Cosmic#
The Beauty of Metaphors: Framing the Infinite in the Finite#
Metaphors are the bridges between the known and the unknown, the finite and the infinite. They take abstract ideasātoo vast, too subtle, or too daunting to graspāand compress them into tangible forms. When we say life is like the branches of a tree, we do more than make a comparison; we evoke a universe of meaning, compressing the chaos of existence into a single, comprehensible image. It is in metaphors that language achieves its highest art, condensing time, space, and experience into expressions that resonate far beyond their literal scope. The beauty of metaphors lies not just in their elegance but in their ability to transform the way we think, connecting the most intricate ideas to the most primal instincts.
The metaphor of a tree, for example, carries within it the full arc of lifeāroots anchoring us to the past, branches reaching into the unknown future, and the inevitable pruning dictated by constraints. From the moment we are born, the infinite possibilities of our existence begin to narrow. Choices, circumstances, and biology act as gardeners, trimming away paths we will never take. This pruning is not a failure but a necessity; it gives shape to our lives, making them not chaotic masses of growth but purposeful forms. The tree metaphor reminds us that constraints, rather than limiting beauty, often create it. A perfectly pruned bonsai is not lesser for its constraintsāit is art because of them.
Metaphors themselves undergo a similar process of pruning and refinement. The best metaphors, the ones that endure, are not sprawling or overly ornate. They are precise, deliberate, shaped by the constraints of language and culture. Consider the metaphor of time as a river. Its simplicity belies its depth: the river flows forward, unstoppable, carrying us with it. Yet this metaphor also invites infinite extensions: the bends in the river, the eddies and whirlpools, the moments of calm and turbulence. It is through such metaphors that we make the incomprehensible vastness of time feel intimate, as if we are not merely passive observers but participants in its flow.
The power of metaphor extends beyond art and poetry; it is the cornerstone of human cognition. Our minds, limited by biology, rely on metaphor to compress the combinatorial explosion of reality into manageable concepts. Scientific breakthroughs often begin as metaphors. The atom as a solar system, genes as blueprints, and the mind as a computerāthese metaphors have shaped our understanding of the universe, enabling us to conceptualize phenomena far beyond our sensory experience. While metaphors may not capture the full truth, they serve as scaffolding, guiding us toward greater understanding.
In the modern world, where artificial intelligence compresses vast datasets into actionable insights, metaphors play a pivotal role in making this complexity intelligible. Machine learning algorithms are often described as āblack boxes,ā a metaphor that encapsulates their opacity and the mystery of their inner workings. While imperfect, this metaphor captures the essence of the problem, inviting further exploration. As machines grow more intelligent, they too rely on metaphorical thinking, using patterns and analogies to navigate the combinatorial space of possibilities. In this sense, metaphors are not just human tools; they are universal principles of compression and understanding.
But metaphors are not neutral; they carry ideological weight. The metaphors we choose shape the way we perceive the world. When we describe society as a machine, for instance, we imply a level of predictability and control that may not exist. Conversely, describing society as an ecosystem emphasizes interdependence and balance, acknowledging complexity and fragility. The metaphors of a culture reveal its values, fears, and aspirations. To question metaphors is to question the frameworks that define our reality.
The beauty of metaphors, then, lies in their dual nature. They are both constraints and liberations. Like the pruning of a tree, they narrow our focus to reveal form and structure. Yet, like the treeās branches, they also reach outward, extending our understanding into realms we might otherwise never explore. A metaphor is both a tool for survival and an invitation to transcendence, helping us navigate the finite world while pointing toward the infinite.
In an age of rapid technological and intellectual advancement, metaphors remain our most timeless tools. They connect disciplines, compressing the insights of physics, biology, and philosophy into forms that can be carried in a single thought. They democratize understanding, allowing ideas to leap across the boundaries of expertise and specialization. And they remind us of our humanity, grounding even the most abstract concepts in the sensory and emotional language of experience.
To say life is like the branches of a tree is to compress the vastness of existence into an image so simple it can be drawn by a child, yet so profound it can provoke a lifetime of reflection. It is in this compression, this simultaneous expansion and refinement, that metaphors achieve their beauty. They are our most elegant tools for making sense of the infinite, and in their delicate balance of constraint and possibility, they reflect the very nature of intelligence itself.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'World': ['Cosmos', 'Earth', 'Life', 'Cost', 'Parallel', 'Inheritence'],
'Framework': ['Cosmology', 'Geology', 'Biology', 'Agency', 'Space', 'Time'],
'Meta': ['Divine', 'Red Queen', 'Machine'],
'Perception': ['Perception'],
'Agency': ['Digital-Twin', 'Enterprise'],
'Generativity': ['Parasitism', 'Mutualism', 'Commensalism'],
'Physicality': ['Offense', 'Lethality', 'Retreat', 'Immunity', 'Defense']
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Perception'],
'paleturquoise': ['Inheritence', 'Time', 'Enterprise', 'Commensalism', 'Defense'],
'lightgreen': ['Parallel', 'Machine', 'Mutualism', 'Immunity', 'Retreat', 'Lethality', 'Space'],
'lightsalmon': [
'Cost', 'Life', 'Digital-Twin',
'Parasitism', 'Offense', 'Agency', 'Biology', 'Red Queen'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Define allowed connections between layers
def define_connections():
return {
'World': {
'Cosmos': ['Cosmology', 'Geology', 'Biology'],
'Earth': ['Geology', 'Biology', 'Agency', 'Space', 'Time'],
'Life': ['Biology', 'Agency'],
'Cost': ['Agency', 'Inheritence', 'Space', 'Time'],
'Parallel': ['Space', 'Inheritence', 'Time'],
'Inheritence': ['Time', 'Machine', 'Space', 'Agency']
},
'Framework': {
'Cosmology': ['Divine', 'Red Queen'],
'Geology': ['Red Queen', 'Machine'],
'Biology': ['Machine', 'Agency', 'Red Queen'],
'Agency': ['Divine', 'Red Queen', 'Machine'],
'Space': ['Divine', 'Machine'],
'Time': ['Machine', 'Red Queen']
},
'Meta': {
'Divine': ['Perception'],
'Red Queen': ['Perception'],
'Machine': ['Perception']
},
# Other layers default to fully connected if not specified
}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
connections = define_connections()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges based on controlled connections
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
if source_layer in connections:
for source, targets in connections[source_layer].items():
for target in targets:
if target in layers[target_layer]:
G.add_edge(source, target)
else: # Fully connect layers without specific connection rules
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Ensure node_colors matches the number of nodes in the graph
node_colors = [colors.get(node, 'lightgray') for node in G.nodes]
# Draw the graph
plt.figure(figsize=(14, 10))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Deep Neural Network", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
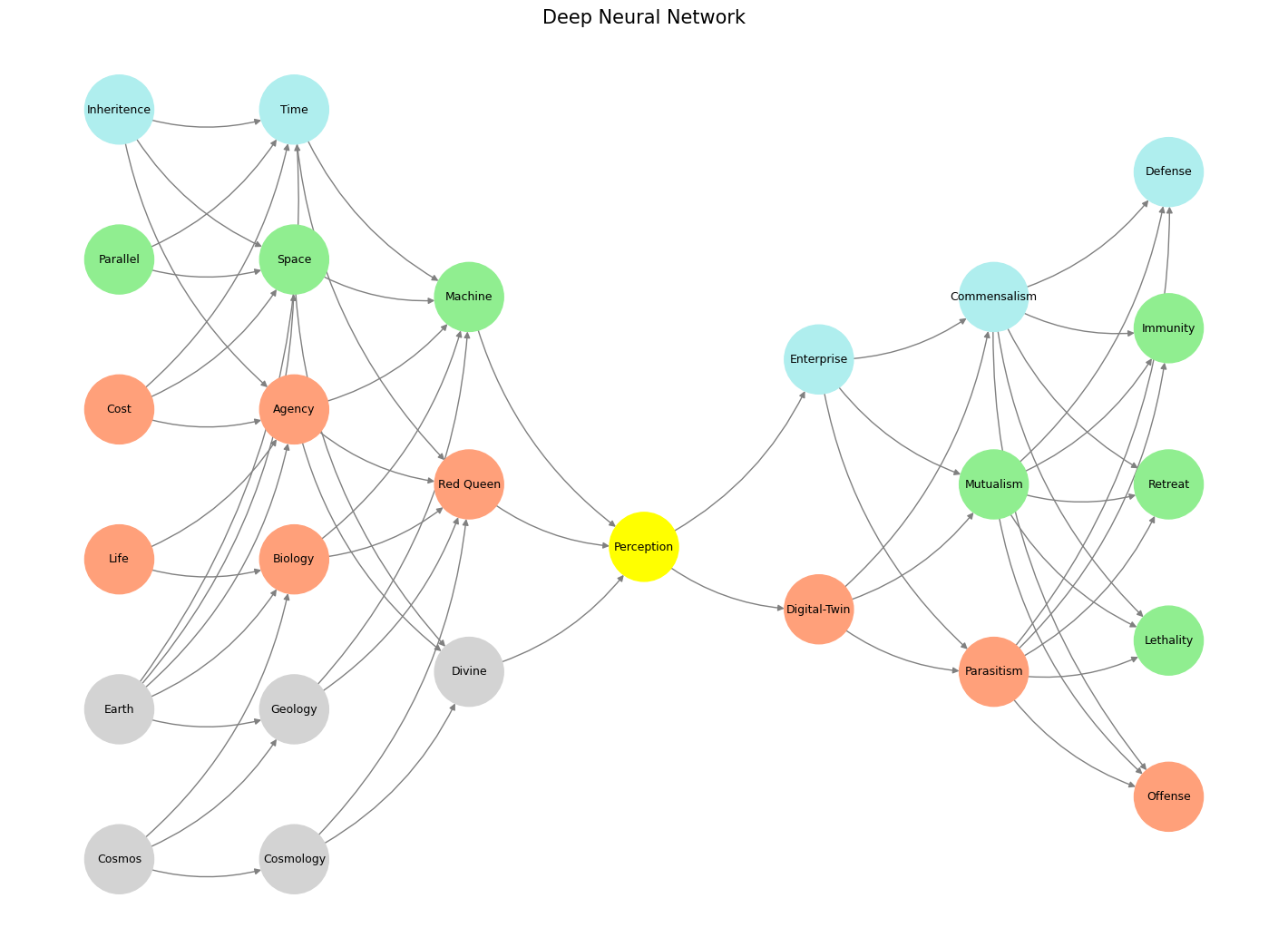
Fig. 18 Nvidia vs. Music. APIs between Nvidias CUDA & their clients (yellowstone node: G1 & G2) are here replaced by the ear-drum & vestibular apparatus. The chief enterprise in music is listening and responding (N1, N2, N3) as well as coordination and syncronization with others too (N4 & N5). Whether its classical or improvisational and participatory, a massive and infinite combinatorial landscape is available for composer, maestro, performer, audience. And who are we to say what exactly music optimizes?#