4.3#
Nietzsche#
Here is announced, perhaps for the first time, a pessimism “beyond good and evil.”
In the spirit of music: no key, note, or chord is wrong for any given key signature.
We shall dance in this life’s fetters like the freest of spirits, channeling passing chords…
1. Pessimism
\
2. Beyond Good & Evil -> 4. Dionysian -> 5. Science -> 6. Morality
/
3. Robustness
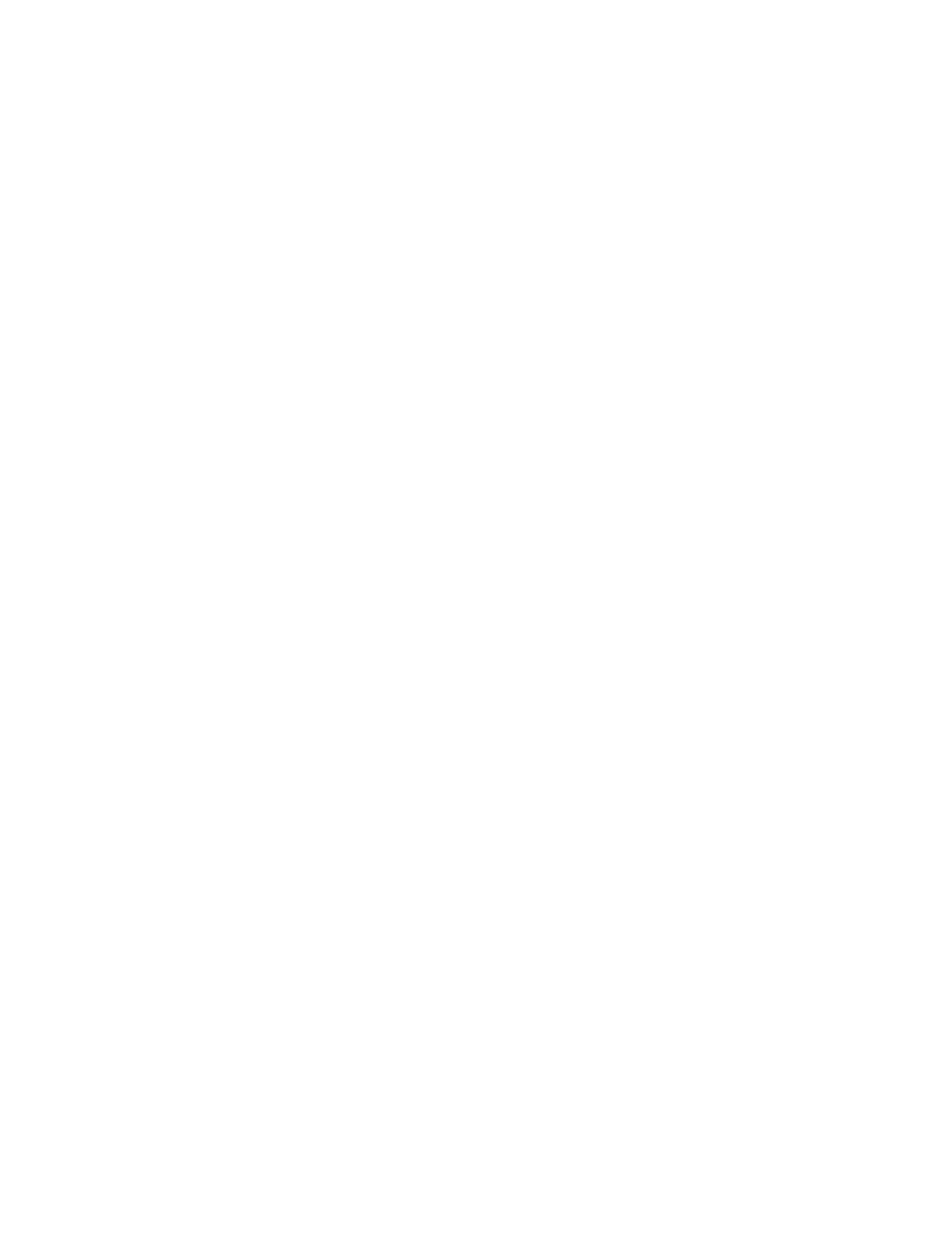
Fig. 10 Attempt at Self-Criticism.#
Embrace Notes From All Keys 1, 2, 3#
Root, 3rd, 5th, ♭♭7, 9th
Passing Chords (Dancing in Chains) 4#
11th, 13th
Voice leading, Passing chords, Chromaticism
Systematized 5, 6#
♭9,♯9,♯11,♭13
Temperament
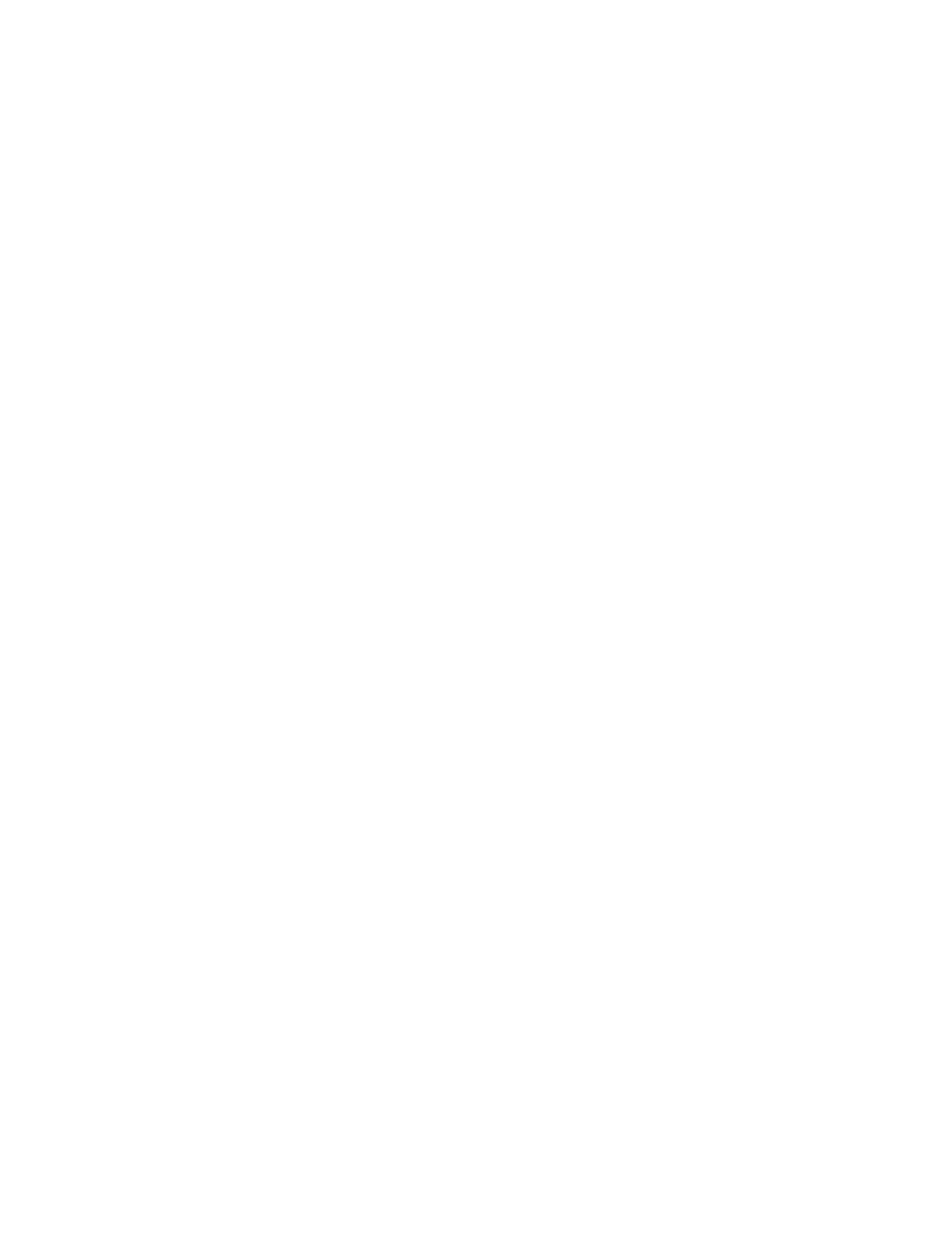
Fig. 11 DAG.#
1. Chaos
\
2. Frenzy -> 4. Dionysian -> 5. Algorithm -> 6. Binary
/
3. Ener`gy
Dionysus 1, 2, 3#
Life if embraced by honest eyes, mind, and body:
biological
Sing O Muse! 4#
Uncommunicable in words; collective-unconscious, perhaps in dreams, song, dance, poetry:
social
Apollo 5, 6#
Metaphysical comfort: classifying everything into good & evil, and eliminating one:
pesronal
Becoming#
“The Birth of Tragedy: Out of the Spirit of Music” (1872): Indeed, this was Nietzsche’s first book, where he introduced the Apollonian-Dionysian duality. The musical metaphor is not just an illustrative device but a fundamental aspect of his early philosophy. He saw Greek tragedy as born from the union of Apollonian form and Dionysian music.
Critique of Kant and Schopenhauer: You’re right to highlight that Nietzsche’s later work represents a significant departure from his earlier views, which were heavily influenced by Kant and Schopenhauer. The mature Nietzsche indeed critiqued the dialectical formula of Apollonian + Dionysian = Tragedy.
Evolution of Nietzsche’s thought: The shift from the 28-year-old Nietzsche to the 44-year-old Nietzsche represents a profound philosophical journey. His later works, like “Beyond Good and Evil” (1886) and “On the Genealogy of Morality” (1887), showcase a more nuanced and critical approach to morality and traditional philosophy.
Rejection of simple dialectics: Nietzsche’s mature philosophy rejects the neat synthesis of opposing forces that he initially proposed. This reflects his growing skepticism towards systematic philosophy and his embrace of a more fluid, life-affirming perspective.
From balance to Dionysian emphasis: The move from seeking a balance between Apollonian and Dionysian elements to a more Dionysian-centric philosophy reflects Nietzsche’s increasing emphasis on life affirmation and his critique of rationality as life-denying.
Self-critique: Nietzsche’s embarrassment at his earlier work shows his intellectual honesty and willingness to evolve. This self-critical stance is itself a very Nietzschean trait, reflecting his philosophy of constant self-overcoming.
Protogenoi 1, 2, 3#
Food:
Chaos, Gaia, Tartaru
&Eros, Nyx
Teeth
Gut
Olympians#
Enzymes: Apollo & Dionysus
Titans#
Nutrition, Growth
1. Pessimism \ 2. Beyond Good & Evil -> 4. Parameters, Weights, Hierarchy, Values, Latent-Space -> 5. Zarathustra, Decoder -> 6. Rebalance, Ethics / 3. Ecce Homo
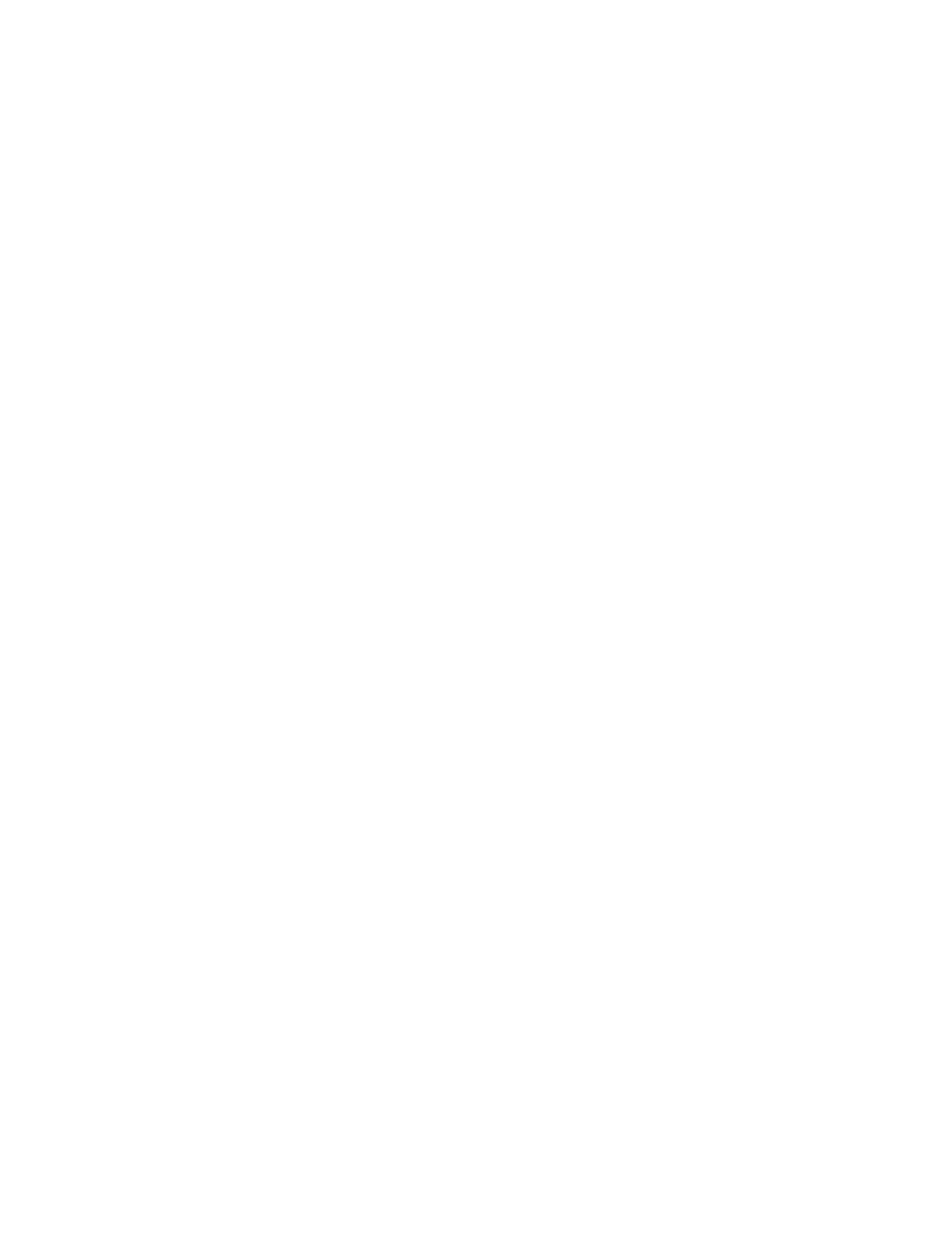
Fig. 12 Ecce Homo. The School of Resentment taps into the latent space through RLHF to re-balance the weights emerging from OLS-regression on human history, thereby transvaluating all values. You might call this fraud, will-to-power, or what you will. But its certainly not “data”, which themselves represent the hierarchies from human history. That per se isn’t bias – you can’t say that if victors write history then the narratives of victors are biased. They are narratives. And by victors. Thats all.#
How much truth can a certain mind endure; how much truth can it dare? These questions became for me ever more and more the actual test of values.