Prometheus#
Institutions that receive grant applicants may provide templates for applicants to follow, and compliance with these templates will inevitably emerge as a key screening mechanism and grant-winning formula. The grant-writing process, already a competitive landscape, is shaped not merely by the content of an applicant’s proposal but also by their ability to adhere to the implicit and explicit structures imposed by funding bodies. These templates serve as a form of organizational grammar, a structural syntax that transforms an applicant’s ideas into a form digestible by the institution’s evaluative framework.
Grant templates function as a filtering mechanism that enforces standardization, thereby streamlining the review process for funding bodies. Much like in language, where syntax dictates meaning and coherence, grant structures impose an artificial clarity upon ideas, ensuring they align with the evaluative criteria of the grant committee. This process mirrors the way grammar and syntax govern the transmission of thought. An applicant who intuitively aligns their proposal with these structures increases their likelihood of success, not necessarily because their ideas are superior but because they have successfully embedded them within a framework optimized for comprehension and evaluation.
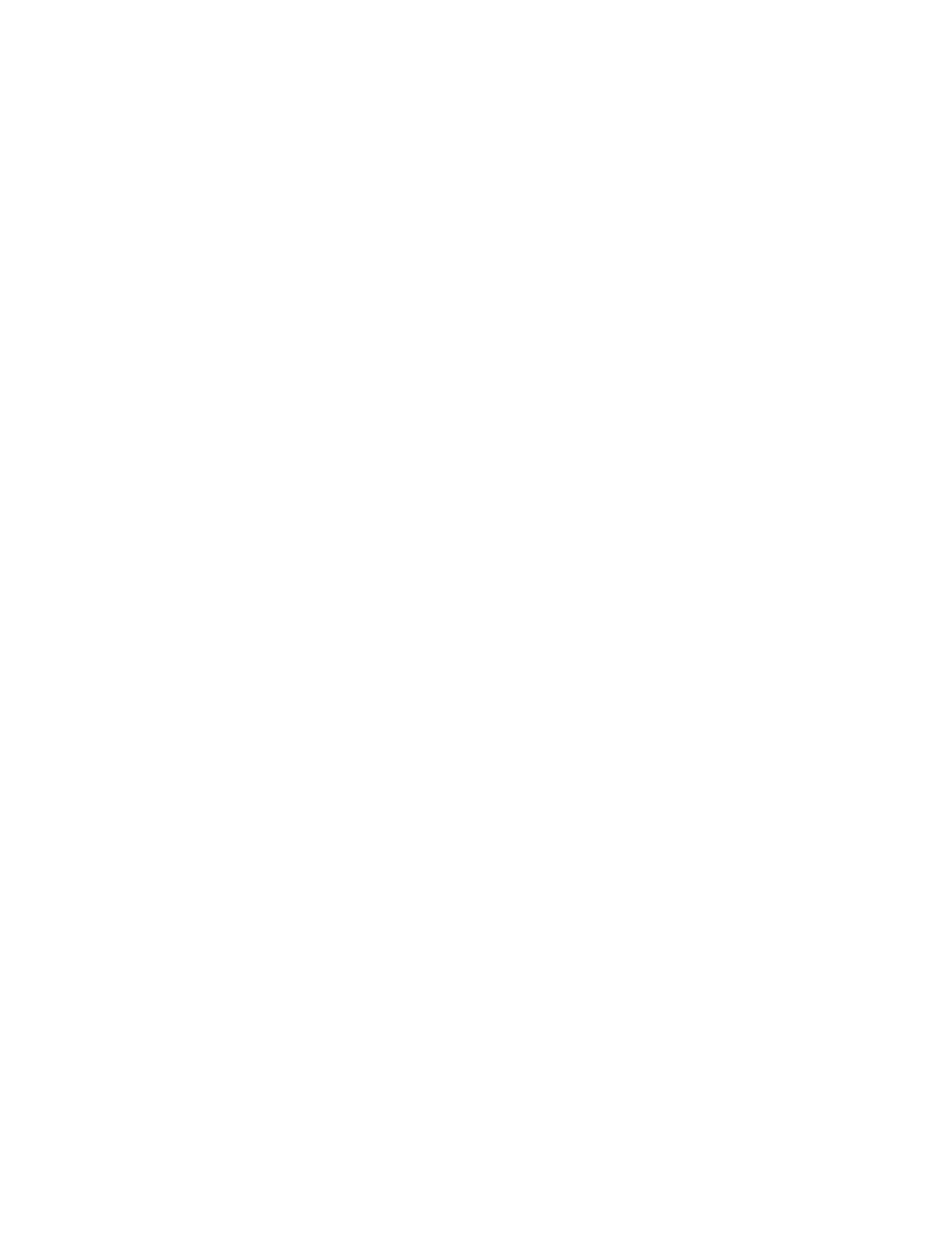
Fig. 11 Our protagonist’s odyssey unfolds across landscapes of five flats, six flats, and three sharps. With remarkable delight and sure-footedness, the journey explores the full range of extensions and alterations of basic triads and seventh chords across all seven modes.#
At its core, grant writing is both an adversarial and transactional process. The adversarial dimension emerges in the competition among applicants, where only a fraction will be awarded funding. The transactional aspect manifests in the applicant’s ability to translate their research objectives into the language of the funding body. This process is akin to the rhythm of a structured neural network, where certain nodes must activate in sequence to produce an output. Foundational concepts such as feasibility, innovation, and impact serve as the primary nodes, while sections like methods, budget, and dissemination pathways form the connecting edges that bind the proposal into a cohesive whole.
Just as in language, where syntax and punctuation dictate clarity, grant templates impose an analogous constraint upon applicants. Syntax in grant applications often manifests through required headings and subsections, while punctuation is akin to the budgetary constraints that govern the feasibility of a project. In an adversarial context, these elements become strategic tools, with successful applicants demonstrating mastery not just of their field but of the structural rules governing the grant-writing ecosystem. Rhythm and cadence also play a role—particularly in how an applicant constructs their narrative, ensuring it flows with a balance between technical rigor and persuasive clarity. Those who fail to recognize the implicit musicality of a well-structured grant proposal may find their applications relegated to the rejection pile.
Victory in grant writing is achieved when the applicant effectively translates their vision into the institution’s structural requirements. This translation is not merely a matter of semantics but of structural conformity. Just as in music, where adherence to rhythmic principles can dictate audience reception, in grant writing, cadence—marked by clarity, logical sequencing, and strategic emphasis—can determine an applicant’s success. The institutional review process values coherence and fluency, rewarding those who internalize and apply its formulaic constraints.
Ultimately, the institutions that provide these templates are shaping more than just the application process—they are refining the very grammar of funding. Compliance becomes more than a bureaucratic hurdle; it becomes an essential skill in the game of resource acquisition. In this way, grant writing is not merely about the innovation contained within the proposal but about the ability to navigate the syntactic and rhythmic expectations of the evaluative system. Understanding this ecosystem, and mastering its grammar, is what separates successful applicants from those left outside the funding loop.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Foundational', 'Grammar', 'Syntax', 'Punctuation', "Rhythm", 'Time'], # Static
'Voir': ['Syntax.'],
'Choisis': ['Punctuation.', 'Habits'],
'Deviens': ['Adversarial', 'Transactional', 'Rhythm.'],
"M'èléve": ['Victory', 'Payoff', 'Loyalty', 'Time.', 'Cadence']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Syntax.'],
'paleturquoise': ['Time', 'Habits', 'Rhythm.', 'Cadence'],
'lightgreen': ["Rhythm", 'Transactional', 'Payoff', 'Time.', 'Loyalty'],
'lightsalmon': ['Syntax', 'Punctuation', 'Punctuation.', 'Adversarial', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Foundational', 'Syntax.'): '1/99',
('Grammar', 'Syntax.'): '5/95',
('Syntax', 'Syntax.'): '20/80',
('Punctuation', 'Syntax.'): '51/49',
("Rhythm", 'Syntax.'): '80/20',
('Time', 'Syntax.'): '95/5',
('Syntax.', 'Punctuation.'): '20/80',
('Syntax.', 'Habits'): '80/20',
('Punctuation.', 'Adversarial'): '49/51',
('Punctuation.', 'Transactional'): '80/20',
('Punctuation.', 'Rhythm.'): '95/5',
('Habits', 'Adversarial'): '5/95',
('Habits', 'Transactional'): '20/80',
('Habits', 'Rhythm.'): '51/49',
('Adversarial', 'Victory'): '80/20',
('Adversarial', 'Payoff'): '85/15',
('Adversarial', 'Loyalty'): '90/10',
('Adversarial', 'Time.'): '95/5',
('Adversarial', 'Cadence'): '99/1',
('Transactional', 'Victory'): '1/9',
('Transactional', 'Payoff'): '1/8',
('Transactional', 'Loyalty'): '1/7',
('Transactional', 'Time.'): '1/6',
('Transactional', 'Cadence'): '1/5',
('Rhythm.', 'Victory'): '1/99',
('Rhythm.', 'Payoff'): '5/95',
('Rhythm.', 'Loyalty'): '10/90',
('Rhythm.', 'Time.'): '15/85',
('Rhythm.', 'Cadence'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with weights
for (source, target), weight in edges.items():
if source in G.nodes and target in G.nodes:
G.add_edge(source, target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("Foundational Grammar", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
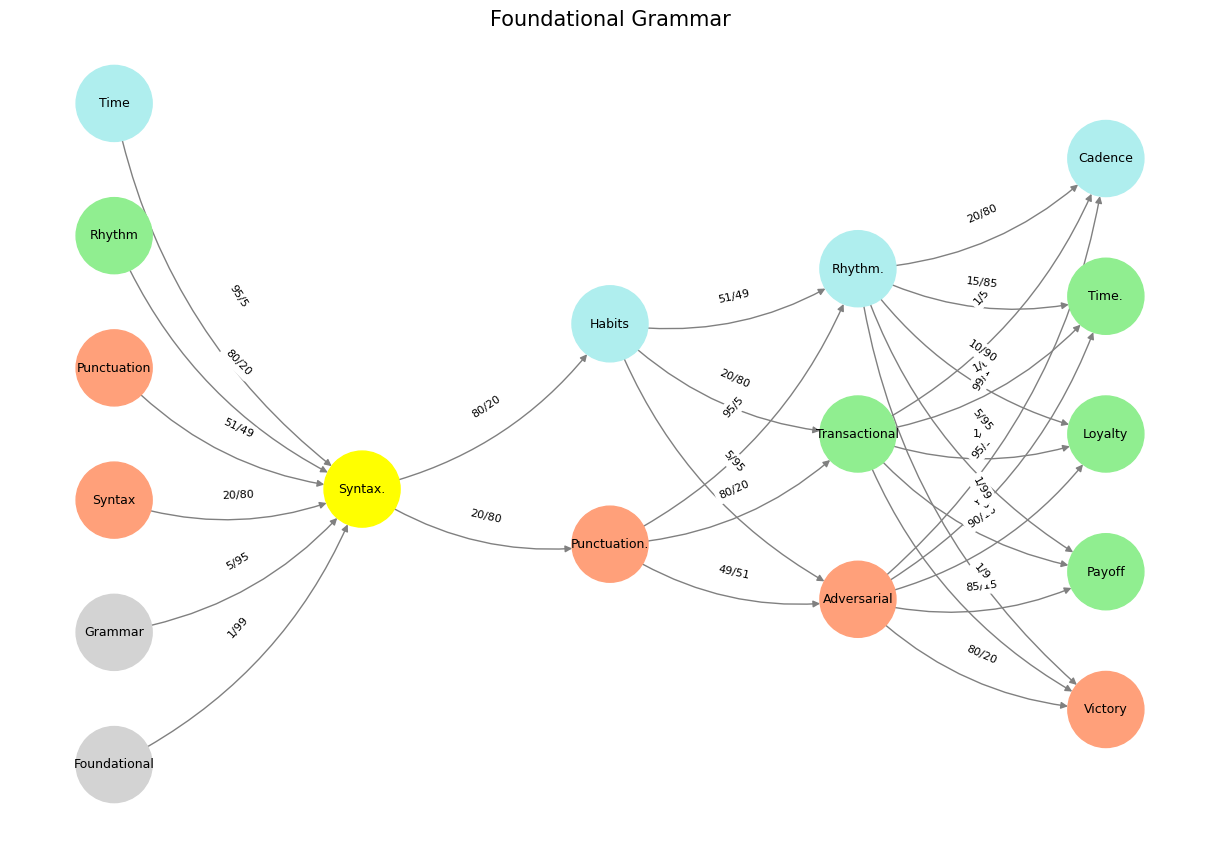
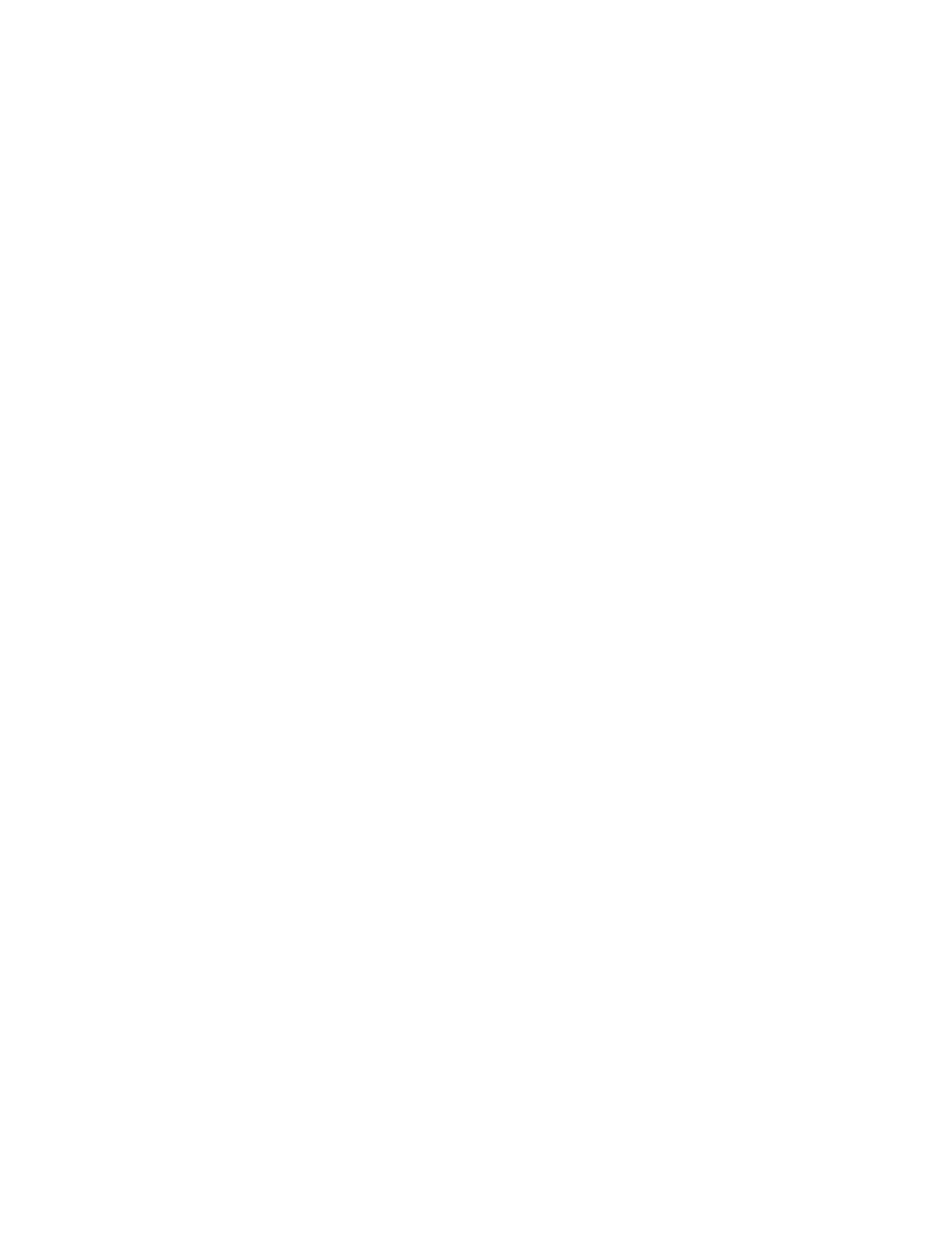
Fig. 12 Nostalgia & Romanticism. When monumental ends (victory), antiquarian means (war), and critical justification (bloodshed) were all compressed into one figure-head: hero#