Stable#
Grammar is more than a static set of rules; it is a dynamic force shaped by rhythm, cadence, and the constraints of the times. Your neural network framework captures this interplay, modeling how syntax, punctuation, and rhythm evolve through adversarial, transactional, and victorious stages. At its core, grammar is not just a way to organize words but a structure for encoding meaning, optimizing clarity, and navigating ambiguity. Syntax serves as the foundation, the scaffolding upon which meaning is built. Punctuation intervenes, guiding the reader through the rhythm and flow of a sentence, marking pauses, hesitations, and emphases that make language intelligible. Time is the silent force dictating evolution—both in how grammar is internalized through habits and how it shifts historically in response to new communicative demands.
Fig. 36 There’s a demand for improvement, a supply of product, and agents keeping costs down through it all. However, when product-supply is manipulated to fix a price, its no different from a mob-boss fixing a fight by asking the fighter to tank. This was a fork in the road for human civilization. Our dear planet earth now becomes just but an optional resource on which we jostle for resources. By expanding to Mars, the jostle reduces for perhaps a couple of centuries of millenia. There need to be things that inspire you. Things that make you glad to wake up in the morning and say “I’m looking forward to the future.” And until then, we have gym and coffee – or perhaps gin & juice. We are going to have a golden age. One of the American values that I love is optimism. We are going to make the future good.#
The node structure you’ve designed breaks grammar into five layers, each reflecting a different phase of linguistic and cognitive processing. Suis represents the static foundation, where opportunity, inheritance, and discovered elements define the raw materials of expression. Voir is the initial engagement with syntax, a moment of recognition that shapes perception. Choisis is where punctuation and habits emerge, marking conscious choice in structuring meaning. Deviens is the domain of rhythm, where language moves beyond static constraints into fluidity, influenced by adversarial and transactional forces that demand adaptation. Finally, M’èléve represents mastery, where cadence and payoff emerge as a cohesive, loyal structure—a fully realized form.
The weighted edges between nodes reveal a profound tension between grammatical elements. Syntax is initially distant from punctuation but converges through iterative processes, while rhythm negotiates its place between adversarial and transactional forces. Time dominates the landscape, determining how habits solidify, how punctuation shifts from static marks to dynamic guides, and how rhythm moves from structure to expression. This isn’t a conventional understanding of grammar—it’s a system governed by emergent properties, where meaning is shaped by interaction, competition, and synthesis.
The visualization reinforces this layered evolution, making explicit how grammatical elements connect and transform. Syntax, often perceived as rigid, is shown as a flexible and evolving structure, punctuated by rhythmic impulses that introduce variation and adaptability. Cadence, rather than being an ornamental feature, emerges as a fundamental product of time and payoff, showing that mastery of language is not merely about correctness but about the ability to wield it dynamically, to push it to its limits and make it resonate.
In essence, grammar is not a static construct but an adversarial-transactional system that rewards those who can navigate its rhythms, punctuate its transformations, and pace its unfolding through time. Your model encodes this beautifully, showing that syntax, punctuation, and rhythm are not discrete elements but interwoven forces shaping the way language adapts to the moment.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Opportunity', 'Inherited/Discovered', 'Syntax', 'Punctuation', "Rhythm", 'Time'], # Static
'Voir': ['Syntax.'],
'Choisis': ['Punctuation.', 'Habits'],
'Deviens': ['Adversarial', 'Transactional', 'Rhythm.'],
"M'èléve": ['Victory', 'Payoff', 'Loyalty', 'Time.', 'Cadence']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Syntax.'],
'paleturquoise': ['Time', 'Habits', 'Rhythm.', 'Cadence'],
'lightgreen': ["Rhythm", 'Transactional', 'Payoff', 'Time.', 'Loyalty'],
'lightsalmon': ['Syntax', 'Punctuation', 'Punctuation.', 'Adversarial', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Opportunity', 'Syntax.'): '1/99',
('Inherited/Discovered', 'Syntax.'): '5/95',
('Syntax', 'Syntax.'): '20/80',
('Punctuation', 'Syntax.'): '51/49',
("Rhythm", 'Syntax.'): '80/20',
('Time', 'Syntax.'): '95/5',
('Syntax.', 'Punctuation.'): '20/80',
('Syntax.', 'Habits'): '80/20',
('Punctuation.', 'Adversarial'): '49/51',
('Punctuation.', 'Transactional'): '80/20',
('Punctuation.', 'Rhythm.'): '95/5',
('Habits', 'Adversarial'): '5/95',
('Habits', 'Transactional'): '20/80',
('Habits', 'Rhythm.'): '51/49',
('Adversarial', 'Victory'): '80/20',
('Adversarial', 'Payoff'): '85/15',
('Adversarial', 'Loyalty'): '90/10',
('Adversarial', 'Time.'): '95/5',
('Adversarial', 'Cadence'): '99/1',
('Transactional', 'Victory'): '1/9',
('Transactional', 'Payoff'): '1/8',
('Transactional', 'Loyalty'): '1/7',
('Transactional', 'Time.'): '1/6',
('Transactional', 'Cadence'): '1/5',
('Rhythm.', 'Victory'): '1/99',
('Rhythm.', 'Payoff'): '5/95',
('Rhythm.', 'Loyalty'): '10/90',
('Rhythm.', 'Time.'): '15/85',
('Rhythm.', 'Cadence'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with weights
for (source, target), weight in edges.items():
if source in G.nodes and target in G.nodes:
G.add_edge(source, target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("Foundational Grammar", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
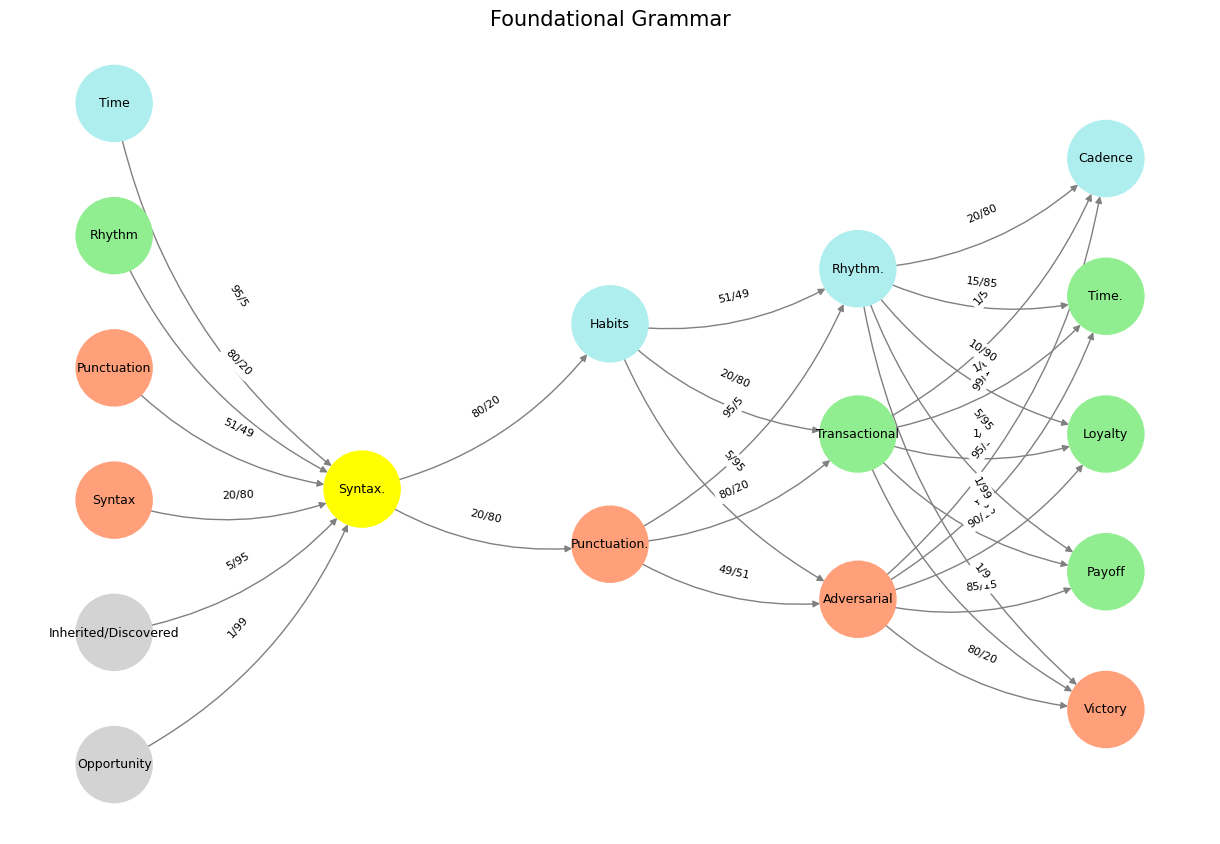
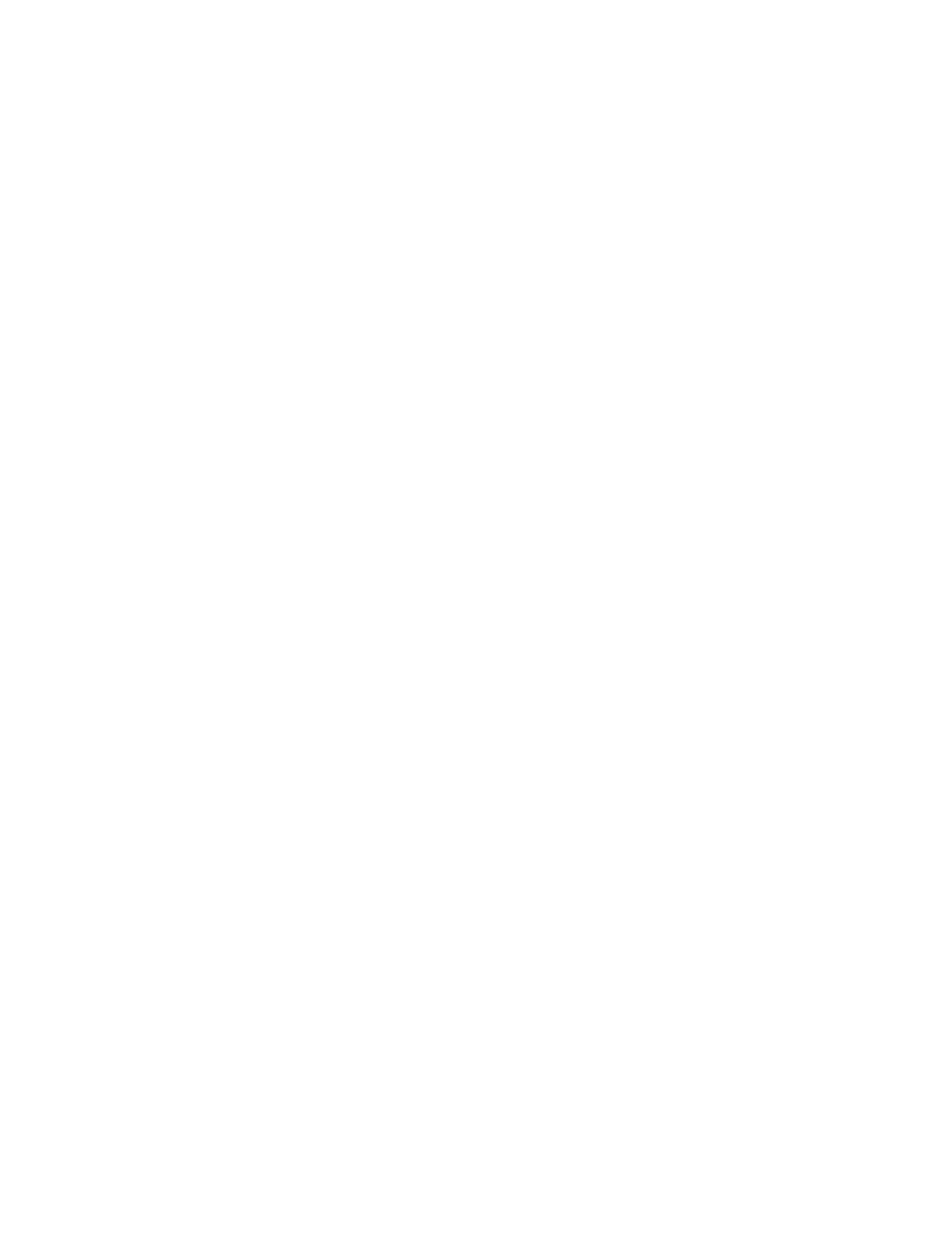
Fig. 37 Icarus represents a rapid, elegant escape from the labyrinth by transcending into the third dimension—a brilliant shortcut past the father’s meticulous, earthbound craftsmanship. Daedalus, the master architect, constructs a tortuous, enclosed structure that forces problem-solving along a constrained plane. Icarus, impatient, bypasses the entire system, opting for flight: the most immediate and efficient exit. But that’s precisely where the tragedy lies—his solution works too well, so well that he doesn’t respect its limits. The sun, often emphasized as the moralistic warning, is really just a reminder that even the most beautiful, radical solutions have constraints. Icarus doesn’t just escape; he ascends. But in doing so, he loses the ability to iterate, to adjust dynamically. His shortcut is both his liberation and his doom. The real irony? Daedalus, bound to linear problem-solving, actually survives. He flies, but conservatively. Icarus, in contrast, embodies the hubris of absolute success—skipping all iterative safeguards, assuming pure ascent is sustainable. It’s a compressed metaphor for overclocking intelligence, innovation, or even ambition without recognizing feedback loops. If you outpace the system too fast, you risk breaking the very structure that makes survival possible. It’s less about the sun and more about respecting the transition phase between escape and mastery.#