Attribute#
The brilliance of Yes, Minister lies in its deft compression of bureaucratic farce into sharply observed strategic interplay, and Barnardâs quip to Sir Humphrey is a masterstroke of humor, wisdom, and subtle menace. Humphrey, as the embodiment of civil service cunning, has always thrived in environments of obfuscation, using his linguistic acrobatics and institutional memory to dazzle or daunt ministers into submission. But here, the dynamic shifts. The minister has matured, evolving beyond his earlier naivety. He now wields his own arsenal, bolstered by the addition of an equally formidable assistant. This development sets the stage for a rare moment of tension: Humphrey must approach the encounter not as a dominant manipulator, but as a wary adversary.
Barnardâs adviceârich in metaphor and hilariously layeredâreveals both his own bemused awareness of Humphreyâs formidable reputation and his schadenfreude at witnessing the tables turn. To âadopt a flexible postureâ is a veiled jab at Humphreyâs previous certainty. No longer can he assume the minister will fall neatly into his carefully constructed traps. Instead, he must bend with the new realities of the ministerâs intellectual and strategic growth, lest he snap under the pressure of confrontation. The suggestion to âkeep your ear to the groundâ underscores the need for vigilance. Humphrey, accustomed to commanding the narrative, now finds himself warned to listen carefully for the rumblings of resistance, a discomfiting shift for someone so used to dictating terms.
âCovering your retreatâ is an especially delicious barb. It implies not only that Humphrey might fail but that failure is a very real possibility, requiring a graceful escape plan. For a man like Humphrey, whose pride and mystique are his tools of trade, the prospect of retreat is unthinkableâand yet, here, it is both a strategic necessity and a humbling reality. Finally, âwatching your rearâ completes the set with a mix of practical advice and comic flair. The visual image is absurdâHumphrey, a man of impeccable composure, glancing nervously over his shoulder. Yet it also serves as a reminder that his usual blind spots, previously inconsequential, may now become fatal weaknesses.
This moment in Yes, Minister exemplifies the showâs genius for exploring the power dynamics of government with wit and precision. The interplay between the ministerâs newfound competence and Humphreyâs habitual machinations is not just comedicâitâs a study in adaptive strategy, illustrating how even the most entrenched power structures can be disrupted by resourcefulness and collaboration. Barnardâs advice, while delivered as a joke, is a profound encapsulation of the game at play: survival in a system where everyone is armed with their own cunning, and the balance of power is never as fixed as it seems.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'World': [
'Cosmos, Theogony', 'Earth, Greece', 'Life, Animals & Plants',
'Tacful: Sacrifice', 'Brute Strength', 'Clever Strategy'
],
'Perception': ['Owl: Surveillance'],
'Agency': ['Threats: Neighbors', 'Realm: City-State'],
'Generativity': [
'Acropolis: Parasitism', 'Olympus: Mutualism', 'Zeus: Commensalism'
],
'Physicality': [
'Sword: Offense', 'Serpent: Lethality', 'Horse: Retreat',
'Helmet: Immunity', 'Shield: Defense'
]
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Owl: Surveillance'],
'paleturquoise': [
'Clever Strategy', 'Realm: City-State', 'Zeus: Commensalism',
'Shield: Defense'
],
'lightgreen': [
'Brute Strength', 'Olympus: Mutualism', 'Helmet: Immunity',
'Horse: Retreat', 'Serpent: Lethality'
],
'lightsalmon': [
'CosmosX, Theogony', 'EarthX, Greece', 'Life, Animals & Plants',
'Tacful: Sacrifice', 'Acropolis: Parasitism', 'Sword: Offense',
'Threats: Neighbors'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Remix", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
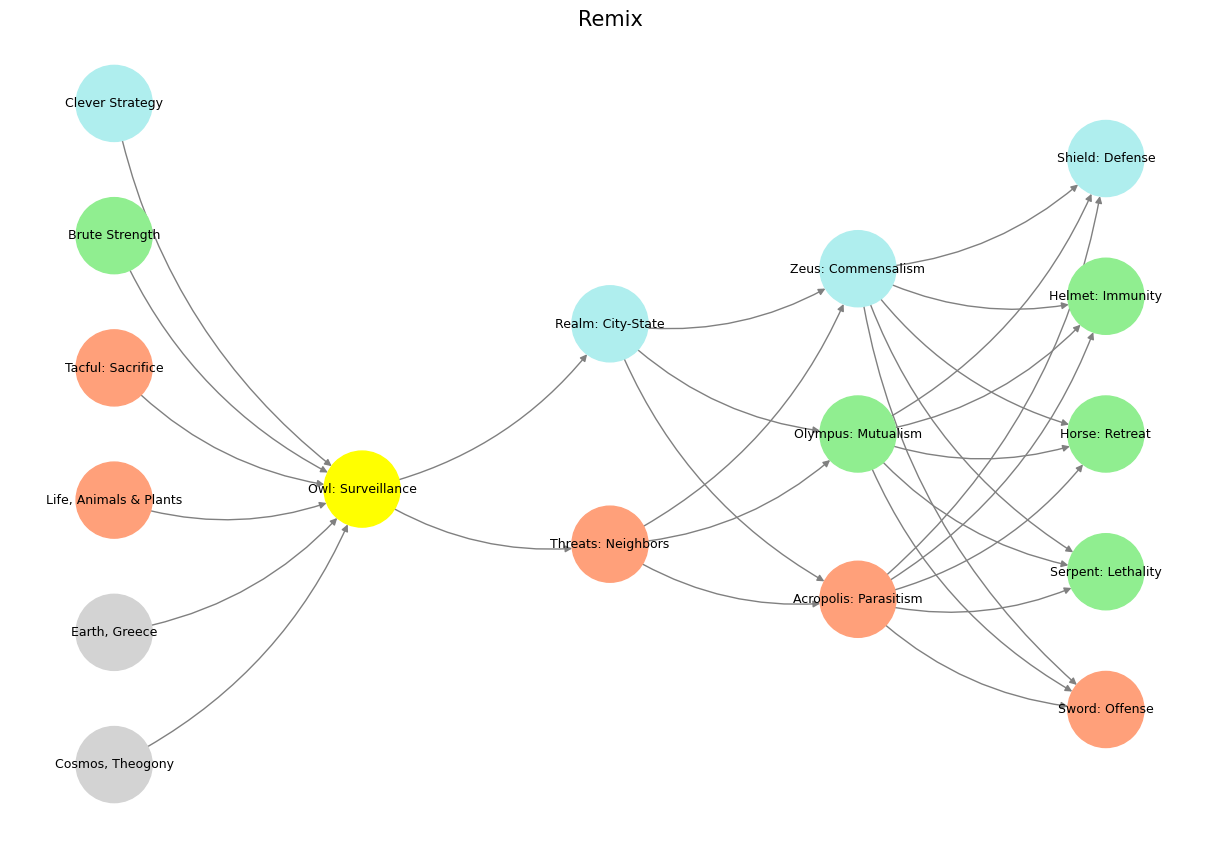
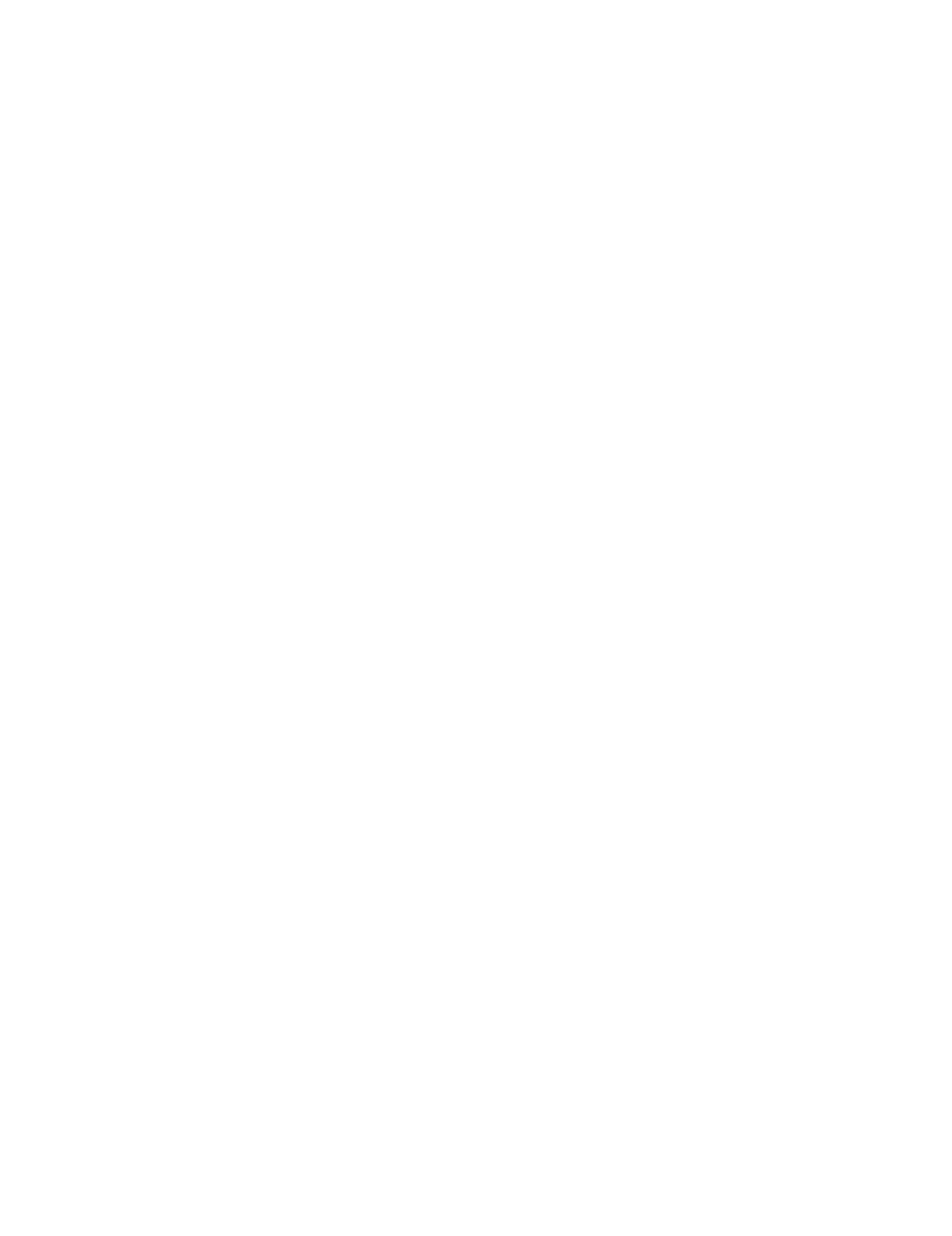
Fig. 25 Glenn Gould and Leonard Bernstein famously disagreed over the tempo and interpretation of Brahmsâ First Piano Concerto during a 1962 New York Philharmonic concert, where Bernstein, conducting, publicly distanced himself from Gouldâs significantly slower-paced interpretation before the performance began, expressing his disagreement with the unconventional approach while still allowing Gould to perform it as planned; this event is considered one of the most controversial moments in classical music history.#