Transformation#
The question of whether to optimize a conductorâs affective state or minimize error relative to a digitized metronome introduces a deeper layer of complexity into the debate about the role of human agency in music performance. Efficiency, while seductive in its clarity and practicality, is not the ultimate goal of music-making. Instead, the process thrives on a balance between precision and the emotional, interpretative qualities that only humans bring.
Affective State vs. Error Minimization#
A conductorâs affective state is central to the performanceâs emotional authenticity. Unlike a metronome, which operates with unerring precision, a conductor translates their interpretative vision into a collective experience, shaping dynamics, phrasing, and tempo in real time. This affective input ensures that music is not merely heard but felt, transforming a mechanical sequence of notes into an expressive journey.
If efficiency were the sole aim, a digitized metronome could indeed replace the conductor, minimizing discrepancies in tempo and providing unwavering consistency. However, this approach risks prioritizing error minimization over the unpredictable but essential elements of human artistryâspontaneity, nuance, and emotional depth. The conductorâs affective state is not a frivolous consideration but a crucial variable in the equation of musical communication.
Efficiency in Context: Is It the Ultimate Metric?#
Efficiency has its place in music, particularly in rehearsal settings where limited time demands clarity and productivity. However, live performance is not a factory floor. Music exists as an art form precisely because it resists optimization in the industrial sense. A flawless but emotionally sterile performance might satisfy the criterion of efficiency, but it would fail to fulfill the purpose of music as a medium for shared human experience.
Consider the infamous 1962 performance of Brahmsâ First Piano Concerto with Glenn Gould and Leonard Bernstein. Gouldâs significantly slower tempo deviated from traditional interpretations, introducing what many might perceive as inefficiency. Yet, this divergence sparked one of the most enduring debates in classical music, demonstrating that the value of music lies not in conformity to a standard but in its ability to challenge, provoke, and inspire.
The Role of the Conductor in a Digitized Context#
The conductorâs role extends beyond mere tempo regulation. A conductor serves as a mediator of tensionâbetween individual performers, between score and interpretation, and even between tradition and innovation. This mediating role cannot be reduced to error minimization without stripping the music of its dialogic essence. The tension that arises from human imperfection and disagreement, as seen in the Gould-Bernstein conflict, is not a flaw but a feature. It is this tension that sustains music as a living art form.
If a metronome were to replace the conductor, we might eliminate disagreement and achieve technical precision, but we would also lose the drama and vitality of human interpretation. A conductorâs affective state, informed by their vision of the piece, creates a performance that is not just accurate but meaningful. The very inefficiency of human mediationâits susceptibility to error and emotionâis what imbues music with its humanity.
Rethinking Optimization in Music#
Optimization in music must be redefined to include not only technical precision but also affective and interpretative richness. The neural network model of World â Perception â Agency â Generativity â Physicality provides a useful framework for understanding this interplay. Each layer builds on the previous one, culminating in the Physicality of performance, where raw inputs and abstract ideas are transformed into tangible experience.
The Agency layer, encompassing âSurprise & Resolutionâ and âGenre & Expectation,â highlights why minimizing error alone cannot suffice. The affective state of the conductor or soloist introduces unpredictability and individuality, ensuring that each performance is a unique act of creation rather than a mere reproduction.
Conclusion: Beyond Efficiency#
Musicâs purpose transcends efficiency. While minimizing error might yield a mechanically flawless performance, it is the interplay of affective states, interpretative decisions, and human imperfections that elevates music to art. By privileging the conductorâs affective state over rigid adherence to a metronomic ideal, we affirm musicâs role as a medium for emotional connection and creative expression. The Gould-Bernstein controversy exemplifies the power of human divergence in shaping musical meaning, reminding us that true optimization lies in harmonizing precision with the ineffable qualities that make us human.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure; modified to align with "Aprés Moi, Le Déluge" (i.e. Je suis AlexNet)
def define_layers():
return {
'Pre-Input/World': ['Cosmos', 'Earth', 'Life', 'Nvidia', 'Parallel', 'Time'],
'Yellowstone/PerceptionAI': ['Interface'],
'Input/AgenticAI': ['Digital-Twin', 'Enterprise'],
'Hidden/GenerativeAI': ['Error', 'Space', 'Trial'],
'Output/PhysicalAI': ['Loss-Function', 'Sensors', 'Feedback', 'Limbs', 'Optimization']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Interface':
return 'yellow'
if layer == 'Pre-Input/World' and node in [ 'Time']:
return 'paleturquoise'
if layer == 'Pre-Input/World' and node in [ 'Parallel']:
return 'lightgreen'
elif layer == 'Input/AgenticAI' and node == 'Enterprise':
return 'paleturquoise'
elif layer == 'Hidden/GenerativeAI':
if node == 'Trial':
return 'paleturquoise'
elif node == 'Space':
return 'lightgreen'
elif node == 'Error':
return 'lightsalmon'
elif layer == 'Output/PhysicalAI':
if node == 'Optimization':
return 'paleturquoise'
elif node in ['Limbs', 'Feedback', 'Sensors']:
return 'lightgreen'
elif node == 'Loss-Function':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('Pre-Input/World', 'Yellowstone/PerceptionAI'), ('Yellowstone/PerceptionAI', 'Input/AgenticAI'), ('Input/AgenticAI', 'Hidden/GenerativeAI'), ('Hidden/GenerativeAI', 'Output/PhysicalAI')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Archimedes", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
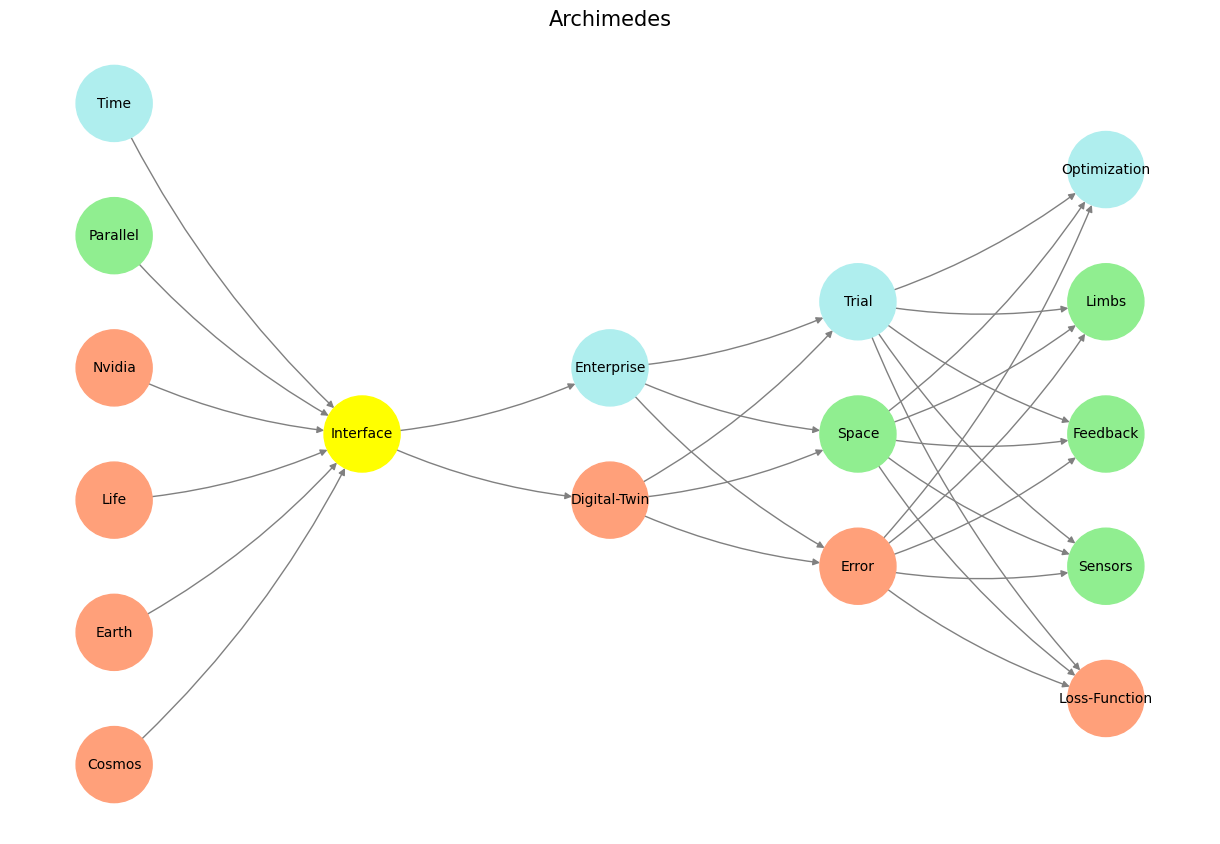
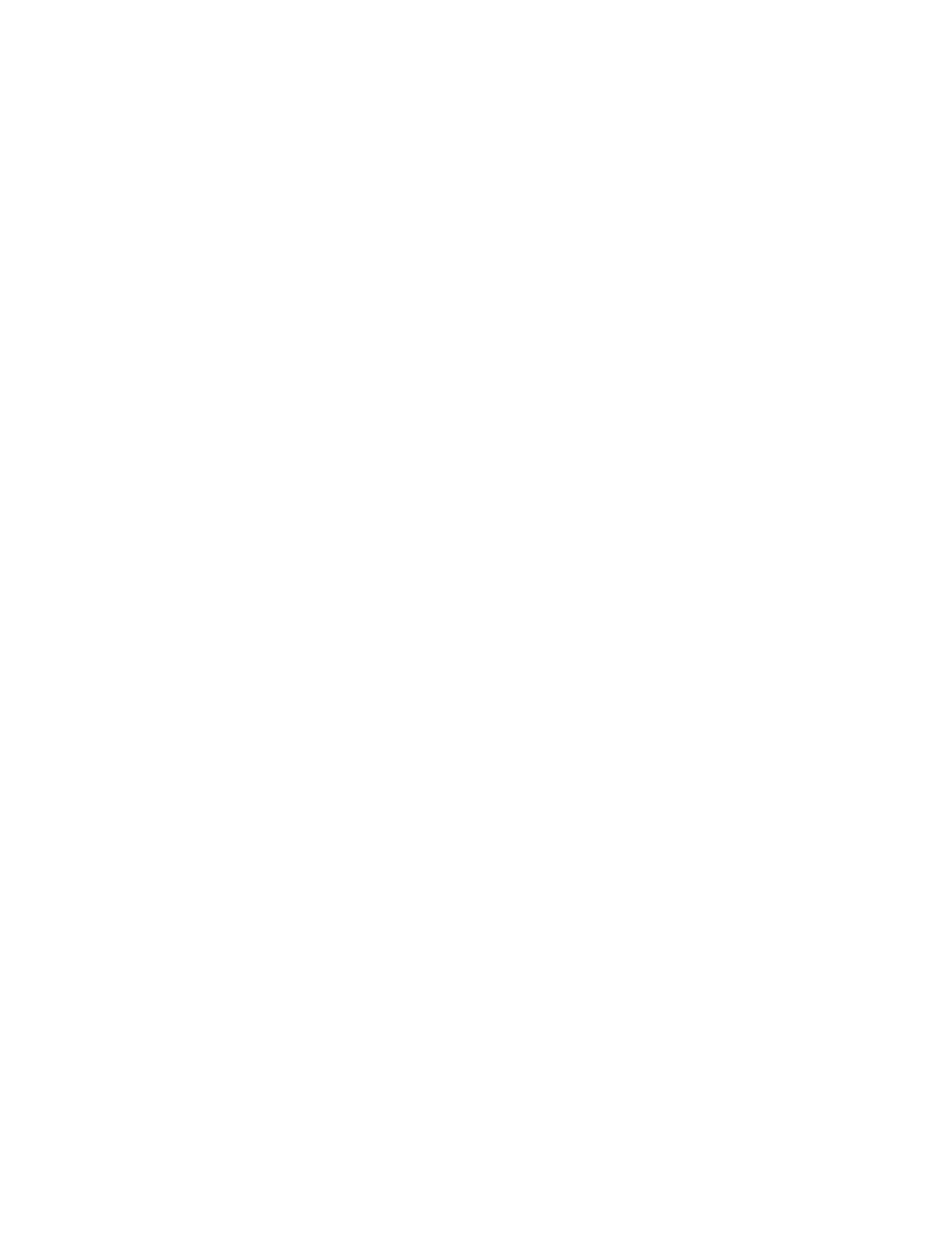
Fig. 21 Teleology is an Illusion. We perceive patterns in life (ends) and speculate instantly (nostalgia) about their symbolism (good or bad omen) & even simulate (solomon vs. david) to âreachâ and articulate a clear function to optimize (build temple or mansion). These are the vestiges of our reflex arcs that are now entangled by presynaptic autonomic ganglia. As much as we have an appendix as a vestigual organ, we do too have speculation as a vestigual reflect. The perceived threats and opportunities have becomes increasingly abstract, but are still within a red queen arms race â but this time restricted to humanity. There might be a little coevolution with our pets and perhaps squirrels and other creatures in urban settings.#