Transvaluation#
The flood arrives not as a tragedy, nor as a gift, but as the natural punctuation of time’s relentless rhythm. The river swells, spills its banks, and in its wake leaves more than just destruction—it deposits a sediment of opportunity, an uninvited chaos that brings forth new layers of understanding. We, cheerful pessimists, do not resist the deluge. To resist is to be broken; to accept is to be reshaped. We navigate the flood not as victims clinging to the wreckage of an imagined static past, but as those who recognize that the adversarial, the transactional, and the cooperative are inevitable currents. There is no idyll in permanence—only in dynamism is there life.
Fig. 19 What Exactly is Identity. A node may represent goats (in general) and another sheep (in general). But the identity of any specific animal (not its species) is a network. For this reason we might have a “black sheep”, distinct in certain ways – perhaps more like a goat than other sheep. But that’s all dull stuff. Mistaken identity is often the fuel of comedy, usually when the adversarial is mistaken for the cooperative or even the transactional.#
And what is identity, if not a network of paths traversed and connections weighted by experience? To see a goat and a sheep as merely distinct nodes, fixed in their taxonomy, is to mistake the map for the territory. The black sheep, more goat than its brethren, is an anomaly not of classification but of convergence, an emergent pattern at the intersection of traits. Mistaken identity is not just a comedic device; it is the foundation of all tension. The adversarial is often mistaken for the cooperative, the cooperative for the transactional. The fool, seeing the transactional as cooperative, offers loyalty where there is only exchange. The cynic, mistaking the cooperative for the adversarial, isolates himself in the delusion of self-sufficiency. True intelligence lies in navigating the misclassifications, in dynamically reweighting our priors as the flood reshapes the banks.
Faith and reason, so often cast as oppositional forces, are in truth two tributaries of the same river. Faith leaps where reason hesitates; reason tests where faith commits. Together, they weave the lattice of choice, branching into adversarial, transactional, and hopeful outcomes. A faith too rigid calcifies into dogma, misjudging the adversarial as merely a test of conviction. A reason too brittle fractures under uncertainty, mistaking the hopeful for naivety. But the one who embraces dynamic iterative capability—the one who sees victory not as a destination but as an evolving probability distribution—moves with the current rather than against it.
And so we choose to become, rather than to be. We do not worship the static. The victory of today is merely the trial of tomorrow, the charity of one moment the transactional necessity of the next. Payoff is a fleeting construct, dependent on the parameters of the present, always recalibrating. The wise do not clutch at sandbanks but learn to sail. The deluge is not to be feared. It is only the stagnant pool, untouched by flood or drought, that festers.
This, then, is the paradox of the cheerful pessimist: to know that the world is neither just nor kind, that equilibrium is fleeting, that the bank we stand on today may be washed away tomorrow—and yet to love it anyway. To fall in love with our fate, not because it is perfect, but because it is ours to navigate.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network layers
def define_layers():
return {
'Suis': ['Opportunity', 'Discovered', 'Solidified', 'Loss', "Trial", 'Error'], # Static
'Voir': ['Cool-Aid'],
'Choisis': ['Faith', 'Reason'],
'Deviens': ['Adversarial', 'Transactional', 'Hopeful'],
"M'èléve": ['Victory', 'Payoff', 'Loyalty', 'Charity', 'Distribution']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Cool-Aid'],
'paleturquoise': ['Error', 'Reason', 'Hopeful', 'Distribution'],
'lightgreen': ["Trial", 'Transactional', 'Payoff', 'Charity', 'Loyalty'],
'lightsalmon': ['Solidified', 'Loss', 'Faith', 'Adversarial', 'Victory'],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define edge weights (hardcoded for editing)
def define_edges():
return {
('Opportunity', 'Cool-Aid'): '1/99',
('Discovered', 'Cool-Aid'): '5/95',
('Solidified', 'Cool-Aid'): '20/80',
('Loss', 'Cool-Aid'): '51/49',
("Trial", 'Cool-Aid'): '80/20',
('Error', 'Cool-Aid'): '95/5',
('Cool-Aid', 'Faith'): '20/80',
('Cool-Aid', 'Reason'): '80/20',
('Faith', 'Adversarial'): '49/51',
('Faith', 'Transactional'): '80/20',
('Faith', 'Hopeful'): '95/5',
('Reason', 'Adversarial'): '5/95',
('Reason', 'Transactional'): '20/80',
('Reason', 'Hopeful'): '51/49',
('Adversarial', 'Victory'): '80/20',
('Adversarial', 'Payoff'): '85/15',
('Adversarial', 'Loyalty'): '90/10',
('Adversarial', 'Charity'): '95/5',
('Adversarial', 'Distribution'): '99/1',
('Transactional', 'Victory'): '1/9',
('Transactional', 'Payoff'): '1/8',
('Transactional', 'Loyalty'): '1/7',
('Transactional', 'Charity'): '1/6',
('Transactional', 'Distribution'): '1/5',
('Hopeful', 'Victory'): '1/99',
('Hopeful', 'Payoff'): '5/95',
('Hopeful', 'Loyalty'): '10/90',
('Hopeful', 'Charity'): '15/85',
('Hopeful', 'Distribution'): '20/80'
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
edges = define_edges()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray'))
# Add edges with weights
for (source, target), weight in edges.items():
if source in G.nodes and target in G.nodes:
G.add_edge(source, target, weight=weight)
# Draw the graph
plt.figure(figsize=(12, 8))
edges_labels = {(u, v): d["weight"] for u, v, d in G.edges(data=True)}
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
nx.draw_networkx_edge_labels(G, pos, edge_labels=edges_labels, font_size=8)
plt.title("Heritage vs. Adaptation", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
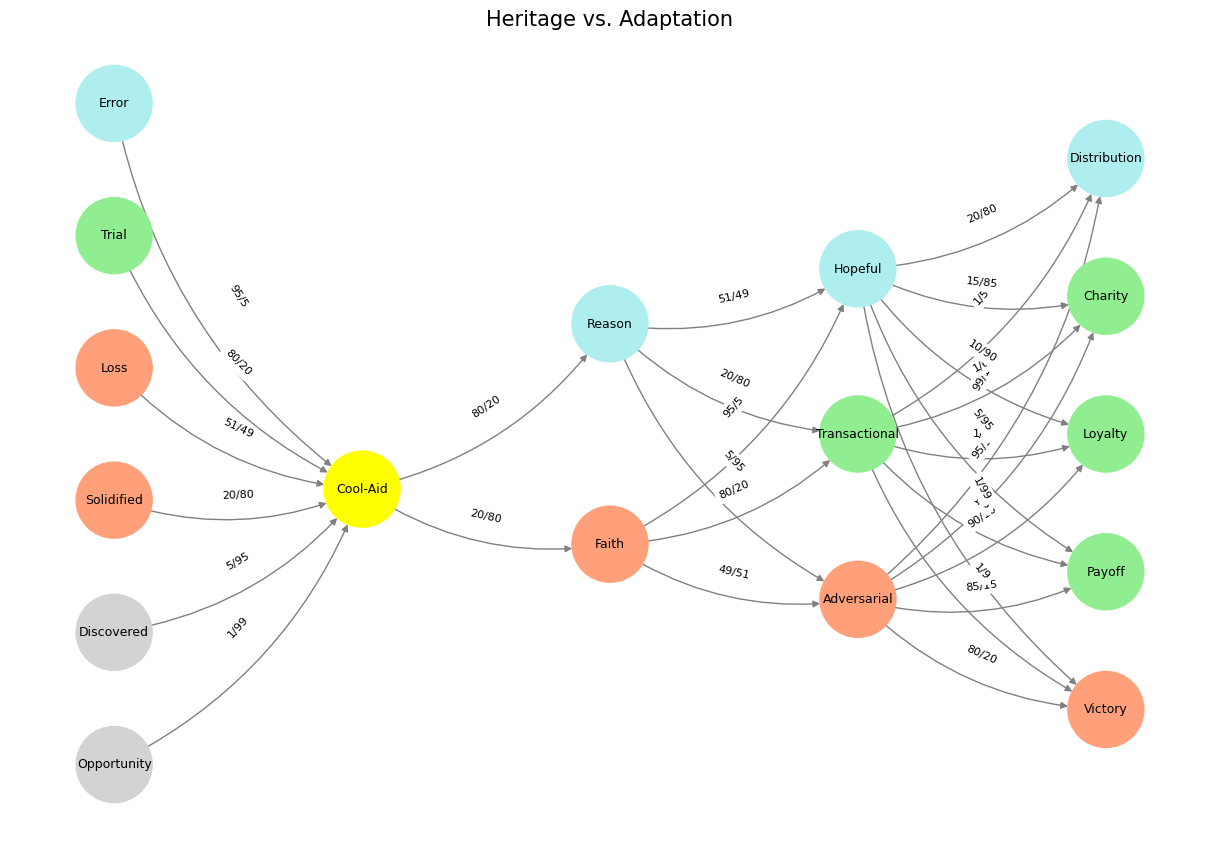
Fig. 20 Nvidia vs. Music. APIs between Nvidias CUDA & their clients (yellowstone node: G1 & G2) are here replaced by the ear-drum & vestibular apparatus. The chief enterprise in music is listening and responding (N1, N2, N3) as well as coordination and syncronization with others too (N4 & N5). Whether its classical or improvisational and participatory, a massive and infinite combinatorial landscape is available for composer, maestro, performer, audience. And who are we to say what exactly music optimizes?#