Revolution#
Prosody and grammar are distinct yet interwoven elements of language, operating at different levels of structure and cognition. Grammar, in its strictest sense, consists of the rules governing syntax and morphology, defining how words combine into meaningful sentences. Prosody, on the other hand, is the rhythm, stress, and intonation of speech, shaping the way language is perceived and interpreted in real-time. Though separate, these two systems interact dynamically, with prosody influencing grammatical interpretation and grammar providing the underlying framework within which prosody operates.
Fig. 21 What Exactly is Identity. A node may represent goats (in general) and another sheep (in general). But the identity of any specific animal (not its species) is a network. For this reason we might have a “black sheep”, distinct in certain ways – perhaps more like a goat than other sheep. But that’s all dull stuff. Mistaken identity is often the fuel of comedy, usually when the adversarial is mistaken for the cooperative or even the transactional.#
The most fundamental distinction between prosody and grammar lies in their respective rule structures. Grammar is explicit, formalized, and largely symbolic—it consists of hierarchies of phrases, rules of agreement, and systematic word formation processes. It operates within discrete boundaries, such as subject-verb-object order or case marking in inflected languages. Prosody, in contrast, is fluid, continuous, and gradient. It does not function through categorical rules but rather through patterns of stress, pitch, and duration that signal emphasis, boundaries, and speaker intent. One can misplace a comma in writing and still be understood, but a misplaced prosodic emphasis in speech can completely alter meaning, sometimes to the point of ambiguity or contradiction.
Despite their differences, grammar and prosody are deeply interconnected. Syntax, for example, strongly influences prosodic phrasing. A complex sentence with embedded clauses naturally encourages pauses and tonal shifts that mirror syntactic hierarchy. Likewise, prosody serves as a disambiguating force in spoken language, resolving syntactic ambiguity that might otherwise require additional words. Consider the phrase, “She said John left yesterday.” With a prosodic pause before “yesterday,” the sentence implies that John left yesterday. Without the pause, it suggests that she made the statement yesterday. Prosody thus serves as an interpretative overlay on grammar, guiding listeners toward the intended meaning.
Prosody is also crucial to information structure—determining which elements of a sentence are foregrounded or backgrounded. Stress patterns can shift meaning without altering grammatical structure, as in “I only gave JOHN the book” versus “I only GAVE John the book.” Here, prosody alone dictates the focal point of the statement, highlighting the distinction between what is new or important and what is assumed. In written text, capitalization or italics might substitute for prosodic emphasis, but in speech, such nuances are exclusively handled through vocal modulation.
The neural underpinnings of prosody and grammar further illustrate their distinction. Brodmann’s Area 22, which is part of the superior temporal gyrus, plays a key role in processing language, but its function is lateralized between the hemispheres. The left hemisphere, dominant for language in most people, handles the fine-grained grammatical structures of syntax and morphology. It is here that the rules of sentence construction, word order, and agreement are processed. The right hemisphere, in contrast, specializes in the more holistic, global aspects of language, including prosody. It governs the interpretation of intonation, emotion, and speech melody, making it critical for understanding sarcasm, irony, and the pragmatic intent behind statements. This lateralization explains why patients with damage to the left hemisphere often struggle with grammatical processing (resulting in agrammatism), whereas damage to the right hemisphere can impair the ability to recognize emotional tone or differentiate between a statement and a question when prosody is the only distinguishing feature.
Ultimately, while grammar provides the scaffolding of language, prosody gives it texture and nuance. A rigidly grammatical sentence can be utterly ambiguous without prosody, just as a richly inflected prosodic pattern may be incomprehensible without grammatical coherence. Language is a symphony of structure and sound, with the left and right hemispheres of the brain working in concert to produce meaning. Without prosody, speech would be monotonous and lifeless; without grammar, it would be chaos. Their interplay is what makes human language not just a system of rules but a living, expressive medium.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Foundational', 'Grammar', 'Syntax', 'Punctuation', "Rhythm", 'Time'], # Static
'Voir': ['Syntax.'],
'Choisis': ['Punctuation.', 'Melody'],
'Deviens': ['Adversarial', 'Transactional', 'Rhythm.'],
"M'èléve": ['Victory', 'Payoff', 'Loyalty', 'Time.', 'Cadence']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Syntax.'],
'paleturquoise': ['Time', 'Melody', 'Rhythm.', 'Cadence'],
'lightgreen': ["Rhythm", 'Transactional', 'Payoff', 'Time.', 'Loyalty'],
'lightsalmon': ['Syntax', 'Punctuation', 'Punctuation.', 'Adversarial', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Foundational', 'Syntax.'): '1/99',
('Grammar', 'Syntax.'): '5/95',
('Syntax', 'Syntax.'): '20/80',
('Punctuation', 'Syntax.'): '51/49',
("Rhythm", 'Syntax.'): '80/20',
('Time', 'Syntax.'): '95/5',
('Syntax.', 'Punctuation.'): '20/80',
('Syntax.', 'Melody'): '80/20',
('Punctuation.', 'Adversarial'): '49/51',
('Punctuation.', 'Transactional'): '80/20',
('Punctuation.', 'Rhythm.'): '95/5',
('Melody', 'Adversarial'): '5/95',
('Melody', 'Transactional'): '20/80',
('Melody', 'Rhythm.'): '51/49',
('Adversarial', 'Victory'): '80/20',
('Adversarial', 'Payoff'): '85/15',
('Adversarial', 'Loyalty'): '90/10',
('Adversarial', 'Time.'): '95/5',
('Adversarial', 'Cadence'): '99/1',
('Transactional', 'Victory'): '1/9',
('Transactional', 'Payoff'): '1/8',
('Transactional', 'Loyalty'): '1/7',
('Transactional', 'Time.'): '1/6',
('Transactional', 'Cadence'): '1/5',
('Rhythm.', 'Victory'): '1/99',
('Rhythm.', 'Payoff'): '5/95',
('Rhythm.', 'Loyalty'): '10/90',
('Rhythm.', 'Time.'): '15/85',
('Rhythm.', 'Cadence'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with weights
for (source, target), weight in edges.items():
if source in G.nodes and target in G.nodes:
G.add_edge(source, target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("Grammar is the Ecosystem", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
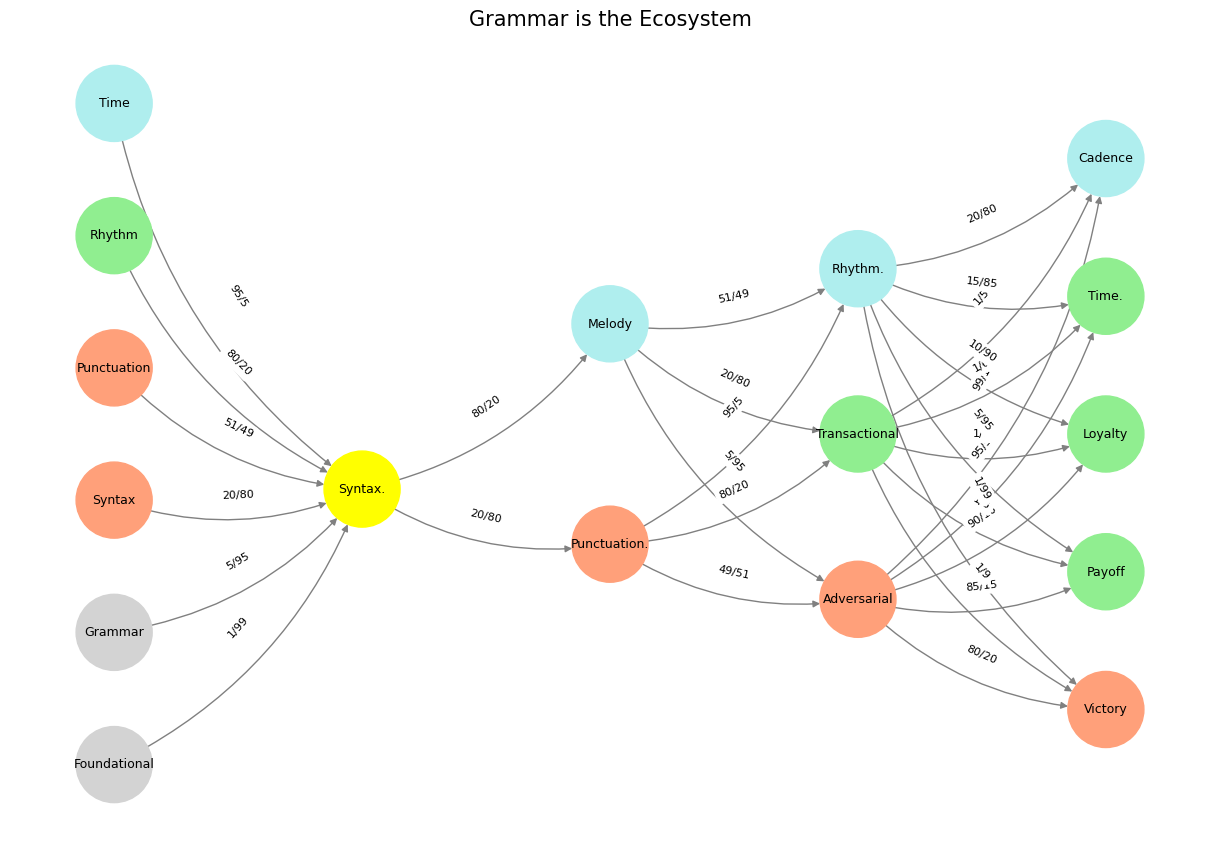

Fig. 22 Francis Bacon. He famously stated “If a man will begin with certainties, he shall end in doubts; but if he will be content to begin with doubts, he shall end in certainties.” This quote is from The Advancement of Learning (1605), where Bacon lays out his vision for empirical science and inductive reasoning. He argues that starting with unquestioned assumptions leads to instability and confusion, whereas a methodical approach that embraces doubt and inquiry leads to true knowledge. This aligns with his broader Novum Organum (1620), where he develops the Baconian method, advocating for systematic observation, experimentation, and the gradual accumulation of knowledge rather than relying on dogma or preconceived notions.#