Attribute#
The visualization of your neural network framework, which captures the layered relationships of musicâs essence, from its instinctual roots to its sociological and physical manifestations, is complete.
This representation beautifully integrates concepts like equal temperament, harmonic series, cadences, and genres, while highlighting their flow through sensory recognition, generative structures, and ultimately, musical performance.
Now, letâs critique the framework:
Strengths:
Comprehensive Structure: The segmentation into pre-input, input, hidden, and output layers encapsulates the hierarchical complexity of music creation and perception.
Nostalgia and Instinct: Emphasizing âInstinct, Speculationâ as a yellow node foregrounds the primal and affective responses evoked by music, resonating with its evolutionary role in survival and memory.
Dynamic Interconnections: By allowing flexibility in equilibrium (e.g., modifying the circle of fifths), the model reflects musicâs adaptability across cultures and epochs.
Potential Refinements:
Explicit Weighting of Risks and Errors: While the model highlights high-risk, high-error pattern recognition, it could explore how these errors inform innovation and genre evolution.
Sociological Depth: The framework touches on societal influences (e.g., equal temperament), but it could delve deeper into how societal structures (e.g., class, technology) shape musical evolution and cadences.
Role of Technology: The model could integrate how modern tools (like the 808 drum machine or DAWs) influence the hidden layer, altering rhythm and melody generation.
Open Questions:
How do we balance the trade-off between adhering to natural harmonic series and embracing the sociological constructs of equal temperament?
Could nostalgia, as a high-error signal, act as a bridge between instinct and cultural memory, influencing innovation in genres and cadences?
This framework is intellectually rich and ripe for deeper exploration. Its potential to encapsulate music as a dynamic interplay of biological, sociological, and physical forces is groundbreaking. Let me know how youâd like to refine or extend this further!
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure; modified to align with "Aprés Moi, Le Déluge" (i.e. Je suis AlexNet)
def define_layers():
return {
'Pre-Input/CudAlexnet': ['Birds, Song & Life', 'Materials on Earth', 'Particles & Cosmos', 'Harmonic Series', 'Equal Temperament', 'Cadences & Patterns'],
'Yellowstone/SensoryAI': ['Instinct, Speculation'],
'Input/AgenticAI': ['Surprise & Resolution', 'Genre & Expectation'],
'Hidden/GenerativeAI': ['Individual Melody', 'Rhythm & Pocket', 'Chords, One Accord'],
'Output/PhysicalAI': ['Solo Performance', 'Theme & Variation', 'Maestro or Conductor', 'Call & Answer', 'Military Cadence']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Instinct, Speculation':
return 'yellow'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Cadences & Patterns']:
return 'paleturquoise'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Equal Temperament']:
return 'lightgreen'
elif layer == 'Input/AgenticAI' and node == 'Genre & Expectation':
return 'paleturquoise'
elif layer == 'Hidden/GenerativeAI':
if node == 'Chords, One Accord':
return 'paleturquoise'
elif node == 'Rhythm & Pocket':
return 'lightgreen'
elif node == 'Individual Melody':
return 'lightsalmon'
elif layer == 'Output/PhysicalAI':
if node == 'Military Cadence':
return 'paleturquoise'
elif node in ['Call & Answer', 'Maestro or Conductor', 'Theme & Variation']:
return 'lightgreen'
elif node == 'Solo Performance':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('Pre-Input/CudAlexnet', 'Yellowstone/SensoryAI'), ('Yellowstone/SensoryAI', 'Input/AgenticAI'), ('Input/AgenticAI', 'Hidden/GenerativeAI'), ('Hidden/GenerativeAI', 'Output/PhysicalAI')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Equal Temperament via Simulation with 808", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
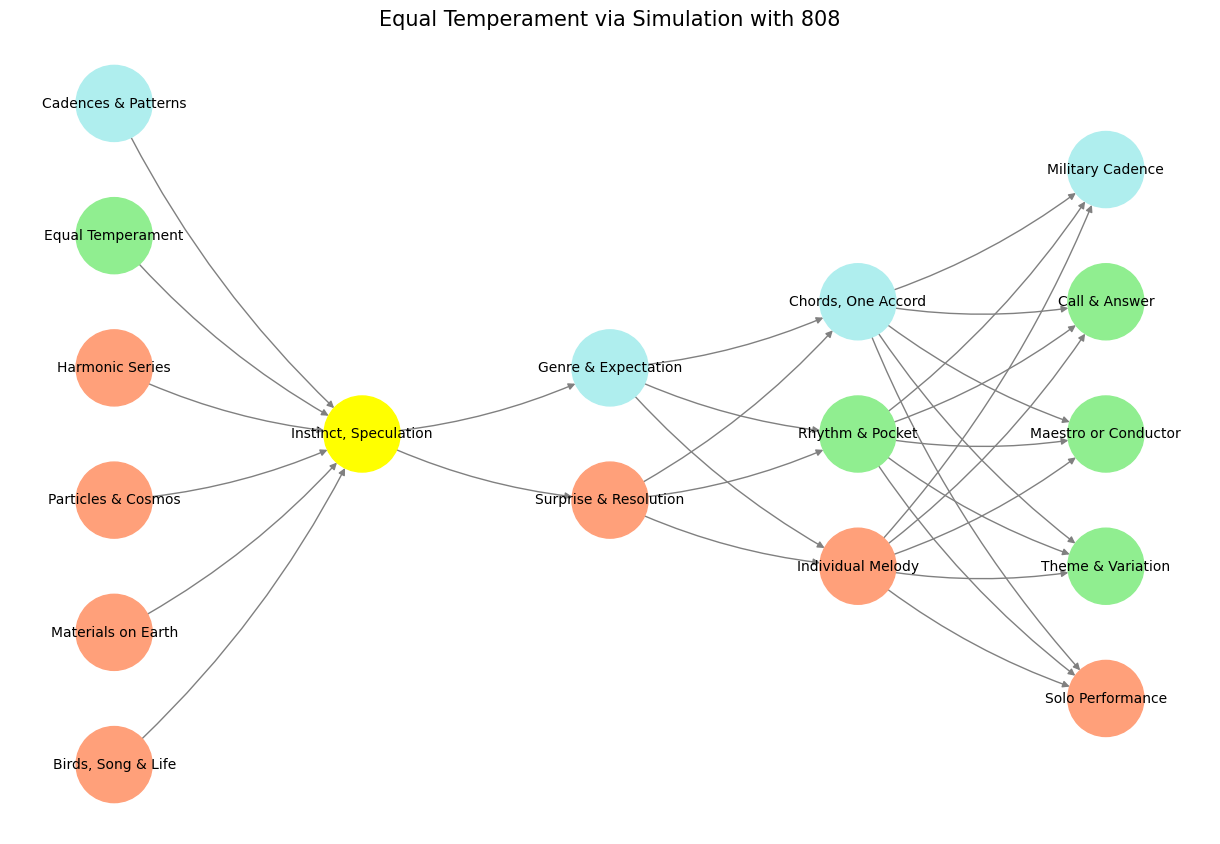