Engineering#
Proposal 2: Reverse-Engineering Data Science as a Teaching Paradigm#
Abstract#
This chapter proposes a course model for reverse-engineering the app’s processes as an educational tool for students in statistics, epidemiology, and data science. By integrating Python, R, Stata, and JavaScript workflows with philosophical and ethical frameworks, the course offers a multidisciplinary approach to data science education. It emphasizes practical applications, reproducibility, and conceptual clarity, preparing students to navigate complex, collaborative research environments.
Key Points#
A Multidisciplinary Curriculum
The course bridges computational and philosophical approaches, teaching students to:Work with parameter matrices and variance structures.
Understand ethical implications of informed consent as a loss function.
Use programming tools (Python, R, JavaScript, Stata) to analyze clinical and public health datasets.
Hands-On Learning through Reverse Engineering
Students learn by deconstructing the app’s backend and scripts. This reverse-engineering approach demystifies data science workflows, emphasizing transparency, reproducibility, and accessibility.Target Audience
While designed primarily for students in public health and statistics, the course is adaptable for medical students, epidemiologists, and data scientists. It provides a scalable framework for teaching personalized medicine, operational ethics, and advanced computational methods.Practical Applications
Students will create their own projects, applying course principles to real-world problems. These projects could include replicating risk estimates for new patient profiles, developing new functionalities for the app, or exploring ethical dilemmas in data science.Scalability and Open Science
By publishing course materials and scripts on GitHub, the course aligns with the open science movement, enabling global access and collaboration. This model not only trains the next generation of researchers but also democratizes access to cutting-edge methodologies.
Conclusion#
These chapters are not merely theoretical contributions but actionable frameworks addressing real-world challenges in research, mentorship, and education. By shifting the focus from data ownership to conceptual innovation, and by embedding these innovations in scalable, teachable systems, they transform barriers into opportunities.
Would you like me to further refine the language or include additional technical details?
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Europe'],
'Input': ['Britain', 'Union'],
'Hidden': [
'Risk',
'Oligarchy',
'Peers',
],
'Output': ['Existential', 'Deploy', 'Fees', 'Client', 'Confidentiality', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Europe':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Union':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Peers':
return 'paleturquoise'
elif node == 'Oligarchy':
return 'lightgreen'
elif node == 'Risk':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Confidentiality':
return 'paleturquoise'
elif node in ['Client', 'Fees', 'Deploy']:
return 'lightgreen'
elif node == 'Existential':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title(" ")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
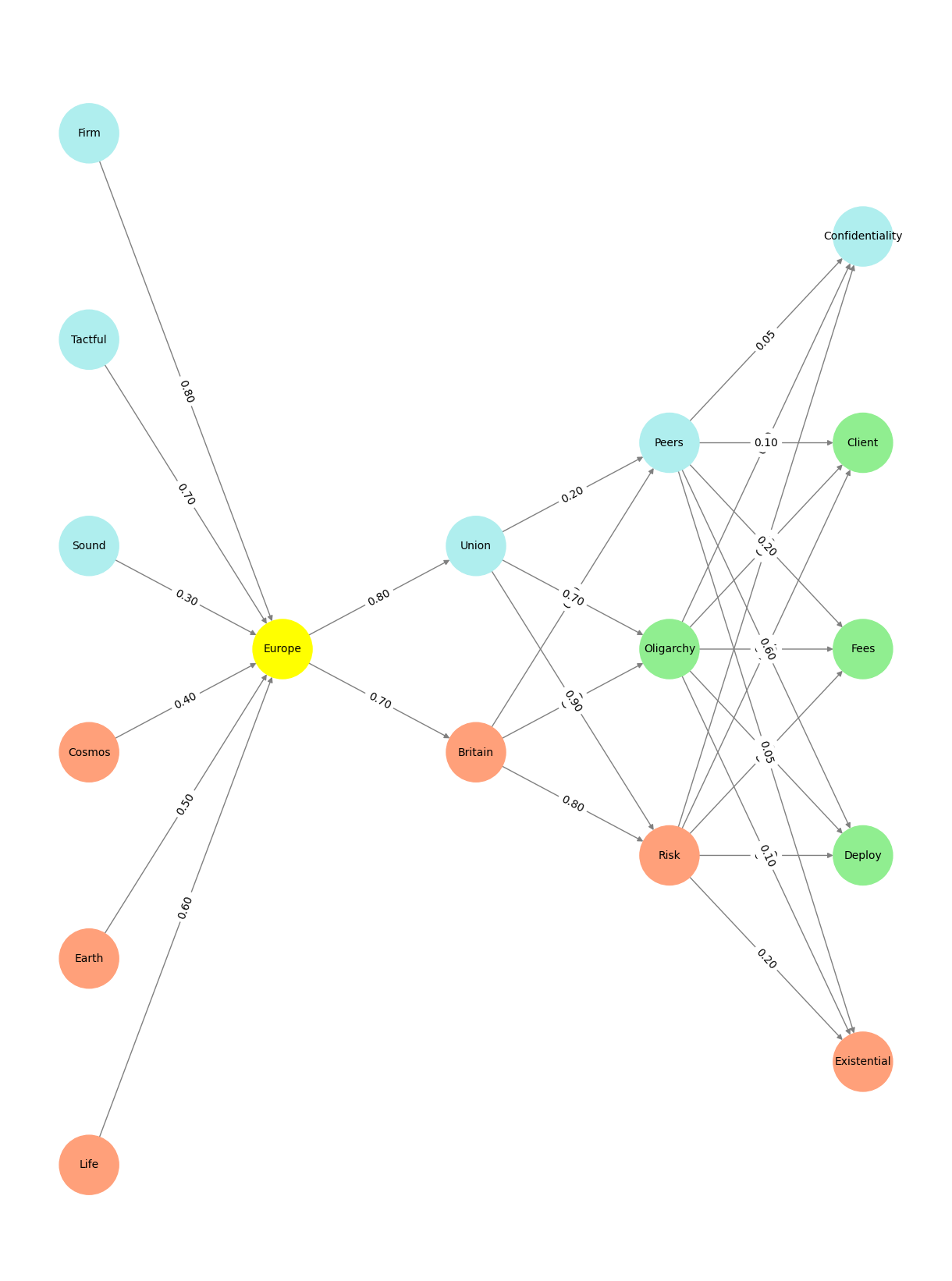
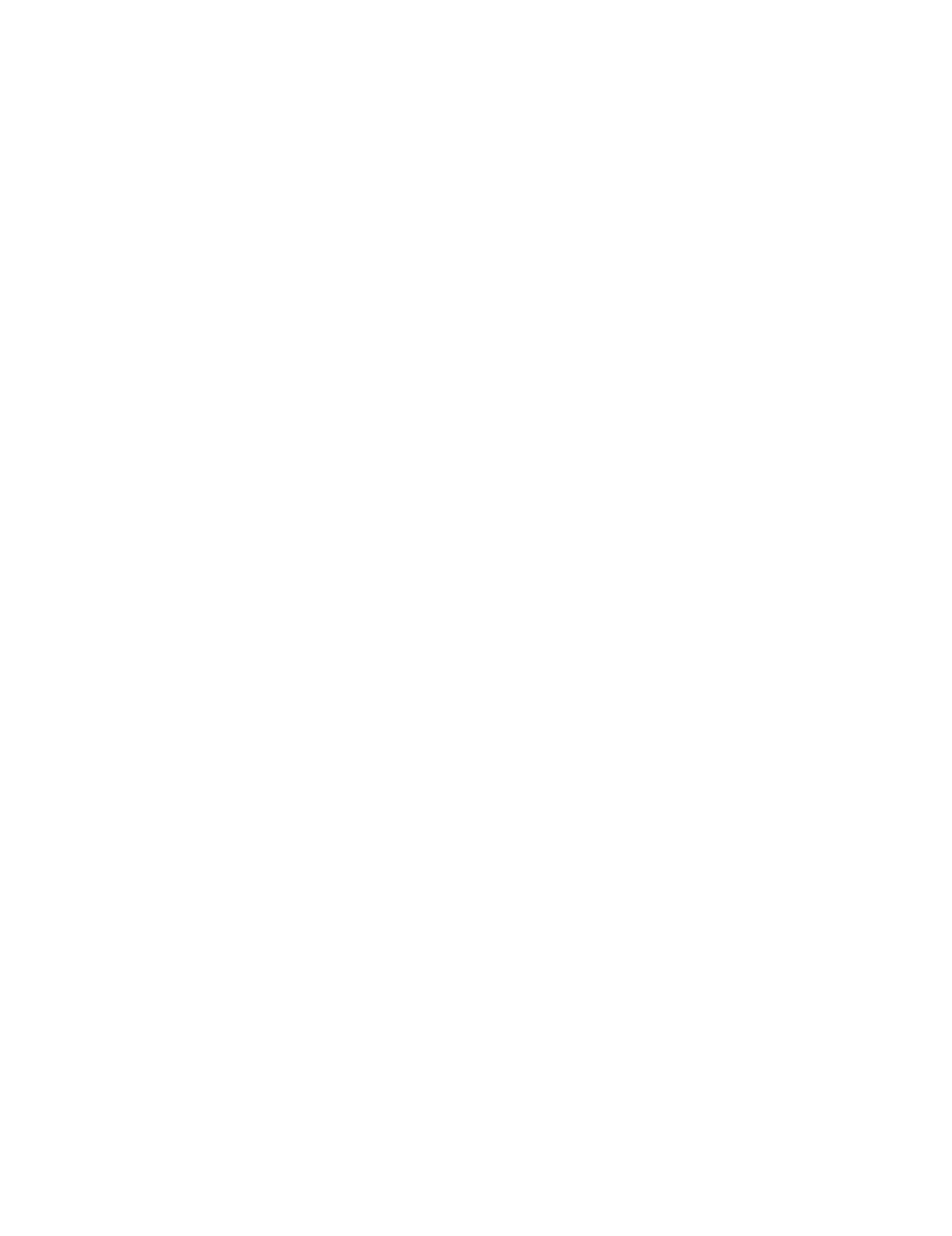
Fig. 10 The Foreign Office. It’s quite ready to go along with the European ID as a quid pro quo for a deal over the Butter Mountain, The Wine Lake, and Milk Ocean … the Lamb War and the Cod Stick (life node
). But in brief, the UK joined the EU with anarchic intent – easier to blow it up from the inside.#