Cunning Strategy#
The Living Kidney Donor App: Preserving Antiquarian Traditions, Empowering Critical Insights, and Realizing Monumental Goals#
The app Iâve been developing for living kidney donor candidates is not just a tool; it is a bridge between the timeless principles of antiquarian reverence, the sharp edge of critical analysis, and the monumental aspiration of informed consent. It offers a platform where the wisdom of the past, the scrutiny of the present, and the ideals of the future convergeâa true embodiment of the balance seen in The School of Athens, where antiquarian memory, adversarial rigor, and monumental vision coexist.
Antiquarian Foundations: Preserving the Controlled Population#
At the heart of this app lies the most venerable tradition in the study of living kidney donors: the controlled population. Antiquarian history reminds us that the foundational papers in this fieldâthe finest and most enduringâhave always respected the necessity of comparing donors with a rigorously defined group of non-donors. This tradition safeguards the integrity of our understanding by isolating the attributable risk of donation, ensuring that risks are not conflated with the baseline risks inherent in simply being human.
The app is steeped in this antiquarian sensibility. It does not merely present data; it traces its lineage. Every beta coefficient, every covariance matrix, is tied to an open canvas of how the controlled population was constructed. It invites critique and improvement, fostering continuity with the past while allowing for evolution. In this way, the app embodies the antiquarian spirit: preserving the rigor and transparency of the tradition, even as it adapts to modern needs.
Critical Insights: Introducing Dynamic Personalization#
While rooted in antiquarian reverence, the app also recognizes the limitations of static models that dominated the past. Static modes, while foundational, often rely on preselected subgroupsâspecific age ranges, racial categories, or other rigid demographicsâthat cannot accommodate the individual variability of modern donor candidates. This is where the app introduces a critical element: dynamic personalization.
By integrating dynamic, patient-specific characteristics, the app allows users to generate individualized risk profiles. It moves beyond static reporting to offer an interactive experience where users can explore how their unique attributesâage, health status, lifestyleâaffect their risks. This critical approach mirrors Athenaâs shield and spear in The School of Athens: an internal adversarial training that keeps the app sharp, adaptive, and capable of meeting the demands of Gen Z users who have grown up interacting with iPhones and crave dynamic, interactive learning tools.
This critical layer is not an abandonment of tradition but an enhancement. Just as Athenaâs spear is not wielded without her shield, the appâs dynamic features are built on the firm foundation of antiquarian rigor. It respects the static even as it transcends it, offering a critique that is constructive rather than destructive.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Surveillance'],
'Input': ['Anarchy', 'Monarchy'],
'Hidden': [
'Risk',
'Oligarchy',
'Peers',
],
'Output': ['Existential', 'Deploy', 'Fees', 'Client', 'Confidentiality', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Surveillance':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Monarchy':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Peers':
return 'paleturquoise'
elif node == 'Oligarchy':
return 'lightgreen'
elif node == 'Risk':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Confidentiality':
return 'paleturquoise'
elif node in ['Client', 'Fees', 'Deploy']:
return 'lightgreen'
elif node == 'Existential':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title(" ")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
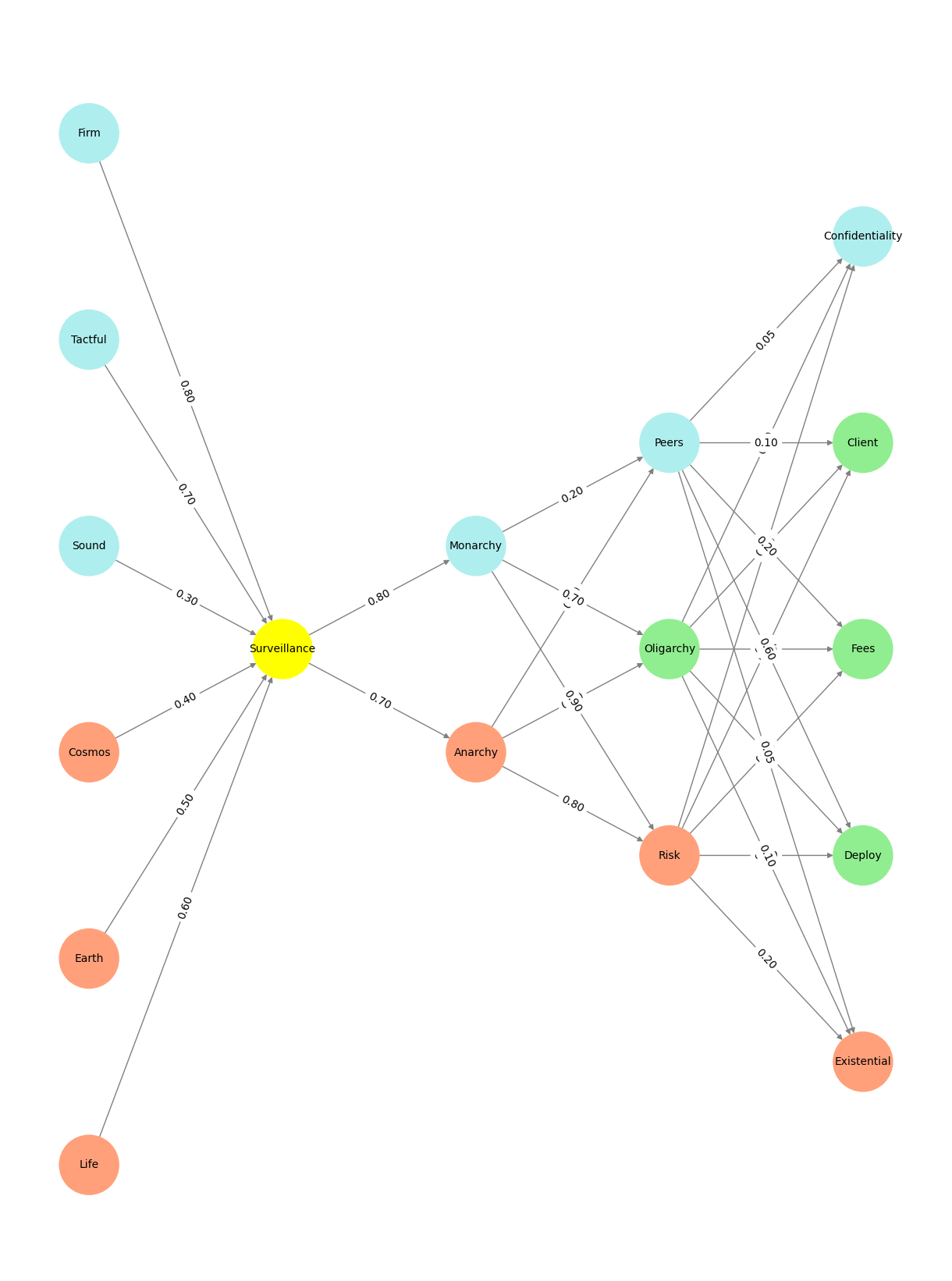
Monumental Aspiration: Informed Consent as the Ideal#
The ultimate goal of the app is nothing less than monumental: to elevate informed consent to its rightful place as a cornerstone of ethical decision-making. True informed consent is not about presenting absolute risks; it is about elucidating attributable risks. It empowers donor candidates to understand the additional risk associated with their decision to donate, grounded in the context of their baseline risks.
This monumental vision is where the app reaches its apex. By seamlessly integrating antiquarian rigor and critical dynamism, it creates a platform where candidates can make decisions that are as informed as they are personal. It upholds the ideal that every decision should be based not on fear or incomplete information but on a clear understanding of the balance between risks and benefits.
Bridging Generations: From Antiquarian Sensibilities to Gen Z Interactivity#
In this modern age, where Gen Z students and patients demand transparency and interactivity, the app offers a revolutionary approach. It invites users not only to engage with the outputsâbeta coefficients, variance matricesâbut also to trace their origins. It fosters a culture of back engineering, where users can deconstruct and critique the data models, understanding how conclusions are reached and gaining confidence in the process.
This is not merely a tool for decision-making; it is an educational platform, one that embodies the balance of Apollo, Athena, and the philosophers. It preserves the memory of the antiquarian, sharpens itself through critical analysis, and aspires to monumental goals.
A Call to the Establishment: Continuity and Innovation#
To the establishment, I extend an invitation: see this app not as a challenge to the static traditions of the past but as their natural evolution. Antiquarian rigor remains at its core, preserving the controlled population that defines the finest work in this field. But the app also acknowledges the necessity of critique, introducing dynamic personalization to meet the needs of a new generation. And it aspires to the monumental, elevating informed consent to a new standard of clarity and empowerment.
This app is a synthesis of traditions and innovations, a balance of reverence and critique, a realization of ideals. It is, in every sense, a continuation of the work my mentors pioneeredâand a step forward in the spirit of The School of Athens. Let us collaborate to ensure that this tradition endures, evolves, and inspires the future.