Explore#
Let’s explore networks in biological, social, psychological, computational, and other realms. Networks are not merely diagrams of interconnected systems—they are the lifeblood of existence, the architecture of thought, and the scaffolding of human progress. Within their nodes and edges lies the delicate dance between chaos and order, the fundamental balance that defines our world.
This book is an invitation to navigate a polyphonic world of connections, to uncover patterns buried beneath layers of complexity, and to grasp the interplay between the tangible and the abstract. It is inspired by my journey through diverse domains—academic medicine, computational modeling, philosophical inquiry, and artistic interpretation—and by the realization that these seemingly disparate fields share the same foundational principles: equilibrium, transformation, and meaning.
More than any other course at any other university, more than any revered or resented private school, and in a manner probably unmatched in any other democracy, Oxford PPE pervades British political life. From the right to the left, from the centre ground to the fringes, from analysts to protagonists, consensus-seekers to revolutionary activists, environmentalists to ultra-capitalists, statists to libertarians, elitists to populists, bureaucrats to spin doctors, bullies to charmers, successive networks of PPEists have been at work at all levels of British politics – sometimes prominently, sometimes more quietly – since the degree was established 97 years ago.
— Andy Beckett
Networks, as I will examine them here, are not static structures but living systems—capable of growth and decay, harmony and discord. Biological networks reveal the elegance of embodied systems, from the intricate choreography of neurotransmitters in the brain to the resilience of ecosystems. Social and psychological networks expose the tensions between individual agency and collective dynamics, between identity and the structures that shape it. Computational networks, particularly those driving artificial intelligence, illuminate the creation of new, synthetic forms of understanding.
Each chapter delves into one of these realms, yet they converge on the same central question: how do systems maintain equilibrium, navigate disruptions, and achieve transformation? Whether it’s the resilience of a living kidney donor, the cultural interplay of archetypes in literature, or the algorithms that enable machines to ‘think,’ the answers lie in the connections themselves.
This book is also a response to the growing tokenization of intellectual pursuits—a call to reclaim meaning through embodiment and authentic engagement. It challenges the reduction of human creativity to metrics, urging instead for a deeper integration of the personal and the universal. Like the nodes of a neural network, we are most powerful when we embrace the feedback loops between our individual experiences and the broader systems we inhabit.
Ultimately, this work aims to inspire a new kind of curiosity—one that does not shy away from complexity but thrives on it. It is a journey toward understanding networks not as abstract diagrams but as dynamic, living entities shaping our world, our thoughts, and our futures. Let us begin by following these threads, weaving a tapestry that reflects not only what is but also what could be.
Show code cell source
import networkx as nx
import matplotlib.pyplot as plt
# Define input nodes, including specific neural effects of White Russian components
input_nodes_patient = [
'Oxytocin', 'Serotonin', 'Progesterone', 'Estrogen', 'Adenosine', 'Magnesium', 'Phonetics', 'Temperament',
'Degree', 'Scale', 'ATP', 'NAD+', 'Glutathion', 'Glutamate', 'GABA', 'Endorphin', 'Qualities',
'Extensions', 'Alterations', 'Dopamine', 'Caffeine', 'Testosterone', 'Noradrenaline', 'Adrenaline', 'Cortisol',
'Time', 'Military', 'Cadence', 'Pockets'
]
# Define hidden layer nodes as archetypal latent space with distinct archetypes
hidden_layer_labels_patient = [
'Elevation (Uplift)', 'Approbation (Validation)', 'Castration (Limitation)'
]
# Define output nodes for linear social validation hierarchy
output_nodes_patient = [
'Health', 'Family', 'Community', 'Local', 'Regional', 'NexToken', 'National', 'Global', 'Interstellar'
]
# Initialize graph
G_patient = nx.DiGraph()
# Add all nodes to the graph
G_patient.add_nodes_from(input_nodes_patient, layer='input')
G_patient.add_nodes_from(hidden_layer_labels_patient, layer='hidden')
G_patient.add_nodes_from(output_nodes_patient, layer='output')
# Define key narrative pathways (thickened edges)
thick_edges_patient = [
# Pathways emphasizing Elevation (Uplift)
('GABA', 'Elevation (Uplift)'), # Alcohol acting on GABA: relaxation, inhibition
('Adenosine', 'Elevation (Uplift)'), # Caffeine modulation of adenosine: alertness
('Oxytocin', 'Elevation (Uplift)'), # Social bonding, closeness
('Endorphin', 'Elevation (Uplift)'), # Shared humor and pleasure
('ATP', 'Elevation (Uplift)'), # Cream as an energy source
# Pathways emphasizing Approbation (Validation)
('Dopamine', 'Approbation (Validation)'), # Dopamine's role in reward-seeking and tokenization
('Caffeine', 'Approbation (Validation)'), ('Approbation (Validation)', 'NexToken'), # Drive for focus and achievement
# Pathways emphasizing Castration (Limitation)
('Testosterone', 'Castration (Limitation)'), # Symbolic castration as masculinity diminished or questioned
('Cortisol', 'Castration (Limitation)'), # Stress response undercutting resilience
('Adrenaline', 'Castration (Limitation)'), # Fight-or-flight as undermining composure
('Time', 'Castration (Limitation)'), # Limited temporal resources
('Military', 'Castration (Limitation)'), # Rigid hierarchies suppressing individuality
('Cadence', 'Castration (Limitation)'), # Enforced rhythm restricting freedom
('Pockets', 'Castration (Limitation)') # Symbolic lack of resources or containment
]
# Connect all input nodes to hidden layer archetypes
for input_node in input_nodes_patient:
for hidden_node in hidden_layer_labels_patient:
G_patient.add_edge(input_node, hidden_node)
# Connect hidden layer archetypes to output nodes
for hidden_node in hidden_layer_labels_patient:
for output_node in output_nodes_patient:
G_patient.add_edge(hidden_node, output_node)
# Define layout positions
pos_patient = {}
for i, node in enumerate(input_nodes_patient):
pos_patient[node] = ((i + 0.5) * 0.25, 0) # Input nodes at the bottom
for i, node in enumerate(output_nodes_patient):
pos_patient[node] = ((i + 1.5) * 0.6, 2) # Output nodes at the top
for i, node in enumerate(hidden_layer_labels_patient):
pos_patient[node] = ((i + 3) * 1, 1) # Hidden nodes in the middle layer
# Define color scheme for nodes based on archetypes and White Russian dynamics
node_colors_patient = [
'paleturquoise' if node in input_nodes_patient[:10] + hidden_layer_labels_patient[:1] + output_nodes_patient[:3] else
'lightgreen' if node in input_nodes_patient[10:20] + hidden_layer_labels_patient[1:2] + output_nodes_patient[3:6] else
'lightsalmon' if node in input_nodes_patient[20:] + hidden_layer_labels_patient[2:] + output_nodes_patient[6:] else
'lightgray'
for node in G_patient.nodes()
]
# Set edge widths with thickened lines for key narrative pathways
edge_widths_patient = [3 if edge in thick_edges_patient else 0.2 for edge in G_patient.edges()]
# Draw graph with rotated positions, thicker narrative edges, and archetypal colors
plt.figure(figsize=(14, 30))
pos_rotated = {node: (y, -x) for node, (x, y) in pos_patient.items()}
nx.draw(G_patient, pos_rotated, with_labels=True, node_size=3500, node_color=node_colors_patient,
font_size=9, font_weight='bold', arrows=True, width=edge_widths_patient)
# Add title and remove axes for clean visualization
plt.title("For on His Choice Depends The Sanctity and Health of this Whole State")
plt.axis('off')
plt.show()
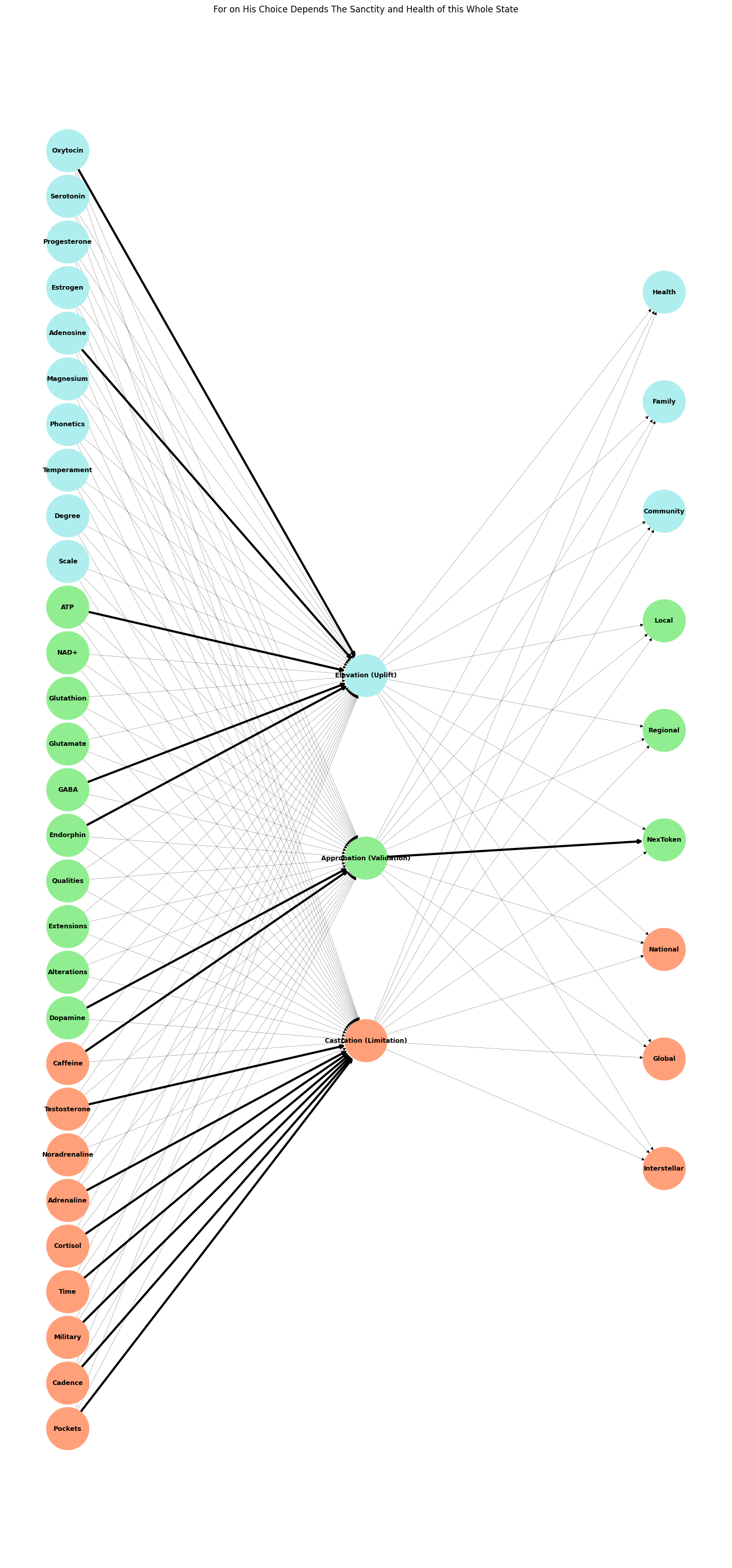
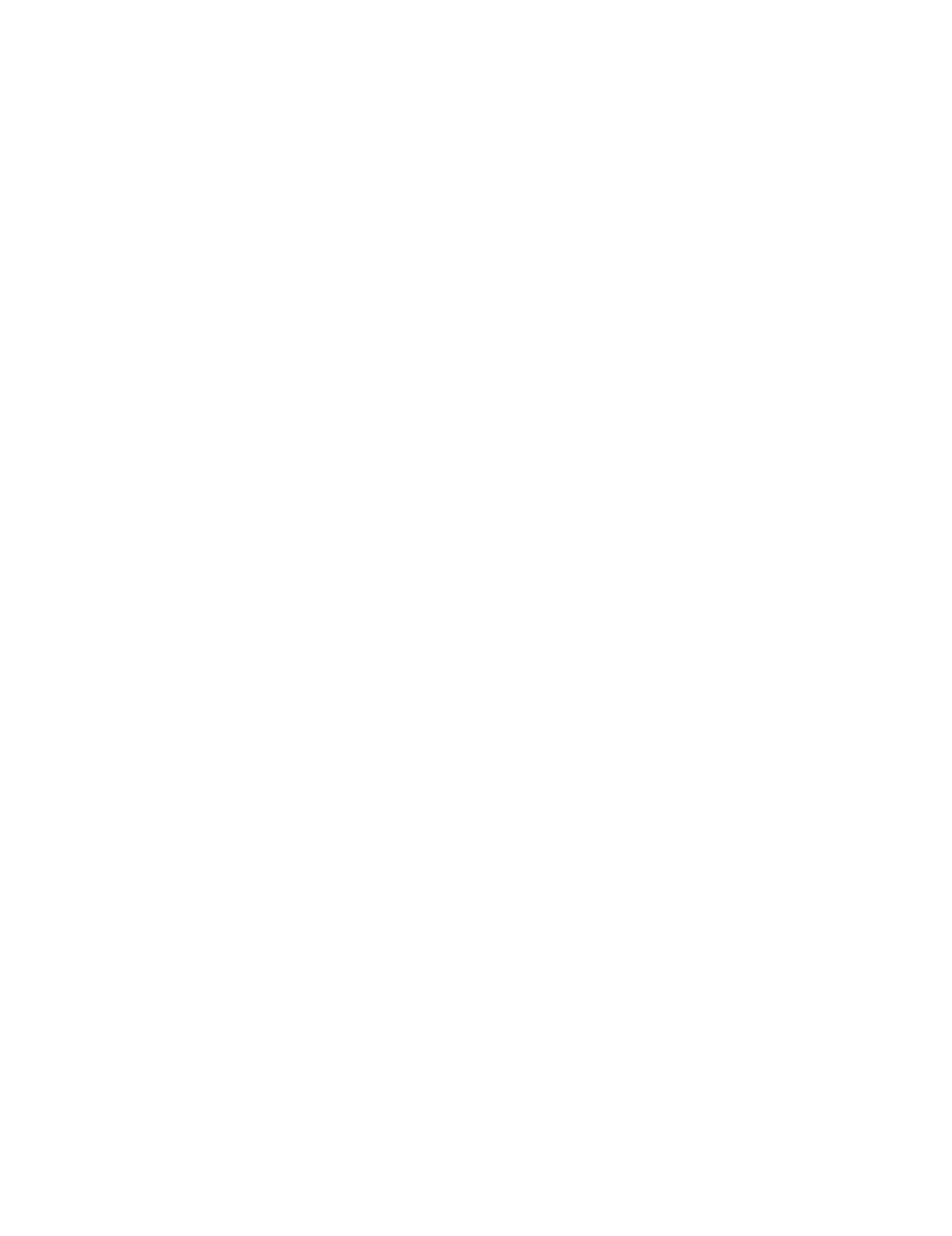
Fig. 1 Yes, Minister! Set primarily in the private office of a British cabinet minister at the fictional Department of Administrative Affairs in Whitehall, Yes Minister chronicles the ministerial career of Jim Hacker (Persona
), played by Paul Eddington. Hacker’s attempts to formulate and implement policy or drive departmental reform are consistently thwarted by the British Civil Service, embodied in the wily Permanent Secretary Sir Humphrey Appleby (Shadow
), played by Nigel Hawthorne. Caught in the crossfire is the Principal Private Secretary Bernard Woolley (Individuation
), played by Derek Fowlds, who must navigate the delicate balance between loyalty to both figures. By framing the characters within Jungian archetypes, we aim to provoke a more active engagement with the psychological underpinnings of the show’s comedic brilliance. But, as with much of comedy’s depth, who would have guessed the roots trace back to Jung? It’s also worth noting that the Minister is an LSE graduate whereas the civil servants are Oxford (PPE). Indeed, this training is steeped in game theory (economics), data science (politics), and neural networks (philosophy). For this reason, entry requirements include a AAA in A-levels, proficiency in Maths, Coding, and AI.#