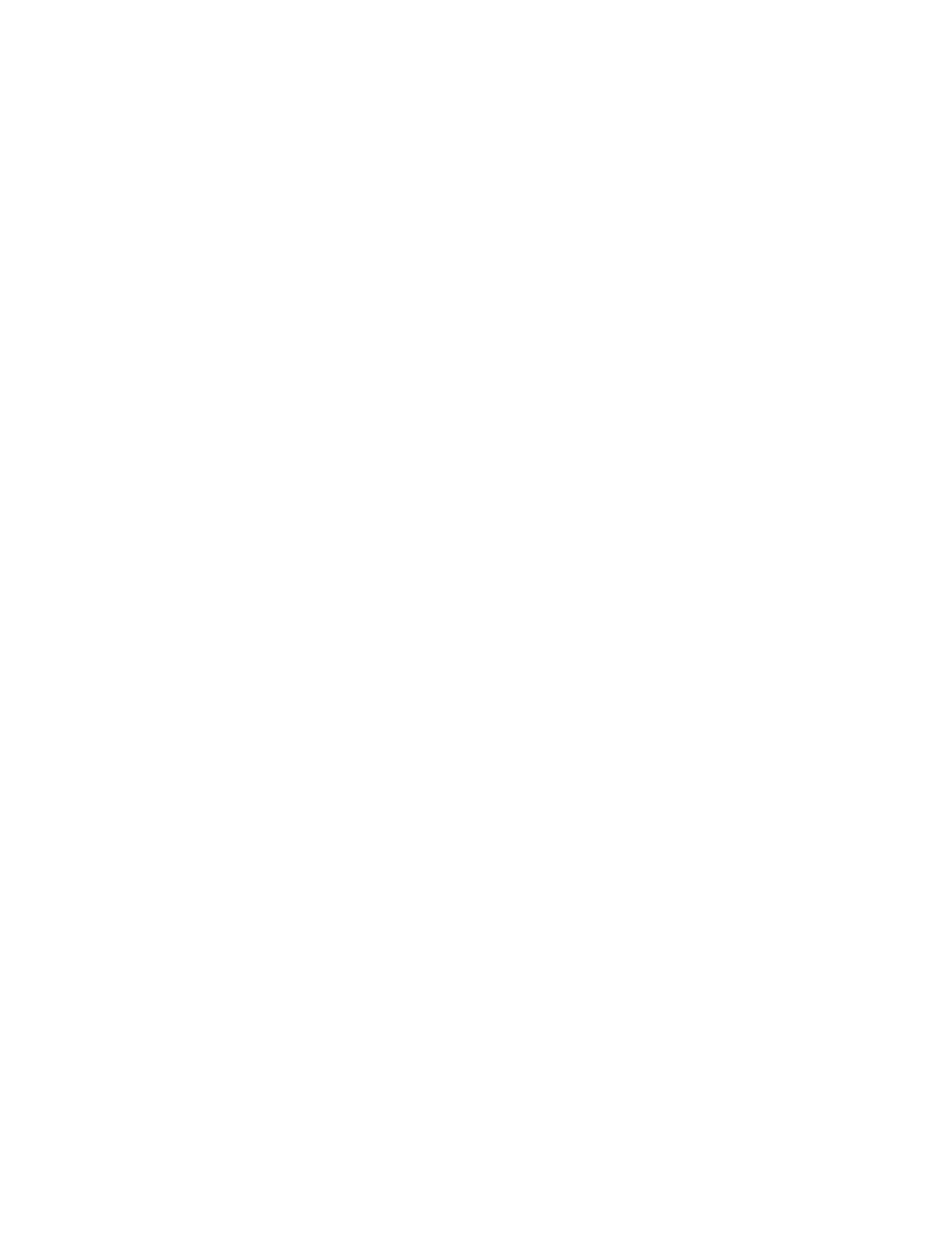
Fig. 17 In this neural network, we see the poetry of governance. Every node, edge, and weight is a verse in the epic of a nation striving to define itself against the backdrop of time. From the Pre-Input of cosmic forces to the Output of modern identity, this system is a tribute to the eternal balancing act of human existence: the quest to belong while reaching for something greater.#