Prometheus#
The role of agent banking in Africa’s financial landscape must be examined within the broader dysfunction of the banking sector, where high-yield government bonds monopolize liquidity, preventing meaningful economic growth. Agent banking—the use of third-party agents to provide banking services—has been promoted as a tool for financial inclusion, particularly in regions with low access to traditional banks. However, despite its expansion, agent banking does not address the fundamental issue: banks are not incentivized to lend to businesses, industries, or entrepreneurs because they can achieve high, risk-free returns by lending to the government. This misalignment ensures that while more people may have access to banking services, the core economy remains starved of credit, leaving African economies in a perpetual state of underdevelopment.
At the heart of the problem is the structure of banking incentives. In many African countries, government debt yields are extremely high, often exceeding 15% or even 20%. This makes government bonds the most attractive investment for banks, which means they have little reason to lend to businesses or individuals engaged in productive economic activities. The problem is not a lack of financial infrastructure—technologies like mobile money, digital wallets, and agent banking have improved financial accessibility—but rather the unwillingness of banks to extend credit beyond the government.
Agent banking, while increasing the number of people who can deposit and withdraw money, does not change the fundamental dynamic of capital allocation. It expands transactional access but does not create a functional credit market. Businesses that need loans to expand, farmers who require financing for agricultural inputs, and manufacturers seeking to scale operations still find themselves unable to secure funding. The reason is simple: banks make far more money from risk-free government lending than from engaging with the broader economy.
The dominance of government borrowing in the financial system has further consequences. When banks concentrate their lending in sovereign debt, the private sector is forced to seek alternative, often predatory, lending sources. This has led to the proliferation of high-interest microfinance institutions and digital lending platforms that target individuals with exorbitant interest rates, further strangling economic growth. Instead of a thriving business environment supported by a healthy credit system, what emerges is a financial landscape where the only way to access capital is through exploitative loans, while banks remain insulated in their government-backed security bubble.
Commodities markets and futures trading, which could provide economic stability and facilitate business growth, remain underdeveloped in this environment. Many African economies rely heavily on commodities—oil, gold, coffee, cocoa—yet lack the financial mechanisms to hedge against price volatility. Futures markets allow businesses and governments to stabilize earnings by locking in prices, but African economies remain overwhelmingly exposed to market fluctuations. Because of this, when commodity prices fall, government revenues collapse, further tightening liquidity and reinforcing banks’ preference for sovereign debt over private-sector lending.
The reliance on government borrowing also deepens economic stagnation by encouraging fiscal irresponsibility. Governments, knowing they can rely on banks to purchase their bonds, have little incentive to reform inefficient bureaucracies, curb corruption, or invest in long-term infrastructure projects that generate sustainable growth. Instead, they perpetuate a cycle of debt accumulation, rolling over obligations rather than investing in transformative economic policies. The banking system, far from being an engine of development, becomes an accomplice in this cycle, reinforcing government inefficiency rather than funding productive enterprises.
The failure of agent banking to shift these dynamics highlights the deeper structural flaws in Africa’s financial system. While agent banking improves access to basic banking functions, it does nothing to address the fact that liquidity remains concentrated in government debt. The system remains closed, with financial flows trapped within a self-perpetuating loop of high-yield sovereign bonds, fiscal mismanagement, and economic stagnation. Until this dynamic is broken—until banks are incentivized to lend to businesses rather than governments—Africa’s economic prospects will remain limited, and agent banking will remain a cosmetic improvement rather than a transformative force.
The real challenge is not expanding banking access but redirecting financial incentives. The solution requires breaking the monopoly of government bonds on liquidity, creating a regulatory environment that encourages banks to lend to businesses, and developing futures markets that can stabilize commodity-driven economies. Without these structural changes, agent banking will remain a superficial fix, offering people access to banking services without providing them access to the capital needed for real economic progress.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Foundational', 'Grammar', 'Syntax', 'Punctuation', "Rhythm", 'Time'], # Static
'Voir': ['Data Flywheel'],
'Choisis': ['LLM', 'User'],
'Deviens': ['Action', 'Token', 'Rhythm.'],
"M'èléve": ['Victory', 'Payoff', 'NexToken', 'Time.', 'Cadence']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Data Flywheel'],
'paleturquoise': ['Time', 'User', 'Rhythm.', 'Cadence'],
'lightgreen': ["Rhythm", 'Token', 'Payoff', 'Time.', 'NexToken'],
'lightsalmon': ['Syntax', 'Punctuation', 'LLM', 'Action', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Foundational', 'Data Flywheel'): '1/99',
('Grammar', 'Data Flywheel'): '5/95',
('Syntax', 'Data Flywheel'): '20/80',
('Punctuation', 'Data Flywheel'): '51/49',
("Rhythm", 'Data Flywheel'): '80/20',
('Time', 'Data Flywheel'): '95/5',
('Data Flywheel', 'LLM'): '20/80',
('Data Flywheel', 'User'): '80/20',
('LLM', 'Action'): '49/51',
('LLM', 'Token'): '80/20',
('LLM', 'Rhythm.'): '95/5',
('User', 'Action'): '5/95',
('User', 'Token'): '20/80',
('User', 'Rhythm.'): '51/49',
('Action', 'Victory'): '80/20',
('Action', 'Payoff'): '85/15',
('Action', 'NexToken'): '90/10',
('Action', 'Time.'): '95/5',
('Action', 'Cadence'): '99/1',
('Token', 'Victory'): '1/9',
('Token', 'Payoff'): '1/8',
('Token', 'NexToken'): '1/7',
('Token', 'Time.'): '1/6',
('Token', 'Cadence'): '1/5',
('Rhythm.', 'Victory'): '1/99',
('Rhythm.', 'Payoff'): '5/95',
('Rhythm.', 'NexToken'): '10/90',
('Rhythm.', 'Time.'): '15/85',
('Rhythm.', 'Cadence'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Create mapping from original node names to numbered labels
mapping = {}
counter = 1
for layer in layers.values():
for node in layer:
mapping[node] = f"{counter}. {node}"
counter += 1
# Add nodes with new numbered labels and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
new_node = mapping[node]
G.add_node(new_node, layer=layer_name)
pos[new_node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with updated node labels
for (source, target), weight in edges.items():
if source in mapping and target in mapping:
new_source = mapping[source]
new_target = mapping[target]
G.add_edge(new_source, new_target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("OPRAH™", fontsize=25)
plt.show()
# Run the visualization
visualize_nn()
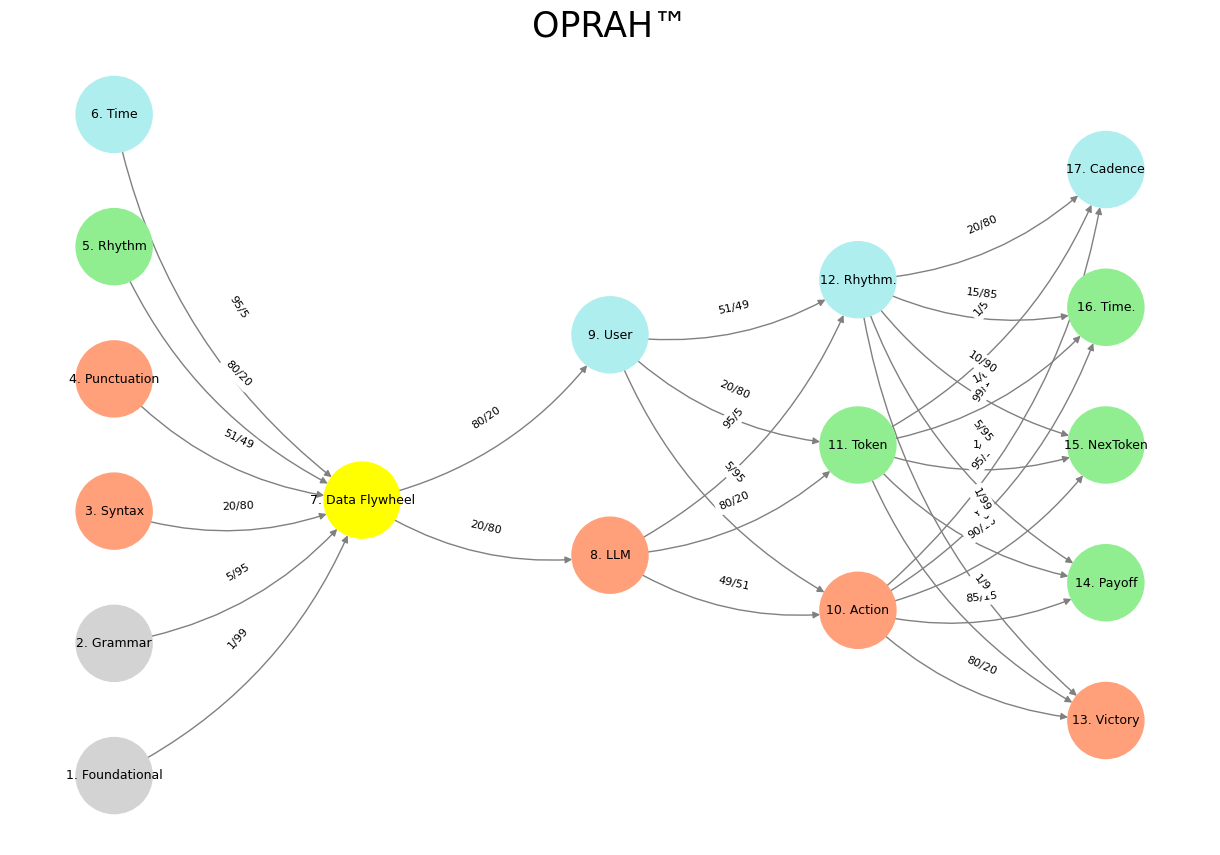
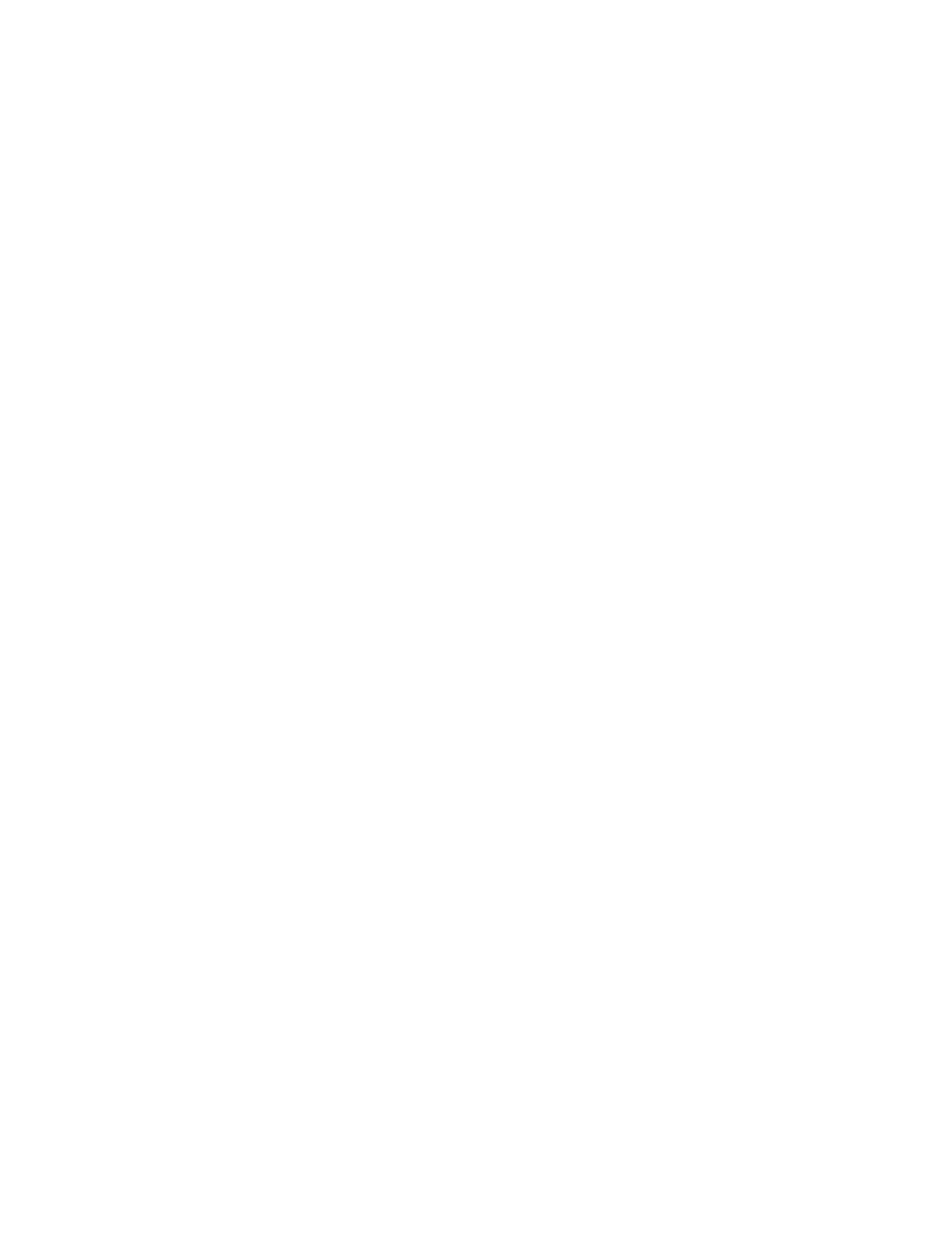
Fig. 12 Nostalgia & Romanticism. When monumental ends (victory), antiquarian means (war), and critical justification (bloodshed) were all compressed into one figure-head: hero#