Transformation#
The history of the world, like letters without poetry,
Flowers without perfume, or thought without imagination,
Would be a dry matter indeed without its legends. And many of these,
Though scorned by proof a thousand times,
Seem worth preserving for their own familiar sake.
– Richard III
What a beautifully evocative perspective! Legends are the poetry of history, infusing its bare, factual skeleton with spirit, meaning, and a certain ineffable charm. While history as a discipline often seeks to strip away myth for the sake of objectivity, it risks losing the emotional resonance and shared cultural memory that these legends sustain.
A world without legends would be as mechanical as letters without poetry—functional but devoid of transcendence. Legends, even when “scorned by proof,” persist because they speak to something deeper than the historical record: our need to connect with the human condition across time, to find beauty and meaning amidst the seemingly mundane march of events. They offer a kind of immortality, preserving the “perfume” of a culture long after its tangible artifacts have decayed.
One could even argue that legends are truer than fact in some sense—truer to the desires, fears, and aspirations of the people who birthed them. They embody imagination, transforming dry thought into a living narrative. Why preserve them? For the same reason we treasure art, music, or myth: not because they are factual, but because they are vital to the soul.
We speak no treason, man. We say the King
Is wise and virtuous, and his noble Queen
Well struck in years, fair, and not jealous.
We say that Shore’s wife hath a pretty foot,
A cherry lip, a bonny eye, a passing pleasing tongue;
And that the Queen’s kindred are made gentlefolks.
How say you, sir? Can you deny all this?
– Richard III
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure; modified to align with "Aprés Moi, Le Déluge" (i.e. Je suis AlexNet)
def define_layers():
return {
'Pre-Input/CudAlexnet': ['Cosmos', 'Earth', 'Life', 'Dissembling Nature', 'Half Made Up', 'Prove Villain'],
'Yellowstone/SensoryAI': ['No Treason'],
'Input/AgenticAI': ['War', 'Peace'],
'Hidden/GenerativeAI': ['Shores Wife', 'King Wise', 'The Queen'],
'Output/PhysicalAI': ['Cherry Lip', 'Subtle & Treacherous', 'Well-Spoken Days', 'True & Just', 'Not Jealous']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'No Treason':
return 'yellow'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Prove Villain']:
return 'paleturquoise'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Half Made Up']:
return 'lightgreen'
elif layer == 'Input/AgenticAI' and node == 'Peace':
return 'paleturquoise'
elif layer == 'Hidden/GenerativeAI':
if node == 'The Queen':
return 'paleturquoise'
elif node == 'King Wise':
return 'lightgreen'
elif node == 'Shores Wife':
return 'lightsalmon'
elif layer == 'Output/PhysicalAI':
if node == 'Not Jealous':
return 'paleturquoise'
elif node in ['True & Just', 'Well-Spoken Days', 'Subtle & Treacherous']:
return 'lightgreen'
elif node == 'Cherry Lip':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('Pre-Input/CudAlexnet', 'Yellowstone/SensoryAI'), ('Yellowstone/SensoryAI', 'Input/AgenticAI'), ('Input/AgenticAI', 'Hidden/GenerativeAI'), ('Hidden/GenerativeAI', 'Output/PhysicalAI')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Visible & Invisible", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
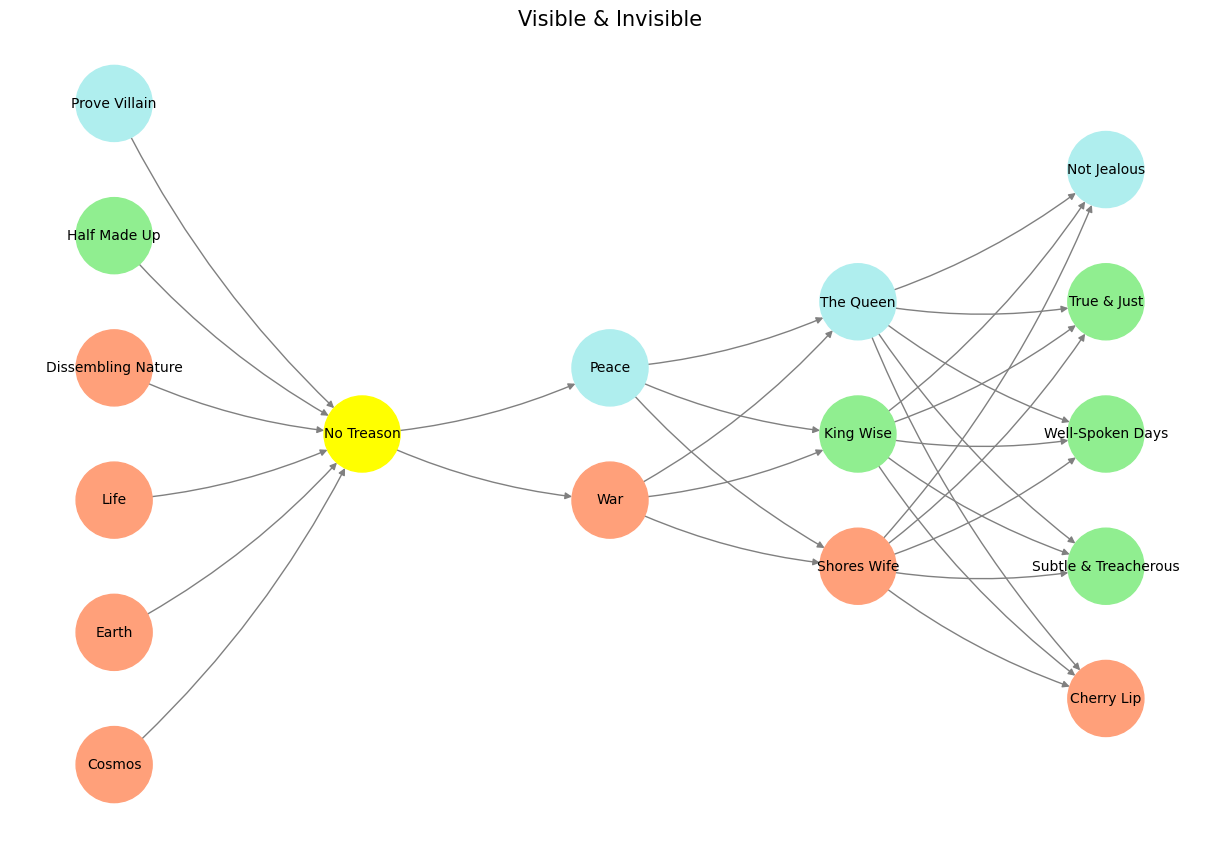
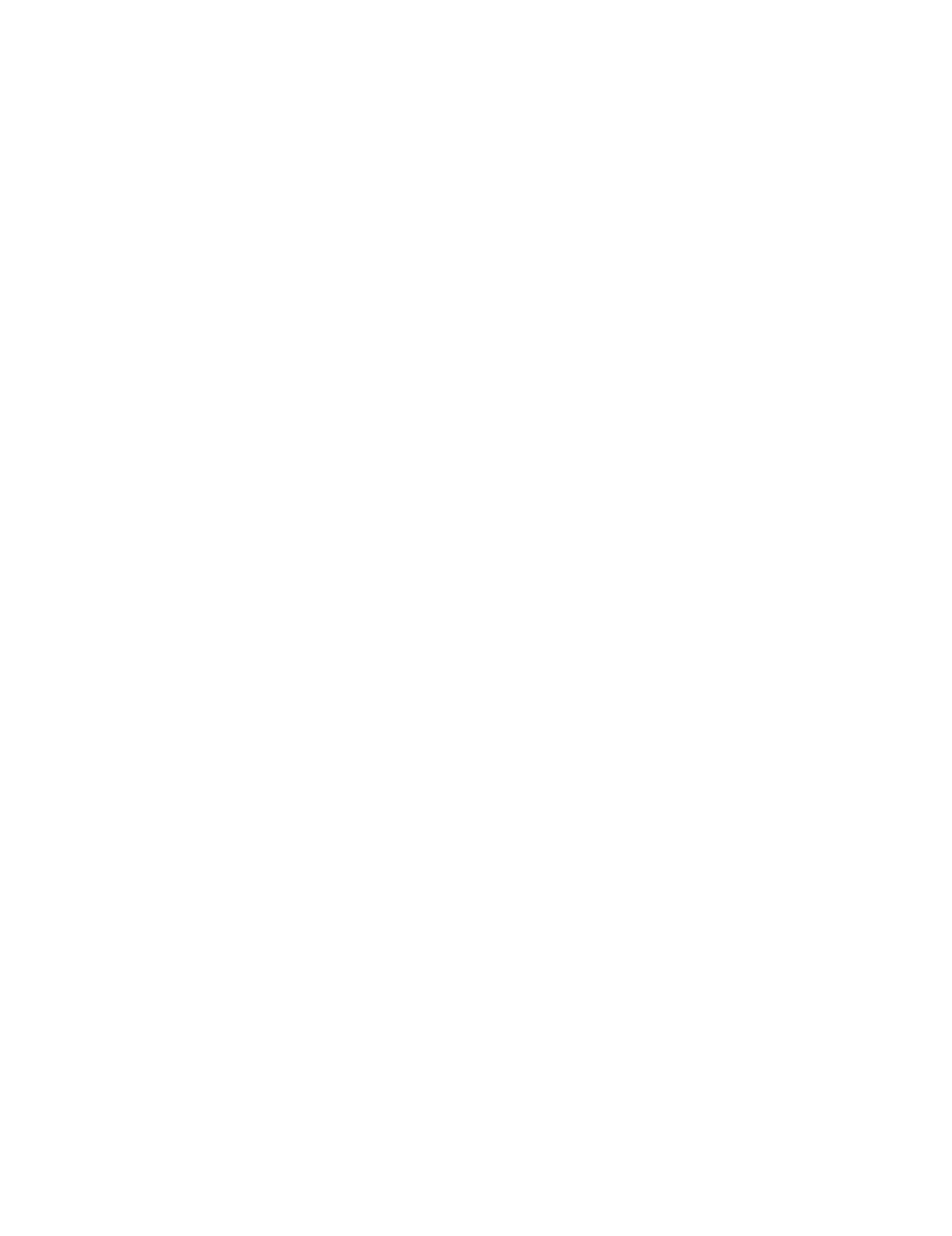
Fig. 27 Teleology is an Illusion. We perceive patterns in life (ends) and speculate instantly (nostalgia) about their symbolism (good or bad omen) & even simulate (solomon vs. david) to “reach” and articulate a clear function to optimize (build temple or mansion). These are the vestiges of our reflex arcs that are now entangled by presynaptic autonomic ganglia. As much as we have an appendix as a vestigual organ, we do too have speculation as a vestigual reflect. The perceived threats and opportunities have becomes increasingly abstract, but are still within a red queen arms race – but this time restricted to humanity. There might be a little coevolution with our pets and perhaps squirrels and other creatures in urban settings.#