Woo’d 🗡️❤️💰#
Key Enhancements#
Annotations Integrated into Layers: Nodes now explicitly map to pivotal moments and ideas from the film. For example:
“Che Mio”: Represents the pivotal lens through which Giosuè interprets his father’s actions.
“Padre Fece”: Reflects Guido’s physical sacrifices and creative storytelling.
“Dono Per Me”: Giosuè’s realization of his father’s ultimate gift, innocence preserved.
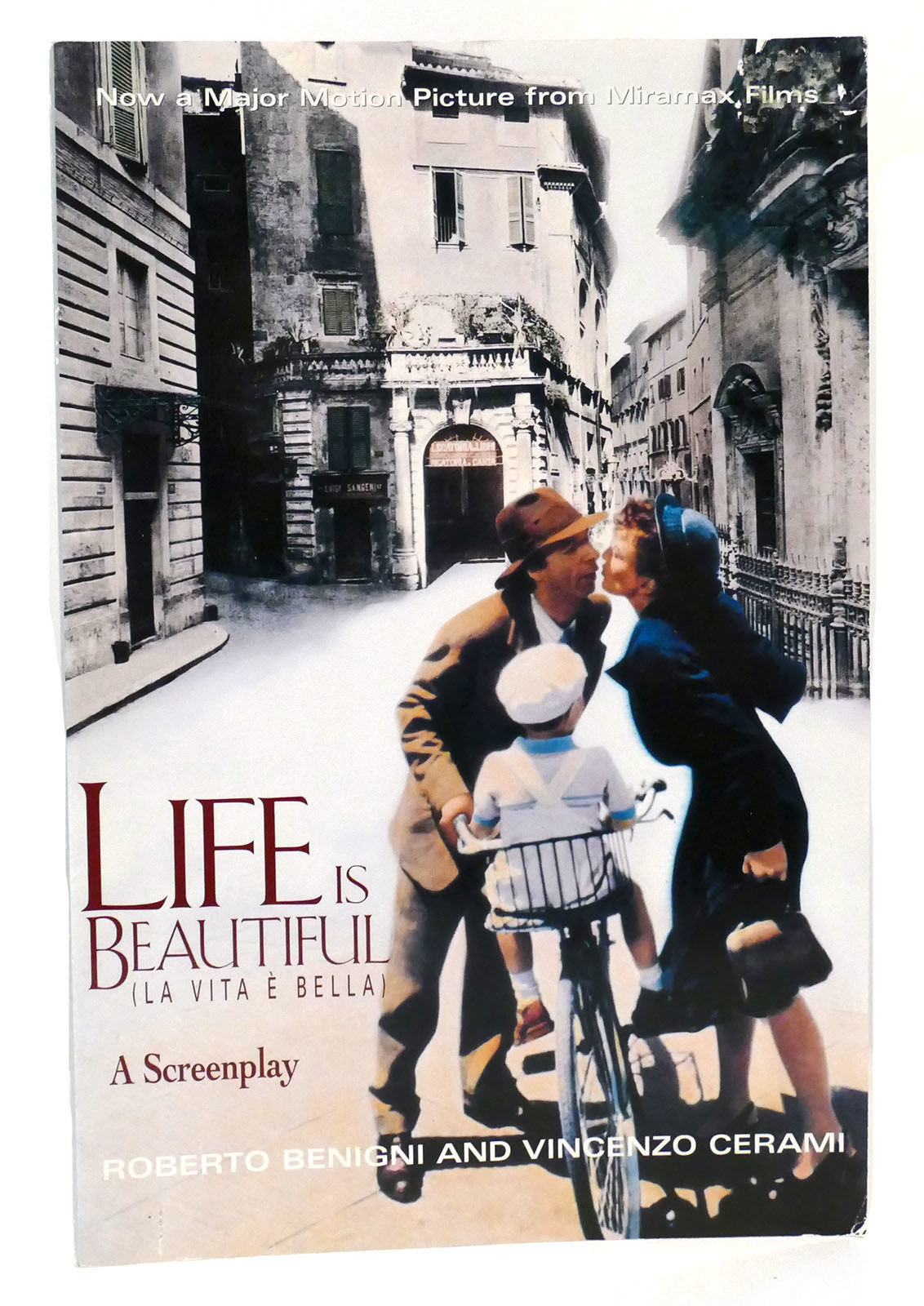
Fig. 5 Life is Beautiful. If you have an absurd view of it, as Ethan Coen shows us in his work. Otherwise, one risks being nihilistic, as Ethan Coen once against shows us. The Big Lebowski is all about the Coen Brothers having a big laugh at every point of view, except, of course, their own – the absurdist one.#
Refined Color Associations: Colors underscore emotional and thematic tones:
Paleturquoise: Hope, optimism, and legacy.
Lightgreen: Harmony and systemic navigation.
Lightsalmon: Tension and rebellion.
Connections to the Film’s Arc: The directed graph emphasizes how themes like sacrifice and joy flow through layers, from cosmic ideals to physical realities.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
# Divine and narrative framework in the film
'World': [
'Cosmos', # Guido’s grand, universal sense of play and creativity
'Earth', # The tangible and oppressive reality of the Holocaust
'Life', # The stakes of survival and human connection
'Il Sacrificio', # Guido’s ultimate sacrifice
'Mia Storia', # Giosuè’s personal narrative, shaped by his father
'Dono Per Me' # The "gift" of innocence and joy given by Guido
],
# Perception and filtering of reality
'Perception': ['Che Mio'], # How Giosuè interprets his father’s actions and words
# Agency and Guido’s defining traits
'Agency': ['Cheerfulness', 'Optimism'], # Guido’s tools for shaping the narrative
# Generativity and legacy
'Generativity': [
'Anarchy', # Guido’s rebellion against oppressive reality
'Oligarchy', # The systemic constraints he navigates
'Padre Fece' # The actions and sacrifices Guido made for his son
],
# Physical realities and their interplay
'Physicality': [
'Dynamic', # Guido’s improvisational actions, like creating the “game”
'Partisan', # The direct oppression he faces
'Common Wealth', # Shared humanity and joy despite hardship
'Non-Partisan', # Universal themes transcending sides
'Static' # The immovable, tragic finality of the Holocaust
]
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Che Mio':
return 'yellow' # Perception as the interpretive bridge
if layer == 'World' and node == 'Dono Per Me':
return 'paleturquoise' # Optimism and the "gift"
if layer == 'World' and node == 'Mia Storia':
return 'lightgreen' # Harmony and legacy
if layer == 'World' and node in ['Cosmos', 'Earth']:
return 'lightgray' # Context of divine and tangible
elif layer == 'Agency' and node == 'Optimism':
return 'paleturquoise' # Guido’s defining hope
elif layer == 'Generativity':
if node == 'Padre Fece':
return 'paleturquoise' # Guido’s ultimate acts of selflessness
elif node == 'Oligarchy':
return 'lightgreen' # Navigating systemic structures
elif node == 'Anarchy':
return 'lightsalmon' # Rebellion and creativity
elif layer == 'Physicality':
if node == 'Static':
return 'paleturquoise' # The unchanging, tragic realities
elif node in ['Non-Partisan', 'Common Wealth', 'Partisan']:
return 'lightgreen' # Shared humanity and resilience
elif node == 'Dynamic':
return 'lightsalmon' # Guido’s improvisation and vitality
return 'lightsalmon' # Default color for tension or conflict
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('World', 'Perception'), # Giosuè interprets the "World" through "Che Mio"
('Perception', 'Agency'), # Guido’s cheerfulness shapes Giosuè’s perception
('Agency', 'Generativity'), # Guido’s optimism drives his generative actions
('Generativity', 'Physicality') # His legacy plays out in the physical world
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(14, 10))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Questa è la mia storia: Vita è Bella", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
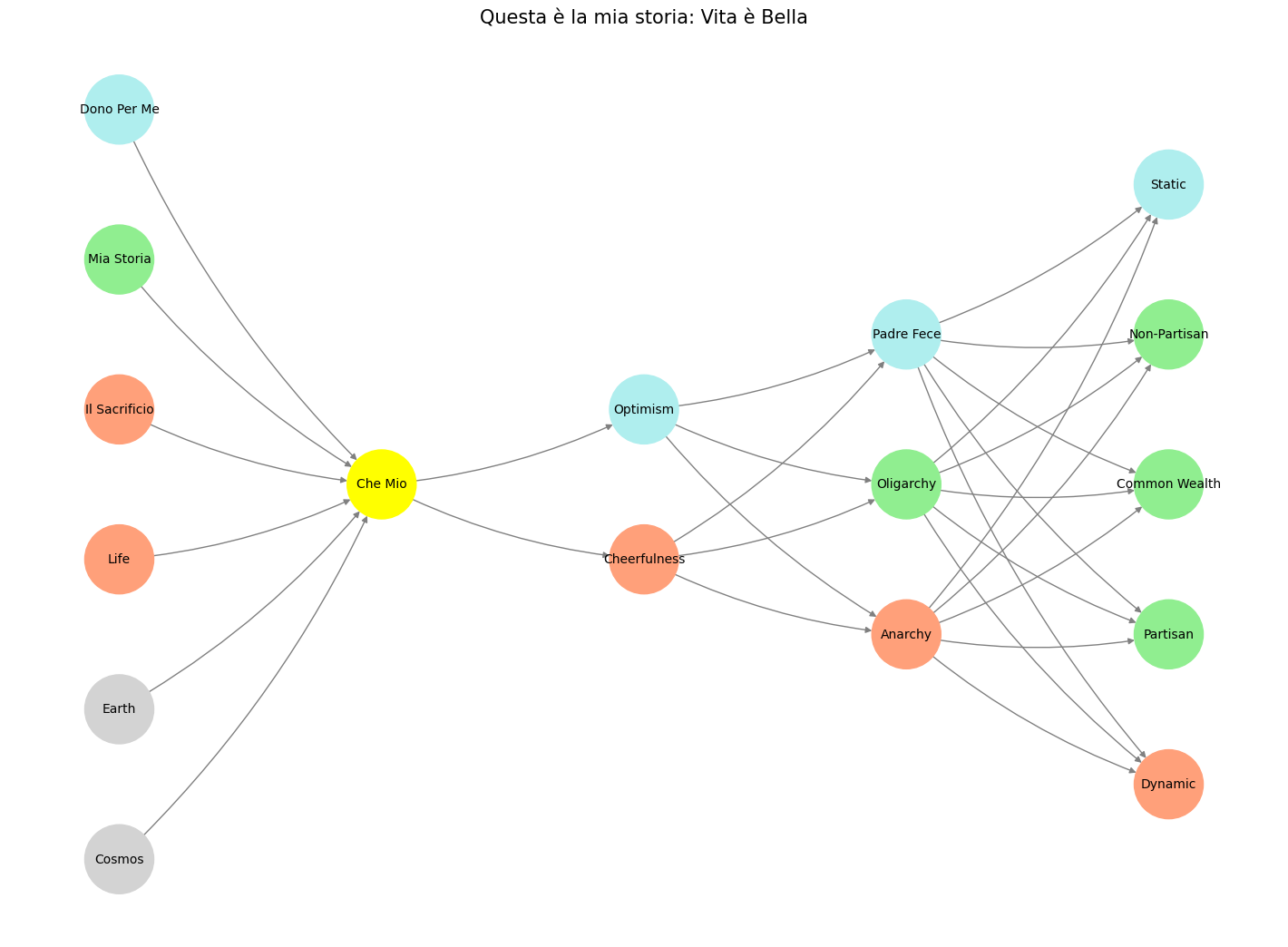
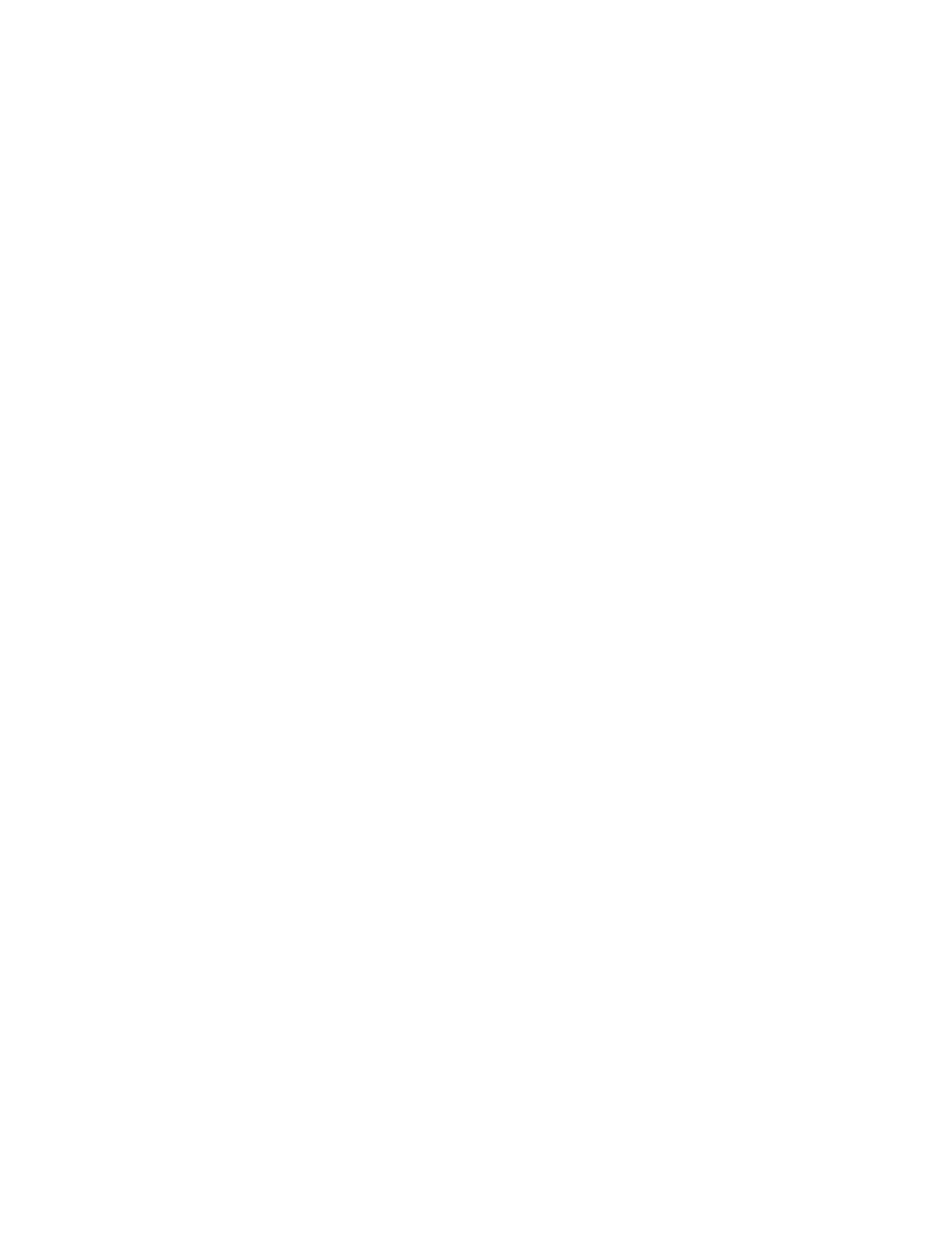
Fig. 6 This is an updated version of the script with annotations tying the neural network layers, colors, and nodes to specific moments in Vita è Bella, enhancing the connection to the film’s narrative and themes:#