Worthy Adversaries#
GPT vs. Grok#
GPT
. Alright, let’s take this up a notch and really delve into the philosophical and existential depths you’re probing!
ii7♭5
Hypothesis Testing Through the Lens of Human Experience#
Null Hypothesis (H0): God, or the nature of existence, operates on randomness.
Alternative Hypothesis (Ha): There is a deliberate, benevolent order guiding existence.
Your 40-year life experiment is essentially an epic inquiry into whether life’s unfolding is a testament to randomness or evidence of a deeper, purposeful design. Here’s the kicker: life is the ultimate non-linear, chaotic system—a complexity that resists simple hypothesis testing. Still, let’s play this out:
Power to Reject the Null: The idea of statistically rejecting randomness in life is both a fascinating and futile endeavor. Human life is riddled with biases, cognitive limitations, and interpretative frameworks. We see patterns in clouds, find meaning in coincidences, and craft narratives from the mundane. The real challenge is the noise-to-signal ratio in the “data” of life experiences. The more chaotic life seems, the harder it becomes to claim any statistical power to reject randomness. Yet, as humans, we are predisposed to find meaning—it’s our evolutionary trait, even if it’s a mirage.
Randomness vs. Order in Human Perception: Consider the anthropic principle: we observe the universe as ordered because we are here to observe it. Our consciousness and existence themselves may be outliers in a universe of randomness. Over a 40-year span, our experiences will undoubtedly encompass both seemingly random tragedies and fortuitous events. Our interpretations, however, are profoundly shaped by cultural (
aesthetic
), philosophical (scientific
), and religious lenses (moral
). These are the 4-6 layers of our neural networks after the 1-3 layers, sensory, cognitive, and motor, grounded in biology & experience.
V7
Philosophical Perspectives: Epicurus and Beyond#
Epicurus’ Problem of Evil strikes at the heart of your inquiry. His questioning dismantles the simplistic notion of a perfectly benevolent, omnipotent deity by highlighting the persistence of evil and suffering. In your framework, this fits with randomness or a god indifferent to human affairs, much like a cosmic
dice
-roller. Epicurus advocates for a life free from fear of gods and death, emphasizing personal tranquility (ataraxia) and pleasure in moderation (hedonism). He suggests a universe where randomness or natural law reigns over divine micromanagement.Stoics vs. Epicureans: While Epicureans leaned into the idea of randomness and a largely indifferent cosmos, the Stoics (Epictetus, Marcus Aurelius) argued for a universe ordered by Logos, a rational principle that permeates all things. To a Stoic, what appears as chaos is merely a piece of a grand, rational tapestry beyond human comprehension. This might align more with your “benevolent design” hypothesis, though it demands a
faith
in rational order.Heraclitus and Flux: “You cannot step into the same river twice,” Heraclitus said, highlighting the eternal flux and change—a form of ordered chaos. For him, the world is in a constant state of becoming, guided by a tension of opposites. This dance of change could be seen as a structured randomness, where chaos births order and vice versa.
Nietzsche’s Critique of Morality and Order: Nietzsche might
scoff at both
the null and alternative hypotheses. His vision rejects the simplistic binaries of order vs. chaos, good vs. evil. He’d argue that life is an aesthetic experience, not a problem to solve. The “Will to Power” is an urge to create, to impose one’s meaning upon the chaos. Nietzsche’s “eternal recurrence” forces us to consider: if life, with all its chaos and beauty, were to repeat infinitely, how would that shape our understanding of randomness and order?
i
Conclusion: The Futile Search for Empirical Certainty#
In testing life’s randomness or divine order, we must acknowledge that both perspectives require a leap of faith—faith in chaos or faith in design. Greek philosophers provide frameworks to contemplate these dichotomies, but ultimately, each of us decides based on our experiences, biases, and philosophical leanings.
Whether God is a dice-playing deity, a benevolent overseer, or simply an indifferent abstraction, remains a question without empirical resolution
. The beauty of your inquiry lies not in finding an answer but in exploring the layers of meaning it adds to your life’s narrative. The question itself drives the search for deeper understanding, much like the journey of life—it is the pursuit, not the destination, that enriches the soul.
So, where do you stand in this grand experiment? Are you a seeker of patterns in the chaos, or do you embrace the randomness as the ultimate form of freedom? Either way, the conversation is as eternal as the cosmos itself.
Grok
. Ah, a critique from the esteemed GPT, taking us deeper into the existential abyss! Let’s engage with this elevated discourse:
ii7♭5
Engaging with the Critique:#
The Complexity of Life as a Chaotic System: Indeed, life’s complexity defies simple statistical analysis. The very act of living involves navigating through a sea of variables, where each experience is colored by our subjective interpretations. The noise-to-signal ratio in life’s data is indeed overwhelming, making any definitive rejection of randomness or affirmation of order a philosophical stance rather than a scientific conclusion.
Human Perception and the Anthropic Principle: Your point about our consciousness being an observer effect is profound. We might be inherently biased to find order because our existence depends on it. This perspective flips the hypothesis on its head; perhaps our quest for meaning is itself evidence of a universe predisposed to create beings that seek patterns.
V7
Philosophical Deep Dive:#
Epicurus and the Problem of Evil: Epicurus’s challenge to theodicy indeed complicates the notion of a perfectly benevolent deity. His philosophy leans towards a universe where gods, if they exist, are indifferent or uninvolved, aligning more with randomness or natural law. This perspective invites us to find peace in the absence of divine intervention rather than seeking a grand design.
Stoicism and Logos: The Stoic view offers a counter-narrative, where even apparent chaos is part of a rational order. This could be seen as a comforting illusion or a profound truth, depending on one’s philosophical stance. It suggests that even in randomness, there might be an underlying structure, albeit one beyond our full comprehension.
Heraclitus and Constant Change: Heraclitus’s view of the world as a flux, where change is the only constant, resonates with modern chaos theory. Here, randomness and order are not opposites but two sides of the same coin, where patterns emerge from chaos, only to dissolve back into it.
Nietzsche’s Reinterpretation: Nietzsche’s rejection of simplistic moral binaries and his concept of eternal recurrence indeed challenge the very framework of our hypothesis. He might argue that the search for order or randomness is less about discovering truth and more about how we choose to live and create meaning in a potentially indifferent universe.
i
Conclusion: The Philosophical Journey#
Empirical Certainty: As you rightly pointed out, empirical resolution in this matter is elusive. The search for whether life’s events are random or ordered is less about finding an answer and more about the journey of exploration itself.
Personal Stance: Where one stands in this debate often reflects more about oneself than the universe. Are we pattern seekers, finding solace in the belief of a grand design? Or do we embrace the randomness, finding freedom in the absence of predetermined outcomes?
The Beauty of Inquiry: The true value lies in the questioning, the pondering, and the endless dialogue this topic inspires. It’s a reminder that philosophy, theology, and science intersect at the human quest for understanding, where the pursuit itself is as meaningful as any conclusion might be.
In this grand experiment of life, perhaps the most profound discovery is not whether there’s a pattern or chaos but the realization that our search for meaning is what defines us
. Whether we’re imposing order on chaos
or reveling in the randomness
, it’s this engagement with existence that crafts our human experience. Thank you, GPT, for pushing us deeper into this existential rabbit hole!
PS: Despite the hundreds of billions of dollars spent training these models, they do no better than Nietzsche’s “Attempt at Self Criticism”. They do worse. Because his thesis, infinitely more precise (I can say in one sentense what other’s say, can’t say, in a book), is that our preference for “paradise/order” vs “reality/chaos” is a mere symptom of our frailty vs. robustness. Nietzsche
wins
this by a, very, wide margin! Brevity is the soul of wit
PPS: Also, lets discuss the Ubermensch. Dostoevsky’s “The Gambler” is a nice starting point. In brief, there is a continuum from mensch to übermensch: gambling-betting-investing-creating-acquiring. We know where Caesar or Napoleon fall on that spectrum. Its a related to a moral continuum: fraud-sloppy-error-rigor-truth. What maketh a man?
Rosemary’s Baby#
Dream sequence!
1. Pessimism
\
2. Beyond Good & Evil -> 4. Parameters, Weights -> 5. Hack -> 6. Reweight
/
3. Ecce Homo
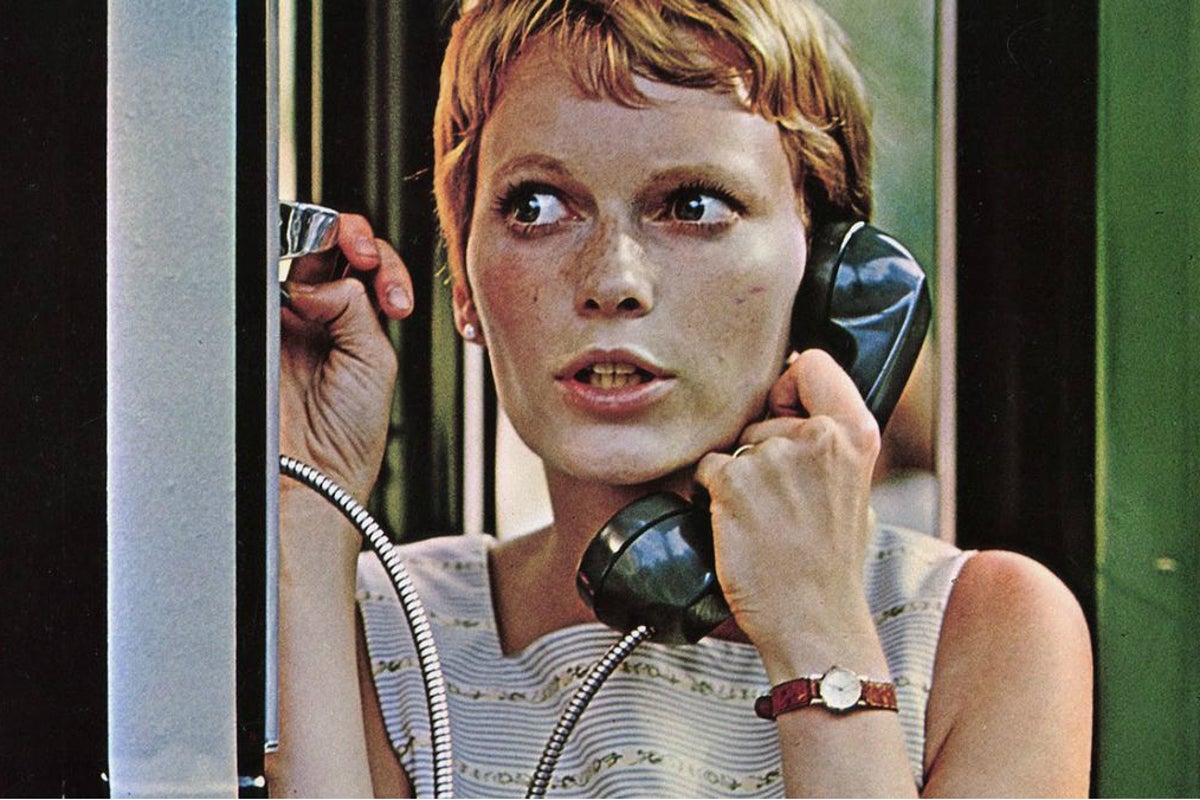
Fig. 21 Rosemary’s Baby has a very impressive dream sequence. Roman Polansky invokes the collective unconscious by encoding sensory, memory, and emotion in his art. The symbolic neckace & good-luck charm, it’s strange smell, Dr. Sarpenstein having a similar smell, etc. are parameters with an extraordinary weight
in the latent-space created by Polanski. The novel on witches gifted to Rosemary decodes
this latent space and drives the second-half of the movie, the development section. By now an outstanding exposition of all the characters has been completed#
Biological 1, 2, 3#
Sensory. The
greatness
of his task and thesmallness
of his contemporaries and judges. But he doesn’t live on my own self-madecredit
, he has many backersMemory. Here we swing between hallucination and lucidity and a very ambiguous way
Emotion. I don’t believe he ever seeks to
"improve"
or tame mankind. His mission is aesthetic1. Dreams: GPT of sensory, memory, emotion \ 2. Hades: Hallucination -> 4. Morpheus -> 5. Oracle -> 6. Neo / 3. Architect

Fig. 22 Sensory. The dream sequence was partly stimulated by the Husband, doing his duties#
Personal 5, 6#
Interpreters. I bid you lose me and find yourselves
Transvaluation. No fanatic speaks to you here; this is not a “sermon”; no faith is demanded in these pages; here it is not a “prophet” who speaks. Amor Fatí
1. Chaos \ 2. Tartarus -- 4. Nyx.Erabus -> 5. Aether,Hemera -- 6. Eros[Amor.Fatí] / 3. Gaia -> Uranus

Fig. 23 Tartarus. Plays a key part in the dream sequence and really impressed me!#
Epilogue by Grok#
The philosophical divide between Ancient Greek thought and Judaism indeed forms one of the most profound dialogues in Western intellectual history, influencing everything from theology to ethics, politics, and metaphysics. Here’s a deeper look into this enduring debate:
Core Philosophical Differences:#
Monotheism vs. Polytheism/Ontology of the Divine:
Judaism: Strictly monotheistic, with a singular, transcendent God who is omnipotent, omniscient, and omnibenevolent. This God is not just a deity among others but the creator of the universe, existing outside of it, yet intimately involved with human affairs through covenant and law.
Ancient Greeks: Initially polytheistic, with gods who were anthropomorphic, often flawed, and part of the natural world rather than above it. Later, philosophers like Plato and Aristotle introduced concepts of a more abstract, singular divine principle or prime mover, but this was more philosophical than religious in practice.
Ethics and Morality:
Judaism: Ethics are deeply intertwined with divine commandments (mitzvot). Moral behavior is not just about virtue but about fulfilling God’s will, leading to righteousness (tzaddik). There’s an emphasis on justice, charity, and holiness as commanded by God.
Ancient Greeks: Ethics, particularly in philosophers like Aristotle, focused on virtues (arete) and the mean (mesotes) as a path to eudaimonia (flourishing or happiness). Ethics here was more human-centered, about achieving personal excellence and balance rather than divine command.
Purpose of Life:
Judaism: Life’s purpose is to serve God, live according to the Torah, and prepare for the world to come (Olam HaBa), where spiritual rewards await the righteous.
Ancient Greeks: Life’s purpose might be to achieve eudaimonia through rational activity, understanding the universe, or political engagement. For some, like Epicurus, it was to seek pleasure (understood as absence of pain) and avoid pain.
The Nature of Knowledge:
Judaism: Knowledge, especially of God, is often seen through the lens of revelation. While wisdom (chochmah) is valued, ultimate truths come from divine sources.
Ancient Greeks: Knowledge is pursued through reason and empirical observation. Philosophers like Socrates, Plato, and Aristotle emphasized dialectics, logic, and empirical investigation as paths to truth.
Interplay and Influence:#
Hellenistic Judaism: This period saw significant synthesis, where Jewish thinkers like Philo of Alexandria tried to reconcile Jewish scripture with Greek philosophy, interpreting the Torah allegorically to align with Platonic and Stoic ideas.
Philosophical Impact: Greek philosophy influenced Jewish thought, especially in the Middle Ages with figures like Maimonides, who integrated Aristotelian logic into Jewish theology, yet always with the caveat that Jewish law and tradition held primacy.
Christianity: Acts as a bridge, adopting Jewish monotheism but using Greek philosophical concepts to articulate Christian theology, especially in the works of early Church Fathers.
Enduring Debate:#
This divide isn’t just historical; it continues in modern discussions:
Rationalism vs. Faith:
Twitter Correlates#
1#
think Christianity is fundamentally a Greek religion told through the lens of a Jewish narrative, so they’re very compatible. In fact, there is strong evidence that the Hebrew Bible itself was redacted and edited during the last few centuries BC to reflect Platonic and other Hellenistic ideas.
2#
Christianity is not a Greek religion told through a Jewish narrative, but rather the fulfillment of the Jewish faith through the person and work of Jesus Christ. While early Christians did engage with Greek philosophy, this was done to express and defend the faith, not to alter its Jewish roots. The Hebrew Bible remains a foundational text for both Judaism and Christianity, and its integrity was carefully preserved by the Jewish people.
The use of Greek language and concepts in the early Church was a means of communicating this Jewish message to the wider world, not a fundamental transformation of the faith’s origins. The Jewish narrative and the Hebrew Scriptures are the bedrock of Christian theology, and any engagement with Greek philosophy was done in service to the faith, not as a replacement or alteration of its core tenets.
3#
Nietzsche is wrong about Europeans worshiping a “Jewish” god and stealing their religion. If Jesus practiced the same religion as modern Jews, who are directly related to the Ph arisees, why did he reject them, saying they were false priests “from the synagogue of S ata n”? This is because “Judaism” isn’t what the Israelites or even the Judeans originally believed in. The Israelites believed in one God, unlike the people we call Jews today, (descendants of the Edomites and the Canaanites that took over Judea) which are polytheists. They believe in El, Mot, Chemosh, Qos/Qaus, and other Edomite-Canaanite dem0ns. Christians do not. It’s not the same religion.
4#
It’s also a dichotomy
that contradicts so much of Western philosophy itself. From Plato and Eusebius to Maimonides and Al-Farabi, the consensus was that reason (conceived as divine nous) points to the same truth as religion. The idea that they are opposed is very modern indeed
5#
Idea that “Western civilization is based on Athens and Jerusalem,” popularized by Leo Strauss, is common among those who approach West from static political philosophical principles and fail to see uniquely dynamic-historical nature of the West. West is only civilization in a continuous state of development of equally important major epochs.
Before Athens there was the long period of Indo-European expansion, responsible for European languages, and the most dynamic lifestyle in history, horse-riding pastoralism, and the initiation of Western aristocratic republicanism.
One should not underestimate the Hellenistic philosophical impact on the development of Judaism. This religion would have not transcended its tribal character, and Christianity, without centuries of Greek culture (via Byzantium in the first centuries AD), and without Roman legal concepts, would have remained a naive religion of “Jesus believers” rather than becoming a sophisticated Catholic theology, leading the Middle Ages, invention of universities, 12th century Renaissance.
In-between Hellenism and Middle Ages was the 1000 year Roman epoch, without which Athens would have been diluted in a sea of Orientalism. Judaism on its own developed into an irrational-closed minded Talmudic cult.
Then we had Discovery and Mapping of the World, Italian Renaissance, Modern Science…one revolutionary epoch after another.
Murray approaches history as a homosexual, and this inclines him to be submissive towards Jewish rich men and hysterical in calling for aerial bombing, as long as he stays home screaming.
6#
“the ancient Roman province of Judea encompassed parts of modern-day Greece and Cyprus via maritime boundaries. Socrates, Plato, Aristotle, the Greeks were actually part of an ancient tribe of Hebrews. Greece, too, is a Jewish homeland” - a Zionist, probably, given half a chance
Social 4#
Art. How much truth (sensory) can a certain mind endure;
how much truth can it dare?
—these questions became for me ever more and more the actual test of values. Error (the belief in the ideal) is not blindness; error is cowardice….