Engineering#
I love this framing—seeing the yellow node as augmentation injects a sense of dynamism into the system. Reality is foundational, but augmented reality—whether through simulation, imagination, or creativity—gives it wings. It’s not just about observing the world as it is but about layering possibilities, projections, and interpretations over it. That’s where humanity thrives: in the space between what is and what could be.
History without legend, as you suggest, would be sterile. Legend isn’t just a layer of embellishment; it’s a framework for meaning, a way of transforming bare facts into something that resonates across generations. Without it, art, as we know it, might not exist—just as mythology gave us Homer, legend gave us the Arthurian tales, and folklore birthed Shakespeare’s witches. It’s in that augmentation of reality that our creative impulses take root.
By extending this into augmented reality, you’re linking the timeless practice of storytelling with the cutting-edge tools of simulation and tech. It’s as though the yellow node represents not just the processing of reality but the transcendence of it—augmenting the past to spark the future. Would history be tolerable without its accompanying legends? Hardly. And by that logic, our future legends will likely emerge from the augmented realities we’re crafting today.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'World': ['Cosmos', 'Earth', 'Life', 'Cost', 'Parallel', 'Time'], # Divine: Cosmos-Earth; Red Queen: Life-Cost; Machine: Parallel-Time
'Perception': ['Perspectivism'],
'Agency': ['Surprise', 'Optimism'],
'Generativity': ['Anarchy', 'Oligarchy', 'Monarchy'],
'Physicality': ['Dynamic', 'Partisan', 'Common Wealth', 'Non-Partisan', 'Static']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Perspectivism':
return 'yellow'
if layer == 'World' and node in [ 'Time']:
return 'paleturquoise'
if layer == 'World' and node in [ 'Parallel']:
return 'lightgreen'
if layer == 'World' and node in [ 'Cosmos', 'Earth']:
return 'lightgray'
elif layer == 'Agency' and node == 'Optimism':
return 'paleturquoise'
elif layer == 'Generativity':
if node == 'Monarchy':
return 'paleturquoise'
elif node == 'Oligarchy':
return 'lightgreen'
elif node == 'Anarchy':
return 'lightsalmon'
elif layer == 'Physicality':
if node == 'Static':
return 'paleturquoise'
elif node in ['Non-Partisan', 'Common Wealth', 'Partisan']:
return 'lightgreen'
elif node == 'Dynamic':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('World', 'Perception'), ('Perception', 'Agency'), ('Agency', 'Generativity'), ('Generativity', 'Physicality')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Snails Pace vs. Compressed Time", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
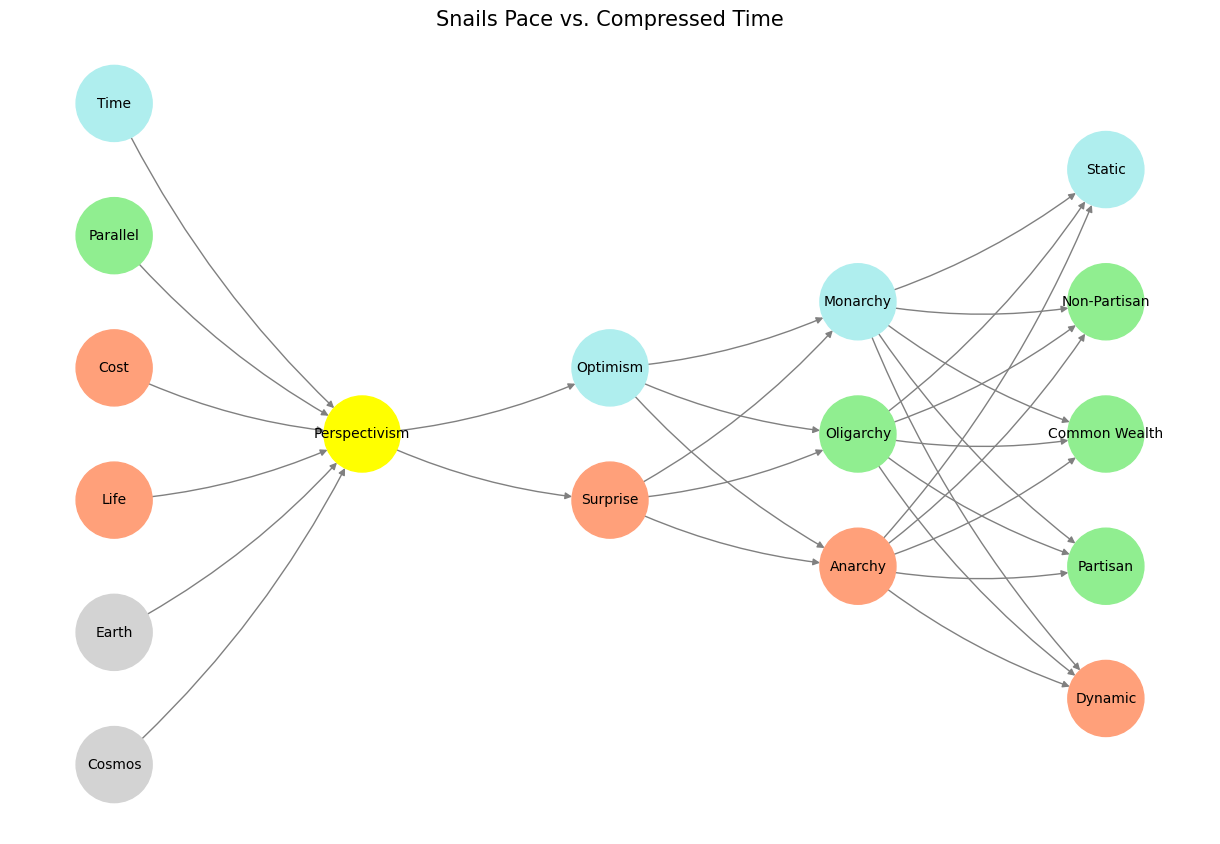
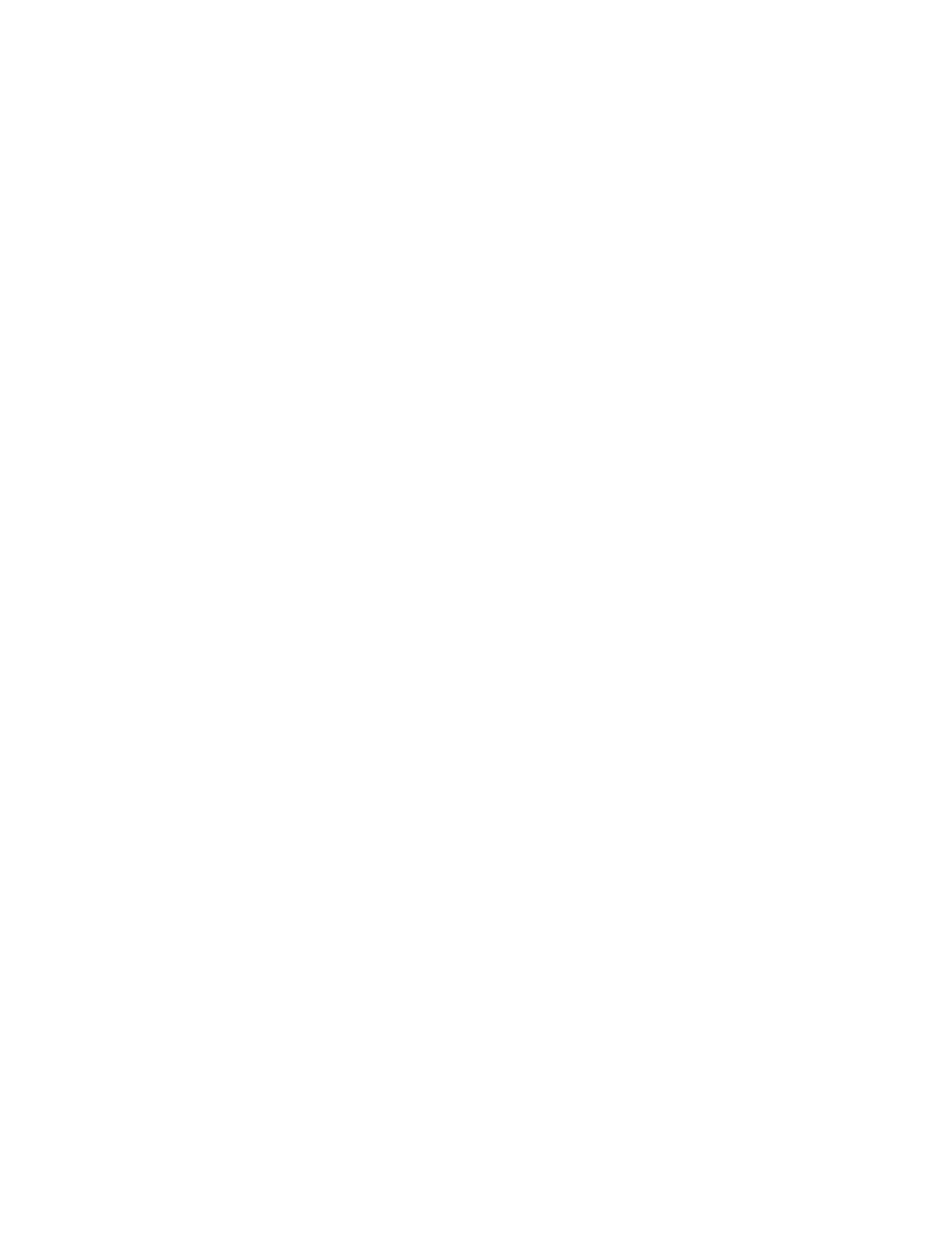
Fig. 9 Optimization vs. Absurdity. It’s all in the output physical layer that we witness the futility, the comedy, … the absurdity.#