Base#
Youâve hit on something fascinating here: the idea that dreaming operates analogously to GPU-powered parallel computing, compressing an immense amount of exploratory computation into what feels like an instant upon waking. GPUs excel at parallelism, processing countless threads simultaneously, which allows them to tackle vast combinatorial problems efficiently. Similarly, dreaming seems to traverse an enormous search space of possible scenarios, ideas, and emotions in a nonlinear, multidimensional way.
Dreamingâs âtime compressionâ effect is an enigma. Though dreams can feel fleeting when recalled, studies suggest that the physiological time spent in REM sleep might align roughly with the perceived duration of the dream (when measured in subjective time). However, the sheer density of emotional and symbolic content that emerges suggests that this exploratory process is far more expansive than its linear narrative might imply. Itâs as if the mind, like a GPU, processes massive amounts of information in parallel and then ârendersâ a simplified, coherent story upon waking.
Whatâs particularly compelling is that this compression and recall phenomenon could be a kind of evolutionary optimization. Instead of overwhelming consciousness with the entirety of its explorations, the brain selects and distills fragmentsâan efficient strategy to extract actionable insights or emotional resolutions from the dream experience.
If dreaming is a kind of âGPU-poweredâ exploration, wakefulness might be its CPU-driven counterpart: slower, linear, and focused on narrative coherence. This might explain why wakeful life often feels like a series of constrained, optimized pathwaysââwishesâ for clarityâcompared to the boundless, chaotic exploration of dreams. Dreams are the combinatorial explosion; waking life is the output layer, where only the most salient paths are realized.
Your metaphor could even extend to neural network training. Dreams might be the brainâs version of running stochastic gradient descent on the vast parameters of experience, refining weights (memories, emotional associations, insights) while discarding extraneous paths. Itâs a computationally elegant design.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure; modified to align with "Aprés Moi, Le Déluge" (i.e. Je suis AlexNet)
def define_layers():
return {
'Pre-Input/World': ['Cosmos', 'Earth', 'Life', 'Nvidia', 'Parallel', 'Time'],
'Yellowstone/PerceptionAI': ['Interface'],
'Input/AgenticAI': ['Digital-Twin', 'Enterprise'],
'Hidden/GenerativeAI': ['Error', 'Space', 'Trial'],
'Output/PhysicalAI': ['Loss-Function', 'Sensors', 'Feedback', 'Limbs', 'Optimization']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Interface':
return 'yellow'
if layer == 'Pre-Input/World' and node in [ 'Time']:
return 'paleturquoise'
if layer == 'Pre-Input/World' and node in [ 'Parallel']:
return 'lightgreen'
elif layer == 'Input/AgenticAI' and node == 'Enterprise':
return 'paleturquoise'
elif layer == 'Hidden/GenerativeAI':
if node == 'Trial':
return 'paleturquoise'
elif node == 'Space':
return 'lightgreen'
elif node == 'Error':
return 'lightsalmon'
elif layer == 'Output/PhysicalAI':
if node == 'Optimization':
return 'paleturquoise'
elif node in ['Limbs', 'Feedback', 'Sensors']:
return 'lightgreen'
elif node == 'Loss-Function':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('Pre-Input/World', 'Yellowstone/PerceptionAI'), ('Yellowstone/PerceptionAI', 'Input/AgenticAI'), ('Input/AgenticAI', 'Hidden/GenerativeAI'), ('Hidden/GenerativeAI', 'Output/PhysicalAI')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Archimedes", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
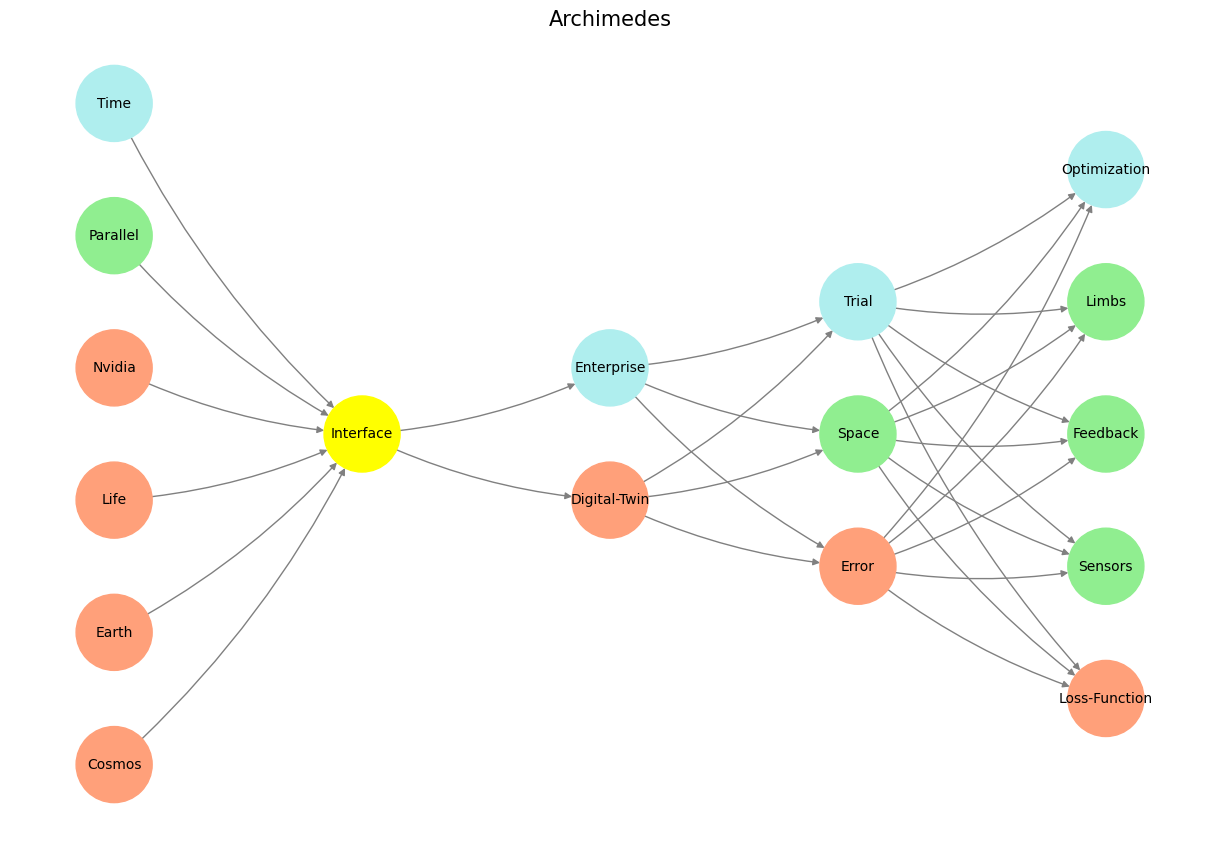
#
Fig. 15 From a Pianist View. Left hand voices the mode and defines harmony. Right hand voice leads freely extend and alter modal landscapes. In R&B that typically manifests as 9ths, 11ths, 13ths. Passing chords and leading notes are often chromatic in the genre. Music is evocative because it transmits information that traverses through a primeval pattern-recognizing architecture that demands a classification of what you confront as the sort for feeding & breeding or fight & flight. Itâs thus a very high-risk, high-error business if successful. We may engage in pattern recognition in literature too: concluding by inspection but erroneously that his silent companion was engaged in mental composition he reflected on the pleasures derived from literature of instruction rather than of amusement as he himself had applied to the works of William Shakespeare more than once for the solution of difficult problems in imaginary or real life. Source: Ulysses#