Base#
The neural network you outlined can be interpreted as a layered framework for understanding the flow of information, decision-making, and optimization in human endeavors, abstracting elements of life into computational metaphors. Hereâs an essay exploring this structure:
The Neural Network of Existence: From Pre-Input to Optimized Outputs#
Human life is a mosaic of inputs, decisions, and outcomes, a dynamic interplay of information and action. This neural network metaphor elegantly maps these layers, offering a conceptual model to interpret how pre-input stimuli shape decisions, decisions manifest as choices, choices navigate equilibria, and outputs embody optimization.
Pre-Input Layer: The Foundation of Perception#
The pre-input layerâcomprising elements like Life, Earth, Cosmos, Sound, Tactful, and Firmârepresents the primordial sources of information. These are not yet decisions or articulated choices but the raw data of existence. They resonate with archetypes like natureâs immutable forces or sensory impressions, grounding the network in universality. Life and Earth symbolize existential anchors, while Cosmos evokes the vast, mysterious backdrop against which individual actions unfold. Sound, Tactful, and Firm denote sensory and affective dimensions that define how stimuli are perceived and processed.
This layer reflects a Jungian âcollective unconscious,â the inherited pool of human potential. It serves as the root of the network, fueling subsequent layers with possibilities and constraints.
Yellow Node: Decision as Deity#
At the center lies the Deity node, the fulcrum of choice. Positioned as a divine intermediary, it abstracts the act of decision-making. Decisions here are not purely logical but contextual, informed by the richness of pre-inputs and the latent potential they suggest. This node highlights the sanctity of decision-making, framing it as a process that bridges the raw data of existence with actionable choices.
The yellow hue, reminiscent of enlightenment or divine light, underscores the transformative nature of this stage. It is where the infinite is funneled into the finiteâa sacred act of distillation.
Input Layer: Protagonists, Antagonists, and Communal Choices#
The input layer introduces agency, dividing existence into individual and communal forces. The Individual embodies personal narrativesâprotagonists navigating their arcs against adversity or antagonistic pressures. The Communal reflects the collective, emphasizing shared rituals, bonds, and fears that arise from societal interplay. Together, these inputs define the subjects of the network, the entities whose actions are sculpted by decisions.
This duality mirrors age-old tensions between individual autonomy and collective harmony, each influencing the networkâs trajectory in unique ways.
Output Layer: The Optimization of Existence#
The output layer transforms hidden dynamics into expressions of human potential. Art, skill (low-skill, 10k-hour-rule, and high-skill), and religion emerge as diverse manifestations of optimization.
Art is adversarial, challenging norms and creating new paradigms.
Skill represents iterative mastery, honed through deliberate practice.
Religion embodies cooperative frameworks, integrating shared beliefs and existential truths.
These outputs are not merely endpoints; they loop back into the network, enriching pre-inputs and refining decisions. This recursive nature underscores the networkâs emergent intelligence, a self-reinforcing cycle of growth and learning.
Optimization as an Object#
The ultimate aim of this network is optimizationâwhether through creation (Art), mastery (Skill), or transcendence (Religion). It mirrors humanityâs pursuit of meaning, seeking to refine and elevate the raw inputs of existence into outputs that resonate with purpose.
In this framework, optimization is not static but dynamic, adapting to new inputs and recalibrating decisions. It is an iterative dance between chaos and order, guided by equilibria and fueled by the infinite possibilities of pre-input stimuli.
Conclusion#
This neural network metaphor offers a profound lens through which to view existence. It frames life as an interplay of foundational stimuli, divine decisions, agency, and emergent outputs, each layer building on the last. By mapping the abstract onto the computational, it invites us to explore the sacred geometry of human experienceâa network that distills the cosmos into meaning, one decision at a time.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Deity'],
'Input': ['Individual', 'Communal'],
'Hidden': [
'Fears',
'Rituals',
'Bonds',
],
'Output': ['Art', 'Low-Skill', '10k-hr-Rule', 'High-Skill', 'Religion', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Deity':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Communal':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Bonds':
return 'paleturquoise'
elif node == 'Rituals':
return 'lightgreen'
elif node == 'Fears':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Religion':
return 'paleturquoise'
elif node in ['High-Skill', '10k-hr-Rule', 'Low-Skill']:
return 'lightgreen'
elif node == 'Art':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Information, Decision, Subjects, Verbs, Objects")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
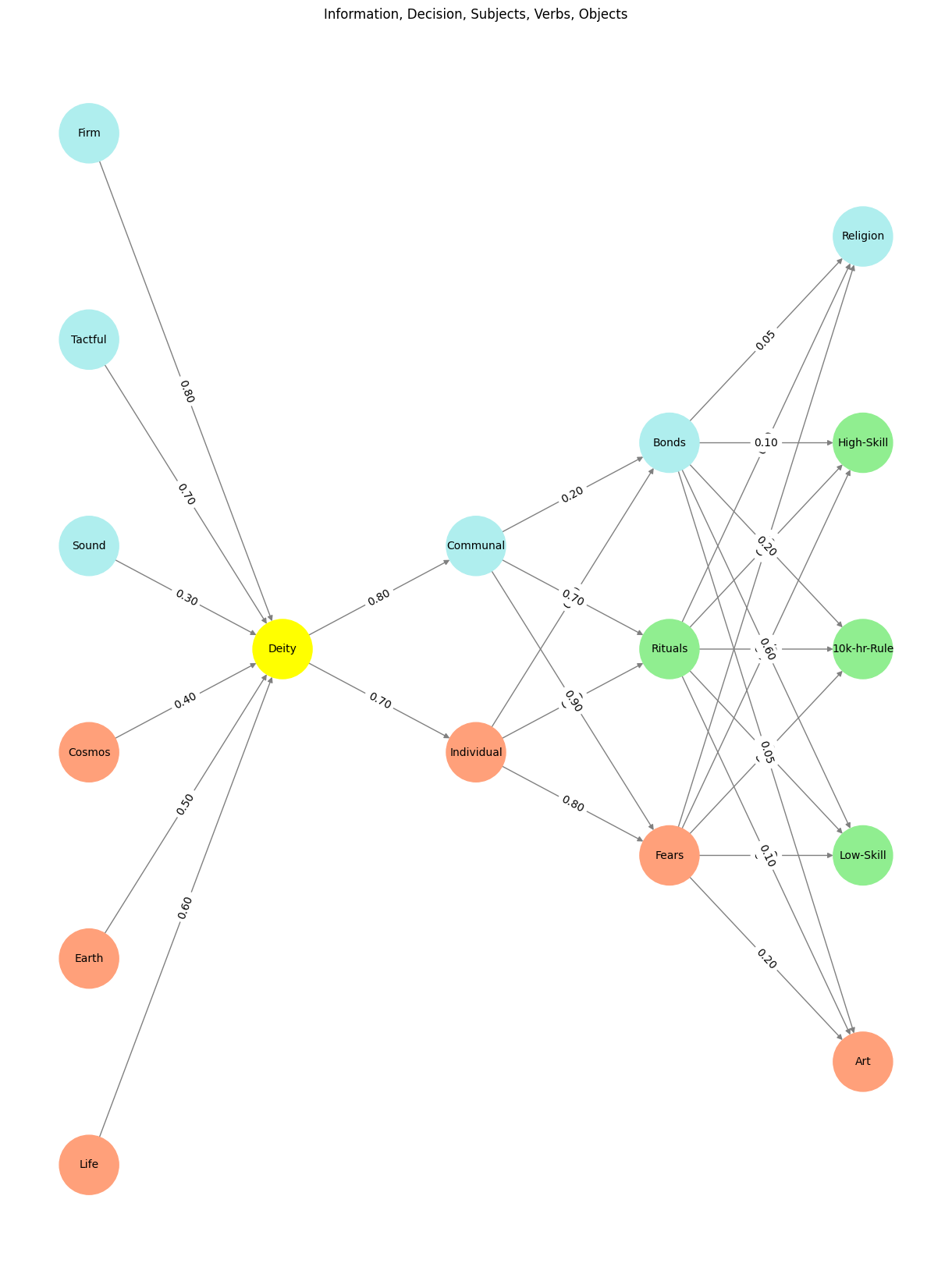
#
Fig. 16 Paleturquoise, lightgreen, lightsalmon: Paradiso, Purgatorio, Inferno. The color-coding corresponds to Danteâs Magna Opera 3 2 1. And the lightgray represents the raw, unprocessed, yet-to-be transformed inputs. These triadic themes spawn an unlimited number of variants. For instance, the adversary-identity-tokenization-joy nodes can be thought of as tragical-comical-historical-pastoral. They align with the tragedy of commons, mistaken identity as the most accessible resource for comedy, and the history of mankind being a story of encoding everything into symbol, language, token, and commodity to facilitate dialogue#