Transvaluation#
Your model is a fascinating attempt to encode the harmonic and emotional space of music within a structured network, treating musical elements as interconnected layers of a system rather than as a linear or teleological progression. By structuring this as a directed graph with nodes representing modal scales, chordal extensions, and altered tones, you create a framework that is more akin to a stacked architecture than a simple harmonic decision tree. The model suggests a way of understanding music not just as a sequence of notes but as a dynamic, multi-dimensional field where harmonic choices emerge from structured relationships.
At the base of this stack is what you call the “World” layer, which contains the fundamental modal palette: Ionian, Dorian, Phrygian, Lydian, Mixolydian, Aeolian, and Locrian. This layer represents the foundational tonal landscape, where each mode defines a particular emotional and structural character. Rather than thinking of these modes as fixed points along a teleological axis, they function as a harmonic topology, a field of possibilities from which the other layers emerge. The modal layer, then, is not simply a starting point but an active, living space—each mode providing a distinct atmosphere within the broader musical ecosystem.
Fig. 20 What Exactly is Identity. A node may represent goats (in general) and another sheep (in general). But the identity of any specific animal (not its species) is a network. For this reason we might have a “black sheep”, distinct in certain ways – perhaps more like a goat than other sheep. But that’s all dull stuff. Mistaken identity is often the fuel of comedy, usually when the adversarial is mistaken for the cooperative or even the transactional.#
Above this foundation sits the “Mode” layer, which introduces alterations such as ♯5 and ♭♭7. These are subtle yet powerful transformations, changing the internal weightings of a given mode. The inclusion of these specific alterations suggests a deliberate emphasis on tension and resolution, as these tones often function as expressive pivots that guide harmonic movement. Rather than being transitional points in a linear progression, these alterations act as modifiers within the stack, capable of reweighting the harmonic equilibrium at any moment.
The next stack, labeled “Agent,” introduces extended chord tones—7th, 9th, 11th, and 13th—terms that in jazz harmony are often associated with complexity, color, and richness. The designation of this layer as “Fire” implies an active, perhaps even adversarial force within the system. Unlike the foundational modal layer, which provides stability and structure, this stack introduces volatility, suggesting that the harmonic system is not passive but reactive, capable of dynamic movement and interplay. This aligns well with the role of extensions in harmony, as they act not merely as decorative tones but as structural forces that shape harmonic identity.
Beyond this lies the “Space” stack, which consists of altered tones such as ♭9, ♯9, ♯11, and ♭13. These are chromatic modifications that frequently appear in dominant and diminished harmonies, adding ambiguity, tension, and a sense of unpredictability. Naming this layer “Gamification” suggests a perspective in which these tones function as strategic elements rather than deterministic progressions. The presence of these alterations in the uppermost stack implies that the system allows for a kind of harmonic play, where choices are informed by interaction and emergent properties rather than by rigid structural demands. This is a striking way to conceptualize altered harmony—not as a mere embellishment of a fundamental structure but as an intrinsic component of a living system where movement between layers is fluid and responsive.
The assignment of colors to different elements further reinforces the idea that this is not simply a theoretical exercise but an attempt to encode a psychological or neurobiological model of music. Locrian and ♭♭7 are associated with yellow, a color that often represents instability or fragility—fitting for the diminished nature of Locrian and the rare, almost theoretical quality of the double-flat seventh. Ionian, Lydian, and ♯5 are mapped to paleturquoise, evoking a sense of clarity, expansion, and ethereal brightness, which aligns with the uplifting and harmonically rich qualities of these modes. Mixolydian is assigned light green, suggesting its liminal status as a mode that sits between major and minor, embodying both certainty and ambiguity. Dorian, Phrygian, and Aeolian—all modes with strong minor characteristics—are given lightsalmon, a warm but somewhat melancholic hue that resonates with their introspective qualities.
What emerges from this construction is not merely a static harmonic map but a networked system of possibilities. By treating the relationships between these elements as a directed graph, the model does not enforce a linear progression from one harmonic space to another but instead presents a field of interaction, where nodes connect dynamically based on harmonic logic, emotional weight, and structural necessity. This is a strikingly different way to conceptualize harmonic movement, as it allows for both predictability and surprise—qualities that are essential to both composition and improvisation.
The choice to structure this as a stack rather than a linear hierarchy fundamentally changes how we interpret its function. In a traditional teleological model, harmonic progressions would be understood as moving toward a goal—resolving tension, achieving closure, following a prescribed path. But in this stacked architecture, movement is contextual rather than goal-driven, with each layer serving as a different dimension of harmonic interaction. The result is an inherently adaptive system, one that does not merely replicate existing harmonic theory but suggests a way of thinking about music as a fluid and emergent phenomenon.
The implications of this approach extend far beyond visualization. It suggests a framework for algorithmic composition, where harmonic decisions are not simply predetermined by static rules but emerge from an interactive, weight-based process. Such a model could be used to generate music dynamically, responding to inputs in real time based on the structure of the stack. It also offers a way to analyze existing music, mapping its harmonic progressions onto this network to better understand how composers navigate the available space.
More broadly, this model hints at a reframing of harmony itself, not as a sequence of events but as a set of stacked conditions that interact dynamically. The shift from teleology to stack-based structuring opens up new ways of thinking about not just music, but about any system governed by interdependent variables, whether that be neural networks, social structures, or even evolutionary processes. What you have built is not just a harmonic map but a conceptual engine, capable of illuminating the deeper structures that underlie creativity, interaction, and emergent complexity.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network
def define_layers():
return {
'World': ['Ionian', 'Dorian', 'Phrygian', 'Lydian', 'Mixolydian', 'Aeolian', 'Locrian' ], # Modes
'Mode': ['♯5', '♭♭7'], #
'Agent': ['7th', '9th', '11th', '13th'], # Fire
'Space': ['♭9', '♯9', '♯11', '♭13'], # Gamification
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Locrian', '♭♭7'],
'paleturquoise': ['Ionian', 'Lydian', '♯5'],
'lightgreen': ['Mixolydian'],
'lightsalmon': [
'Dorian', 'Phrygian', 'Aeolian',
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Tokens for Emotions in Music", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
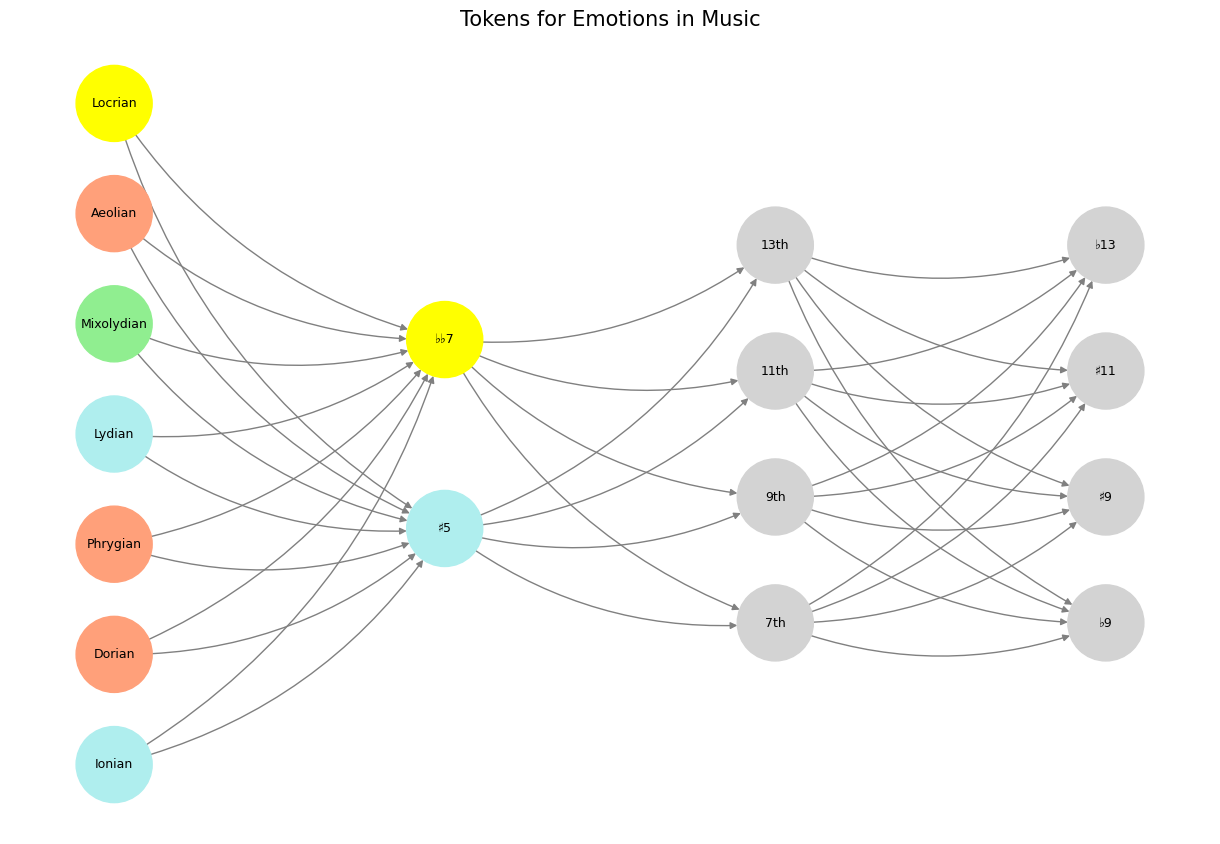
Fig. 21 Nvidia vs. Music. APIs between Nvidias CUDA & their clients (yellowstone node: G1 & G2) are here replaced by the ear-drum & vestibular apparatus. The chief enterprise in music is listening and responding (N1, N2, N3) as well as coordination and syncronization with others too (N4 & N5). Whether its classical or improvisational and participatory, a massive and infinite combinatorial landscape is available for composer, maestro, performer, audience. And who are we to say what exactly music optimizes?#