System#
Citizen Kane#
Case Study: Capturing the Essence of Citizen Kane through a Neural Network
Introduction#
Orson Welles’ Citizen Kane is a masterpiece, layered with themes of identity, commodification, and the adversarial nature of human relationships. The film’s central narrative—Charles Foster Kane’s pursuit of wealth, power, and the elusive “Rosebud”—is a rich ground for neural network interpretation. This case study demonstrates how the proposed neural network structure encapsulates the film’s intricate dynamics, visualizing the pathways from inputs (resourcefulness, resources) through the hidden layers (identity, tokenization, adversarial networks) to diverse outputs (joy, capital, and ecosystem).
Neural Network Layers and Themes in Citizen Kane#
Input Layer:
Resourcefulness and Resources represent Kane’s foundational capabilities and wealth, the starting points of his life’s trajectory.
These inputs symbolize the tension between Kane’s intrinsic potential (resourcefulness) and extrinsic wealth (resources).
Hidden Layer:
Identity (Self, Family, Community, Tribe): Reflects Kane’s fractured relationships and shifting allegiances, from his mother to his romantic partners and professional colleagues.
Tokenization/Commodification: Captures how Kane transforms relationships and ideals into commodities—his newspaper empire and even love become transactional.
Adversary Networks (Biological): Highlights the biological and psychological conflicts driving Kane’s adversarial interactions, including his competitive spirit and personal vendettas.
Output Layer:
Joy: The fleeting moments of true happiness, exemplified by childhood memories and his yearning for “Rosebud.”
Freude: A nod to collective joy and deeper fulfillment Kane fails to achieve.
Kapital: His amassed wealth and power, which dominate his life’s output but fail to satisfy.
Schaden: The destruction and harm Kane leaves in his wake, both to himself and others.
Ecosystem: The broader societal and environmental ripple effects of his actions, including the impact of his media empire.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
layers = {
'Input': ['Resourcefulness', 'Resources'],
'Hidden': [
'Identity (Self, Family, Community, Tribe)',
'Tokenization/Commodification',
'Adversary Networks (Biological)',
],
'Output': ['Joy', 'Freude', 'Kapital', 'Schaden', 'Ecosystem']
}
# Adjacency matrix defining the weight connections
weights = {
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Visualizing the Neural Network
def visualize_nn(layers, weights):
G = nx.DiGraph()
pos = {}
node_colors = []
# Add input layer nodes
for i, node in enumerate(layers['Input']):
G.add_node(node, layer=0)
pos[node] = (0, -i)
node_colors.append('lightgray')
# Add hidden layer nodes
for i, node in enumerate(layers['Hidden']):
G.add_node(node, layer=1)
pos[node] = (1, -i)
if node == 'Identity (Self, Family, Community, Tribe)':
node_colors.append('paleturquoise')
elif node == 'Tokenization/Commodification':
node_colors.append('lightgreen')
elif node == 'Adversary Networks (Biological)':
node_colors.append('lightsalmon')
# Add output layer nodes
for i, node in enumerate(layers['Output']):
G.add_node(node, layer=2)
pos[node] = (2, -i)
if node == 'Joy':
node_colors.append('paleturquoise')
elif node in ['Freude', 'Kapital', 'Schaden']:
node_colors.append('lightgreen')
elif node == 'Ecosystem':
node_colors.append('lightsalmon')
# Add edges based on weights
for i, in_node in enumerate(layers['Input']):
for j, hid_node in enumerate(layers['Hidden']):
G.add_edge(in_node, hid_node, weight=weights['Input-Hidden'][i, j])
for i, hid_node in enumerate(layers['Hidden']):
for j, out_node in enumerate(layers['Output']):
# Adjust thickness for specific edges
if hid_node == "Identity (Self, Family, Community, Tribe)" and out_node == "Kapital":
width = 6
elif hid_node == "Tokenization/Commodification" and out_node == "Kapital":
width = 6
elif hid_node == "Adversary Networks (Biological)" and out_node == "Kapital":
width = 6
else:
width = 1
G.add_edge(hid_node, out_node, weight=weights['Hidden-Output'][i, j], width=width)
# Draw the graph
plt.figure(figsize=(12, 8))
edge_labels = nx.get_edge_attributes(G, 'weight')
widths = [G[u][v]['width'] if 'width' in G[u][v] else 1 for u, v in G.edges()]
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=widths
)
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Visualizing Capital Gains Maximization")
plt.show()
visualize_nn(layers, weights)
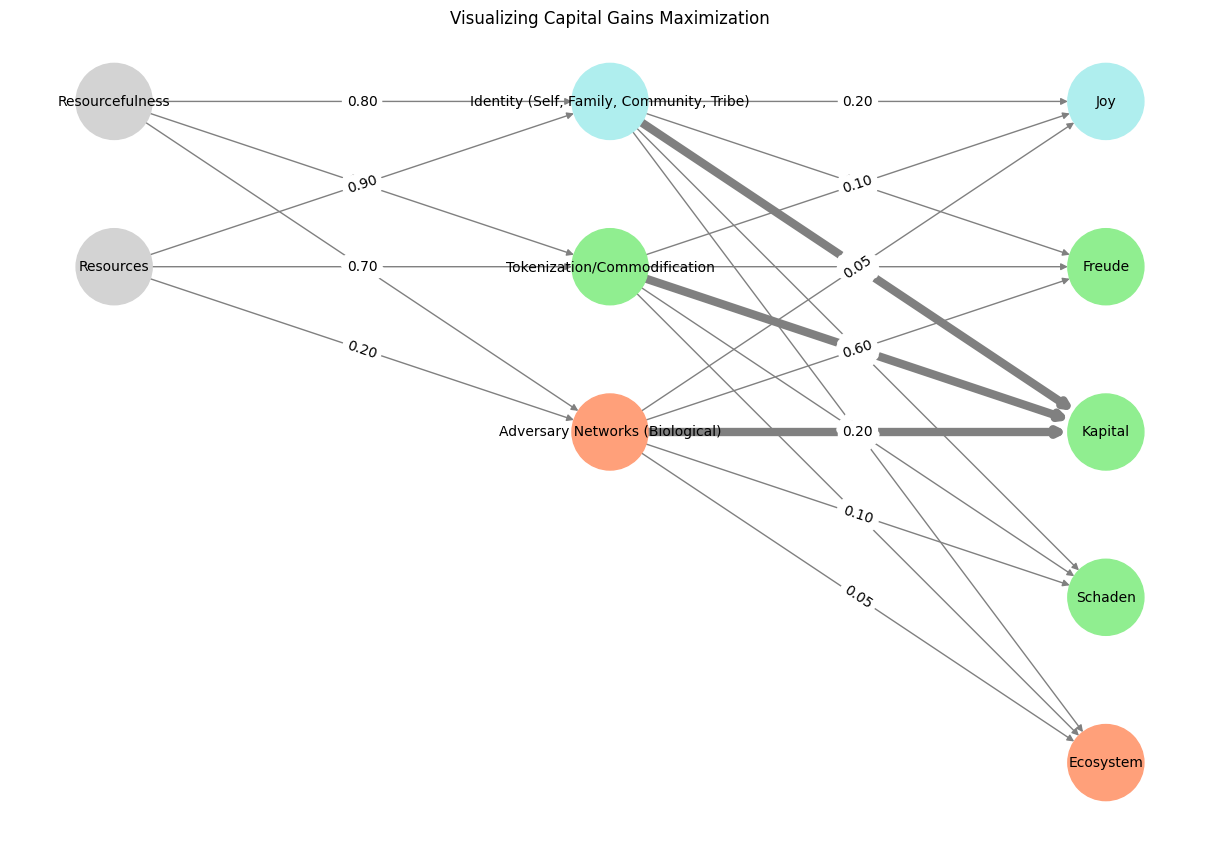
#
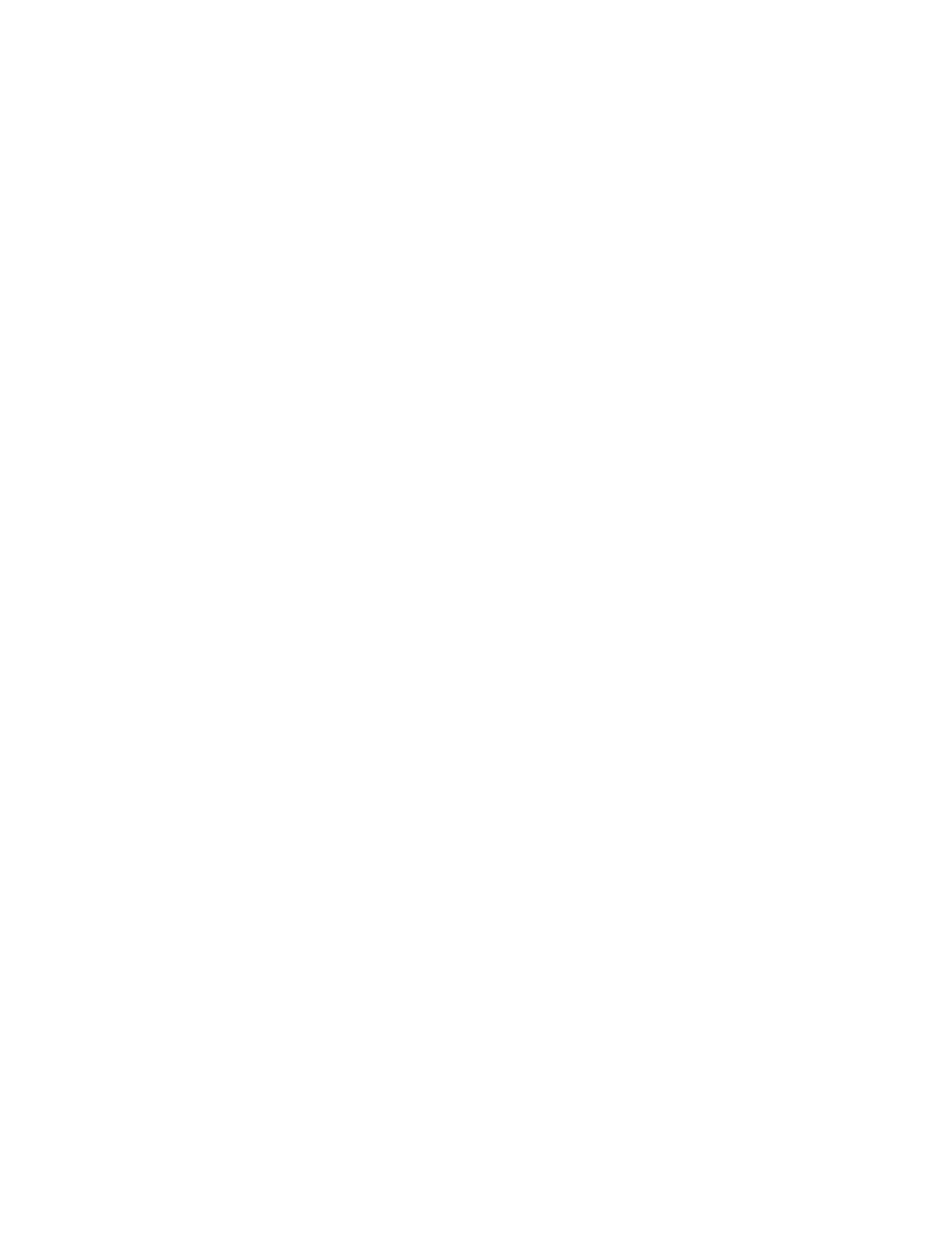
Fig. 11 This neural network marked a breakthrough, achieving clarity by compressing the input nodes into two. In the next iteration, it became evident that further compression into a single node was not only feasible but mirrored the human mind’s historical tendency to do so—encapsulated in the concept of God.#
Neural Network Visualization#
The neural network’s adjacency matrix and weighted connections align closely with the thematic structures of Citizen Kane. Key observations from the visualization include:
Strength of Identity and Tokenization:
The edges from Identity and Tokenization to Kapital have the heaviest weights, reflecting Kane’s obsession with commodification and material success. These pathways mirror the narrative arc of Kane sacrificing personal relationships and authenticity for power and wealth.
Adversarial Networks and Ecosystem:
The hidden node Adversary Networks contributes meaningfully to Kapital but also connects weakly to Ecosystem, suggesting the destructive externalities of Kane’s adversarial pursuits. His inability to reconcile these outputs symbolizes his failure to create a lasting, harmonious legacy.
Neglected Joy:
The relatively weak connections from Identity to Joy emphasize Kane’s disconnection from authentic happiness. Despite vast resources, the neural network highlights a systemic failure to channel these toward meaningful personal fulfillment.
Insights from the Model#
Compression of Complexity:
The model distills Citizen Kane’s multifaceted narrative into a structured framework, illustrating the flow from raw potential to outcomes, with the hidden layers serving as the locus of transformation and conflict.
Quantifying Tragedy:
The visualization starkly shows the dominance of tokenization and adversarial dynamics over pathways to joy, capturing the essence of Kane’s tragedy—a life spent accumulating everything except what truly mattered.
Emergent Themes:
The model’s Ecosystem output encapsulates the emergent societal impacts of Kane’s empire, an often-overlooked but critical aspect of the film’s legacy.
Conclusion#
This neural network framework elegantly captures the thematic depth of Citizen Kane, offering a novel lens for understanding the interplay between resources, identity, and outcomes. By visualizing these dynamics, the model not only pays homage to Welles’ cinematic genius but also underscores the timeless relevance of the film’s exploration of power, loss, and the human condition.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Life','Earth', 'Cosmos', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Rosebud'],
'Input': ['Evil', 'Good'],
'Hidden': [
'Islam',
'Narrator',
'Judeo-Christian',
],
'Output': ['Deeds', 'Discord', 'Kapital', 'Harmony', 'Ascetic', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Rosebud':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Good':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Judeo-Christian':
return 'paleturquoise'
elif node == 'Narrator':
return 'lightgreen'
elif node == 'Islam':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Ascetic':
return 'paleturquoise'
elif node in ['Harmony', 'Kapital', 'Discord']:
return 'lightgreen'
elif node == 'Deeds':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Nietzsche's Neural Network")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
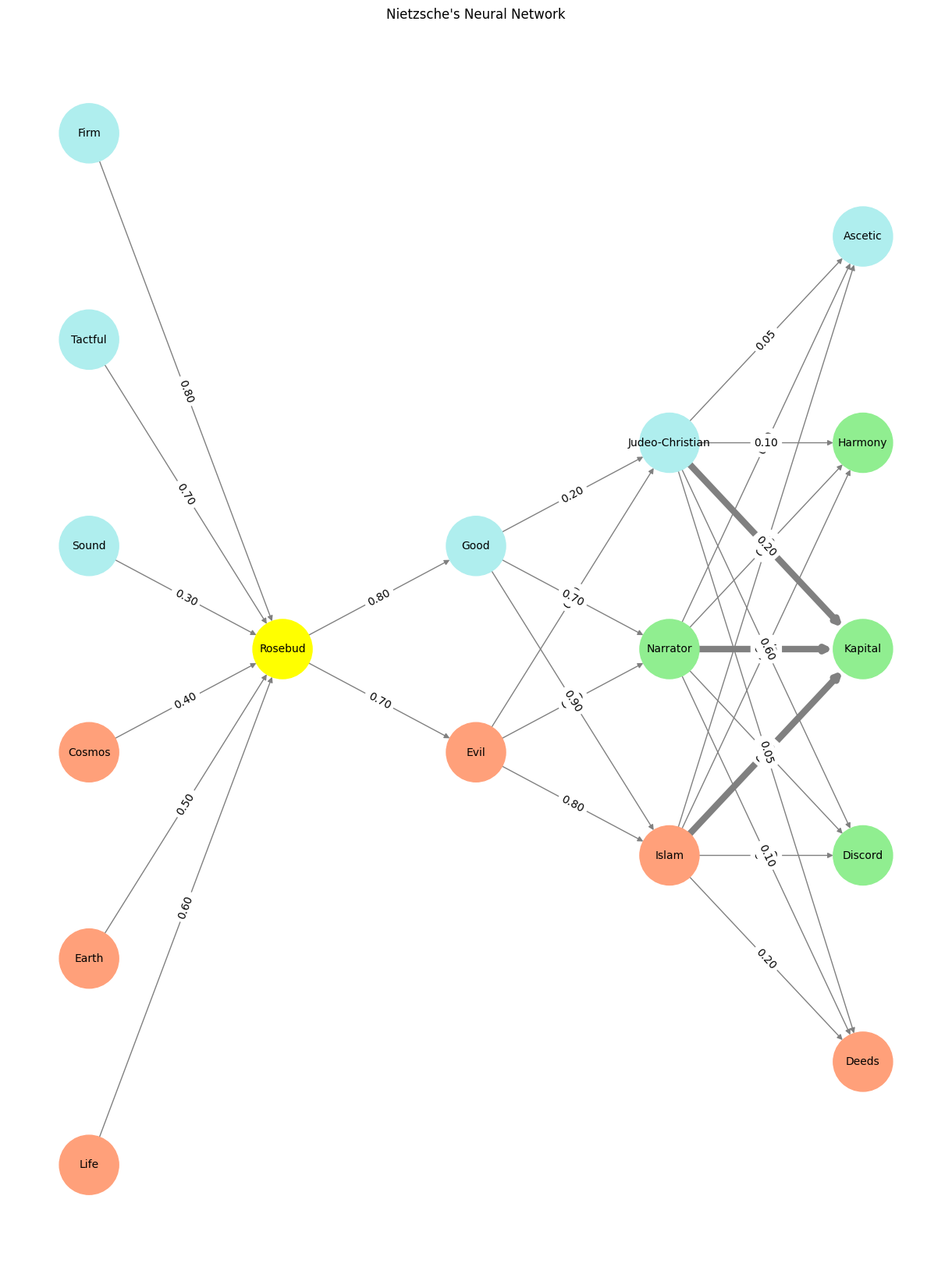
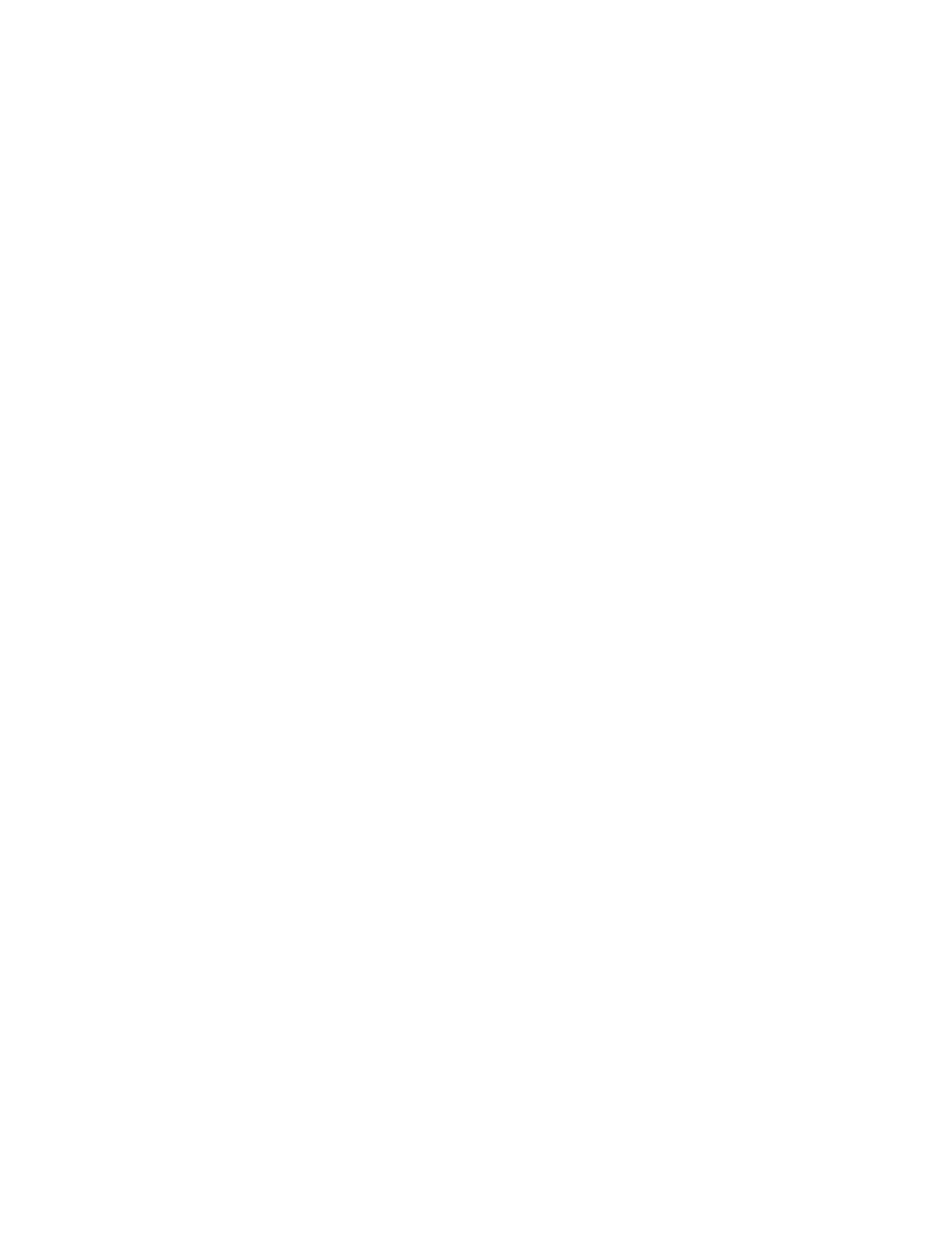
Fig. 12 This neural network was developed by iteratively backpropagating through the edges of the previous design (see above) and refining it until we achieved a well-optimized loss function.#
Backpropagated Climactic Rosebud to Reconfigure#
The Neural Network of Citizen Kane: An Essay on Rosebud, Discord, and Ascetic Harmony#
At its core, Citizen Kane is a story of profound neural and narrative compression—a journey through the vast expanse of a man’s life, distilled into the haunting simplicity of a single word: “Rosebud.” By reinterpreting Kane’s labyrinthine psyche through the structure of this neural network, we can uncover the interplay of biological, sociological, and psychological dynamics that shape his rise and fall.
Pre-Input Layer: Life, Earth, Cosmos, and the Foundations of Kane’s World#
The neural network’s “Pre-Input” layer represents the primordial forces—the unshaped cosmos of Charles Foster Kane’s early life. Here, “Life,” “Earth,” and “Cosmos” symbolize his biological and existential inheritance: the untamed Colorado wilderness, the goldmine that secures his family’s wealth, and the boundless possibilities it opens. Yet alongside this potential, there is the shaping force of “Sound,” “Tactful,” and “Firm”—qualities that anticipate the voice of authority and the rigid structures that will define Kane’s adult life.
“Rosebud,” as the central node in the “Yellowstone” layer, is born of this elemental substrate. It is not merely a sled or a token of lost innocence; it is the neural anchor that ties Kane’s ambition to his biological and emotional roots. Positioned between the cosmic (Pre-Input) and the moral (Input), it embodies the compression of a life lived between vast, unfathomable forces and the intimate tug of childhood memory.
Input Layer: Good, Evil, and the Moral Dualities of Kane#
The Input layer distills these primordial forces into a binary moral framework: “Good” and “Evil.” Kane’s choices, his public image as a crusader for social justice, and his private betrayals (of love, friendship, and trust) reflect this fundamental duality. The weighted connections from “Rosebud” to these nodes underscore its role as both a moral compass and a site of unresolved tension. The sled is a touchstone not because it represents good or evil but because it exists as a pre-moral fragment of identity, untouched by the later complexities of life.
Output Layer: Deeds, Discord, Kapital, Harmony, and Asceticism#
The final outputs of the network are the material and metaphysical consequences of Kane’s life.
Deeds: Kane’s tangible accomplishments, from building his media empire to his philanthropic gestures, resonate as achievements weighted heavily toward ego and self-expression.
Discord: This node captures the fractured relationships and ideological battles that mark Kane’s life. The widening gap between his public image and private despair is a direct output of unresolved conflicts in the Hidden layer.
Kapital: Kane’s wealth, symbolized as a node of immense influence, is both the enabler of his ambitions and the isolating force that alienates him from genuine human connection. Its connections to “Rosebud” and “Discord” highlight the ways in which material success fails to address existential needs.
Harmony: A fleeting, almost spectral output, Harmony is the dream of unity that Kane chases but never achieves. It exists as a faint echo of the “Good” in the Input layer, perpetually drowned out by the louder signals of Discord and Kapital.
Asceticism: Finally, Asceticism represents the renunciation Kane never achieves—a life stripped of excess and driven by inner peace. It is the paleturquoise node, serene and unattainable, contrasting sharply with the salmon tones of his deeds and discord.
Rosebud: The Keystone Node#
The node of “Rosebud,” glowing yellow in the network’s Yellowstone layer, is not merely a bridge between the layers but the crux of the entire system. Its connections to Kapital and Discord suggest its role as the unfulfilled promise of meaning—a reminder of Kane’s humanity buried beneath the machinery of wealth and power.
In Kane’s final moments, the sled burns not as a literal object but as a symbolic release of the network’s weighted complexity. The neural system collapses into a singularity, with Rosebud’s destruction marking the dissolution of Kane’s identity into the cosmos.
Conclusion: The Compression of Ambiguity into Emergence#
Through the lens of this neural network, Citizen Kane emerges as a story of compression and emergence. The ambiguity of Kane’s life—the conflicting narratives, the moral dualities, and the unattainable ideals—mirrors the structure of the network, where weighted connections and layered nodes translate complexity into emergent meaning.
Ultimately, Kane is not a man but a system: a collection of inputs shaped by biology and ambition, compressed by memory and morality, and outputted into the world as deeds, discord, and the persistent question of Rosebud. The genius of Welles’ film lies in its ability to compress this sprawling network into a single, burning word—a signal that echoes long after the network’s collapse.