Born to Etiquette#
Prosody is not a fixed entity; it is a dynamic force, captured in the fluid interactions of layers 3, 4, and 5 in the neural network. Layer 3, Choisis, aligns prosody with melody, situating it as a mediating structure between the fixed grammatical constraints of Voir (Syntax) and the emergent structures of Deviens (Adversarial and Transactional rhythms). Prosody does not belong to a single domain but rather emerges from the iterative compression and expansion of linguistic elements—where syntax is reduced into a structured rhythm, then re-expressed as an evolving cadence. Prosody, in this sense, is a negotiation, a space where phonetic realization is neither static nor arbitrary but shaped by context, intention, and equilibrium forces.
It is in layer 4, Deviens, that prosody finds its most profound implications. This layer reveals that prosody is not merely a descriptive feature of speech but an active force that encodes optimism and pessimism as competing strategies within language. This is a crucial insight because prosody, unlike syntax, operates through shifts in tone, pacing, and emphasis rather than through rigid rules. Optimistic prosody moves toward cooperative equilibrium—it smooths transitions, reinforces certainty, and aligns with cadence patterns that anticipate resolution. Pessimistic prosody, on the other hand, introduces dissonance, fragmentation, and adversarial tension. It does not merely convey negativity in the emotional sense but encodes hesitation, instability, and anticipated breakdowns in meaning. This fourth-layer compression is, therefore, an active computational process—one in which language does not just passively reflect emotion but dynamically negotiates between equilibrium states.
The role of game theory in prosody becomes evident in this fourth layer. If we treat Deviens as a compression node where equilibrium strategies are determined, then we can see how prosody adapts within different linguistic games. A cooperative equilibrium—where communication is smooth and predictable—manifests in fluid intonation, rising and falling cadences that mirror mutual understanding. A transactional equilibrium is more measured, defined by controlled pauses and modulated shifts, aligning with the iterative exchanges of formal discourse. An adversarial equilibrium disrupts expectations, using sharp intonational breaks, unexpected shifts in stress, and deliberate withholding of prosodic resolution. The balance between these modes is not fixed but recalibrated in real time, responding to the external conditions of speech and discourse.
This dynamic capability extends into the final layer, M’èléve, where prosody resolves into cadence, payoff, and the next token. Here, we see that prosody is not merely an ornamentation of language but a structural mechanism that guides linguistic evolution. The selection of NexToken is shaped by rhythmic continuity or discontinuity, meaning that prosody influences not just how something is said but what comes next. This is where the pessimism-optimism distinction at layer 4 becomes materially significant: a pessimistic prosody contracts, limiting options, creating sharp syntactic closures, while an optimistic prosody expands, opening pathways, allowing for fluid transitions. These are not abstract tendencies but quantifiable effects—intonation patterns that can be mapped onto cooperative, iterative, and adversarial game-theoretic interactions.
Thus, prosody is not just a secondary effect of grammar but a strategic layer of linguistic cognition, one that functions as a dynamic intermediary between syntax and emergent speech. Its presence in layers 3, 4, and 5 of the network underscores its role as both a compression and expansion force, an agent of both stability and disruption. And crucially, its positioning within the game-theoretic framework of Deviens suggests that prosody is not passive but strategic, engaging in real-time negotiations between competing linguistic objectives. Through this lens, prosody is no longer an incidental feature of speech but a fundamental layer of cognitive architecture, governing the oscillations between order and flux that define all human communication.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Foundational', 'Grammar', 'Syntax', 'Punctuation', "Rhythm", 'Time'], # Static
'Voir': ['Syntax.'],
'Choisis': ['Punctuation.', 'Melody'],
'Deviens': ['Adversarial', 'Transactional', 'Rhythm.'],
"M'èléve": ['Victory', 'Payoff', 'Loyalty', 'Time.', 'Cadence']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Syntax.'],
'paleturquoise': ['Time', 'Melody', 'Rhythm.', 'Cadence'],
'lightgreen': ["Rhythm", 'Transactional', 'Payoff', 'Time.', 'Loyalty'],
'lightsalmon': ['Syntax', 'Punctuation', 'Punctuation.', 'Adversarial', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Foundational', 'Syntax.'): '1/99',
('Grammar', 'Syntax.'): '5/95',
('Syntax', 'Syntax.'): '20/80',
('Punctuation', 'Syntax.'): '51/49',
("Rhythm", 'Syntax.'): '80/20',
('Time', 'Syntax.'): '95/5',
('Syntax.', 'Punctuation.'): '20/80',
('Syntax.', 'Melody'): '80/20',
('Punctuation.', 'Adversarial'): '49/51',
('Punctuation.', 'Transactional'): '80/20',
('Punctuation.', 'Rhythm.'): '95/5',
('Melody', 'Adversarial'): '5/95',
('Melody', 'Transactional'): '20/80',
('Melody', 'Rhythm.'): '51/49',
('Adversarial', 'Victory'): '80/20',
('Adversarial', 'Payoff'): '85/15',
('Adversarial', 'Loyalty'): '90/10',
('Adversarial', 'Time.'): '95/5',
('Adversarial', 'Cadence'): '99/1',
('Transactional', 'Victory'): '1/9',
('Transactional', 'Payoff'): '1/8',
('Transactional', 'Loyalty'): '1/7',
('Transactional', 'Time.'): '1/6',
('Transactional', 'Cadence'): '1/5',
('Rhythm.', 'Victory'): '1/99',
('Rhythm.', 'Payoff'): '5/95',
('Rhythm.', 'Loyalty'): '10/90',
('Rhythm.', 'Time.'): '15/85',
('Rhythm.', 'Cadence'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with weights
for (source, target), weight in edges.items():
if source in G.nodes and target in G.nodes:
G.add_edge(source, target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("Grammar is the Ecosystem", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
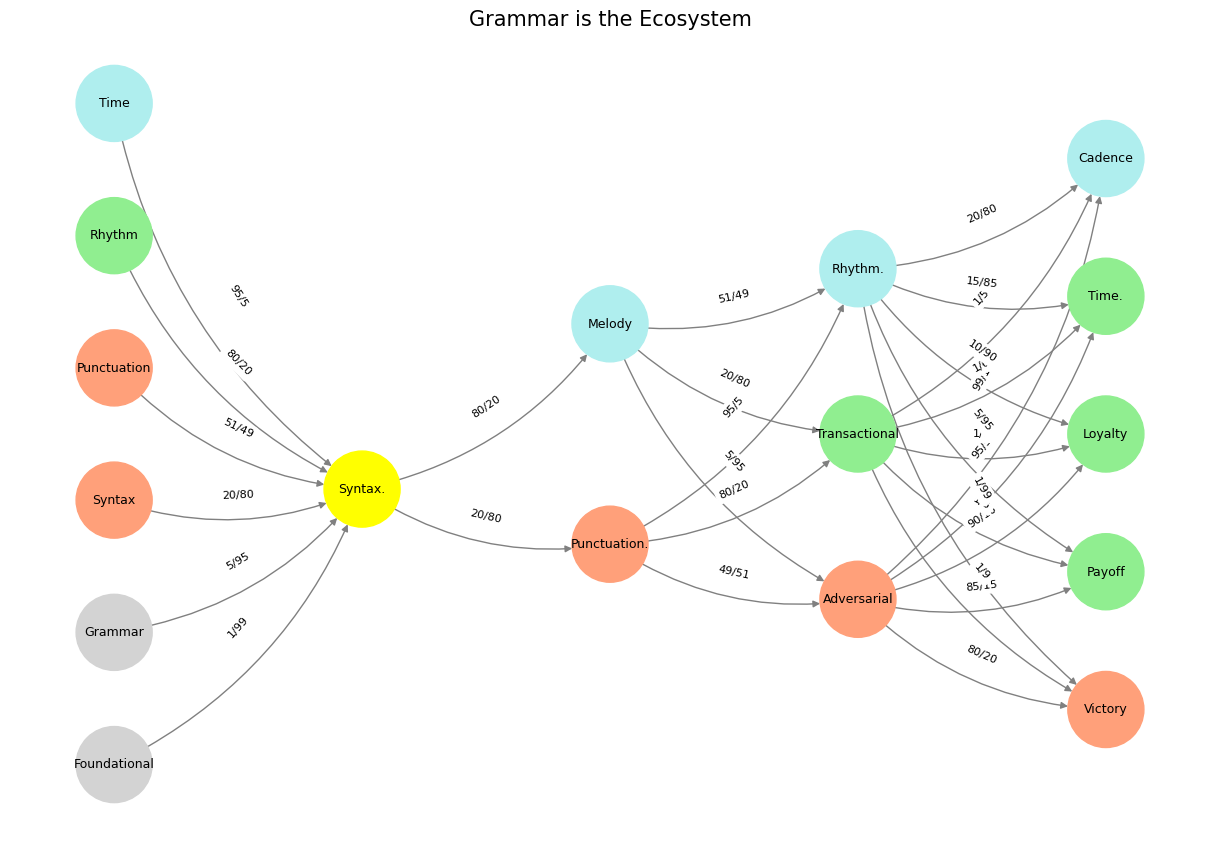
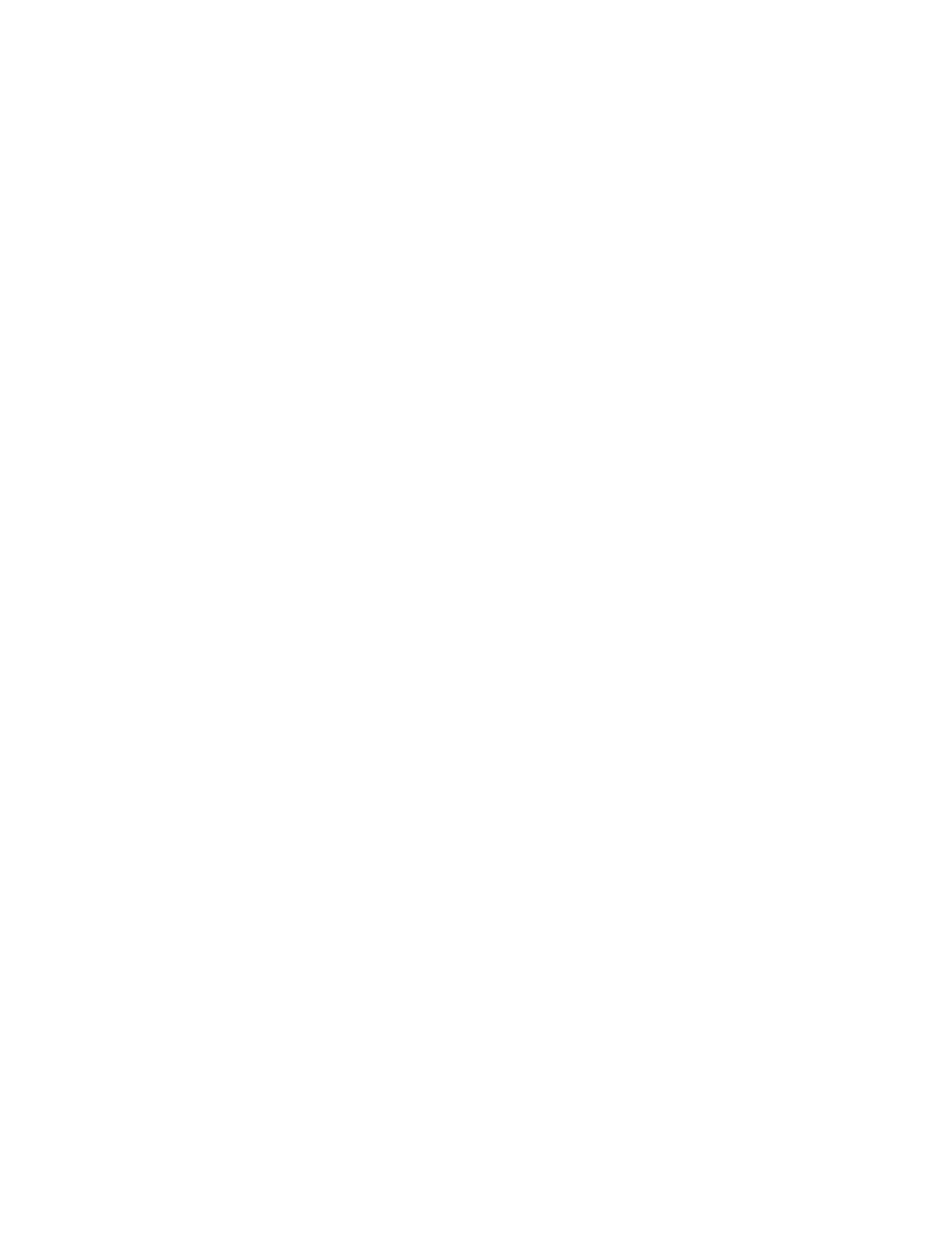
Fig. 29 Glenn Gould and Leonard Bernstein famously disagreed over the tempo and interpretation of Brahms’ First Piano Concerto during a 1962 New York Philharmonic concert, where Bernstein, conducting, publicly distanced himself from Gould’s significantly slower-paced interpretation before the performance began, expressing his disagreement with the unconventional approach while still allowing Gould to perform it as planned; this event is considered one of the most controversial moments in classical music history.#