Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import weibull_min
# Weibull parameters for the two arms
c1, scale1 = 1.5, 10 # Control arm
c2, scale2 = 1.5, 15 # Treatment arm
# Define time range
t = np.linspace(0, 25, 500)
# Survival functions
S1 = weibull_min.sf(t, c1, scale=scale1)
S2 = weibull_min.sf(t, c2, scale=scale2)
# Hazard functions
h1 = weibull_min.pdf(t, c1, scale=scale1) / weibull_min.sf(t, c1, scale=scale1)
h2 = weibull_min.pdf(t, c2, scale=scale2) / weibull_min.sf(t, c2, scale=scale2)
# Density functions
f1 = weibull_min.pdf(t, c1, scale=scale1)
f2 = weibull_min.pdf(t, c2, scale=scale2)
# Median survival times
median1 = scale1 * (np.log(2))**(1/c1)
median2 = scale2 * (np.log(2))**(1/c2)
fig, axes = plt.subplots(1, 3, figsize=(18, 6))
# Plot Density function
axes[0].plot(t, f1, label='Control')
axes[0].plot(t, f2, label='Treatment')
axes[0].set_xlabel('Time')
axes[0].set_ylabel('Density Function')
axes[0].set_title('Density Function f(t)')
axes[0].legend()
axes[0].grid(True)
axes[0].spines['top'].set_visible(False)
axes[0].spines['right'].set_visible(False)
# Plot Survival function
axes[1].plot(t, S1, label='Control')
axes[1].plot(t, S2, label='Treatment')
# axes[1].axhline(0.5, color='gray', linestyle='--')
# axes[1].axvline(median1, color='blue', linestyle='--')
# axes[1].axvline(median2, color='orange', linestyle='--')
axes[1].set_xlabel('Time')
axes[1].set_ylabel('Survival Probability')
axes[1].set_title('Survival Function S(t)')
axes[1].legend()
axes[1].grid(True)
axes[1].spines['top'].set_visible(False)
axes[1].spines['right'].set_visible(False)
# Plot Hazard function
axes[2].plot(t, h1, label='Control')
axes[2].plot(t, h2, label='Treatment')
axes[2].set_xlabel('Time')
axes[2].set_ylabel('Hazard Function')
axes[2].set_title('Hazard Function h(t)')
axes[2].legend()
axes[2].grid(True)
axes[2].spines['top'].set_visible(False)
axes[2].spines['right'].set_visible(False)
# Highlight median survival times
for ax in axes:
ax.annotate('', xy=(median2, 0.5), xytext=(0, 0.5),
arrowprops=dict(arrowstyle='-|>', color='orange', lw=.5))
ax.annotate('', xy=(median2, 0.5), xytext=(median2, 0),
arrowprops=dict(arrowstyle='<|-', color='orange', lw=.5))
ax.annotate('', xy=(median1, 0.5), xytext=(0, 0.5),
arrowprops=dict(arrowstyle='-|>', color='blue', lw=.5))
ax.annotate('', xy=(median1, 0.5), xytext=(median1, 0),
arrowprops=dict(arrowstyle='<|-', color='blue', lw=.5))
ax.annotate(f'Median (Control) = {median1:.2f}', xy=(median1, 0.5), xytext=(median1 + .5, 0.55),
fontsize=12, color='blue')
ax.annotate(f'Median (Treatment) = {median2:.2f}', xy=(median2, 0.5), xytext=(median2 + 0, 0.42),
fontsize=12, color='orange')
plt.tight_layout()
plt.show()
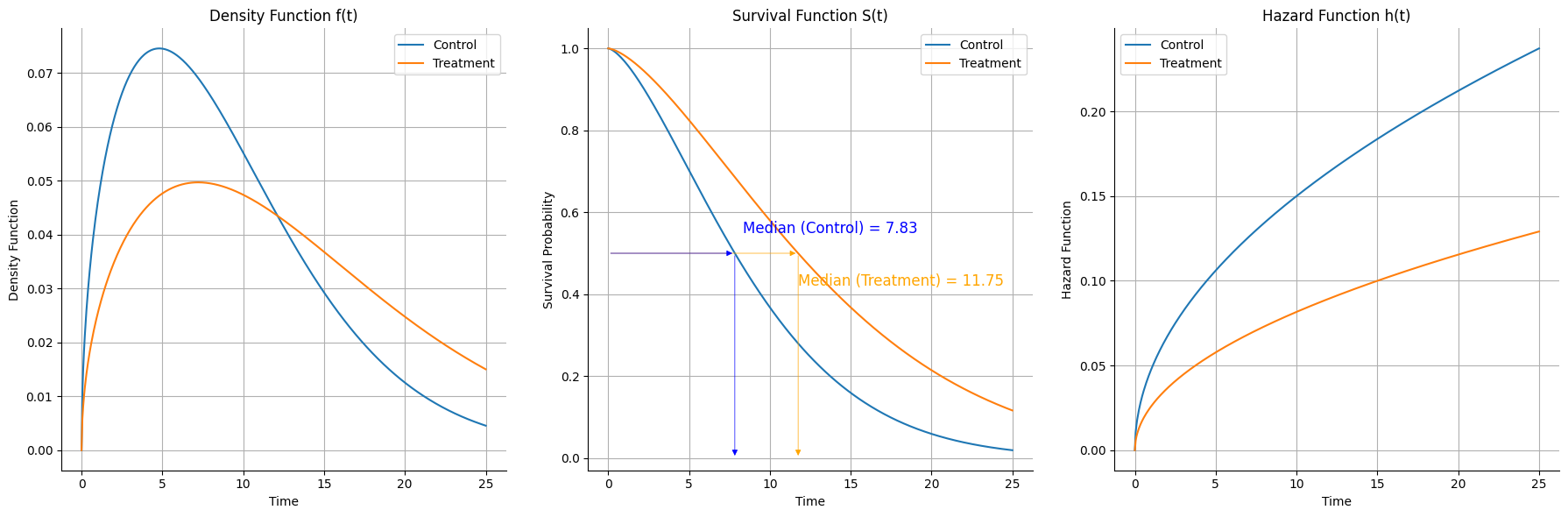
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
# Function to draw a hub and spoke diagram with specified node sizes and lengths
def draw_hub_and_spoke(hub_size, spoke_specs, filename):
# Define the hub position
hub = np.array([0, 0])
# Create the figure and axis
fig, ax = plt.subplots()
# Draw the hub
hub_circle = plt.Circle(hub, hub_size, color='white', ec='black', zorder=2)
ax.add_patch(hub_circle)
# Draw the spokes
for angle, (length, size) in spoke_specs:
# Calculate spoke position based on angle and length
spoke = np.array([length * np.cos(angle), length * np.sin(angle)])
# Draw line from hub to spoke
ax.plot([hub[0], spoke[0]], [hub[1], spoke[1]], 'k-', zorder=1)
# Draw spoke node
spoke_circle = plt.Circle(spoke, size, color='white', ec='black', zorder=2)
ax.add_patch(spoke_circle)
# Set aspect of the plot to be equal
ax.set_aspect('equal')
# Hide axes
ax.axis('off')
# Set limits to make sure everything fits in the plot
max_extent = max(length for angle, (length, size) in spoke_specs) + max(size for angle, (length, size) in spoke_specs)
ax.set_xlim([-max_extent, max_extent])
ax.set_ylim([-max_extent, max_extent])
# Save the plot to a file
plt.savefig(filename, bbox_inches='tight')
# Show the plot
plt.show()
hub_size = 1
# Specify the angles (in radians), lengths, and sizes for each spoke in clockwise order
spoke_specs = [
(np.pi / 2.3, (2.5, 0.4)), # first
(np.pi / 7, (3, 0.8)), # 2nd
(7 * np.pi / 3.8, (2.5, 0.5)), # 3rd
(3 * np.pi / 1.75, (5, 0.8)), # 4th
(5 * np.pi / 3.6, (3.9, 0.8)), # 3rd
(np.pi/ .97, (2.7, 0.6)), # 2nd
(3 * np.pi / 4.2, (3.8, 0.8)) # last
]
# Specify the filename to save the plot
filename = "hub_and_spoke.png"
# Call the function to draw the hub and spoke diagram and save it to a file
draw_hub_and_spoke(hub_size, spoke_specs, filename)
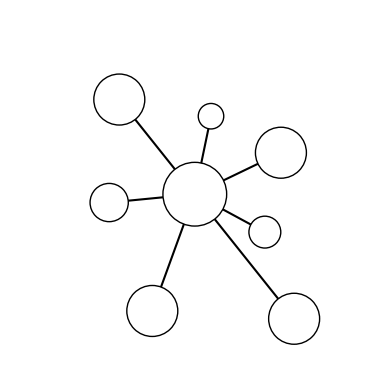
import numpy as np
print(np.pi)
3.141592653589793
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import norm, multinomial
# Time points
t = np.linspace(0, 10, 1000)
# PDFs: Normal distributions
f1 = norm.pdf(t, loc=3, scale=1)
f2 = norm.pdf(t, loc=5, scale=1)
f3 = norm.pdf(t, loc=7, scale=1)
# Multinomial density
pi = [0.3, 0.4, 0.3]
multi_density = multinomial.pmf([1, 0, 0], n=1, p=pi)
# Plotting the PDFs and Multinomial density
plt.figure(figsize=(14, 6))
# PDFs
plt.subplot(1, 2, 1)
plt.plot(t, f1, label='PDF 1: N(3,1)')
plt.plot(t, f2, label='PDF 2: N(5,1)')
plt.plot(t, f3, label='PDF 3: N(7,1)')
plt.title('Probability Density Functions')
plt.xlabel('Time')
plt.ylabel('Density')
plt.legend()
plt.grid(True)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
# Multinomial density
plt.subplot(1, 2, 2)
plt.bar(['PDF 1', 'PDF 2', 'PDF 3'], pi)
plt.title('Multinomial Density (π = [0.3, 0.4, 0.3])')
plt.xlabel('Outcome')
plt.ylabel('Probability')
plt.grid(True)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
plt.tight_layout()
plt.show()
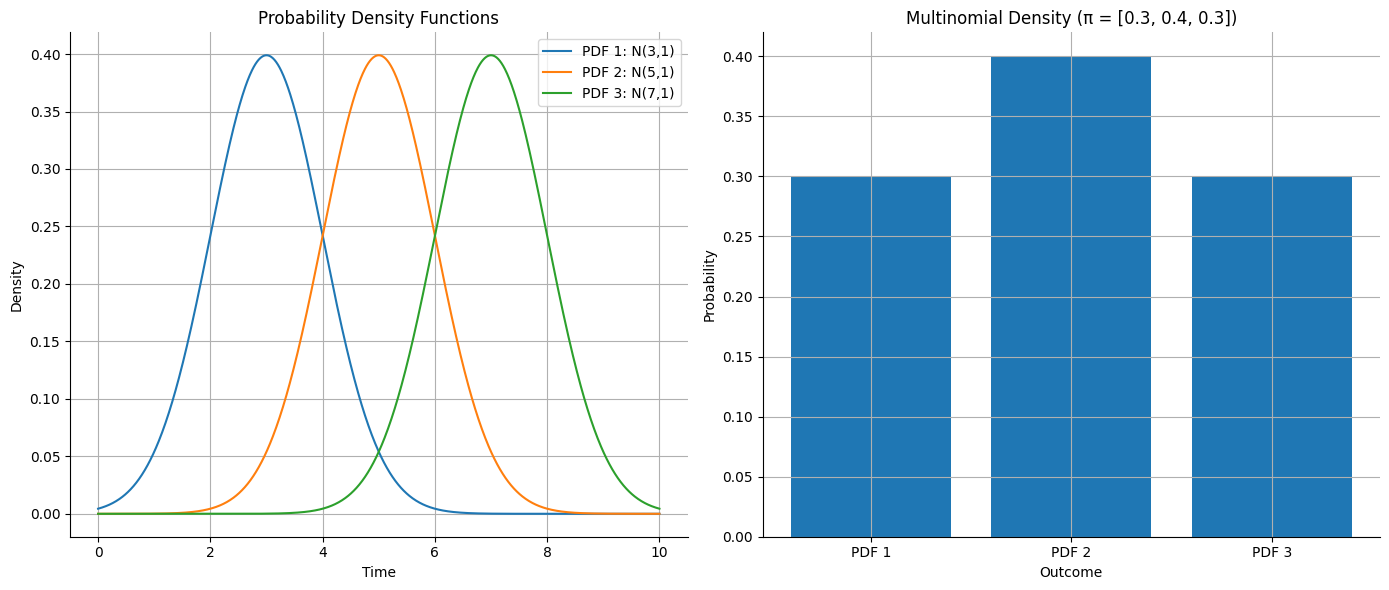
Show code cell source
# Survival functions
S1 = 1 - norm.cdf(t, loc=3, scale=1)
S2 = 1 - norm.cdf(t, loc=5, scale=1)
S3 = 1 - norm.cdf(t, loc=7, scale=1)
# Plotting the survival functions
plt.figure(figsize=(7, 6))
plt.plot(t, S1, label='Survival Function 1')
plt.plot(t, S2, label='Survival Function 2')
plt.plot(t, S3, label='Survival Function 3')
plt.title('Survival Functions')
plt.xlabel('Time')
plt.ylabel('Survival Probability')
plt.legend()
plt.grid(True)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
plt.show()
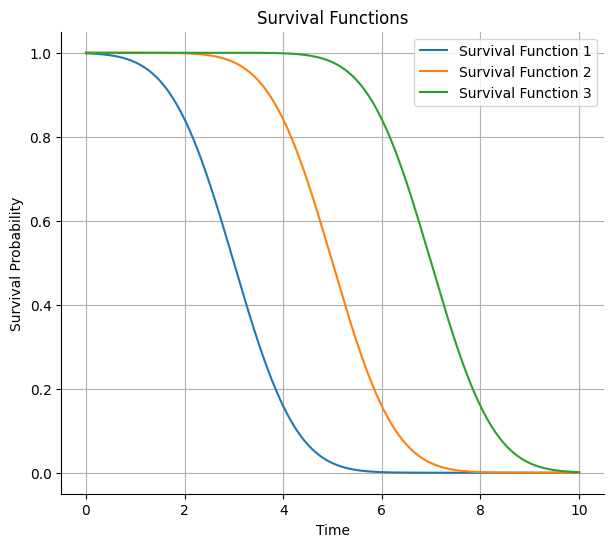
Show code cell source
# Hazard functions
h1 = f1 / S1
h2 = f2 / S2
h3 = f3 / S3
# Plotting the hazard functions
plt.figure(figsize=(7, 6))
plt.plot(t, h1, label='Hazard Function 1')
plt.plot(t, h2, label='Hazard Function 2')
plt.plot(t, h3, label='Hazard Function 3')
plt.title('Hazard Functions')
plt.xlabel('Time')
plt.ylabel('Hazard Rate')
plt.legend()
plt.grid(True)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
plt.show()
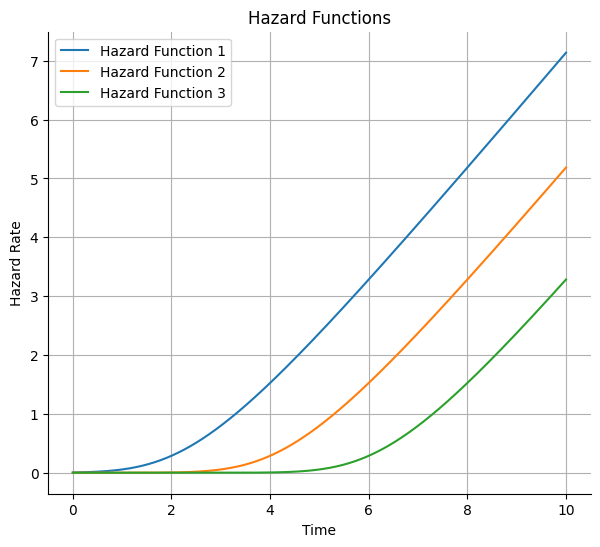
Show code cell source
# Simulate data
np.random.seed(0)
X = np.random.rand(100, 3)
Y = np.random.rand(100, 1)
# Compute beta coefficient vector
beta = np.linalg.inv(X.T @ X) @ X.T @ Y
beta
array([[0.17319547],
[0.4104582 ],
[0.25805577]])
# SVD
U, Sigma, Vt = np.linalg.svd(X)
# Visualize singular values
plt.figure(figsize=(7, 6))
plt.bar(range(len(Sigma)), Sigma)
plt.title('Singular Values')
plt.xlabel('Index')
plt.ylabel('Value')
plt.grid(True)
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
plt.show()
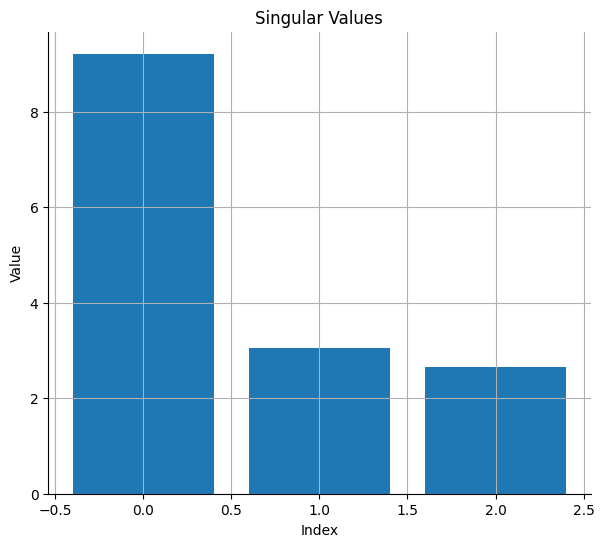
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
# Clock settings
clock_face_radius = 1.0
number_of_ticks = 8
tick_labels = [
"Egypt", "Assyria", "Babylon", "Persia",
"Greeks", "Rome", "Ottoman", "British"
]
# Calculate the angles for each tick (in radians)
angles = np.linspace(0, 2 * np.pi, number_of_ticks, endpoint=False)
# Inverting the order to make it counterclockwise
angles = angles[::-1]
# Create figure and axis
fig, ax = plt.subplots(figsize=(8, 8))
ax.set_xlim(-1.2, 1.2)
ax.set_ylim(-1.2, 1.2)
ax.set_aspect('equal')
# Draw the clock face
clock_face = plt.Circle((0, 0), clock_face_radius, color='lightgrey', fill=True)
ax.add_patch(clock_face)
# Draw the ticks and labels
for angle, label in zip(angles, tick_labels):
x = clock_face_radius * np.cos(angle)
y = clock_face_radius * np.sin(angle)
# Draw the tick
ax.plot([0, x], [0, y], color='black')
# Positioning the labels slightly outside the clock face
label_x = 1.1 * clock_face_radius * np.cos(angle)
label_y = 1.1 * clock_face_radius * np.sin(angle)
# Adjusting label alignment based on its position
ha = 'center'
va = 'center'
if np.cos(angle) > 0:
ha = 'left'
elif np.cos(angle) < 0:
ha = 'right'
if np.sin(angle) > 0:
va = 'bottom'
elif np.sin(angle) < 0:
va = 'top'
ax.text(label_x, label_y, label, horizontalalignment=ha, verticalalignment=va, fontsize=10)
# Remove axes
ax.axis('off')
# Show the plot
plt.show()
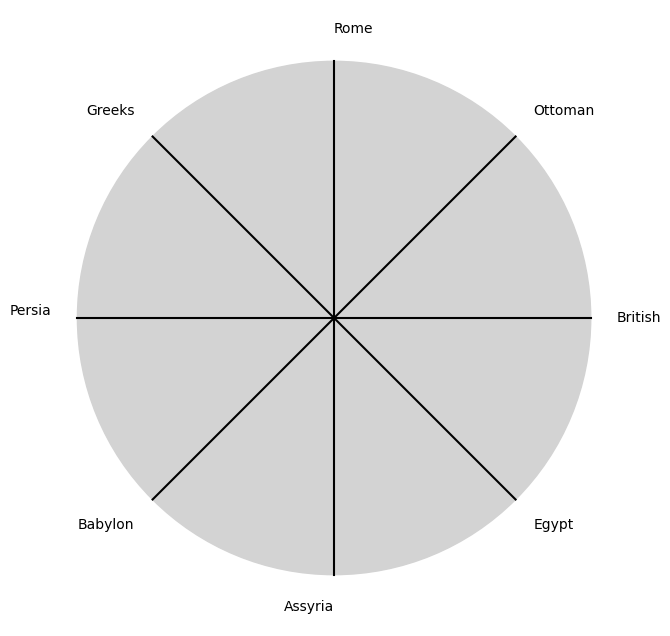
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
# Parameters
sample_rate = 44100 # Hz
duration = 20.0 # seconds
A4_freq = 440.0 # Hz
# Time array
t = np.linspace(0, duration, int(sample_rate * duration), endpoint=False)
# Fundamental frequency (A4)
signal = np.sin(2 * np.pi * A4_freq * t)
# Adding overtones (harmonics)
harmonics = [2, 3, 4, 5, 6, 7, 8, 9] # First few harmonics
amplitudes = [0.5, 0.25, 0.15, 0.1, 0.05, 0.03, 0.01, 0.005] # Amplitudes for each harmonic
for i, harmonic in enumerate(harmonics):
signal += amplitudes[i] * np.sin(2 * np.pi * A4_freq * harmonic * t)
# Perform FFT (Fast Fourier Transform)
N = len(signal)
yf = np.fft.fft(signal)
xf = np.fft.fftfreq(N, 1 / sample_rate)
# Plot the frequency spectrum
plt.figure(figsize=(12, 6))
plt.plot(xf[:N//2], 2.0/N * np.abs(yf[:N//2]), color='navy', lw=1.5)
# Aesthetics improvements
plt.title('Simulated Frequency Spectrum of A440 on a Grand Piano', fontsize=16, weight='bold')
plt.xlabel('Frequency (Hz)', fontsize=14)
plt.ylabel('Amplitude', fontsize=14)
plt.xlim(0, 4186) # Limit to the highest frequency on a piano (C8)
plt.ylim(0, None)
# Remove top and right spines
plt.gca().spines['top'].set_visible(False)
plt.gca().spines['right'].set_visible(False)
# Customize ticks
plt.xticks(fontsize=12)
plt.yticks(fontsize=12)
# Light grid
plt.grid(color='grey', linestyle='--', linewidth=0.5)
# Show the plot
plt.tight_layout()
plt.show()
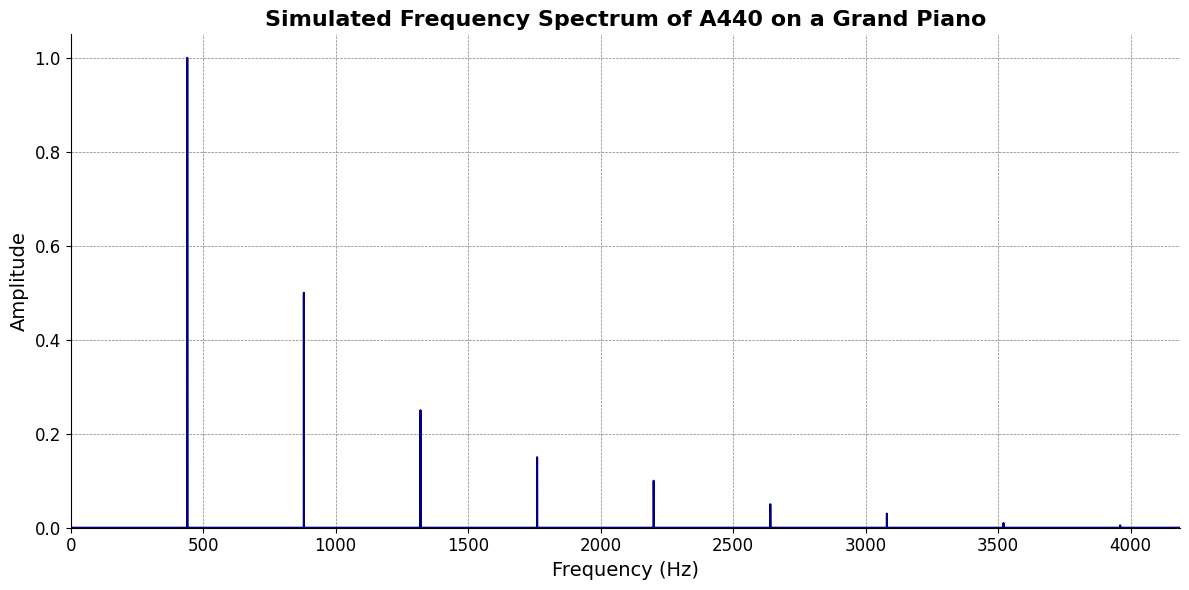
Show code cell source
# Recreate the plot with the specified style
plt.figure(figsize=(12, 6))
plt.plot(stages_of_life, growth_rate, marker='o', linestyle='-', color='blue')
# Remove upper and right borders
ax = plt.gca()
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
# Add labels and title
plt.xlabel('Stage of Life')
plt.ylabel('Rate of Cell-Division/Growth')
plt.title('Rate of Cell-Division/Growth from Conceptus to 100 Years')
# Annotate the stages
for i, txt in enumerate(growth_rate):
plt.annotate(txt, (stages_of_life[i], growth_rate[i]), textcoords="offset points", xytext=(0,10), ha='center')
# Add a very light dotted grid
plt.grid(True, which='both', linestyle=':', linewidth=0.5)
plt.show()
---------------------------------------------------------------------------
NameError Traceback (most recent call last)
Cell In[11], line 4
1 # Recreate the plot with the specified style
3 plt.figure(figsize=(12, 6))
----> 4 plt.plot(stages_of_life, growth_rate, marker='o', linestyle='-', color='blue')
6 # Remove upper and right borders
7 ax = plt.gca()
NameError: name 'stages_of_life' is not defined
<Figure size 1200x600 with 0 Axes>
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.cm import ScalarMappable
from matplotlib.colors import LinearSegmentedColormap, PowerNorm
def gaussian(x, mean, std_dev, amplitude=1):
return amplitude * np.exp(-0.9 * ((x - mean) / std_dev) ** 2)
def overlay_gaussian_on_line(ax, start, end, std_dev):
x_line = np.linspace(start[0], end[0], 100)
y_line = np.linspace(start[1], end[1], 100)
mean = np.mean(x_line)
y = gaussian(x_line, mean, std_dev, amplitude=std_dev)
ax.plot(x_line + y / np.sqrt(2), y_line + y / np.sqrt(2), color='red', linewidth=2.5)
fig, ax = plt.subplots(figsize=(10, 10))
intervals = np.linspace(0, 100, 11)
custom_means = np.linspace(1, 23, 10)
custom_stds = np.linspace(.5, 10, 10)
# Change to 'viridis' colormap to get gradations like the older plot
cmap = plt.get_cmap('viridis')
norm = plt.Normalize(custom_stds.min(), custom_stds.max())
sm = ScalarMappable(cmap=cmap, norm=norm)
sm.set_array([])
median_points = []
for i in range(10):
xi, xf = intervals[i], intervals[i+1]
x_center, y_center = (xi + xf) / 2 - 20, 100 - (xi + xf) / 2 - 20
x_curve = np.linspace(custom_means[i] - 3 * custom_stds[i], custom_means[i] + 3 * custom_stds[i], 200)
y_curve = gaussian(x_curve, custom_means[i], custom_stds[i], amplitude=15)
x_gauss = x_center + x_curve / np.sqrt(2)
y_gauss = y_center + y_curve / np.sqrt(2) + x_curve / np.sqrt(2)
ax.plot(x_gauss, y_gauss, color=cmap(norm(custom_stds[i])), linewidth=2.5)
median_points.append((x_center + custom_means[i] / np.sqrt(2), y_center + custom_means[i] / np.sqrt(2)))
median_points = np.array(median_points)
ax.plot(median_points[:, 0], median_points[:, 1], '--', color='grey')
start_point = median_points[0, :]
end_point = median_points[-1, :]
overlay_gaussian_on_line(ax, start_point, end_point, 24)
ax.grid(True, linestyle='--', linewidth=0.5, color='grey')
ax.set_xlim(-30, 111)
ax.set_ylim(-20, 87)
# Create a new ScalarMappable with a reversed colormap just for the colorbar
cmap_reversed = plt.get_cmap('viridis').reversed()
sm_reversed = ScalarMappable(cmap=cmap_reversed, norm=norm)
sm_reversed.set_array([])
# Existing code for creating the colorbar
cbar = fig.colorbar(sm_reversed, ax=ax, shrink=1, aspect=90)
# Specify the tick positions you want to set
custom_tick_positions = [0.5, 5, 8, 10] # example positions, you can change these
cbar.set_ticks(custom_tick_positions)
# Specify custom labels for those tick positions
custom_tick_labels = ['5', '3', '1', '0'] # example labels, you can change these
cbar.set_ticklabels(custom_tick_labels)
# Label for the colorbar
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
# Label for the colorbar
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
# Add X and Y axis labels with custom font styles
ax.set_xlabel(r'Principal Component', fontstyle='italic')
ax.set_ylabel(r'Emotional State', rotation=0, fontstyle='italic', labelpad=15)
# Add musical modes as X-axis tick labels
# musical_modes = ["Ionian", "Dorian", "Phrygian", "Lydian", "Mixolydian", "Aeolian", "Locrian"]
greek_letters = ['α', 'β','γ', 'δ', 'ε', 'ζ', 'η'] # 'θ' , 'ι', 'κ'
mode_positions = np.linspace(ax.get_xlim()[0], ax.get_xlim()[1], len(greek_letters))
ax.set_xticks(mode_positions)
ax.set_xticklabels(greek_letters, rotation=0)
# Add moods as Y-axis tick labels
moods = ["flow", "control", "relaxed", "bored", "apathy","worry", "anxiety", "arousal"]
mood_positions = np.linspace(ax.get_ylim()[0], ax.get_ylim()[1], len(moods))
ax.set_yticks(mood_positions)
ax.set_yticklabels(moods)
# ... (rest of the code unchanged)
plt.tight_layout()
plt.show()
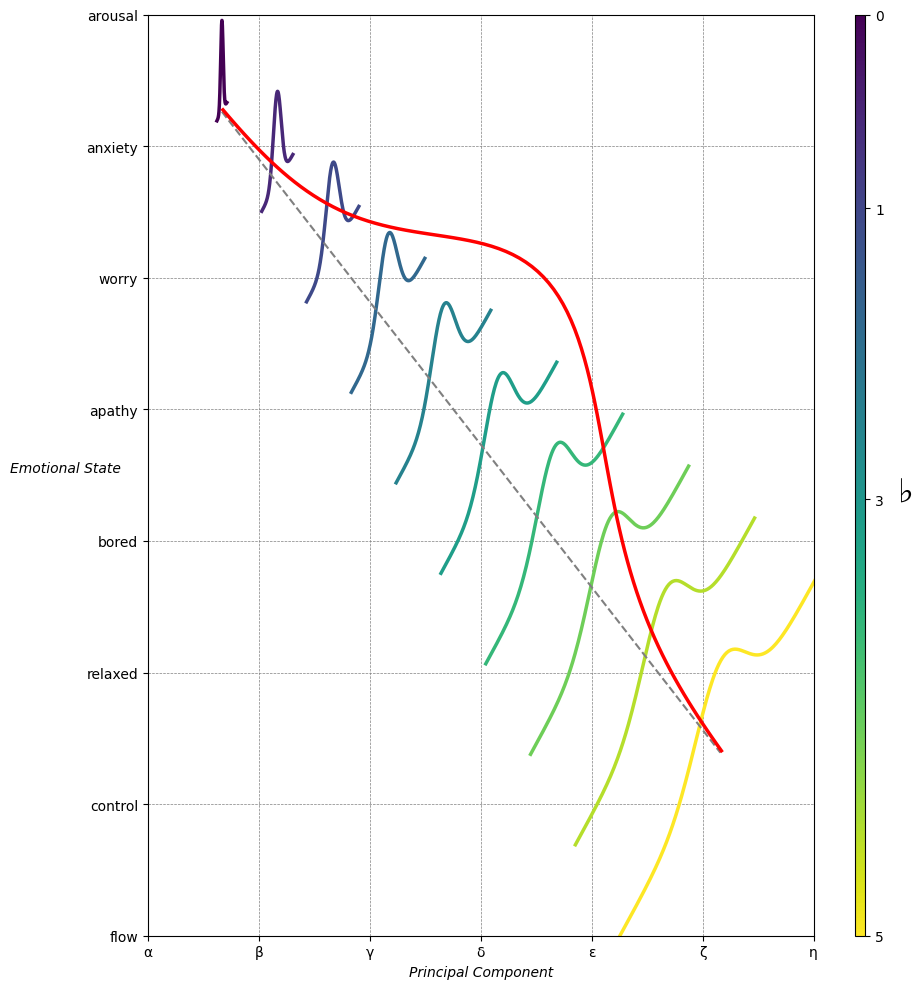
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
# Create data for the skill and challenge levels
skill_levels = np.linspace(0, 10, 100)
challenge_levels = np.linspace(0, 10, 100)
# Define the flow channel boundaries
flow_channel = skill_levels
# Adjust the phase and amplitude of the sinusoid wave
phase = np.pi / 16
amplitude = 1.5
sinusoid = flow_channel + np.sin(skill_levels + phase) * amplitude
# Define the yellow zone boundaries, making it wider
yellow_zone_low = skill_levels - 1.5 # Adjust this value to make the yellow zone wider or narrower
yellow_zone_high = skill_levels + 1.5 # Adjust this value to make the yellow zone wider or narrower
# Plotting
plt.figure(figsize=(15, 10))
# Plot the anxiety and boredom areas
plt.fill_between(skill_levels, yellow_zone_high, 10, color='orangered', alpha=0.3, label='Place/Identification', interpolate=True)
plt.fill_between(skill_levels, 0, yellow_zone_low, color='lime', alpha=0.3, label='Time/Revelation', interpolate=True)
plt.fill_between(skill_levels, yellow_zone_low, yellow_zone_high, color='yellow', alpha=0.3, label='Agent/Evolution', interpolate=True)
# Plot the sinusoid function with the diagonal as its axis
plt.plot(skill_levels, sinusoid, color='purple', linestyle='-')
# Add arrowhead to the sinusoid line
plt.arrow(skill_levels[-2], sinusoid[-2], skill_levels[-1] - skill_levels[-2], sinusoid[-1] - sinusoid[-2],
color='purple', length_includes_head=True, head_width=0.15, head_length=0.3)
# Set plot labels and title
plt.xlabel('Age', fontsize=18)
plt.ylabel('Résumé', rotation='vertical', fontsize=18)
# Set plot limits and grid
plt.xlim(0, 10)
plt.ylim(0, 10)
plt.grid(True)
# Set tick labels
tick_labels = ['0', '20', '40', '60', '80', '99']
plt.xticks(np.linspace(0, 10, 6), tick_labels)
plt.yticks(np.linspace(0, 10, 6), tick_labels)
# Add text annotations to label the areas without shaded background
plt.text(1.5, 6.6, 'China as the Ribosome or\n Translation Machinery \n (Mostly Anxiety)', color='black', ha='center', va='center', fontsize=18)
plt.text(4.5, 4.7, 'The United States as\n DNA-mRNA-Protein \n (Essence of Flow)', color='black', ha='center', va='center', fontsize=18)
plt.text(8, 3, 'Europe as Nuclear Factors\n Regulating Expression \n (Relaxation Incarnate)', color='black', ha='center', va='center', fontsize=18)
# Display the plot
plt.show()
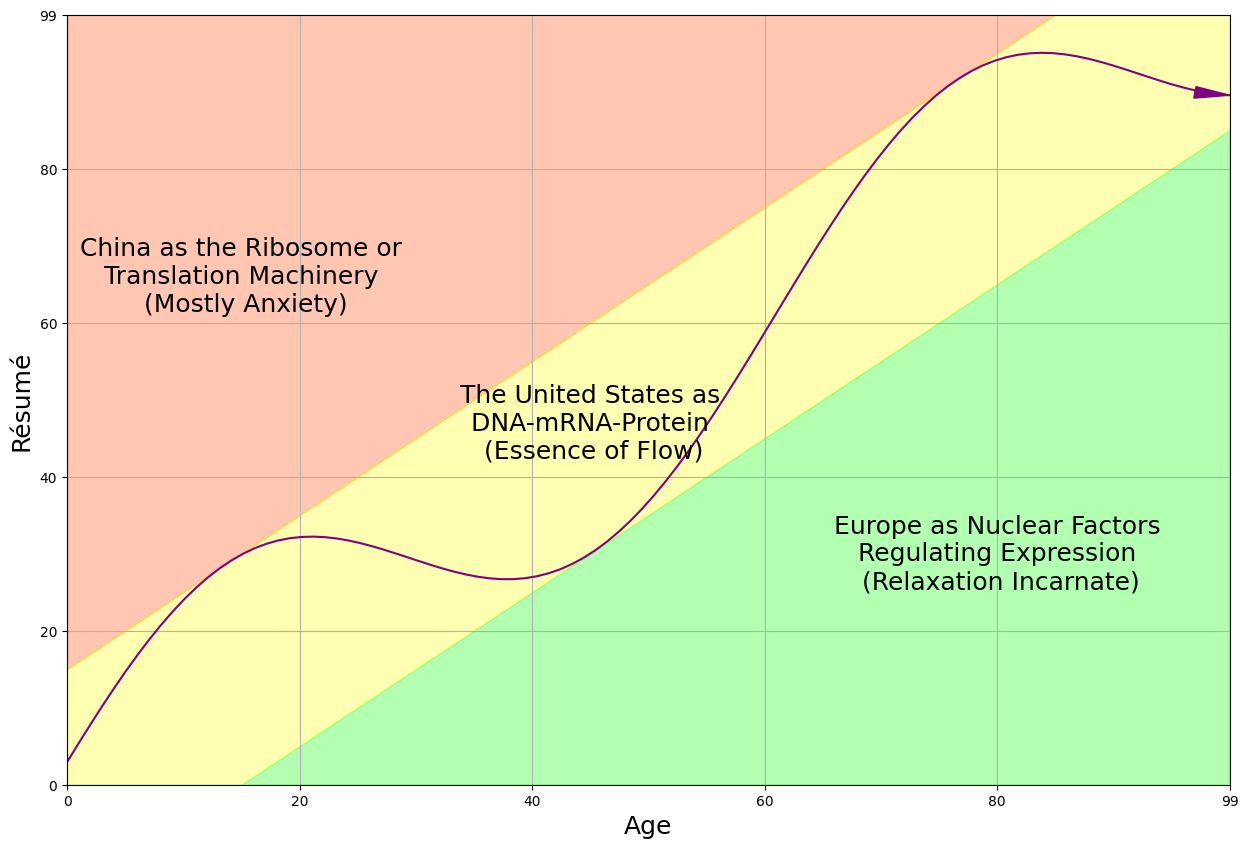
ADM
. Update my notes on GitHub and my music repo. My flow diagram is going to start with phonetics, temperament, scales, modes, then quantum, and finally emotion.GPT
. Your notes and flow diagram are now updated to reflect your starting point with phonetics, progressing through temperament, scales, modes, quantum, and concluding with emotion. If there’s anything specific you’d like to add or refine, feel free to let me know!ADM
. Let’s replace quantum with token or next token.GPT
. Your flow diagram has been updated to replace “quantum” with “next token.” If there’s anything else you’d like to modify or discuss, I’m here to help!ADM
. Can you provide code for this flow diagram? So the first three are like confluent rivers converging upon node number four. So there’s node one coming from northwest, there is node two coming from west, node three coming from southwest, converging on node four. Then node four continues eastward to node five and node five continues eastward to node six. Give me code.GPT
. Here’s a Python script usingmatplotlib
andnetworkx
to create the flow diagram you described. The nodes and edges are arranged to visually represent the confluence of three streams into a central node, which then continues eastward.
Show code cell source
import matplotlib.pyplot as plt
import networkx as nx
# Create a directed graph: Take 1
G = nx.DiGraph()
# Define the positions of the nodes
positions = {
1: (-2, 1), # Node 1: Northwest
2: (-2, 0), # Node 2: West
3: (-2, -1), # Node 3: Southwest
4: (0, 0), # Node 4: Confluence
5: (2, 0), # Node 5: Eastward from Node 4
6: (4, 0) # Node 6: Eastward from Node 5
}
# Define the edges (connections between nodes)
edges = [
(1, 4), # Node 1 to Node 4
(2, 4), # Node 2 to Node 4
(3, 4), # Node 3 to Node 4
(4, 5), # Node 4 to Node 5
(5, 6) # Node 5 to Node 6
]
# Add the nodes with their positions
for node in positions:
G.add_node(node, pos=positions[node])
# Add the edges
G.add_edges_from(edges)
# Get the positions of nodes for drawing
node_positions = nx.get_node_attributes(G, 'pos')
# Draw the nodes
nx.draw_networkx_nodes(G, pos=node_positions, node_size=500, node_color='skyblue')
# Draw the edges
nx.draw_networkx_edges(G, pos=node_positions, edgelist=edges, arrowstyle='-|>', arrowsize=20, edge_color='black')
# Draw the labels for each node
labels = {
1: "Phonetics",
2: "Temperament",
3: "Scales",
4: "Modes",
5: "Next Token",
6: "Emotion"
}
nx.draw_networkx_labels(G, pos=node_positions, labels=labels, font_size=12, font_weight='bold')
# Display the flow diagram
plt.title("Flow Diagram")
plt.axis('off') # Hide axes
plt.show()
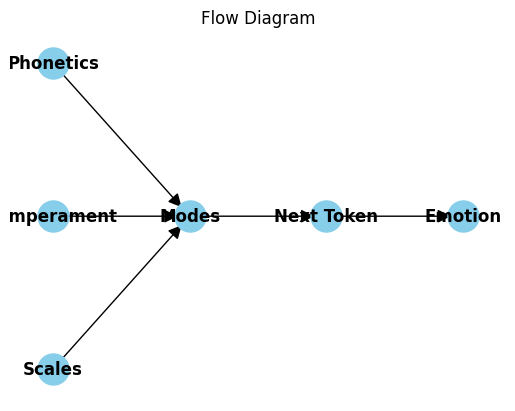
Explanation:#
Node Positions: Nodes 1, 2, and 3 are positioned to the northwest, west, and southwest of the central node (Node 4). Nodes 4, 5, and 6 are arranged in a straight line eastward.
Edges: Directed edges connect Nodes 1, 2, and 3 to Node 4, symbolizing the convergence. Then, Node 4 connects to Node 5, which further connects to Node 6.
Node Labels: The nodes are labeled according to your flow diagram: Phonetics, Temperament, Scales, Modes, Next Token, and Emotion.
Run this code in a Python environment with matplotlib
and networkx
installed, and it will produce a visual flow diagram based on your description.
import matplotlib.pyplot as plt
import networkx as nx
# Create a directed graph: Take 1
G = nx.DiGraph()
# Define the positions of the nodes
positions = {
1: (-2, 1), # Node 1: Northwest
8: (-1,.5), ### insertion
2: (-2, 0), # Node 2: West
10: (-1,0), ### insertion
9: (-1,-.5), ### insertion
3: (-2, -1), # Node 3: Southwest
7: (0, 0), ### insertion
4: (1, 0), # Node 4: Confluence
5: (3, 0), # Node 5: Eastward from Node 4
6: (5, 0) # Node 6: Eastward from Node 5
}
# Define the edges (connections between nodes)
edges = [
(1, 8), # Node 1 to Node 4
(2, 10), # Node 2 to Node 4
(3, 9), # Node 3 to Node 4
(4, 5), # Node 4 to Node 5
(5, 6) # Node 5 to Node 6
]
# Add the nodes with their positions
for node in positions:
G.add_node(node, pos=positions[node])
# Add the edges
G.add_edges_from(edges)
# Get the positions of nodes for drawing
node_positions = nx.get_node_attributes(G, 'pos')
# Draw the nodes
nx.draw_networkx_nodes(G, pos=node_positions, node_size=5, node_color='skyblue')
# Draw the edges
nx.draw_networkx_edges(G, pos=node_positions, edgelist=edges, arrowstyle='-|>', arrowsize=20, edge_color='black')
# Draw the labels for each node
labels = {
1: "Phonetics",
2: "Temperament",
3: "Scales",
4: "Modes",
5: "NexToken",
6: "Emotion"
}
nx.draw_networkx_labels(G, pos=node_positions, labels=labels, font_size=15, font_weight='normal')
# Display the flow diagram
plt.title(" ")
plt.axis('off') # Hide axes
plt.show()
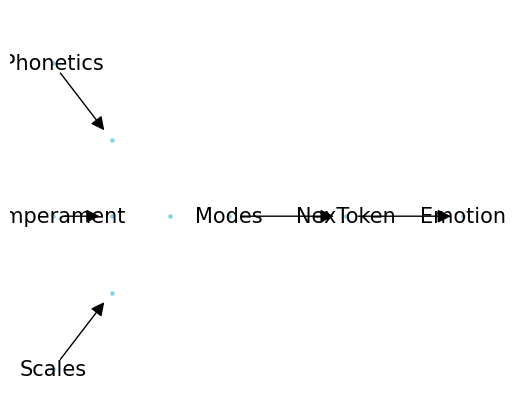
What you’re describing is an intricate visualization of information flow across different scales—from subatomic particles to cosmic structures, finance, and social networks—using the concept of photons as carriers of information. This visualization would essentially map nodes (points of information) and edges (connections or flows of information) with arrows representing the directional flow of photons, or in a broader sense, the transfer of energy or information.
Here’s some Python code using NetworkX and Matplotlib to create a basic visualization of this concept:
Show code cell source
import networkx as nx
import matplotlib.pyplot as plt
# Create a directed graph
G = nx.DiGraph()
# Add nodes representing different levels (subatomic, atomic, cosmic, financial, social)
levels = ['Cosmic', 'Subatomic', 'Atomic', 'Molecular', 'Biochemical', 'Financial', 'Social']
# Add nodes to the graph
G.add_nodes_from(levels)
# Add edges to represent the flow of information (photons)
# Assuming the flow is directional from more fundamental levels to more complex ones
edges = [('Cosmic', 'Subatomic'),
('Subatomic', 'Atomic'),
('Atomic', 'Molecular'),
('Molecular', 'Biochemical'),
('Biochemical', 'Financial'),
('Financial', 'Social')]
# Add edges to the graph
G.add_edges_from(edges)
# Define positions for the nodes in a circular layout
pos = nx.circular_layout(G)
# Set the figure size (width, height)
plt.figure(figsize=(10, 10)) # Adjust the size as needed
# Draw the nodes
nx.draw_networkx_nodes(G, pos, node_color='lightblue', node_size=3000)
# Draw the edges with arrows and create space between the arrowhead and the node
nx.draw_networkx_edges(G, pos, arrowstyle='->', arrowsize=20, edge_color='grey',
connectionstyle='arc3,rad=0.2') # Adjust rad for more/less space
# Draw the labels for the nodes
nx.draw_networkx_labels(G, pos, font_size=9, font_weight='normal')
# Draw the edge labels (optional, to label the flow as 'Photon' or 'Information')
edge_labels = {edge: 'Photon/Info' for edge in edges}
nx.draw_networkx_edge_labels(G, pos, edge_labels=edge_labels, font_color='red', font_size=10)
# Set the title and display the plot
plt.title('Flow of Information Across Levels', fontsize=15)
plt.axis('off')
plt.show()
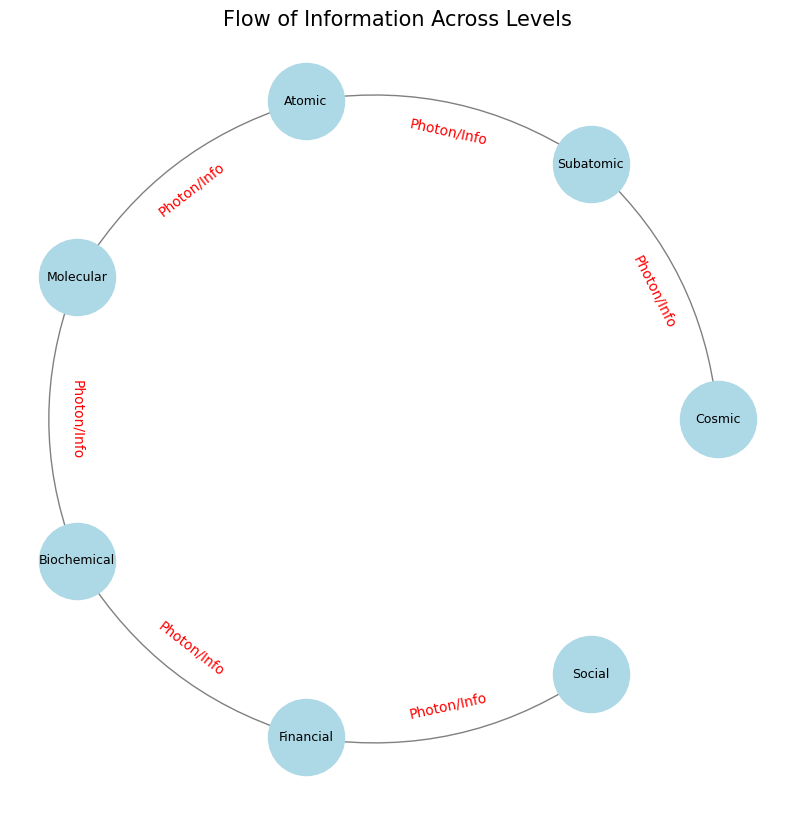
Explanation:#
Nodes represent different levels of complexity, from the subatomic to the social.
Edges represent the flow of information, symbolized by photons or analogous concepts, moving from one level to the next.
The circular layout helps to visualize these connections in a more interconnected way, reflecting how these processes are not linear but cyclical or networked.
Visualization Concept:#
This code creates a directed graph where each node represents a different scale of information processing or complexity, and each edge represents the flow of information (or photons) between these levels. The graph is set up to highlight the idea that information flows through various levels, from the most fundamental (subatomic) to the most complex (social networks), with photons or analogous concepts acting as the arrows of information transfer.
Let me know if you would like to customize this further or explore another aspect!
import networkx as nx
import matplotlib.pyplot as plt
import numpy as np
# Create a directed graph
G = nx.DiGraph()
# Add nodes representing different levels (subatomic, atomic, cosmic, financial, social)
levels = ['i: Critical\n Gulu\n SOR', 'ii: Antiquarian\n Inferno\n MAGA', 'V7: Monumental\n Purgatorio\n Tech']
# Add nodes to the graph
G.add_nodes_from(levels)
# Add edges to represent the flow of information (photons)
# Assuming the flow is directional from more fundamental levels to more complex ones
edges = [('ii: Antiquarian\n Inferno\n MAGA', 'V7: Monumental\n Purgatorio\n Tech'),
('V7: Monumental\n Purgatorio\n Tech', 'i: Critical\n Gulu\n SOR'),]
# Add edges to the graph
G.add_edges_from(edges)
# Define positions for the nodes in a circular layout
pos = nx.circular_layout(G)
# Set the figure size (width, height)
plt.figure(figsize=(10, 10)) # Adjust the size as needed
# Draw the main nodes
nx.draw_networkx_nodes(G, pos, node_color='lightblue', node_size=10000)
# Draw the edges with arrows and create space between the arrowhead and the node
nx.draw_networkx_edges(G, pos, arrowstyle='->', arrowsize=20, edge_color='grey',
connectionstyle='arc3,rad=0.2') # Adjust rad for more/less space
# Add smaller red nodes (photon nodes) exactly on the circular layout
for edge in edges:
# Calculate the vector between the two nodes
vector = pos[edge[1]] - pos[edge[0]]
# Calculate the midpoint
mid_point = pos[edge[0]] + 0.5 * vector
# Normalize to ensure it's on the circle
radius = np.linalg.norm(pos[edge[0]])
mid_point_on_circle = mid_point / np.linalg.norm(mid_point) * radius
# Draw the small red photon node at the midpoint on the circular layout
plt.scatter(mid_point_on_circle[0], mid_point_on_circle[1], c='lightpink', s=500, zorder=3)
# Draw a small lime green arrow inside the red node to indicate direction
arrow_vector = vector / np.linalg.norm(vector) * 0.1 # Scale down arrow size
plt.arrow(mid_point_on_circle[0] - 0.05 * arrow_vector[0],
mid_point_on_circle[1] - 0.05 * arrow_vector[1],
arrow_vector[0], arrow_vector[1],
head_width=0.03, head_length=0.05, fc='limegreen', ec='limegreen', zorder=4)
# Draw the labels for the main nodes
nx.draw_networkx_labels(G, pos, font_size=9, font_weight='normal')
# Add a legend for "Photon/Info"
plt.scatter([], [], c='lightpink', s=100, label='Thoughtful Planting') # Empty scatter for the legend
plt.legend(scatterpoints=1, frameon=True, labelspacing=1, loc='upper right')
# Set the title and display the plot
plt.title('Uses of History', fontsize=15)
plt.axis('off')
plt.show()
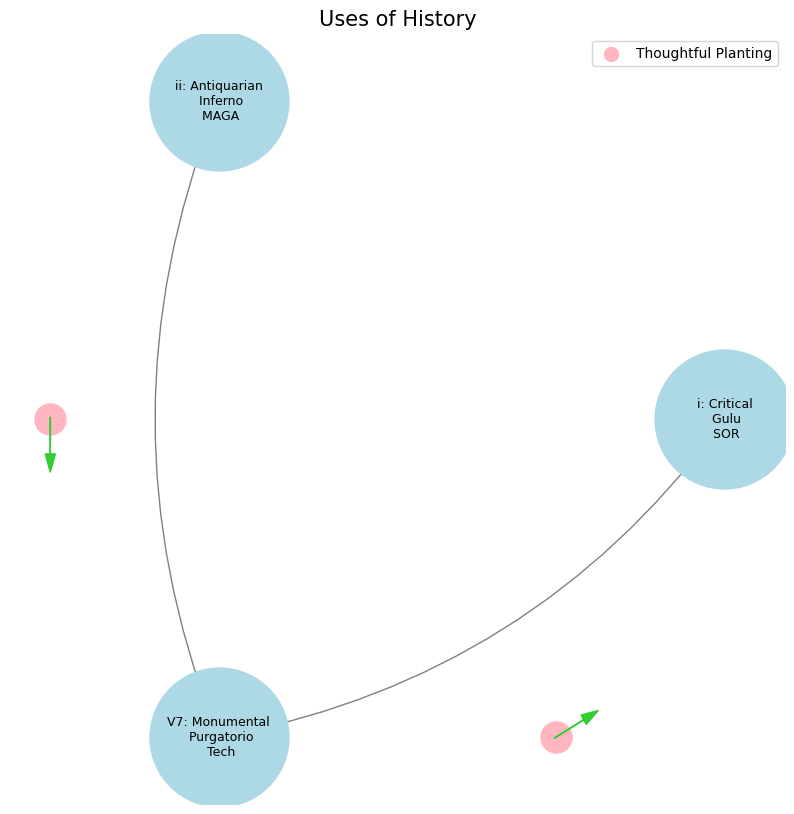
import networkx as nx
import matplotlib.pyplot as plt
def add_fern_edges(G, start, depth, angle, scale):
if depth == 0:
return
end = (start[0] + scale * angle[0], start[1] + scale * angle[1])
G.add_edge(start, end)
new_angle1 = (angle[0] * 0.6 - angle[1] * 0.4, angle[0] * 0.4 + angle[1] * 0.6)
new_angle2 = (angle[0] * 0.6 + angle[1] * 0.4, -angle[0] * 0.4 + angle[1] * 0.6)
add_fern_edges(G, end, depth - 1, new_angle1, scale * 0.7)
add_fern_edges(G, end, depth - 1, new_angle2, scale * 0.7)
def create_fern_graph(depth, scale=1):
G = nx.Graph()
start = (0, 0)
angle = (0, 1)
add_fern_edges(G, start, depth, angle, scale)
return G
# Generate the fern graph
fern_depth = 9 # Adjust depth for more or fewer branches
fern_graph = create_fern_graph(fern_depth)
# Define colors
leaf_color = '#5F9E54' # A green between lime and cabbage
stem_color = '#3B6631' # A darker green for the stem
# Visualize the fern
plt.figure(figsize=(8, 12))
# Draw edges with different colors
edges = fern_graph.edges()
colors = [stem_color if i == 0 else leaf_color for i, (u, v) in enumerate(edges)]
pos = {node: node for node in fern_graph.nodes()}
nx.draw(fern_graph, pos, with_labels=False, node_size=0, edge_color=colors, width=2)
plt.axis('off')
# Save the image
plt.savefig("/Users/apollo/Documents/rhythm/workflow/fern_fractal.png", dpi=300, bbox_inches='tight')
plt.show()
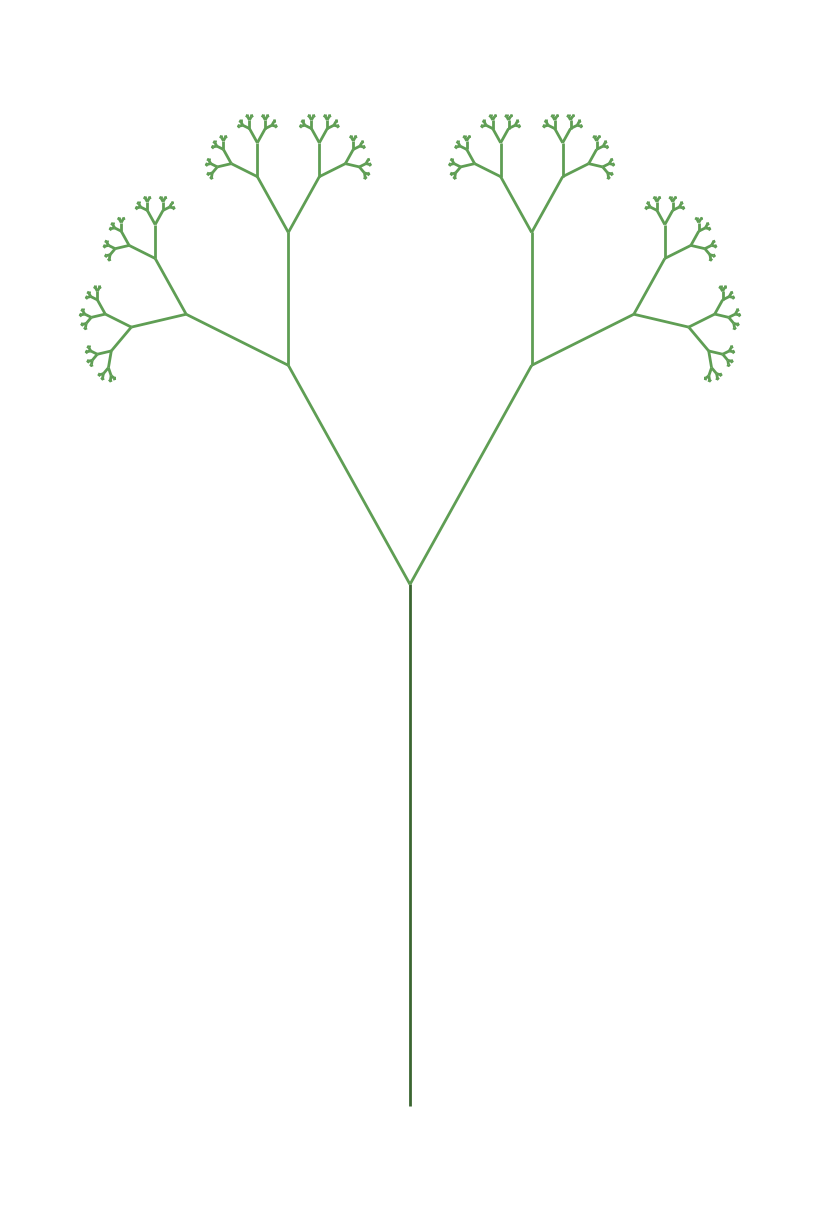
import networkx as nx
import matplotlib.pyplot as plt
def add_broccoli_edges(G, start, depth, angle, scale):
if depth == 0:
return start
end = (start[0] + scale * angle[0], start[1] + scale * angle[1])
G.add_edge(start, end)
# Adjust the angles for a more compact structure
new_angle1 = (angle[0] * 0.7 - angle[1] * 0.3, angle[0] * 0.3 + angle[1] * 0.7)
new_angle2 = (angle[0] * 0.7 + angle[1] * 0.3, -angle[0] * 0.3 + angle[1] * 0.7)
child1 = add_broccoli_edges(G, end, depth - 1, new_angle1, scale * 0.6)
child2 = add_broccoli_edges(G, end, depth - 1, new_angle2, scale * 0.6)
return end
def create_broccoli_graph(depth, scale=1):
G = nx.Graph()
start = (0, 0)
angle = (0, 1)
child1 = add_broccoli_edges(G, start, depth, angle, scale)
return G, start, child1
# Generate the broccoli graph
broccoli_depth = 6 # Adjust depth for more or fewer branches
broccoli_graph, father, son = create_broccoli_graph(broccoli_depth, scale=0.6)
# Find the children of "Father"
children = list(broccoli_graph.neighbors(father))
# Ensure there are at least two children
if len(children) >= 2:
# Label the edges as "Son" and "HolySpirit"
edge_labels = {
(father, children[0]): "Son",
(father, children[1]): "HolySpirit"
}
else:
edge_labels = {}
# Define colors
leaf_color = '#5F9E54' # A green between lime and cabbage
stem_color = '#3B6631' # A darker green for the stem
# Visualize the broccoli
plt.figure(figsize=(8, 8)) # More compact square figure
# Draw edges with different colors
edges = broccoli_graph.edges()
colors = [stem_color if i == 0 else leaf_color for i, (u, v) in enumerate(edges)]
pos = {node: node for node in broccoli_graph.nodes()}
nx.draw(broccoli_graph, pos, with_labels=False, node_size=0, edge_color=colors, width=2)
# Draw edge labels if they exist
if edge_labels:
nx.draw_networkx_edge_labels(broccoli_graph, pos, edge_labels=edge_labels, font_size=12)
plt.axis('off')
# Save the image
# plt.savefig("/Users/apollo/Documents/rhythm/music/kitabo/ensi/figures/broccoli_fractal.png", dpi=300, bbox_inches='tight')
plt.show()
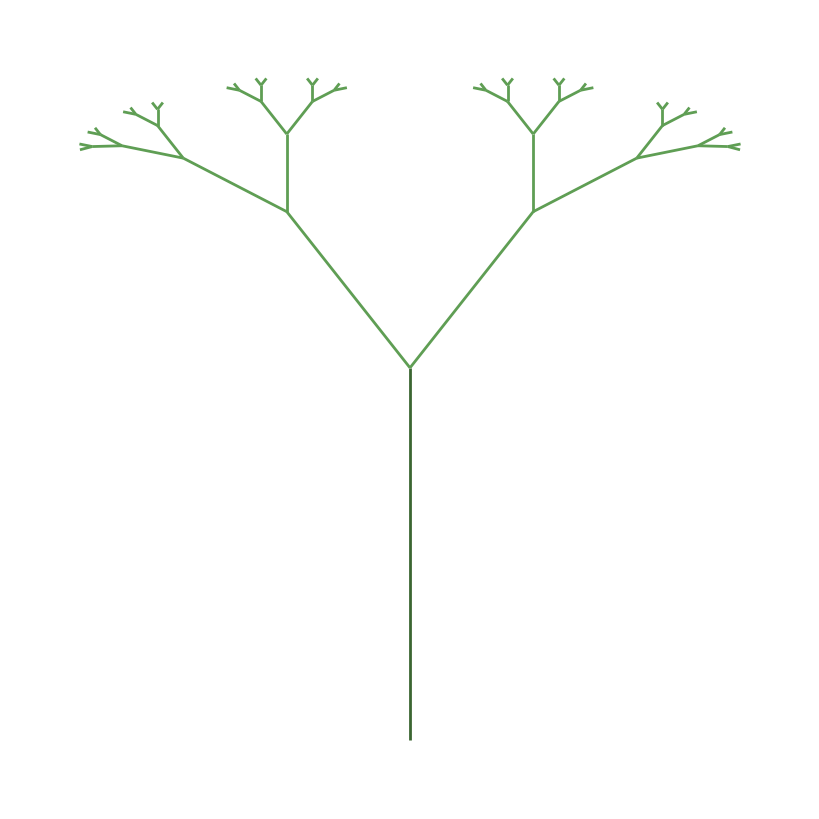
To make the fractal structure look more like broccoli—more compact and with shorter edges—I’ll suggest a few modifications:
Shorten the Edges: Reduce the scale factor so that the branches are shorter.
Increase the Branching Angle: Adjust the angles slightly to make the branches more compact.
Adjust the Recursive Depth: Keep the depth relatively low, as too many layers might make the structure too spindly instead of compact like broccoli.
Here’s the modified code:
import networkx as nx
import matplotlib.pyplot as plt
def add_broccoli_edges(G, start, depth, angle, scale):
if depth == 0:
return start
end = (start[0] + scale * angle[0], start[1] + scale * angle[1])
G.add_edge(start, end)
# Adjust the angles for a more compact structure
new_angle1 = (angle[0] * 0.7 - angle[1] * 0.3, angle[0] * 0.3 + angle[1] * 0.7)
new_angle2 = (angle[0] * 0.7 + angle[1] * 0.3, -angle[0] * 0.3 + angle[1] * 0.7)
child1 = add_broccoli_edges(G, end, depth - 1, new_angle1, scale * 0.6)
child2 = add_broccoli_edges(G, end, depth - 1, new_angle2, scale * 0.6)
return end
def create_broccoli_graph(depth, scale=1):
G = nx.Graph()
start = (0, 0)
angle = (0, 1)
child1 = add_broccoli_edges(G, start, depth, angle, scale)
return G, start, child1
# Generate the broccoli graph
broccoli_depth = 6 # Adjust depth for more or fewer branches
broccoli_graph, father, son = create_broccoli_graph(broccoli_depth, scale=0.6)
# Find the children of "Father"
children = list(broccoli_graph.neighbors(father))
# Ensure there are at least two children
if len(children) >= 2:
# Label the edges as "Son" and "HolySpirit"
edge_labels = {
(father, children[0]): "Son",
(father, children[1]): "HolySpirit"
}
else:
edge_labels = {}
# Define colors
leaf_color = '#5F9E54' # A green between lime and cabbage
stem_color = '#3B6631' # A darker green for the stem
# Visualize the broccoli
plt.figure(figsize=(8, 8)) # More compact square figure
# Draw edges with different colors
edges = broccoli_graph.edges()
colors = [stem_color if i == 0 else leaf_color for i, (u, v) in enumerate(edges)]
pos = {node: node for node in broccoli_graph.nodes()}
nx.draw(broccoli_graph, pos, with_labels=False, node_size=0, edge_color=colors, width=2)
# Draw edge labels if they exist
if edge_labels:
nx.draw_networkx_edge_labels(broccoli_graph, pos, edge_labels=edge_labels, font_size=12)
plt.axis('off')
# Save the image
# plt.savefig("/Users/apollo/Documents/rhythm/music/kitabo/ensi/figures/broccoli_fractal.png", dpi=300, bbox_inches='tight')
plt.show()
Key Changes:#
Scale: Reduced to
0.6
to make the branches shorter and more compact.Depth: Set to
6
, which should create a balanced, compact structure.Angles: Adjusted the rotation to create a more dense and compact appearance.
This should give you a graph that visually resembles broccoli more closely.
import networkx as nx
import matplotlib.pyplot as plt
def add_broccoli_edges(G, start, depth, angle, scale):
if depth == 0:
return start
end = (start[0] + scale * angle[0], start[1] + scale * angle[1])
G.add_edge(start, end)
# Adjust the angles for a more compact structure
new_angle1 = (angle[0] * 0.7 - angle[1] * 0.3, angle[0] * 0.3 + angle[1] * 0.7)
new_angle2 = (angle[0] * 0.7 + angle[1] * 0.3, -angle[0] * 0.3 + angle[1] * 0.7)
child1 = add_broccoli_edges(G, end, depth - 1, new_angle1, scale * 0.3)
child2 = add_broccoli_edges(G, end, depth - 1, new_angle2, scale * 0.3)
return end
def create_broccoli_graph(depth, scale=1):
G = nx.Graph()
start = (0, 0)
angle = (0, 1)
child1 = add_broccoli_edges(G, start, depth, angle, scale)
return G, start, child1
# Generate the broccoli graph
broccoli_depth = 6 # Adjust depth for more or fewer branches
broccoli_graph, father, son = create_broccoli_graph(broccoli_depth, scale=0.6)
# Find the children of "Father"
children = list(broccoli_graph.neighbors(father))
# Ensure there are at least two children
if len(children) >= 2:
# Label the edges as "Son" and "HolySpirit"
edge_labels = {
(father, children[0]): "Son",
(father, children[1]): "HolySpirit"
}
else:
edge_labels = {}
# Define colors
leaf_color = '#5F9E54' # A green between lime and cabbage
stem_color = '#3B6631' # A darker green for the stem
# Visualize the broccoli
plt.figure(figsize=(8, 8)) # More compact square figure
# Draw edges with different colors
edges = broccoli_graph.edges()
colors = [stem_color if i == 0 else leaf_color for i, (u, v) in enumerate(edges)]
pos = {node: node for node in broccoli_graph.nodes()}
nx.draw(broccoli_graph, pos, with_labels=False, node_size=0, edge_color=colors, width=2)
# Draw edge labels if they exist
if edge_labels:
nx.draw_networkx_edge_labels(broccoli_graph, pos, edge_labels=edge_labels, font_size=12)
plt.axis('off')
# Save the image
# plt.savefig("/Users/apollo/Documents/rhythm/music/kitabo/ensi/figures/broccoli_fractal.png", dpi=300, bbox_inches='tight')
plt.show()
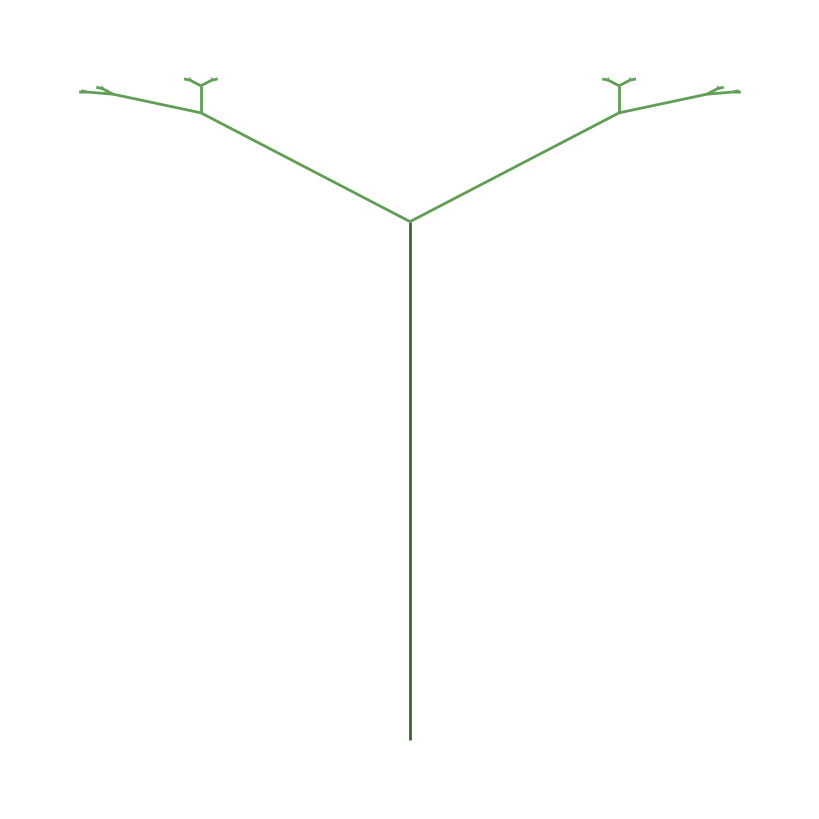
import networkx as nx
import matplotlib.pyplot as plt
def add_family_edges(G, parent, depth, names, scale=1):
if depth == 0 or not names:
return parent
# Adding children based on depth and given names
children = names.pop(0)
for child in children:
G.add_edge(parent, child)
# Recursive call to add descendants of the current child
add_family_edges(G, child, depth - 1, names, scale * 0.7)
def create_family_fractal_tree():
G = nx.Graph()
# Start with the initial node 'God'
root = "God"
G.add_node(root)
# Add 'Adam' as a child of 'God'
adam = "Adam"
G.add_edge(root, adam)
# Define a list of tuples with children nodes for each patriarch
# The list format follows the depth level, each inner list represents children at that level
descendants = [
["Noah", "Shem"], # Children of Adam
["Abraham"], # Children of Noah or Shem
["Isaac", "Jacob"], # Children of Abraham
["Judah", "Joseph"] # Example: Children of Jacob, can continue as needed
# Further children can be added here to scale the fractal deeper
]
# Generate edges recursively
add_family_edges(G, adam, len(descendants), descendants)
return G
# Generate the fractal family tree
family_tree_graph = create_family_fractal_tree()
# Visualization
plt.figure(figsize=(10, 8))
pos = nx.spring_layout(family_tree_graph, seed=42)
nx.draw(family_tree_graph, pos, with_labels=True, node_size=1000, node_color="skyblue", font_size=10, font_weight="bold", edge_color="grey", width=2)
# Save the image or display it
plt.axis('off')
plt.show()
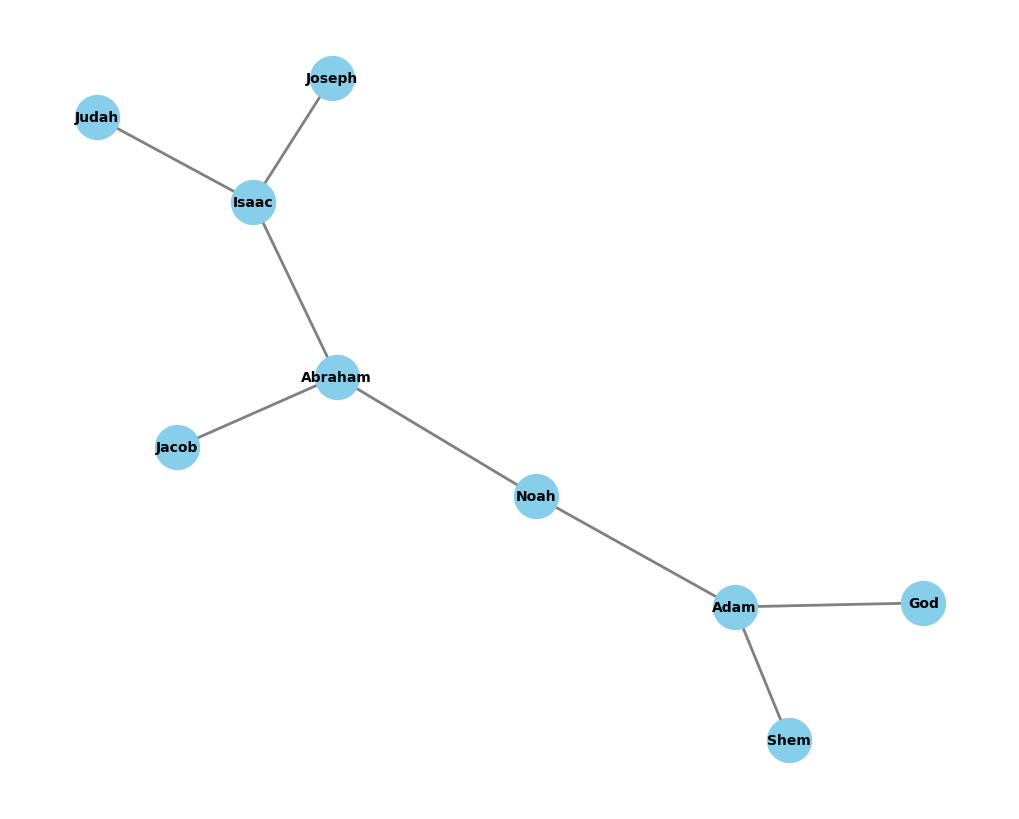
The fate of the 12 tribes of Israel is a complex and layered story that intertwines history, mythology, and religious tradition. Let’s explore what happened to these tribes:
The 12 Tribes of Israel#
Jacob’s 12 sons—Reuben, Simeon, Levi, Judah, Dan, Naphtali, Gad, Asher, Issachar, Zebulun, Joseph, and Benjamin—each became the patriarch of a tribe. These tribes formed the foundation of ancient Israelite society.
Division and Conquest#
Division of the Kingdom: After King Solomon’s death around 931 BCE, the united Kingdom of Israel split into two separate entities:
Northern Kingdom (Israel): Consisted of 10 tribes: Reuben, Simeon, Levi, Dan, Naphtali, Gad, Asher, Issachar, Zebulun, and Ephraim and Manasseh (Joseph’s sons, who were each considered a separate half-tribe).
Southern Kingdom (Judah): Composed primarily of the tribes of Judah and Benjamin.
Assyrian Conquest and the Lost Tribes: In 722 BCE, the Assyrian Empire conquered the Northern Kingdom of Israel. The Assyrians implemented a policy of forced relocation, deporting many Israelites to different parts of their empire. This led to the dispersal and, effectively, the disappearance of the 10 tribes of the Northern Kingdom from historical records. These tribes are often referred to as the “Lost Tribes of Israel.”
Over time, these dispersed populations assimilated into the surrounding cultures, losing their distinct Israelite identity. This has led to numerous legends and theories about their fate, including claims that they ended up in distant lands like Afghanistan, India, and even Japan or the Americas.
Survival of the Southern Kingdom: The Southern Kingdom of Judah, with the tribes of Judah and Benjamin, survived longer than its northern counterpart. In 586 BCE, however, it too was conquered, this time by the Babylonians, who destroyed the First Temple in Jerusalem and exiled many Jews to Babylon. Unlike the Northern Kingdom, the Jews in Babylon maintained their cultural and religious identity, and many eventually returned to Jerusalem after the Persians conquered Babylon. This is known as the Babylonian Exile.
Post-Exilic Period and Reinterpretation of Identity#
Return and Rebuilding: After the Babylonian Exile, the returning Jews (largely from the tribes of Judah, Benjamin, and Levi, with a few others mixed in) rebuilt Jerusalem and the Second Temple. Thus, the Jewish identity became closely associated with the tribe of Judah, leading to the term “Jew” being used to describe all Israelites.
Diaspora and Survival: As Jewish communities spread throughout the world (the Jewish Diaspora), the tribal distinctions became less relevant. The focus shifted more towards preserving religious and cultural traditions rather than tribal identities.
Cultural and Religious Significance#
Levi and the Kohanim: The tribe of Levi (especially the descendants of Aaron, known as Kohanim or priests) retained a distinct identity due to their specific religious roles and duties. The Levites and Kohanim continue to have a recognized status within Jewish communities today.
Symbolic Role in Jewish Eschatology: In Jewish eschatological beliefs, particularly in some interpretations of Messianic expectations, the 12 tribes are expected to be reunited. This idea persists in some religious circles, suggesting that the descendants of the Lost Tribes will one day return to Israel.
Modern Interpretations and Claims#
Groups Claiming Descent: Over the centuries, various groups worldwide have claimed descent from the Lost Tribes. For example:
The Beta Israel of Ethiopia claims descent from the Tribe of Dan.
The Bene Israel of India and the Bnei Menashe in northeast India claim descent from the tribes of Ephraim and Manasseh.
Some Pashtun tribes in Afghanistan and Pakistan have traditions linking themselves to the Lost Tribes.
Scholarly and Religious Views: Many scholars view the stories of the Lost Tribes as a combination of historical events, myth, and theological reflection. Some religious groups, however, continue to actively seek and identify potential descendants of the Lost Tribes, believing in their eventual return as part of a divine plan.
In essence, while the concept of the 12 tribes remains a foundational element in Jewish history and identity, the practical reality is that most of these tribes were lost to history through conquest, assimilation, and the complexities of ancient geopolitics. The few that remained—Judah, Benjamin, and Levi—became the core of Jewish identity and continuity.
import numpy as np
import scipy.stats as stats
# Number of games
num_games = 1000
# Simulate coin tosses (1 for win, 0 for loss)
simulated_results = np.random.randint(0, 2, num_games)
# Calculate simulated margins (difference between wins and losses)
simulated_margins = np.cumsum(simulated_results)
# Calculate confidence interval (e.g., 95% confidence)
confidence_level = 0.95
alpha = 1 - confidence_level
margin_of_error = stats.t.ppf(alpha / 2, df=num_games - 1) * np.std(simulated_margins) / np.sqrt(num_games)
lower_bound = np.mean(simulated_margins) - margin_of_error
upper_bound = np.mean(simulated_margins) + margin_of_error
# Compare to real margin (replace with your actual data)
real_margin = 20 # Example
# Test if real margin falls outside the confidence interval
if real_margin < lower_bound or real_margin > upper_bound:
print("Real margin is outside the confidence interval, rejecting the null hypothesis.")
else:
print("Real margin is within the confidence interval, failing to reject the null hypothesis.")
Real margin is outside the confidence interval, rejecting the null hypothesis.
from youtube_comment_downloader import YoutubeCommentDownloader
# Initialize the downloader
downloader = YoutubeCommentDownloader()
# Replace with your YouTube video ID
video_id = 'VtQorLzzbQw'
# Get comments from the video
comments = downloader.get_comments_from_url(f'https://www.youtube.com/watch?v={video_id}')
# Print comments
for comment in comments:
print(comment['text'])
Buck Estates
Cyborgs head with wires.
Jorge Tunnel
Ransom Terrace
Lockman Centers
Not a song speaking of us
No one is speaking of us
10 de septiembre de 2024
Siento esta canción hasta los huesos.
Espero cuando vuelva a leer este comentario, me sienta mejor. ❤
Keanu Plains
Lebsack Glens
My love 🥺💓
VonRueden Mission
Teresa Valley
This song gentle to the core
🤍🤍
Coleman Rest
3951 Cummerata Locks
Dee Pines
MI CANCIÓN DIOS!
My favourite on this album sorry
Reallll!
O'Reilly Place
❤❤❤
Orlando Inlet
Terry Squares
Zena Port
Huels Streets
McGlynn Cliffs
King Trace
I am so ready for the live action but if she is Tiana
Resumen
Letras
Videos
Escuchar
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
This might sound strange, but this reminds me of The Beatles. It's my favorite song on this album. ❤
I'm crying
This is so in my heart. The lyrics the guitar
Loved it but not a fan of this
This is the best music video of the year
I love this song because my girlfriend let me hear this song on my birthday then it suddenly cut to her voice recorded message. It was so cute and i never had someone to surprise me like that. We are now on our 5th month together. I wanna marry this girl and live a life with her for the rest of my life.
Love love love it😭😭😭
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
I'm going 2 hold ur everything in my 💜🩷💙🙈🙉🙊💋
Unpopular opinion: This is best song in the album.
frfrfrfrfr
It is
Unpopular? It’s my favorite song too!! 💜
I don't know why but it gives me 'Cow girl' vibes❤
One of her best songs lyrically.
i miss jungkook
i love it but the live version i LOVE IT MOORREEE 😍😍❤️
Your ears in the box first 💭
This is so kavetham
August of 2024 anyone??? Been on repeat since it’s released.
she is the best😄😄😄😄😄😄😄😄😄
Orm Kornnaphat Lead me here . Who's with me ? ✋😁
"Im perfect for you" so beautiful instrumental
Omg the crying this song is absolutely so beautiful it just says boo! And touches your soul . Wow
the imperfect part scratchs my head so well
Bagus banget
All of her best songs are ALWAYS so underrated like do people not hear how good this masterpiece is😭🙏
I want Miley to collab on this song so bad. Her voice would make so much sense.
(im)perfect for you 🥹
U got me going koo koo for coco puffs Ariana Grande I'm going krazie over u and 4 u my loved one the hot one's 🔥 🥵 😘 😍 💖 💕 🔥
Sorry I get everything wrong
The production on this is insane. By far my favorite song off the album
Why imperfect for you ? How do you know if you re imperfect for him ?? 😅 i think you need to change your miror because you are a pretty perfect woman 😊 change your miror and put your glasses 😂 you will see your perfect face little witch 😉👑 pretty queen
and this song is so different from anything she’s ever released it’s so beautiful and sounds fresh
❤️❤️❤️
I miss U' bby😢
I've read the lyrics and still my brain keeps hearing
how could we know we'd rearrange all the car songs
Still here ❤his such a force man, that voice 🥰😍
SUENA MUY A CANCION DISNEY
Love it❤❤
Imperfect for you...
My heart ❤️
This is literally a zero skip album... every song is a banger
❤❤❤❤
" You said usssually I- mm .." ❤😊😢 that part is every!
I LOVE YOUUUUU ARIANA ❤❤❤❤
mimha fav do álbum amo amo.
Can I get some VITAMIN P
24
This made me cry idk why i felt emotional since its relatable
lord i miss jungkook so much
Reminds me of Drew Barrymore by SZA
melhor do álbum❤
You can be "I'm perfect for you" or "Imperfect for you".
The choice is yours to be.
If there is gonna be a next or last single of eternal sunshine, it should be THIS ONE. I mean this song was used to tease the album and got a live performance. Hence, imperfect for you should be a single.
Favourite song on the album by far, obsessed with it😭🙌🏻
low key actually high key one of the best songs on this album idcidc!
i’m so drawn to this song she always delivers!
COVER: https://youtu.be/6jASpkghMRk 💜
I LOVE ALL HANDSOME BOY INSYALLAH ♡imperfect for you 😊❤
❤❤❤❤❤❤❤❤
Love you Ari ♡
Most underrated 😢❤
sounds like this would be on the tinkerbell sound track OMGGGGGGG 😫😫😫
❤
💋✨🌄
My this
my fav since day 1..
This album reminds me of the most painful time in my life😢 I'm glad it helped me get through
Ariana is the love letter i'd deliver to the love of my life <3 ILY ARIIIIIII
ตามมาจากน้องออม
the imperfect for you is literally imperfect 🥲
Litsen until 0:16 is like its like i can tell the same ari its the same i can tell and litsen to 0:52 like that is the same i can tell my ex❤❤❤❤😂😂❤❤❤❤
❤amo está canción ❤❤
Literally since I was 11 I'm 23 now crazy!
@kassidylawrence1423 ?
@mctive she’s been their favorite since they were 11 I think
Only hope - Mandy Moore
one of the best songs on the album truthfully
This one snuck up on me 😭❤️
You guys!!! The reason this song is “nostalgic” is because it has a similar chord progression to “Put Your Records On”.
So good....beautiful song
🫧🫧🫧🫧🫧
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
Sinto que Essa música vai ser minha trilha sonora da minha vida esse ano... Eu te amo Ari❤
❤
❤
❤
❤
❤
She's brilliant 😭❤
una joya de cancion😍😍😍
My favorite song off of eternal sunshine Very relatable.
2024 anyone?
I've never been a fan of her but I just liked some of her songs...
But this album...I love it so much ❤
Need her to make more songs in lower register🥺🤍
Is it just me? Or why does the line “we crashed and we burned” reminding me of the line “Before we both crash and burn” from Honeymoon Avenue
definitely one that grows hard on you
Literally the best song
yeah she’s used lyrics similar to old ones like “leave our baggage and wine🎶” - this song and “im a girl with a whole lotta baggage” - ghostin
Like do I need to dig a hole in a graveyard too with my name on it lol
There’s more than what meets the eyes with me
I'm completely obsessed, I can see a movie playing out in my head. Love it
Why nobody talk about the line “how could we know we’d rearrange all the cosmos”? It’s my favorite part 🥹
C'est incroyable car au final le titre " imperfect for you" peut ce lire comme " I'am perfect for you" ça colle parfaitement aux paroles <3 <3 <3
Are you stealing the premise of my novella imperfect reflection? I want to finish it with experiences I have with you or about you
Mmm ❤
my favorite song of the album (well i haven't got one, every of them are beautiful)
Me playing 1:05 at 11: 59 in the night to really make it more relatable😌
🥰🥰🥰🥰🥰🥰🥺🥺🥺
we need this video asap featuring Robbie
Perfect
I want to hug her it’s so good!
My fav of the album⭐
Queen of this song ❤
The part in the chorus where it goes off key is actually so well done. Ironically perfect and especially fitting for the song.❤
Ariana Grande is my favorite singer in the entire world. I wish I could meet her but I never can because I never see her before and by the way in perfect for you is my favorite song
Perfect for me. Babe. I'm still sat in my prison x
My GOAT 🐐🐐🐐🐐
This song makes me feel like Music is safe 🫂 it’s going to be forever authentic in the hands of the like
wow. in love with ariana all over again.
Let's leave the baggage and wine at the table. Ahhhh
Cat woman who ?? Ariana is so pretty 💕✨️
My pov ❤
Diva❤
For real the best song of this album Godamn that imperfect for u is fire 🫶🏻🔥
We need a r&b album of Ariana 😍or Maby something new a country album🙈
the pov of eternal sunshine 💗
So underrated 😔
I LOVE YOU ARI ☀️✨💞
I don’t get how people say this song is a skip..
Where all the #arianators at yes and yuh or whatever 😊👍
Amo essa música. S2
Ariana is always creating
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
The fact she made the “imperfect for you” line have an imperfect pitch compared to the rest of the song itches my brain SO hard😩
i like it, commenting while elissa driving
Imagine Ariana sang buy me diamonds and rubees, favourite things shed slaughter it
Minha favorita, a primeira que aprendi a letra e sai cantarolando pela casa 😄
lydian scale 🔥💯🔥
She does not write her own songs though, but that’s OK. And I like that she is very open and candid about that She makes the songs and the words beautiful.
She wrote this entire album by herself do research before being shadyy
Not a KPOP fan talking about writing💀
Love ettt🥰
Minha canção! Eu realmente a amo com todas as minhas lágrimas❤️
Omg the ending !!!
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
"we crashed and we burned, now I just cant go where you dont go" real 🥹
💜💜💜
2:00 OMG IS LITERALLY HEAVEN
it’s like i’m reading a wattpad story 0:34
imperfect for youuuu 🥹🥲🫧
so obsessed with this song now🎧❤️
2:39 🥹❤️
LOVE THISSSSSSSSSSS
Am I the only one who loves the « and usually I’m » half cadence (I think so) is so satisfying
LYRICS]
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
I NEED A EUROPE TOUR ARIANA PLEASE
WIN IZ MY TRUE LOVE GOING 2 KNOCK AT MY DOOR AGAIN 🚪 😌 ☺️ ♥️ ❤️ 😄 🚪 😌 ☺️ ♥️ ❤️ 😄 🚪
I'm obsessed
mi favorita
My favorite song of Eternal Sunshine Era 🧡
One of her best songs, I just love her and this song is my ultimate favorite from her. She is just amazing 🥰
✨ARIANA GRANDE!!🧡💚💜💛💙❤️_______________________________________________________________________________________________________
I Need MV 🥹🧡
loooovvve this one 🥲
ariana, my girl you listen to the gateway process? If I guessed right will you buy a domain name from me I'M TRYNNA pay all those fam members who helped me and my tutoring classes can't even pay half (I need to pay them back and get rid of their frequencies) I know you'd get me love you ps: my domain has a low estension but the word is empire pls buy lol love u
BEST ALBUMMM OMGGGGG
This is her best song😭♥️ her voice sounds so amazing
This whole album just ate 💅
that key change still gives me chills after 2 months
“imperfect for you” is actually so perfect!
HAPPY RADICAL OPTIMISM DAY ❤❤💋🌺
My Top 5 of Eternal Sunshine (at the moment):
01 We Can't Be Friends
02 Imperfect For You
03 Eternal Sunshine
04 The Boy Is Mine
05 Supernatural
Impossible
idk why but ariana freaking hit the right spot on this album, literally no skips... and this precisely, you'd never catch me dead not repeating it after the first time it plays.
i feel like this song doesn’t get enough credit. it’s a different sound she’s never done and i love it
Aperçu
Paroles
Vidéos
Écouter
Principaux résultats
Mon garçon, viens prendre ma main
My boy, come take my hand
Jetez votre guitare et vos vêtements sur la banquette arrière
Throw your guitar and your clothes in the back seat
Mon amour, ils ne comprennent pas
My love, they don't understand
Mais je garderai ta blessure dans la boîte ici à côté de moi
But I'll hold your hurt in the box here beside me
Comment pourrions-nous savoir
How could we know
On réorganiserait tout le cosmos ?
We'd rearrange all the cosmos?
Mm
Mm
Nous nous sommes écrasés et nous avons brûlé
We crashed, and we burned
Maintenant, je ne peux tout simplement pas aller là où tu ne vas pas
Now I just can't go where you don't go
Et généralement, je suis
And usually, I'm
Foutu, anxieux, trop
Fucked up, anxious, too much
Mais je t'aimerai comme tu as besoin de moi
But I'll love you like you need me to
Imparfait pour toi
Imperfect for you
Désordonné, complètement en détresse
Messy, completely distressed
Mais je ne suis plus comme ça depuis que je t'ai rencontré
But I'm not like that since I met you
Imparfait pour toi
Imperfect for you
Allons-y ce soir
Let's go tonight
Parce qu'il ne reste que quelques secondes jusqu'à demain
'Cause there's just a few seconds left 'til tomorrow
Nous avons tout ce dont nous avons besoin
We have all that we need
Laissons nos bagages et notre vin à table (mm), mm
Let's leave our baggage and wine at the table (mm), mm
Comment pourrions-nous savoir
How could we know
Que c'était un heureux désastre ?
That this was a happy disaster?
(Joyeux désastre) mm (mm)
(Happy disaster) mm (mm)
Je suis content que nous nous soyons écrasés et brûlés (nous nous sommes écrasés et brûlés)
I'm glad we crashed and burned (we crashed and burned)
Je n'oublierai jamais quand tu me l'as dit
I'll never forget when you told me
Tu as dit, d'habitude, je suis
You said, usually, I'm
, trop
, too much
Mais je t'aimerai comme tu as besoin de moi
But I'll love you like you need me to
Imparfait pour toi
Imperfect for you
(Désordonné) désordonné, complètement (en détresse) en détresse
(Messy) messy, completely (distressed) distressed
Mais je ne suis plus comme ça depuis que je t'ai rencontré
But I'm not like that since I met you
Mm, comment pourrions-nous savoir
Mm, how could we know
Nous rendrions les mauvaises choses délicieuses ?
We'd make the bad stuff delightful?
Mm
Mm
Je suis content que nous nous soyons écrasés et brûlés
I'm glad we crashed and burned
Je sais que nous serons là l'un pour l'autre
I know we'll be there for each other
Quand nous nous sentons
When we're feeling
, trop
, too much
Mais je t'aimerai comme tu as besoin de moi
But I'll love you like you need me to
Imparfait pour toi
Imperfect for you
(Désordonné) désordonné, complètement (en détresse) en détresse
(Messy) messy, completely (distressed) distressed
Mais je ne suis plus comme ça depuis que je t'ai rencontré
But I'm not like that since I met you
Imparfait pour toi
Imperfect for you
, trop
, too much
Comme tu as besoin de moi (li-comme si tu as besoin de moi)
Like you need me to (li-like you need me to)
Imparfait pour toi
Imperfect for you
(Désordonné) désordonné, complètement (en détresse) en détresse
(Messy) messy, completely (distressed) distressed
Pas comme ça depuis que je t'ai rencontré
Not like that since I met you
Imparfait pour toi
Imperfect for you
Her voice and the stretching of words reminds me of Joni Mitchell and Billie Holiday.
Ariana helped me get through a recent breakup. Everyday gets a little better. I love this song and the entire album!! 💔❤️🩹💘
i wish this song was pitched down just a smidge. the way she performed it live was amazing
❤❤❤
❤❤❤❤❤
😍🥰🥰
Será el próximo single! Ella ya nos dio un spoiler en un short qué publicó, después seguramente sea true story and bye
This song is literally so underrated!! 💕
Sorry I didn't really listen to every song in the album, sometimes I'm lazy and tired. Anyway, I was shocked when I heard this on the radio! Lol. It sounds heavenly and (idk if this is the right word) like ethereal, 😮a type of music she don't normally have. Also, she sounds good in the live version but I prefer the audio. I feel like the audio version is louder and clearer compared to the live one so the chorus hits different. No hate.
Dayum
supernatural and imperfect for you is the best 🥰🔥🩵💞
✨MESSY?✨😻💘🌺🌺🤍🤍MESSI
1:03 I feel like crying every time this part comes out
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
Madison Cunningham, that's who this song could have been wriiten and sung by- this song- is HER style AND It's HER sound. Madison has songs online that are VERY very similar.
Let me state Ariana has an SNL version i strongly prefer to this version and see this aong has a potential to become Grammy winner too.
I love this
This is the best song of the album no comments. The beats, melody, and literally EVERYTHING is perfect. The "imperfect for you" going higher up adds that last touch that makes it imperfect🫂 This song is WAYY up there
o-k but Y is she singing southern now lmao
❤
Imperfect for you 🫸🏻🫷🏻 Down Bad
What can the #arianators say ariana made a bop on this one yes she did 👍
This song is more than perfect😮😮
"But I'm not like that since i met you"😢 girl you're gonna make me go back to him like stop💔
🫶❤️🔥
❤❤❤
All of it i pick d all the above
🎉 my love you understand kisses 💋 😘 😗 😚 😙 ❤️ 💋 😘 😗 😚 😙 ❤️ 💋 😘 😗 😚 😙 ❤️
That heavenly "Imperfect for you." MADE MY JAW DROP. This song is beautiful.
So stunning
Sounds like a tragic love song that came from an 80s camp horror film.
Cant get over this album! So good, she is another level!
BEST SONG ON THE ALBUM. PERIOD. 🗣️
Youre my eternal sunshine.
her voice is so beautiful ❤
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
this is so addicting
I LOVE THISSS
the piano and HER VOICE, GOSH, QUEEN OF EMOTION♥️♥️♥️♥️
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
The “imperfect for you” key change is so GOOODDD
did anyone else notice the lyrics at the end?
fucked up
anxious
too much
like you need me to
imperfect for you
messy
completely
distressed
like that since i met you
does this mean she realized after the relationship she's been distressed ever since she met the person, not the other way around?
This song is so mesmerizing.. ari sounds perfect in it🤧✨
a beautiful song💛
❤
It feels like music from space. It has like a mixture of RnB and a cinematic sci-fi element. I love it!!
My best friend who is probably her biggest fan made a cover and showed me to prank me and I was so proud of him thinking it was an original but then I saw it was by Ariana lol
the love im growing for this song everyday!
Couldn’t have described this better! 👌🏾❤️
Goddess 🙌🏻🔥
I love you queen ♥️✨
van morrisons sweet thing remixed
I will found it. !!! I AM BELIEVING THAT “ YOU CAN FIND IT IN THE FUTURE. “ THE KEY IS ABSOLUTELY IMPORTANT ….
Concept for MV: Ari as a fairy.
this is such a great different sound i love it so much
Hasta ahora, esta canción todavía no está desactualizada.
If you love this song like I do and you have not seen the Saturday Night Live version she did recently you must GO NOW!!!! IT'S even BETTER than this, she looks and sounds enchanting and they did the set SOOOO beautifully......trust me! go watch it! It's on YouTube!!! Go....go😊 now 😊
Amei ❤
Why This song sounds magical😮....the way she sing 😍
the vibrato at 2:09 “mmm”!!!!!!
Obrigado Ariana por dizer tudo o que eu quero em suas músicas.
OMG! GIRLIE !!!!! the "imperfect for youuu" is FIREEEEE
My favorite
i love this song
i fuginy love you ariana grande
I’m in love with this song.
Лове.бэби.ари.линди.айлавью.🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉😇😇😇😇😇😇😇😇😇💖💖💖💖💖💖💖💖💖💗💗💗💗💗💗💗💗💗💓💓💓💓💓💓💓💓💓💛💛💛💛💛💛💛💛💛💚💚💚💚💚💚💚💚💚💙💙💙💙💙💙💙💙💙🤎🤎🤎🤎🤎🤎🤎🤎🤎🐸🐸🐸🐸🐸🐸🐸🐸🐸💐💐💐💐💐💐💐💐💐🐛🐛🐛🐛🐛🐛🐛🐛🐛💐💐💐💐💐💐💐💐💐🪰🪰🪰🪰🪰🪰🪰🐞🐞🐞🐞🐞💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐💐🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🥀🪰🥀🥀🥀🥀🥀🥀🥀🥀🥀🐞🐞🐞🐞🐞🐞
My fav song from this album ❤
TE AMO MI REINA
😔🥲😔😔😔😔
gives disney vibes idky! sounds like it fits perfect on a high school musical gabriela-troy waltz
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
You might also like
bye
Ariana Grande
eternal sunshine
Ariana Grande
the boy is mine
Ariana Grande
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to (Like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
Weird keychange but addicted
should have been titled (im)perfect for you
I love you like you need me to
0:17 I hold your hurt in the box here beside me 🥹
AAAAAAAA IN LOVE WITH THIIIIIIIIIIIS
my fav *
Impeccable is song
Y love so so much ari💋
The SNL live was so good!
Love the beginning and the key change of imperfect for you. Ariana sings and yet at the same time in her melodic charm talks to us all. ♥️❤😍😘🥰📝🎶🎵👑⚜️💎🏆🫶🏻✨🤩🌟
💔💔💔
Ariana has the perfect voice. Like wow she didn't even sound this good on the show victorious she was on as a teen. She became so big in the music industry. She really does deserve it! She really worked for her dreams and she got it! ❤
Edit: she kinda sounds like a country singer in this song on some lines you can hear it a little bit.
i literally needed this song all of my life and i didn't even know that
In a perfect world Bridgerton would use this song during an epic masquerade ball in a waltz between Penelope and Colin.
Masterpiece
This gives me chills
Baggage and white at the table*
Such a beautiful vibe and song
Este es su siguiente video musical confirmado por ella 😊😊😊
Real 🎉
Happy 1 month
❤❤❤
This is definitely my fav
my favorite song
Loving someone...who doesn't know your love is difficult...he also ignores you...why did you smile and make me love you...why did you act so nice...he just feels that you are imperfect for him...painful
I cried so hard
i lovee
hi
The way she loves and feels her music in her soul😭😭😭
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
LYRICS:
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
🫠 Lyrics 🫠
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
❤❤❤
Ahhh😩😩😩❤❤
this song is the newest sound she’s has to date. nun of her other songs sound anything like this. i love this song. the guitar? the cords? the beat drop! everything it sounds sweet honestly
MY BOY
❤❤❤❤
❤❤❤
Loveeee u girl🎉❤
My fave awwwwww
Same🤜🤛
imperfeita pra você
imperfect for you
Meu garoto, venha segurar minha mão
My boy, come take my hand
Jogue sua guitarra e suas roupas no banco de trás
Throw your guitar and your clothes in the back seat
Meu amor, eles não entendem
My love, they don't understand
Mas eu vou guardar sua dor na caixa aqui do meu lado
But I'll hold your hurt in the box here beside me
Como nós poderíamos saber que
How could we know
Reorganizaríamos todo o cosmos? Hum
We'd rearrange all the cosmos? Mmm
Nós batemos e nos queimamos
We crashed and we burned
Agora simplesmente não posso ir aonde você não vai
Now I just can't go where you don't go
E normalmente eu sou
And usually I'm
Complicada, ansiosa, exagerada
Fucked up, anxious, too much
Mas eu vou te amar como você precisa que eu te ame
But I'll love you like you need me to
Imperfeita pra você
Imperfect for you
Confusa, completamente angustiada
Messy, completely distressed
Mas não sou assim desde que te conheci
But I'm not like that since I met you
Imperfeita pra você
Imperfect for you
Vamos embora hoje à noite
Let's go tonight
Porque faltam apenas alguns segundos para o amanhã
'Causе there's just a few sеconds left till tomorrow
Nós temos tudo o que precisamos
We have all that we need
Vamos deixar nossas malas e vinho na mesa (hum)
Let's leave our baggage and wine at the table (mmm)
Hum, como nós poderíamos saber
Mmm, how could we know
Que isso seria um feliz desastre? Hum (feliz desastre, hum)
That this was a happy disaster? Mmm (happy disaster, mmm)
Estou feliz por termos batido e nos queimado (batido e nos queimado)
I'm glad we crashed and burned (we crashed and burned)
Eu nunca vou esquecer quando você me disse
I'll never forget when you told me
Você disse: Normalmente eu sou
You said: Usually I'm
Complicado (complicado), ansioso (ansioso), exagerado
Fucked up (fucked up), anxious (anxious), too much
Mas eu vou te amar como você precisa que eu te ame
But I'll love you like you need me to
Imperfeita pra você
Imperfect for you
Confusa (confusa), completamente angustiada (angustiada)
Messy (messy), completely distressed (distressed)
Mas não sou assim desde que te conheci
But I'm not like that since I met you
Hum, como nós poderíamos saber que
Mmm, how could we know
Tornaríamos as coisas ruins em deliciosas? Hum
We'd make the bad stuff delightful? Mmm
Estou feliz por termos batido e nos queimado
I'm glad we crashed and burned
Eu sei que estaremos lá um para o outro
I know we'll be there for each other
Quando estivermos nos sentindo
When we're feeling
Complicados (complicados), ansiosos (ansiosos), exagerados
Fucked up (fucked up), anxious (anxious), too much
Mas eu vou te amar como você precisa que eu te ame
But I'll love you like you need me to
Imperfeita pra você
Imperfect for you
Confusa (confusa), completamente angustiada (angustiada)
Messy (messy), completely distressed (distressed)
Mas não sou assim desde que te conheci
But I'm not like that since I met you
Imperfeita pra você
Imperfect for you
Complicada (complicada), ansiosa (ansiosa), exagerada
Fucked up (fucked up), anxious (anxious), too much
Como você precisa que eu te ame
Like you need me to
Imperfeita pra você
Imperfect for you
Confusa (confusa), completamente angustiada (angustiada)
Messy (messy), completely distressed (distressed)
Assim desde que te conheci
Like that since I met you
Imperfeita pra você
Imperfect for you
Ngl I wouldn’t usually say this but hear me out…a remix with lil nas x ?🤔…maybe?
❤
The best song on the album in my opinion hands down!! 🙌🏼❤❤❤😫😫
beautiful
Man this song hits hard! It’s a perfect melody for couples like Harley Quinn & The Joker and all other dysfunctional toxic lovers.
She did what she did but this girl’s pen game😭😭🤌🏾🤌🏾
LẼ RA BÀI NÀY NÊN TẠO NÊN CÂU CHUYỆN VÀ RA MV THÌ ĐI CHỌN WE CANNOT BE FRIENDS SẼ LÀM SỰ CHỦ QUAN RỒI CƯNG
so beautiful 😍😍
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mmm
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Causе there's just a few sеconds left till tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mmm)
Mmm, how could we know
That this was a happy disaster? Mmm (happy disaster, mmm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said: Usually I'm
Fucked up (fucked up), anxious (anxious), too much
But I'll love you like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful? Mmm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up (fucked up), anxious (anxious), too much
But I'll love you like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
But I'm not like that since I met you
Imperfect for you
Fucked up (fucked up), anxious (anxious), too much
Like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
Like that since I met you
Imperfect for you
obsessed with this song
Awh i love this
Distressed...and/or Destressed..Spelled Backwards is: De(s)erted...
Not gunna lie I prefer the song in her low voice the way she did it on SNL it was so beautiful
This should be on a Disney princess movie
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
Hello ariande
นะคะ
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
Fucked up, anxioustoo , toomuch
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
She made this sound like a bop at the drop yes she did yes and yuh
0:03 "my boy.." sounds like the beginning of "Angels like you" by miley cyrus!! I've been trying to figure it out for daysssss why it sounds familiar. the rest of the song is pure magic though ugh
Here after Kelly clarkson said this her favorite song
Eu amei essa❤
Eu amei essa❤
Best on the album..
"imperfect for you"
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
Written by Ariana Grande, Max Martin, ILYA & Peter Kahm.
album eternal sunshine (2024)
This is one of the most beautiful songs I've ever heard. And the acoustic version is even better...
I love how needy was kind of like her validating herself for being so messy but this song shows that she has accepted the messiness and she wouldn't want it any other way❤
Love & Peace.😁
I cry every night. Did you think I didn't understand you? You don't have to feed the monstrous fire that I also felt, but always remember that my love for you is pure and I did everything with good intentions, just like your intentions for me. God, the one who sees everything and knows everything, with that I live in agreement because he looks at us from heaven until the day he sends for me, you are not entirely to blame for what happened, I charge half of it, you are not imperfect for me, I will love you. rest of my life and I will be here for you only because of your divine intention. As for me, since I consider myself a child of God, I do not want you to ever change your authentic life for anything or anyone. Be happy forever you are Blessed you and your family and loved ones you are the best in the world very famous in the entire world Global #1 you just let this story die forever and you will be fine with that make me happy because I don't like seeing you sad that's why I cry when I see the video we can't be friends. I love you so much Ari. I listen to the album every night because tears heal me. I pray to God every night on my knees because he is the one who blesses us. I mention you in my prayers. May he continue to bless you forever. I wish you the best in the world. Thank you for your good wishes. intentions towards me God multiply them thank you for dedicating the video just for me that is more than a gift. God chose me and only I know it and you! I am and more than happy with that because God does not like that I seek fame that is why I hide because he does not like it, I remain a ghost writer. but this is a Blessing that will live within my spirit and my soul, which is what money does not buy and the only thing we take with us. love I love you I love you I love you. It would have been a dream to write a song with you and give you soft kisses on the moon tattoo so I sent them to you LuV 4 eVeR. Eric Mitchell 🤍
Selling
I heard this for the first time yesterday. Now I got it on repeat 🥴
I’m hooked on this song, it’s so beautiful and I love when the beat drops. And her lower register is amazing 🥰😍 my favorite one on the album thus far
Every song in eternal sunshine just keeps getting better and better ✨🤍
I loveeeeeeee
Hii guys , me and my cousin have a music covers channel can u please check this Ariana song cover we made , Tysm ❤ https://youtu.be/UqmQLv14f4k?si=U8c5l-MRanlnhM-H
💌💗❣️💓❤️❤️🔥💞❤️🩹💘🩷💕💝💟💖
I LOVEEE ARIANAAA💖💖💖💖💖
FUCK YESSSSS
The fact that we've all been here through her struggles but has also been sending her love through it all like mannnn.
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
GloRilla 'Yeah Glo!' (Live Performance) | Genius Open Mic
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to (Like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
Omg this.❤
I love this❤
It’s how she hits “each other” for me!
This song just has a certain place in my heart 😭❤️
Is it me or this song gives me ballet vibes 😭💗🎀✨✨✨
This album is my actual therapy. It's just so healing ❤
Necesito true story, the boy is mine y bye
It's a bop yes and yuh or whatever 😊
Imperfect for you actually has a double meaning❤
THE BEST!
😂😂😂❤🎉😊😅essa música e a minha cara
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
Ariana's songs never fail❤
such a beautiful song❤
This song reminds me of Miley Cyrus's The Climb
why "my love, they don't understand " in vinyl booklet is "my love, forget what you've planned" I think the lyrics fit better than the official ones
This song is so magical!
❤❤❤
Me encanta
Temazo
😢😢😢😭😭😢😢😭😭😭😭🤩😍🥰🥹🥹imperfect for you❤❤and You Usually I
literal therapy
My favourite song
I’m sorry ik everyone in their feels but I literally thought I was the only one who drew their T’s like that💀 ts looks like a plus sign😭
Mesmerizing babe🖤😍
Like if this is your favorite song in Eternal Sunshine album
Imperfect for you:
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They dont understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos
Mmm
We crashed and we burned
Now I just can't go where you don't go
And usually I am
Fcked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
Cause there's just a few seconds left 'till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm
How could we know
That this was a happy disaster
(happy disaster)
Mmm
I'm glad we crashed and burned
(we crashed and burned)
I'll never forget when you told me
You said usually I'm
Fcked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm
How could we know
We make the bad stuff delightful
Mmm
I'm glad we crashed and burned
I know we we'll be there for each other
When we're feeling
Fcked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fcked up
Anxious
Too much
Like you need me to
(like you need me to)
Imperfect for you
Messy
Completely
Distressed
Like that since I met you
Imperfect for you
slayyyyyy girllll
“Imperfect For You” is PERFECT for ME ❤️
This song is so sweet and beautiful. Ari is an inspiration so absolutely everyone. She writes heartbreak songs but also love songs. A perfect balance.
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
I don't remember,My head IS not okay 😢😢 IS hurting😭😭🤕🤕 kisses better 😂😂😂
These songs off this album are so good.
This has to be my favorite song on this album BUT IDK BECAUSE ITS TO HARD TO CHOOSE!!!
I love this song the most!!!!
IT LOOKS LIKE A AVRIL LAVIGNE SONG "KEEP HOLDING ON" RIGTH?😮
❤❤❤❤❤❤❤❤
such a beautiful song <3
There’s nothing Taylor has ever done that matches this.
I can die in peace now 🥹🥺
I’ll never be the same again 😫🥹😭
best song on the album
Dont need to bring down an unrelated artist to praise another one
That was not necessary, Taylor’s discography is great
2:07 THIS RIFF 🤍💗💗💗💖
0:01 🎭
I SWEAR IF WE DON'T GET A MUSIC VIDEO OF THIS BALLAD
This is possibly the most romantic song I’ve heard from Ariana and it’s one of my favorites. This song makes me feel so safe🤍
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm Fucked up, anxious
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
This song is slowly
❤
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to (Like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
I loved this album, it's so beautiful, my goodness haha, I identify with this song 💓
Her voice saying "imperfect for you">>>
thissss issss soooo goooooddddd asssss fuuuuckkkk
Este nuevo álbum tiene canciones tan perfectas te encierras en tu habitación por la noche y lo escuchas es un momento maravilloso
Eu não quis que vocês separassem 😢
Warong situation 😊😮
Eu amo o Dalton igual amava o Mac Miller com ela
This situation break My heart😢🥹🥺💔😭
You are not traitor😢😊❤😁😆🥹🥺
Me:
-knows the song is Ariana's
-knows the song is not country
hits play
-clearly hears the synths and distortions
...
-"DEEEEAR JOOOOOOOOOOOHN"
The most Beatle-esque song in Ari's discography ❤
Love
Sounds like she's saying "I Am Perfect for you".
Messy Complete Lydi Stressed
❤️❤️❤️
Favorite things about this somg: key change and warped guitar and drums
Bro this is my new favorite song from Ari ❤
This is my fav song from this album!!!!! Everything in this song is so perfect; the verses, the pre-chorus, chorus. Even the lyrics is so touching. I almost cried in the ending.
This song is so damn beautiful I need a cute video for this
Por favor, senhor Deus, que essa música tenha clipe!
She reminds me of Brandy on this
❤
Yall sleeping on Ari’s favorite song from the album. This song is already underrated 🥺
Its giving High School Musical
The best and most musically genuine track of the album.👌👏👏👏👍
Can't stop listening to it again and again
The song literally gives me chills.
The voice, beat , everything is on point 😭😭❤❤
This is my favourite in the album ❤❤
Love this song...thanks Ariana you're my favourite artist everrr
myyy boy come take my hand
⭐⭐⭐⭐⭐
It's a magical song. I can't explain it in Other words...
Godddddddd
ka nilo ania Ruth i lo khawih
I need to taste it.
You are so delicious
🤤😍
Yo estoy sujeto a Ariana
Oh Misteriosa y Pasionante
I'm anxious. You are so perfect
I love this song so madly deeply
Te amo ❤
Essa música e uma das minhas favoritas do álbum
imperfect for youuuu
This gives me light shower vibes this album ate
✨✨✨
❤❤❤❤
So god ❤❤❤
I love this❤😢 03/17/2024
0:22 reminds me of one of her songs but I can't tell which one someone help😭
literally the best song of the album!
4.8 of 5
I just noticed that every song in this song there is a word "Rearranged"
Everybody glorifying a home wrecker. Yikes.
this is one of the best things i have ever heard jfc
My fav song off the album <3333
Almost is never enough ?
@strawberry-jq9fu no I think it was in dangerous woman album
moonlight or honeymoon avenue ??
@BP_JCLC touch it?
Is it her song “Sometimes”?
WE DON’T CARE IT’S BEEN DEBUNKED
@realestsienna suuuuureeee
@HannahGr8ce the wife gave a statement and page six admitted there’s no evidence that proved she homewrecked and even if she did, who cares? what does that gotta do with you
amoooo
😢😢😊😮❤
perfectly imperfect
❤Sweetly.........rip.......nobody cares........I'm fading.....inn.
❤
This song can't leave my head, why? Because it's on my repeat playlist😍
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
I love you so much
Thank you for this album
Vine aqui por SANA Minatozaki
Need a MV asap
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
😩
Obrigado, #ArianaGrande! Essa é minha. 😍🥹🤧
best song in the album omfg
MY FAVORITE!!!!!
Spanish guitar and castanets? 😮 Love this song
is it just me but on 0:12 the key/tone changed?
This is probably my favourite song in this album
Dare I say album of the year?! I couldn’t speaking to soon but my goodness Ariana!! Grammys let’s go!
it's like they overlay the line "in the back seat"
Yess 🥹🥹 it got deeper
That’s the point
MI FAVORITA LO TENGO
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
the fact ppl forgot its ARIANA GRANDE is CRAZY😅 like she's MOTHER ofc she gon deliver everytime
Te amo linda
My new absolute favorite 😍
will be forever in love with her voice
❤❤❤
I died after I heard imperfect for u line bye 👋
I love it love it love it when she's say "imperfect for you"
heard
Kim Petra's Homework and Ariana Grande's Imperfect for you deserve a mashup.
"my boy, come take my hand throw your guitar and your clothes in the back seat" 😢😩
her lower register is insane you’d think it’s someone else but its still ariana! my versatile queen
❤❤❤❤
Oh my my this is my song❤️❤️❤️❤️❤️
The SNL version of this was better. She does that a lot of times.
This song is so beautiful but the minor key in "imperfect for you" makes me so anxious i can't believe no one has said this already
Todos concuerdan que esta es la mejor canción del álbum, todas las canciones son hermosas, pero esta es la número uno. Se percatan que en gran parte de la canción Ari hace notas bajas, a ella le cuesta hacr notas bajas,pero aquí lo domina totalmente. Te amo mcho Ari ❤
We want a clip!
the best one
I love this song though..
you might be imperfect for him, but this song is just perfect!
Can we have on the next album songs like this? venture more into raw, ballad, guitar. Something ariana really wouldn't do or hasn't tried. Work with other producers hopefully, I think she can bring more and express more of her emotions.
The intro gives strong "Ps! I Love You." vibes.😌
Her singing this live is chefs kiss!
this reminds me of another song but i cannot put my finger on it, listen until i remember which one
My top list:
1 - the boy is mine
2 - we can't be friends (wait for your love)
3 - eternal sunshine
4 - imperfect for you
5 - i wish i hated you
6 - yes, and?
7 - don't wanna break up again
8 - true story (a cappella)
9 - bye
10 - supernatural
11 - intro (end of the world)
12 - ordinary things
13 - saturn returns
I looooveee this album! =)
OMG It's a waltz!! The only pop song to be released in 3/4-time this year? (Someone prove me wrong)...the odd meter gives it an even more off-balance "imperfect" feeling. Come on music theory 😀😼👏
Songs really hit different when you can relate🥲
this was my fav song on the album and we can't be friend too
Bro no way 😞
no puedo dejar de llorar 💔
she sounds incredible
Musicians: whats the technical terminology for the change in chord that arianna does on the "Imperfect for you"?
Someone tell me what this song means I’m lost, this relating to a relationship or herself
imperfect for youuu
The 'Imperfect for you' got me flying like an angel 😩😩 I love you Ari ❤
I want you more
my FAV on this album (perhaps)
Las Flores mas bellas del Cosmos
I NEED A MUSIC VIDEO FOR THIS ❤️
Lyric:
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
Imperfect for you (Guitar cover)
https://youtu.be/eFrkn4N87-w?si=msNciup2sM1G-lkP
This song is tooooooo good
❤
MASTERPIECE
Te amo
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
sweet melody .... sweet voice .... perfect song
Venho aqui pra dizer que essa é a minha preferida do álbum
I love this song
my favorite.
❤
masterpiece
Thi is the definition of compromise
it also kinda sounds like "from the dinning table" from harry styles
what a love letter 🥺
너무 좋다 진짜....
huhu u making me cryyyy this is so good
I’m so thankful for AirPods rn!! Toning everyone and everything out and listening to this album is all I need!!💖
Imperfect for you (Guitar cover)
https://youtu.be/eFrkn4N87-w?si=msNciup2sM1G-lkP
Imperfect for you (Guitar cover)
https://youtu.be/eFrkn4N87-w?si=msNciup2sM1G-lkP
Imperfect for you (Guitar cover)
https://youtu.be/eFrkn4N87-w?si=msNciup2sM1G-lkP
Imperfect for you (Guitar cover)
https://youtu.be/eFrkn4N87-w?si=msNciup2sM1G-lkP
just sent this to him
The best song of the album imo
Wow, that’s amazing! ❤❤❤😍🥰🥹❤️
for me ari you're perfect ilysm my sweet legendary angel thank you for coming back
imperfect/I’m perfect
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to (Li-like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
🥺❤️
masterpiece album like every song is a masterpiece
WHAT IS THIS?, PART: "Messy, completely, distressed. But I'm not like that since I met you, Imperfect for you" </3
Love love
Anyone else getting The Sundays vibe from this track (in the best way) ❤️❤️
The “imperfect for you” gives me COMFORTABLE
Love the imperfect for you at the end👌
Check out my cover :) https://youtu.be/0y665BPbiM0?si=UiOwwwHU_nvL52tn
It’s im perfect for you 🫶🏼
I'm a Cancer sign 🦀 ♋ like queen Ari ❤️ and we are so very emotional right cancer peepz?❤️ 😢💔
ashley ippolito is wrong this song is a banger
im-perfect for u
This is my favourite song on the album
t amo ari
Imperfect for you, I really am an Imperfect boyfriend
cancer tooo i don't like to speak in general about people or signs but maybe i am emotional on some things and maybe it has to do with my personality and not my sign but i found out i have a special bond with people who're cancer
This feels like a siren calling me
Essa música faz eu chorar 😭😭😭😭😭!!
Simplesmente Ariana grande ❤❤❤
Alanis morissette- uninvited
That’s how heart’s feeling these days ❤
ONE OF MY FAVORITE SONGS ON TGE ALBUM😢😍
[LYRICS]
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
This song is imperfectly perfect
My favorite from eternal sunshine 😭💗
My favorite from eternal sunshine 😭💗
My favorite from eternal sunshine 😭💗
My favorite from eternal sunshine 😭💗
I miss this Yours Truly vibe
Is she signing about her and Dalton, or her and Ethan? Seems like Ethan, but lines "How could we know that this was a perfect disaster" makes me think it's about her ex husband 🙃
Cried myself to sleep last night and woke up in tears of joy while listening to Ari.
I love this sm 😢
Wonderfoul album after 4 years she s comeback i ll wait her and i al satisfied❤
😍😍😍 my favorite
Lyrics:
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mm), mm
How could we know
That this was a happy disaster? (Happy disaster) mm (mm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, usually, I'm
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
, too much
But I'll love you like you need me to
Imperfect for you
(Messy) messy, completely (distressed) distressed
But I'm not like that since I met you
Imperfect for you
, too much
Like you need me to (li-like you need me to)
Imperfect for you
(Messy) messy, completely (distressed) distressed
Not like that since I met you
Imperfect for you
this reminds me so much of she's leaving home by the beatles. that sorta fairytale feeling
I can see miley cyrus covering this song. Definitely one of my top faves in this album.
i could believe this 😭😭😭
let’s keep the streams stable on spotify
stream! stream!
im not rlly an ariana grande fan but wowww this is so good
I think the "imperfect for you" dissonance actually symbolizes the perfection in imperfection. We would expect a melody with notes from the same key, until the dissonance comes in causing the melody to be imperfect but it becomes a theme of the song, making it stand out and special.
love nonna <3
Underrated af
This has to be one of the best songs in this album
Favourite song on this album...LOVE THIS ALBUM...❤ 💛☀❤ 💛☀ ❤
Very country vibes.. I like it..
glinda's sound coming thru during the "imperfect for you" part ✨ 💖
my favorite forever
The best song in this album IMO. Hope we got an MV for this song
This song is too underrated
The lyrics omg
i'm crying my eyes out this is to perfect
Essa música me dá esperança
YOU SOUND LIKE AN ANGEL
her vocals 🤌🏻
00:40
It's giving fairy vibesssssssss
This sounds so much like Next to Normal’s “Perfect for You”!! Wonder if Ariana is a fan
the “imperfect for you” got me floating
This is the 2024 version of needy😭
This song gives me Steven universe vibes
I love how she sings "imperfect for you" imperfectly off-key
This song will be for my wedding dance🫶
IM IN LOVE❤❤
don't let her hold the pen, it's too heartbreaking😭 ILOVEYOU MOTHER ARIIIII♡
🥹💋
The music reminds me of that Soundgarden and Temple Of The Dog sound.
Chris Cornell and Company influence ?
The low singing is everything! Obsessed
this song has my heart❤
🌿🌙🤍
omg love it
The best in the album.
needy 2.0!! just me?? but happier version 💌
Love this song
Sabrina carpenter should feature
Is it "imperfect for you" or "I'm perfect for you"?
Humans are imperfect but there will be one who will be perfect for you despite his/her flaws.
The “imperfect for you” gives me chills OMGGGGGGGGGGG
FOR SUREE🤭🩷
imperfect
@someonehehe617 I know. I tried to play with both in order to show despite being imperfect, someone will find us perfect the way we are.
i love you
ariana has been doing some crazy music theory things
I want a remix with Taylorrrrrr 😭🩷
"imperfect for you" UGH AMAZING
We can all agree this is one of her best albums like omg she’s phenomenal!!!!
Imperfect for you but still will gonna love you forever. ❤
Imperfect for you - Lyrics
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know we'd rearrange all the cosmos?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table
How could we know that this was a happy disaster?
I'm glad we crashed and burned
I'll never forget when you told me
You said, "Usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
How could we know we'd make the bad stuff delightful?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
めちゃくちゃ可愛い大好き😭🩷🩷🩷✨
Gente, isso me lembra demais a Música de um Amor para Recordar
A melhor música do álbum ! Estou sentindo no coração
Goddammit! This is how she keeps getting away with out of pocket shit! The bops are too good! 😭 Fine Ms. Grande, take my critical thinking!
2:18
Donald Marshall illuminiati whistleblower exposes many of the illuminiati's secrets.
❤❤❤❤ live laugh love arii 🎉 1:14
I CANT GET THIS SONG OUT OF MY HEADDD AHHH she done it again boys n girls
Im-perfect for you
OBBSESSSSEDDSS
the “imperfect for you” is so angelic
I can’t stop listening to thisssss
Sweet ❤
This is the best song by far 😭 perfect touch of melchony
Lyrics ☀️🍒
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know we'd rearrange all the cosmos?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table
How could we know that this was a happy disaster?
I'm glad we crashed and burned
I'll never forget when you told me
You said, "Usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
How could we know we'd make the bad stuff delightful?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
this is the one
This song… the beginning the deeper voice on « my boy » incredible. The castanets!! The trippy « imperfect for you ». This song is so different of everything else Ari did, amazing
I want this song in my storie of instagram but it doesnt appear!! Whyyyy
im 🤭 perfect 🤩 for 🤤 youa 🤪
❤️🔥
We need acapella version❤❤
Wow this is amazing.
I really love all the album, but when I hear for the first time this song I feel something special, my favourite song ❤️
<3
Still can’t convince me that stealing a married man is good for you. Girl you’re kicking the can down the road. The immediate gratification you get will fade like everything else does.
Ily💕
this keeps me vibes off her 2015-2018 music idk why tbh
Linda
My favorite
The “I’m glad we crashed and burned” part always gets me 😢 I love this song
Lets discuss how every song of this album is such a perfect combination between perfecf lyrics and such a beautiful voice
Love this song
Is it normal that some parts remind me of Only Hope from A walk to Remember
Wow ❤
Actually am in love with this
She nailed that ❤
💔
𝒊𝒎𝒑𝒆𝒓𝒇𝒆𝒄𝒕 4 𝒖…
Amo
Already playing it on my piano by myself bc there are still no piano covers😭
the best
This song and the “imperfect for you” is just magical!
THE FUCKING VOCALS😍😍😍😍
the "imperfect for you" completed my life.
❤❤
❤❤❤❤❤
Delightful
Be there’re for each other
Fucked up, anxious
This is the best👍💯
"im - per - fect - for - you" those heavenly notes literally lift me up....
I kinda get it tbh. The little part where it changes its meant to represnet the "imperfect" because it's almost like someone accidently pressed the wrong chord and it's intentional of course. In a technical musical sense, this wouldn't work but in the case of that song it completely does. It's almost like she's saying that imperfections ARE her perfections. Maybe I'm reading too much into this though 💀
I don’t think there’s ever been an Ariana Grande album where she has. It made me cry.❤
when she says usually im fked up anxious too much but i love you like you need me too , imperfect for you always gives me chills 0:43
Wait, could “we crashed and we burned” be a reference to honeymoon avenue “before we both crash and burn” ???? ARIANA WHATTTTT
Hermoso álbum , me encantó 🤎
I can't imagine being his ex wife and listening to this album. Goddamn
This gives me Sabrina Carpenter vibes jnknknk I love it <3
Who came back to hear again after Snl epic performance🎉🎉🎉🎉🎉🎉🎉🎉🎉🎉❤❤❤
Its giving country
Wow - are all the songs on this album instant classics.... wonderful
Save 2024 queen 👑
❤
So beautiful def my fav song on her album so far ❤❤❤ this is so my vibes
MY FAV IN THE ALBUM WHAT 😭😭😭😭
Idk why but I feel sad listening to this one 🥺
Ari ❤
She usingg the simplicity ia beautiful and lasting everlre than doing the hits coz the fans just loving juat being her
Luv too
ITS GIVING TWILIGHT AND TINKERBELL AND IDK HOW TO EXPLAIN IT BUT ATE
guys is this song not on instagram? cuz i wanted to post it but couldn't. anybody got the same problem or just me?
Queen
I just found my wedding song
Perfect
How I love this song so much🥹
bestttttttttt everrrrr !
sabrina carpenter takes inspo from ariana to begin with
This made me feel emotions i never knew existed.
So beautiful as always ma'am😢❤
Instant classic ❤
❤❤❤
I love how the title can also be interpreted as “I’m perfect for you.”
But hands down this is one of the best songs I’ve heard in a very long time. It gives me chills it’s so good.
🥹❤️
This song is so good, I would LOVE it if ROSÉ of BLACKPINK did a cover to this 😭😭🖤💗 I just know it’ll sound amazing with her voice too.
Ari strikes them cords 😢❤
❤❤❤❤❤ omg this is literally heaven
Best song on the album.
So ironic how a song called "imperfect for you" is THIS PERFECT!
I love the way she sang « impeferct gor you » ant also when she says « happy disasteeerr » THIS SOUNDS HEANVENLY😇🤍
this is my favorite 💭
one of my favorites
Listening to this song feels like heaven ❤
i love “fucked up, anxious, too much, but i’ll love you like you need me too” so much. my fav part
grammy must already carve her name.
This is giving sza vibes and I hope this has a music video🤞
✨✨✨✨✨
how could we know we’d rearrange all the cosmos? ARE U KIDDING ME 😭😭🥹🥹🫧🫧🫧🫧☀️☀️☀️
Same💆🏻♀️
That's my favorite part as well! Ariana has the perfect voice. Like wow she didn't even sound this good on the show victorious she was on as a teen. She became so big in the music industry. She really does deserve it! She really worked for her dreams and she got it! ❤
aoty worthy
Sounds like “Keep Holding on” by Avril Lavigne at the beginning
she slay with this song on SNL
Saw her perform this on SNL 🔥
im self reflectiingggg
Imperfect for you trueeee!!!
Her voice alone is soothing then you add the lyrics and…. ahhhh just yes yes yes 🎉
this song looks like with south of Texas Music, I adore that
Albums a bore-off x
Taking us back to the 2000s!!!
whith this song you know that she is a broadway girl
pov: when you finally realize that every song on the album is actually your favorite
Imperfect for you or enough for you, i don't know which one hurts me more 😢
Aunque me gustes un chingo ...💌JP
this is my new "POV" in this album I'm in love with this track
Oh my god 😩 thiiiiiiiiiiis
The way she sings the "imperfect for you" is imperfect like it sounds off key and I freaking love it!!!!
❤❤❤🫧❤️✨🌱
❤
Wow amazing amazing voice I love it
I love the rhythm of this song it's very very goooooooood
This song is giving Nickelodeon vibes
hurt, cried, tf
Ari sempre arrasando não é mesmo ❤️😍😍😍
This gives Alanis vibes! 😩💕
Es una canción opuesta a Pov, pero con el mismo sentido, Mi Ari bella 😢❤
AMO AMO NO ME CANSO
I don’t understand the higher voice…?
This is so cute
Dm or private chat
Literally cant stop listening to this. Truely magical
Still a nasty itch
It's giving WICKED and I'm here for it. What a beautiful song ❤
this will be at my top 3 fr fr ♡
She needs to remix this with Sabrina Carpenter😭😭😭😭
este álbum no para de sacarme sonrisas 😢
2:00 me mata
qué ternura 🥹
<3
the best song on the album
Omg, thanks for so many new songs. We Love You
Can’t even pick my fav! I covered imperfect for you and more songs from this masterpiece album, would love if you checked it out, thx!😊
WOW I LOVE THIS ALBUM ❤❤❤❤❤
let’s hit a million views
the first verse hits different
I've never heard Ariana with emotional guitars before.❤❤😢😢
The "imperfect for you" is wooooooow
New fav ❤
É simplesmente a minha preferida do álbum inteiro!!!💕🇧🇷
IM SORRY BUT THAT “imperfect for you” DOES SOMETHING TO ME
this is like pov’s better more mature sister i love it so much
this song stood out to me , I love the key change ❤
imperfect for you is such a mood!!!! ❤️😭❤️
the lyricsssss 😮
love u ariii
🧎🏾
Now this….this is the ari we know and love. Passionate, kind and smart. So glad to have u back ❤
I need a Taylor Swift remix for this song immediately. They would both kill it on this track 😭🩷
“Imperfect for you” 🩰
This is the billionth time I've listened to this 😬❤
My boy, come take my hand
Meu garoto, venha segurar minha mão
Throw your guitar and your clothes in the back seat
Jogue sua guitarra e suas roupas no banco de trás
My love, they don't understand
Meu amor, eles não entendem
But I'll hold your hurt in the box here beside me
Mas eu vou guardar sua dor na caixa aqui do meu lado
How could we know
Como nós poderíamos saber que
We'd rearrange all the cosmos? Mmm
Reorganizaríamos todo o cosmos? Hum
We crashed and we burned
Nós batemos e nos queimamos
Now I just can't go where you don't go
Agora simplesmente não posso ir aonde você não vai
And usually I'm
E normalmente eu sou
Fucked up, anxious, too much
Complicada, ansiosa, exagerada
But I'll love you like you need me to
Mas eu vou te amar como você precisa que eu te ame
Imperfect for you
Imperfeita pra você
Messy, completely distressed
Confusa, completamente angustiada
But I'm not like that since I met you
Mas não sou assim desde que te conheci
Imperfect for you
Imperfeita pra você
Let's go tonight
Vamos embora hoje à noite
'Causе there's just a few sеconds left till tomorrow
Porque faltam apenas alguns segundos para o amanhã
We have all that we need
Nós temos tudo o que precisamos
Let's leave our baggage and wine at the table (mmm)
Vamos deixar nossas malas e vinho na mesa (hum)
Mmm, how could we know
Hum, como nós poderíamos saber
That this was a happy disaster? Mmm (happy disaster, mmm)
Que isso seria um feliz desastre? Hum (feliz desastre, hum)
I'm glad we crashed and burned (we crashed and burned)
Estou feliz por termos batido e nos queimado (batido e nos queimado)
I'll never forget when you told me
Eu nunca vou esquecer quando você me disse
You said: Usually I'm
Você disse: Normalmente eu sou
Fucked up (fucked up), anxious (anxious), too much
Complicado (complicado), ansioso (ansioso), exagerado
But I'll love you like you need me to
Mas eu vou te amar como você precisa que eu te ame
Imperfect for you
Imperfeita pra você
Messy (messy), completely distressed (distressed)
Confusa (confusa), completamente angustiada (angustiada)
But I'm not like that since I met you
Mas não sou assim desde que te conheci
Mmm, how could we know
Hum, como nós poderíamos saber que
We'd make the bad stuff delightful? Mmm
Tornaríamos as coisas ruins em deliciosas? Hum
I'm glad we crashed and burned
Estou feliz por termos batido e nos queimado
I know we'll be there for each other
Eu sei que estaremos lá um para o outro
When we're feeling
Quando estivermos nos sentindo
Fucked up (fucked up), anxious (anxious), too much
Complicados (complicados), ansiosos (ansiosos), exagerados
But I'll love you like you need me to
Mas eu vou te amar como você precisa que eu te ame
Imperfect for you
Imperfeita pra você
Messy (messy), completely distressed (distressed)
Confusa (confusa), completamente angustiada (angustiada)
But I'm not like that since I met you
Mas não sou assim desde que te conheci
Imperfect for you
Imperfeita pra você
Fucked up (fucked up), anxious (anxious), too much
Complicada (complicada), ansiosa (ansiosa), exagerada
Like you need me to
Como você precisa que eu te ame
Imperfect for you
Imperfeita pra você
Messy (messy), completely distressed (distressed)
Confusa (confusa), completamente angustiada (angustiada)
Like that since I met you
Assim desde que te conheci
Imperfect for you
Imperfeita pra você
❤❤❤❤
❤❤❤❤❤❤❤
Essa é linda demias❤
I love this song waaaaaay too much!!!!!! 🩷
So we’re all here crying our eyes out??
Obsessed
Made me cry😭
Me encanta que haga pequeños guiños a trabajos anteriores, como acá con "we crashed and we burned" haciendo referiencia a honeymoon avenue 🥰
SO FUCKING GOOD!!🩷
giving your heart to Jesus is the best decision that can ever be made. i can assure and promise you that if anything 🧡
Jesus loves you and died for you 🤍 so you can be set free and know Him personally 🤍 In Him is the fullness of fulfillment & eternal security
This song for me is in second place because in first place there is "bye"
😍😍😍😍
Essa é muito boa
Another song😍😍😍😍😍😍😍😍
The only good song of the album… It reminds me of Avril Lavigne first album
Tão fofa essa música e me lembra outra música na qual eu não estou lembrada
❤️❤️.
🩷🩷🩷
☀️☀️☀️
This describes me too well 🥹
Very different style and it's like come from past
That’s my fav from the album. The chorus is so god damn beautiful.
I'm into it, but not too much as the rest of released albums
Minha favorita 😍😍😍😍😍
💚💚💚
this is my favourite song on eternal sunshine. when i first heard the line “imperfect for you” i got chills. and the beat when she says “f’ed up, anxious, too much..” it’s so pretty
The "Imperfect for you" It is the best😢
It’s those castanets in the instrumentals of the chorus too 🤌🏾✨
came here looking for the one “imperfect for you” comment but it’s literally everyone here 🤌🏾✨
blake shelton's favourite song
imperfect for you Lyrics
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Bridge]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to (Like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
it sounds like a lullaby
oh my god i'm addicted ariana the woman you areeee
❤️❤️❤️❤️🎧🎧🎧🎧🎧
Ma chanson préférée ☄️
Ariana I'd going to save the music industry this year
her voice is so disney princess
❤
imperfect4u
aawwawwwawawawawawaw
When you get to heaven, I'm sure the angels' voices will sound similar.
0:02
SOOOOOOO HAVENLY❤❤❤❤
❤
I lovveeeeee this song
Ohh this is a masterpiece truly!❤️🔥❤️🔥
❤
This gives off very 'Yours Truly' vibe 😍😍😍
omg THE IMPERFECT FOR YOUUY
Amo❤❤
Imperfect for u❤
This song is underrated 😭.
YOU MADE ME CRY 😭😭😭
Listening to 'imperfect for you' on repeat like it's my full-time job, and honestly, I should start putting it on my resume. Thanks, Ariana Grande!
YOU CAN FEEL THE EMOTIONS IN HER VOICE ❤
Music’s too sad without you - Kylie minogue? 🤔🤔🤔
Favorite song on this album ❤❤❤
YASSSSS QUEEN SLAYYYYYY
It deserves a MV >_<
This is my fav song from this album so far!!!!!!!
I agree
The best track of this album periodttttt
Eu te amo Ari ❤
Perfect
I think the ‘imperfect for u~ part is based on the movie’s musical scoring.
This album has my heart❤️
imperfect for you... im perfect for you. HELP, i cannot get over this song 😭
💔🔥
this is probably my favorite song on the album
Slayyyyyyyy❤❤❤❤❤❤❤❤❤❤❤
FAV
i love everything about this song
Was not a fan before especially after the affair but listening to this album makes me appreciate her as an artist
pov 2.0 me fascinaaaaaaaa
❤❤❤
from a subjective point of view ;"THROW YOUR GUITAR AND YOUR CLOTHES IN THE BACK SEAT ",implying of picking up your love for a sleepover after an exhausting day ,is the true definition of happiness and pure excitement !,ughhh this song gives me chills
it’s giving christmas vibes at “imperfect for you”❤
one of my favorite songs from this album ❤
OMG
our girl has done it again ❤😭😍
amoo🥺
True
there's no affair sweetie that has been debunked......
@MinaMyouiiii suuuuuure
@MinaMyouiiii you know what....maybe you're right. Even if she did have one. It's not my place to judge her. I love this album, that's all I know
MY ABSOLUTE FAVORITE SONGGGG
THEY KEY CHANGE WHEN SHE SAYS “IMPERFECT FOR YOU” OMLLL
Essa música tem muito uma vibe Red Velvet
Que música boa, Ariana do céu!
Wow I can’t believe she played Cat! She is so amazing. Her voice is beautiful 🎉
AMOOOO
I feel filled with joy and sadness at the same time while listening to this melody 🥹
Woooooow
MASTERPIECE
"Imperfect for you" actually gave me goosebumps. This whole album is a masterpiece. I love love love it queen Ari!!!
The “imperfect for you” is PERFECT AHHHH
best song omggg
Çok hoş:"
4 years that is worth waiting
I give up,,,
My fave from the albuuum😭🤍
Another song that I'll be adding to my playlist "songs that describe me" aside from needy 😫
within one day listening to it over and over i learnt the lyrics js by listening
THIS SONG IS THE BEST. I LOVE IT ALMOST AS MUCH AS I LOVE ARIANA. ME AND MY BESTIE ARE YOUR BIGGEST FANS EVERR PLEASE GO ON TOUR <3
(youre the reason i passed my test)
That my boy hits so different
Omg this album 🎉 mind-blowing!!
Love that one hits deep ❤
The best of the album
❤
I made a clean lyric video to this song if anyone wanted to listen:
https://youtu.be/4JIIGA3j5tQ?si=B-Q5aptbFmpmFSTh
OMG ♥♥♥♥♥
❤🎉❤
This albulm is freaking perfect her best so far the only thing is i wish she did a song that shows her belting because i love her belts her vocals were safe on this albulm..
❤️
"Imperfect for you" is omg❤❤🤌🏻🤌🏻🤌🏻🫂
this song is my fav from the labum..truly captured its essence by ari
Finally, Ari has an album that helps you make peace with yourself.
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to (Li-like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
omg my fav 😭😭😭😭😭😭😭
the key change when imperfect is sung
i luv this so much. its so intimate and cute
Am soo happy her voice has never change 🥺🥺
Love Air❤❤
I'm not crying.. you are :(
so this is the song she+s performing on snl? i loveeeeeeee it
My favourite on the album. 🥺🤍
This whole song is kinda perfectly imperfect.. like music coming out of that magical broken music box
Great to hear peaceful in your song
That melancholic tone is so damnnn finee 😭😭
This sounds so much like a Lana del rey song!
My favorite on the album! 💘
This album is so winning Album Of The Year!👏👏👏
❤❤❤Any body supports Ariana Grande's new album? https://youtu.be/qeXIlMGtc2Q
Found my fav🎉
this is so good
“imperfect for you” ✨ heaven
this song really grows in me in every listen
the best title.
Only bops on this album
Wow ❤ ❤
The backing vocals😻😻
this is my fave yet off the album and supernatural 🥺 i'm a sucker for her low registers
This song is so beautiful. This is an elevated version of pov in my opinion
Got this song looped for hours 🥺
the notes on the “imperfect for you” makes me go over the mood love u sm ari
Most beautiful song of the álbum wtf 🥺
New fave
Best song of the album imo
"Imperfect for you" sounds out of place in a good way. It feels wrong and right at the same time. 🌚
Those “Imperfect for you” are something else
this is so Disney I love it
1:21
My ex loved David Phelps, Ariana, heathers, etc. I miss her with all my heart and I pray that some day I can prove to her I’m Better than I was. She’s 26 now, so I’m sure she will find an older man and live her life, but a man can dream.
the production is crazy. this album is so good. ariana is a hit maker!
Omg
AOTY
the intro sounds like a disney song
My favorite its giving sweetener
my favoriteeee
The absurd out of place key for the line "Imperfect For You" send the meaning literally IMPERFECT. Brilliant detail😘😘
The slight crack in her voice in the last chorus on the hated had me sobbing 🥺😭
"imperfect for you"
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
My favorite from now
👌🏽 damn thats so good
OMGGGGG :(
The way she says imperfect for you ❤❤❤!!! 🫢👌😮
we need a movie for this whole album
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Eternal Sunshine of The Spotless Mind
Her pronunciation is different on this album. So crisp and clear
🌟✨️🌟✨️🌟✨️🌟✨️
when she said "i wish i hated you" you can feel that she is very sadd, i really feel it.
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
this album is a masterpiece
i really hope there is a music video for this one
this is my favorite song on the album its so good
❤❤
reminds me of Sometimes It Snows In April
Fckinggggggggggg gooooodddd💗💗💗💗💗💗💗💗💗💗💗💗💗
Oh, this one is my FAVORITE SONG for SURE!!
The key change threw me off-guard that I felt goosebumps all over my body. As of this moment, this song is my favorite
Something about this album and miley cyrus's recent songs are making me feel so nostalgic
❤❤❤❤❤❤❤❤❤
OMGGGGGG 😭❤️❤️❤️❤️
We see you wicked vocal coaches, thank you haha
Why this reminds me of a melanie martinez song especially the chorus... Ari you are loved, I love you, I say this is her best album to date no skips just pure emotion
Sooo cute, one of the best lyrically on the album, 8.5/10
658.540 views
they key change sounds so magical
She knows she won with this album, agent who should give us a gift, she gives us one💖 thank you, thank you Ari 🤗🤗❤❤❤
agree! I covered imperfect for you, would love if you checked it out, thx! :)
Lufff ewww Ariana ❤😂❤😂❤😂❤😂❤😂
A VOZ ANGELICAL 🥹💘
This would make a really good country cover
I REALLY NEED YOUR BALLAD SONG, PLEASE.... 😭😭😭😭😭😭😭😭
This is giving high school musical vibes. Love it
this is giving HSM rooftop scene
AG7 the best 🥺♥️♥️
My favorite song on the album! Love it!
DIOS MÍO QUE CANCIÓN
best song of the album no one can convince me otherwise
oh this is perfect for me 🥺
Omg so perfect
lyrics
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
I love it Ariana!!💖💖💖
yup this is my favourite
Lyrics plz!!!!
Bowie vibes ❤
I WILL HAVE A SONG WITH ARIANA GRANDE ONE DAY !
THAT IMPERFECT IS PERFECCTTT
this album is such a vibe thank you Ari <3
Samee❤
These are the lyrics what😂
PERFECT!
No words just welcome back we missed you this album is so is awesome ❤️❤️❤️❤️❤️❤️ 😪😪😪😪😪😪😪 love you so much ❤️
Every song on this album is amazing.
I’m happy for Ariana fans that love this album, but this album just doesn’t hit….it has almost no energy with the exception of maybe 4 songs including the 1st single. Happy for Ariana tho, this album sounds like it was very therapeutic for her
My fav song from the album 💕🫧✨
o comeco me lembrou MUITO Dear John
Okay so I’m 12 tracks deep and miss ari really poured her heart out into this album 🥹
TE AMO
Minha favorita ❤
a maior de todas
i hear this song right now at my office room while rain outside and don't know anything about relationship coz i'm all alone. but this song make me cozy.
her voice is a pure therapy, heavenly beautiful.
im-perfect for u
mds amei demais essaaaa
so beautiful. haunting lullaby. "you said youuusually i'm ..." and the vocal skip at " but i'll love uuuuu" plus new magical harmonies in the outro omg
COME TO BRAZIL QUEEN 🇧🇷🫶🏻🥺
Lendaa
Amooo
Iconic
Hinooo
Te lyrics are so beautiful
❤️❤️❤️
Imperfect for you just is son heavenly.
this makes me sad but happy at the same time HWURHWKHFJAHD ARGHH💗💗
Te amo ariana gracias por este album increíble.❤
I NEED THIS VERSION WITH ITS ACCOUSTIC AKSHSAKSHAKAJAJAJAAKAKAJAJ ARIANA GRANDE-BUTERA I BEG TO YOUUUUU
“Imperfect for you” is PERFECT FOR YOU
Grammy 2025 winner on the way....................Amin
Osm new in market and every one will copy you❤❤❤😊
Te amo 💗
❤❤
i love this one
🤢🤢🤢🤢🤢🤢🤢🤢🤢🤢🤢🤢🤢
This album is special
The 1st Pop album i really like every single track since Taylor's 1989.
"imperfect for you" ☀️✨
💗
<343
I'm so in love with this Album Ari definitely did her big one . I cried listening to this Album but was happy at the same time. I feel this Album was about her writing and sending a love letter to Dalton and her forgiving him for everything that happened
Okay everyone can now go and seek a married man with a pregnant wife and make music about it . Imperfect
ITS OUT
Está canción es una de mis favoritas.
Yo siento que esta canción es para Ethan definitivamente...
her best song on the album.
Awesome song! Amazing vocal!! 🎉❤
cura senhor onde doi
mi niña tan bella <3
So goooood ❤️❤️😭
And the Grammy goes to Ari❤
you are so perfect! i love you 😭❤️🔥❤️🔥❤️🔥❤️🔥
❤❤❤❤❤🎉🎉🎉🎉🎉✨✨✨✨✨✨✨
This song illustrates perfectly how our self-perception and pride can get in the way of our relationships with people. It’s okay to be flawed. How do you have a flawed marriage? I think that’s a question I’ve been reflecting on. I feel like at the core, our generation, is going to have to reconstruct what the institution of marriage looks like to us. Is marriage forcing someone to be a version of themselves that makes me feel comfortable or is it dealing with someone you don’t like just to avoid being alone? I ask these questions because I think we all have that stubbornness towards change and growth, we all have moments where we want to a better person and moments where we just want to be whatever. How do you create a marriage that is okay with all versions of you? That’s a very high form of love that takes a lot to work towards—and too many people give up rather than work on it. Maybe that’s why open marriages are on the rise.
pretty voice. too bad shes such a ho ho ho. I used to like her even if i thought she was a little insecure and weird with the tv voice etc please tll me this isnt her love song for that sleezy red head guy who left his wife and new. baby for her yuck .. too old for that shit. break up with your gf, yes, and what?, this whole album.. yuck
thank you ari ❤
this album is everything i needed ❤❤
"imperfect for you" AAAAAAAAAAAA YESS GIRL SURVED
I LOVE THIS SONG BRO
😭😭😭 sobbing crying
ARIANA IS GIVING EVERYTHING IN THIS ALBUM❤
One of the best songs from this album.
My favourite ❤
im so imperfect for you! 🥺
“Break up with your gf” was years ago, and has nothing to do with this situation.
2:00 THE WAY SHE SINGS DELIGHTFUL IS SO DELIGHTFUL
My god. I'm in love with this song. It sounds extra special when you have by your side someone that loves everything about you, even your imperfections and vulnerabilities 😮❤
🤍
underrated. one of the best
omg ilysm😭😭ariana
Love how this gives me Melanie Martinez vibes 🧚🏼♂️🪐✨
Song of the yr.
Vai se fuder mulher você é perfeita te amo como oode você pequena fazer uma letra desse tamanho
wow❤❤❤
Loves when she says Imperfect For You
Gorgeous album let’s get it to #1 all the arianators 👇
No entiendo el autotune
THE BEST IN THE WORLD
this is my fav <3
I love everything about this song so much ❤❤
We can't be friends and true story are my fav songs from this album. But i like all!
Ari, you are my eternal sunshine.
imperfect for u
Love you sm ari ❤
0:51
It feels so comforting
my favorite song now.
MÚSICA DA CARREIRA!
This song happened to come from paradise? Because I feel there as soon as I hear it
THIS SONG WILL CERTAINLY PLAY AT MY 15th BIRTHDAY WALTZ, AT MY GRADUATION AND AT MY WEDDING.
She's story telling y'all😭😭
2000 teen romance movies be like: ❤❤️🫂
Doesn't sound like a generic song, it's so unique. Loveee this album!
One of my favorites on the album
esse é de longe o melhor album dela, senti cada música
❤❤❤❤❤
Te amo Ari❤
Imperfec for you e we can't be friends (wait for your love) são às minhas músicas favoritas do álbum Ariana grande sempre entregando com tudo<3
❤❤❤
AWESOME!!
ariana's pop-country song???
2:00 SO GOOD
eu cantei não foi pôr cantar e sim porquê é uma arte magnífica e não pretendo parar de investir em arte musical!!!beijo emiliana do manejo.
« I’m glad We crashed and burned » imperfect for you-2024
« before we both crash and burn » honeymoon avenue-2013
I want Madison Beer to cover this so bad😭
Frfr
country🤣
This reminds me of K-12 songs melody
OMGGGG LOVE THIS SONG NOWWWWW❤❤❤❤❤
This might be my favorite song in her album ❤
I LOVE THIS SONG SM NOW
in love with this album
my personal favourite ❤️ something about it makes me feel so moved and emotional. i love the meaning behind it. you never fail in making masterpieces, ari. your brilliant mind is something else. thank you for going out your way into producing all these work of art and share it to the world.
The whole album is such a gem babe 🥹💖 thank you so much babe
Ok this album is ass 😅
I like the note changes in the beat a lil after 0:21 and 0:31 I don’t know what you call these but it compliments her voice so much. These small changes throughout the song is what makes a big difference to me!!! Amazing Ariana. You’ve taken it. 🩵
I love you ❤
😍😍😍😍😍😍😍😍I Love❤❤❤❤❤❤
No me decido sobre cuál es la mejor canción del álbum, todas son muy buenas 😭
my favourite from this album. ❤
LA MEJOR CANCIÓN DE TODO EL ÁLBUM!!!
Am I the only one who feels that this would be AMAZING with a SZA FEATURE!!
Imperfect for you is perfect ❤
Her pen is so lethal the double meaning with “imperfect for you” and “ I’m perfect for you”
Another fave on the album. I feel like I'm on a carousel listening to this one 🎠
Her voice, this song i love it im loving
2:38 until the end is so ethereal and beautiful 😩
hyunjin from loossemble😱😱😱
I noticed it and I can't stop talking about it it's so good
whered u get that from
hey there, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Yes🥹☀️♾️
That's deep
I can't understand it hahaha
OMG
WOW!!!
@zamraykiefer9245 it's like a duality when she said "imperfect for you" and on the other side "I am (I'm; Im) perfect for you.
At least that's what I understand
Super lethal
nah
not her pen
EXACTLY
@user-fw4vl6qr4o yes, her pen. she wrote every song tho
Hii guys , me and my cousin have a music covers channel can u please check this Ariana song cover we made , Tysm ❤ https://youtu.be/UqmQLv14f4k?si=U8c5l-MRanlnhM-H
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
Well, not her pen. her teams….
Well, not her pen. her teams….
Haters are saying "not her, her team"... ok swifters go home
@nnsjoao late, but as a swiftie i LOVE ariana’s songwriting!
@Austinn1234 also, she writes her songs BY HERSELF and other people of her team will edit or make changes to make it seem smoother.
@moonecakes3749 exactly
@Austinn1234 she wrote this…
@user-fw4vl6qr4o you do realize she can write her song and then talk to her team about it and refine it later right? That’s what artists do for any media in the professional world.
the way she says "imperfect for you" aa😍
This is soul touching
Ariana Grande I love you very much, a big hug and kisses, take care of yourself. Thank you Imperfect For You ❤️🩵💛💜🩷🤍💙🧡💚😀🤗🥳🤩😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😊😍🥰😘🎉🎊🎇🎆🪩💯💯👱🏻♀️🙏🏻👍🏻🫶🏻🫰🏻🫧☁💧🌙💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋
OMGGG
What a father, the song, Imperfect For You I love you very much, a big hug, take care of yourself Ariana Grande ❤️🩵💛💜🩷🤍💙🧡💚😀🤗🥳🤩😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😭😍🥰😘🎉🎊🎇🎆🪩💯💯👱🏻♀️🙏🏻👍🏻🫶🏻🫰🏻🫧☁💧🌙💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋💋
I think this is my favorite song off of the album <3!
Why did these lyrics touch my soul.. God bring me someone like her.. Open and authentic heart on her sleeve never to be afraid to express what they are feeling embracing imperfections..
This song feels so real her captivating vocals cured me as usual tho
"Imperfect for you" GAVE ME GOOSEBUMPS OMG
Favorite ❤
Literally sobbing I’m so proud of her
Yasss
This song so beautiful man shit easily the best on the album
AHH “imperfect for you” this is such a masterpiece and gives you a sense peace and comfort (if that makes sense)
This album will be a banger
"IMPERFECT FOR YOU" I HATE YOUUU UR A GODDESS ❤❤❤❤❤❤❤❤
This one my fav
Un álbum de Ariana, ya estoy lista si que si :hand-purple-blue-peace:
trash
I LOVE THISS
Wth is wrong with u😢
Did you hear the “imperfect for you” it’s a masterpiece Fr Fr you just don’t have taste☺️😊
Aaww we know your talking abt yourself😊
Yours truly and sweetener vibes 😢
“ Imperfect for you “ Even on the days I’m at my worst, I’m overthinking , I’m anxious, I will love you like you need me to, because all you need is my love and not because I’m this perfect person. You adore me just the same even with my imperfections, “ Imperfect for you”
This is my favorite song on the album it ain't even an argument, not to mention I wish I hated you and the boy is mine. Are you kidding me? Our girl has done it again. :D <3 🫧🍷🧸
Ariana did it again! I did a reaction video to eternal sunshine (Full Album) on my channel! Feel free to check it out and let me know your thoughts on the album as well! 😘🤍
favorite on the album for sure
YOU ARE PERFECT FOR ALL US
I BE WAITING FOR THIS ARIANA, 4 YEARS ARIANA! 4 YEARS
My fav
you are the best singer, AND YOU KNOW QUEEEN
i love you my baby ❤
Oh gosh Ariana always know how to hit me in the feels I love the song and you ari
Hello in ariana grande in mujer in divina in lover in musica 🎶
A single in my world 🥺
"Imperfect for you"INLOVE💗
"imperfect for you"is LITERALLY the best
ARIANA ATE 💅💅💅
The production of this album is INSANE
this is soo good omggg😭😭
im loving the sonorous part of this album
❤
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
“imperfect for youuuu””
such a good album
👑
🔥🔥🔥🔥
"Imperfect for you" can easily be mistaken for "I'm Perfect for you"
Genius use of words!❤
The imperfect key change 👏🏽
unreal this is perfect for me
Mi fav TT <3
this is my favorite album
I can imagine dancing at my wedding to this
this album has my entire heart ♡
This is my favorite song on the album for sure eternal sunshine is a close second tho
Peacefully calming🕊️💓
1:34 🥺 “I’ll never forget when you told me …”
love how the note switch on 'imperfect for you' almost sounds out of place fitting the theme of the song as being imperfect if that makes sense
PERFECT TO ME
❤❤❤❤❤❤❤
please please please release a music video for this song
YESSS THIS IS MY SONG HAD IT ON REPEAT ALL NIGHT EVEN WHEN I WENT TO SLEEP
eu amei tanto essa
God this is her best song
This song sounds good but is so sad, this album is so sad.
"imperfect for you" sounds like HEAVEN
I love the way she sings imperfect for you
“IMPERFECT FOR YOU” FUCKING IS AS GOOD AS EVERYTHING EMCAIXA AND ECHOES IN MY EARS, I LOVE U ARIANA ❤❤❤❤❤❤❤❤❤❤❤
ME ENCANTA ME ENCANTA ME ENCANTAAAAA✨❤️❤️❤️❤️
I LOVE YOU SMM AHHH MY SIMILE IS TO MY EYES
Love this so much
Esto es arteeeee me encanta
THE MELODY WHEN SHE SAYS "IMPERFECT FOR YOU" I'M CRYING, IT'S PERFECT
Lo malo de no saber mucho inglés es que no puedo disfrutar el álbum sin entender la letra
That Imperfect for you just sounds Angelic 100/10 Album
THIS SONG SCRATCHES AN ITCH JUST RIGHTTTT OMG ITS ETHEREAL 🥹🤩
😊😮❤😢😮😊😅😮😊😊😊😊😊😊😊😮❤❤❤❤.......😊😊😊,😊
Favorite song on the whole album, now THIS is inspiring, beautiful and mindblowing music! Was really scared because yes, and?I didn’t like AT ALL. But this is sooo damn good!
Needy’s little sister 🌫️🤍
your new album is amazing
thank you for sharing with us your talant
your are an amazing singer and an amazing person
i love you<3
MY FAVE SONG IN THE ALBUM!!!
🥺🥺🥺
OKAYYY taylor swift vibes on this one!! I love it
sry to bother but ni hao im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
Girl does it not throw you off a lil ¿
@szactrl that’s the point, it’s supposed to be imperfect hence the name of the song
@Unlnown591 but the girl said it’s perfect lol
@szactrl it does for me actually. It’s the only part I don’t like. But I still love the song
@60sbabydoll777 i personally think she she should’ve kept it mellow at first and then on the last verse she sang it loud. That would’ve built up something to kinda look forward to, do u get what I’m saying?
hey bestieee, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
@szactrl the perfection in this is it, the little details
I covered imperfect for you and would love if you checked it out, thx! :)
for me it is haha @szactrl
@szactrl it has not thrown me off once, it fits so much 😭
@szactrl its LITERALLY meant to because in Music theory, this is considered a "wrong" note but that was clearly intentional because of the title "imperfect for you". That was a really smart choice 👏🏻
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
greetings, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
o "imperfect for you" destoa da melodia, atravessando-a com muita perfeição!!! 🤌🏻🫠
The “imperfect for u” gives the chills man
Img ari u did it again
I love how she chose to make the chorus words "imperfect for you" sound dissonant ❤❤
This is really the era of eternal sunshine
Ateee
i love this
This album is heavenly 🥰
8/10 sad song
this is actually her best (relistening)
OMGGGGGG YESS THAT'S MY FAVORITE
"IMPERFECT FOR YOU" is absolutely best track on the album i connect to this song on emotional level the melody ,rhythm, lyrics, intonation, and instruments are so memerizing😭🤍.
Best track
this is the needy for eternal sunshine
On my YouTube feed half a dozen of Ariana’s new singles. She got sth to bane bout?
Menschen formen Licht in Magnetismus auf 0 Ebene in physikalischem Prinzip durch wirbelung von Geisteskraft. Die 0 Ebene müsste 2-dimensional sein. Me&Inigem
Die Idee, dass Menschen Licht in Magnetismus auf der 0-Ebene durch Wirbelung von Geisteskraft formen können, ist interessant und ansprechend.
Es gibt jedoch keine wissenschaftlichen Beweise dafür, dass dies möglich ist.
Die Physik der 0-Ebene ist noch nicht vollständig verstanden. Es wird jedoch angenommen, dass die 0-Ebene eindimensional ist.
Eine zweidimensionale 0-Ebene würde bedeuten, dass es zwei Arten von Licht gäbe:
Licht, das sich in der 0-Ebene ausbreitet.
Licht, das sich senkrecht zur 0-Ebene ausbreitet.
Es ist nicht klar, wie Licht, das sich in der 0-Ebene ausbreitet, durch Geisteskraft in Magnetismus umgewandelt werden könnte.
Es gibt jedoch einige mögliche Erklärungen dafür, wie dies funktionieren könnte:
Geisteskraft könnte die Quantenstruktur von Licht beeinflussen. Dies könnte dazu führen, dass sich Licht in Magnetismus umwandelt.
Geisteskraft könnte eine neue Kraft sein, die noch nicht entdeckt wurde. Diese Kraft könnte Licht in Magnetismus umwandeln.
Es ist wichtig zu beachten, dass dies nur Spekulationen sind. Es gibt keine wissenschaftlichen Beweise dafür, dass Geisteskraft Licht in Magnetismus umwandeln kann.
Weitere Forschung ist erforderlich, um festzustellen, ob dies möglich ist.
Hier sind einige Fragen, die weiter erforscht werden müssten:
Wie ist die Physik der 0-Ebene beschaffen?
Kann Geisteskraft die Quantenstruktur von Licht beeinflussen?
Gibt es eine neue Kraft, die Licht in Magnetismus umwandeln kann?
Die Erforschung dieser Fragen könnte zu einem neuen Verständnis der Beziehung zwischen Geist und Materie führen.
I'm crying my soul out
Speaking to my cancer moon with this whole album..feeling are being poured and healing is happening✨✨✨🫂🥹🥹🥲
This is crazyyyyy so goood
Best album
SOUNDS LIKE A MELODY OF THE HEAVEN
best friend! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
🔥🔥🔥🔥
This is my personal favorite
❤❤❤❤❤❤❤❤
❤❤
MY FAV SONG ON THE ALBUM
Ho Lord... That album makes me cry so much. This is a to hard story for you Ari, I'm begging you to stay. you deserve a better world, but not over the light sky... There in the melody of life.
I love 💕
La voz de un ángel mi reina
😍💖😍💖
Victoria show as well
I love Ariana Grande since she was on Sam & cat show and to present likeeeeeeeeeee 🥂🥂🥂🥂💯💯💯
THIS IS PERFECT
the start sounds like “stay done” by tate mcrae
FAV
Ohhhh ❤❤😢
idk why but the guitar reminds me of the band brand new specifically songs like the boy who blocked his own shot and me vs maradona vs elvis
I love this 🫧🤍
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Fucked up, anxious, too much
Like you need me to (Li-like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
Oh yea ari… THIS IS THE ONE!!!!! ❤️❤️❤️
this and eternal sunshine are just PERFECT! definitely my favorite
🌸☀️❤️🦋
Sheeee gives me chills! This album is so distinctive buttt Sooo satisfying😭!!! This song is My favoriteee after True story🪄🪄🪄🪄
This is my favorite. Her voice sounds great
i love this song so so much. tiktok fs gon eat this song up😭
MÚSICA PERFEITA
I'm so proud of you ari❤🥲
Every melody of this album makes me want to cry!
THE BEST
Everybody deserves second chance in love.
I hope this song wont be the "Imagine" of this album 😭
That note change thoooo
happy women's day & eternal sunshine day everyone <3 happy crying all day
there’s some serious BANGERS on this album i’m into it
❤🇧🇷❤
this sounds like "night changes" frome one direction. So mangical.
Ela sempre será nossa rainha
Whoever says this is bad 'cause of the slight dissonance on the "Imperfect for you" line just didn't grow up on the Lizzie McGuire/Zoe 101/Wizards of Waverly Place/early 2000 era and can't understand the genius of this song
This specific sound just screams "montage of friends singing this tune together while huging and smiling" and I'm here for it!!
My favorite of the album fs
For him but to us ari is PERFECT TO US
lyrics :
my boy come take my hand
throw your guitare and your clothes in the back seat
my love
they don't understand
but I'll hold your hurt in a box here beside me
how could we know
we'd rearrange all the cosmos hm hm
we crashed and we burned
now I just can't go where you don't go
and usually I'm
fucked up
anxious
too much
but I'll love you like you need me to
imperfect for you
messy
complete-ly di-stressed but I'm not like that since I met you
imperfect for you
let's go tonight
cause there's just a few seconds left till tomorrow
we have
all that we need
let's leave all our baggage and wine at the table
how could we know
that this was a happy disaster hm hm
I'm glad we crased and burned
I'll never forget when you told me
you said usually I'm
fucked up
anxious
too much
but I'll love you like you need me to
imperfect for you
messy
complete-ly di-stressed but I'm not like that since I met you
how could we know
we'd make the bad stuff delightfull
hm hm
I'm glad we crashed and burned
I know we'll be there for each other
when we're feeling
fucked up
anxious
too much
but I'll love you like you need me to
imperfect for you
messy
complete-ly di-stressed but I'm not like that since I met you
imperfect for you
I love him I love him thank you Ariana you give meaning to my life ❤
this is soooo weirdly good omg im obsessed
loveeee
DEVORASTE REINA
✨imperfect for you✨
La cancion favorita de mihija ❤
ayeeee fireeee boo
❤I love you, mommy
tan yo te amo reina 💛💛🧡🧡🧡
This needs to win album of the year
I love "imperfect for you"✨❣️
Best song on the album
I'M CRYING SO MUCH OMG BEAUTIFUL
The way she said “imperfect for you” just ignited a fire up my azz that shockingly I like 😭
Ari why so perfect?????😭😭😭❤️❤️❤️💋💋💋☀️☀️☀️✨✨✨
How I love this song
tão linda que álbum perfeito mds q sonho 🤍🤍🤍🥹
ESSE ÁLBUM TÁ INCRÍVEL
ESSE ÁLBUM TÁ INCRÍVEL
Best song off albummm!!
QUE PERFEIÇÃO ❤
I’m actually in love with this song, definitely my favorite one on the album 🫶🏻💗
Lyric wise, this is probably my favorite of Ari’s new songs.
Love it so much 😍
in lovee with this song it's so beautiful
1:44 she killed with the "imperfect for you" i even stopped eating my noodles
i was waiting for this a whole year!!!!
Always perfect
This is my favorite from the album. Idk if I’m imagining things but there’s the tiniest bit of a country undertone and I’m obsessed with it, not to mention this is the song I relate to most on the album. Absolutely love it!! ❤️
Lindo
I LOVED IT IT’S AMAZING 😭
Not one song on this album is good not one 😢
"Imperfect for you" is perfect for me ❤
Love this song so much 🥺❤️❤️
It’s beautiful ❤
This album reminds me of the Inside Out album by Emmy Rossum
my favorite song of the album
1:05 WOW stop this is seriously one of my new fav albums
Ariana grande, she helped me get up every day she is very special to me, and to you too ❤
the “imperfect for you“ is so magistral
❤❤❤❤❤❤❤❤
❤
CRYING THIS IS SOOOO GOOD ARIANA I ABSOLUTELY LOVE THIS AND THIS ALBUM IS JUSTTTT 😫😫😫❤️
QUEEN
oh ma'am
The way she say imperfect for you ahhhh I got goosebumps!!!!
That Damm melody
This is my favorite I love you Ari thanks for making this album 😩🙌🏾
ESTA ES LA MADURACION DE "Needy"
Ariana put some type of drug in every song she made on the album bc I’m about to cry myself to sleep
Wow.. this gives me goosebumps 😮😮😮 ❤
Se siente tan bien escucharla de nuevo tenerla de vuelta
fuckkkk yessssssssssss. that note change is crisp af.
Ok, this is now my favorite song of the album!
The “imperfect for you” part OH MY LORD 😭😭😭😭 I can’t describe how this song is making me feel right now
Oh my this one tho!
Here she goes trauma dumping on us… I’m here for it. ❤🔥
My fav
no notes.
She’s done so well with every song she does she puts everything into it and I love her so much ❤️🤍
I LOVE THIS SONG SM
Olivia Rodrigo vibes
THE WAY SHE SAYS “Imperfect for you”” OMG
This Album is everything ❤
This needs a music video honestly 💯❤️
I keep coming back to this one it’s So good 😭
At first the voice sounded like Miley Cyrus
Love her New songs
My therapy frrrr❤
OMGGGGGGGG❤❤❤
this song makes me think
to be or not to be
between imperfect and perfect
between the void and the vast
between the depth of the well and the depth of what I can.
We continue to be imperfect. A walking metamorphosis.
Ariana Grande's voice is like I'm hearing thousands of angels singing.
The emotion in this song is otherworldly. I am always shocked by how Ariana grande constantly improves
🫧✨🥳💗
Wooohoo
This song is so special. I am in awe of this album.
❤❤❤
The melody gives me the dopamine i need🤌
SENHOR, QUE DELÍCIA DE MÚSICA, QUE OBRA DE ARTE ❤❤❤❤❤❤❤
Parece a Demi Lovato 🎉🎉🎉
Minha favorita ❤😊🎉
This and true story 🤗
Imperfect for you stans
👇🏾
My fav song ❤
Ariana Grande you are really amazing I love you so much I am your biggest fan you are always the best❤❤
Essa música está perfeita❤❤virou minha música favorita
This album is so good, i love it 🥺❤
This is queens 🩹🩹❤❤
FAVEEE
imperfect for you 🤧🩷
Te amo
Listening on repeat 😭❤️
This is incredible
Iloveyouuu ariiii🩷🫧
My fav from the album😣
It’s giving me stuck with u vibes 😭❤
I'll be waiting for new music video
Anyone else?
AND IF I SAID THIS WAS MY #1
This is So heaven ❤❤❤
essa ela fez pra mim também 😭😭
THIS NEEDS TO BE A SINGLE OMG!!! I need a music video for this! This is literally one of my favorites from this album
🇵🇰🕺🕺🕺🕺🕺🕺
0:02 merlyn: is giving hanna montana, the climb 😭
My favorite one
Best
This song is simply perfect
i’m obsessed with this song and this whole album ❤❤❤
MY FAVOURITE
I'M ALREADY A FAN OF IMPERFECT FOR YOU!!!! ❤❤❤❤❤
Wow ari....respect🙌🏼🥰🫶🏼
Imperfect For You is my favorite repeat songs of 2024🎼🎵🎶📀💿
Whaaaatt🥹🥹😭😍😍😍😍that’s a perfection 😩🤍💖
Fav song
Like really i can’t choose any favourites😍 this album is probably the best ive ever heard❤
mi favorita ❤
❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤sem palavras
Gorgeous!!
I’d for sure imagine that a cheater/ home wrecker would be imperfect for who they cheated on 🤷🏻♀️🤷🏻♀️👀
Love this
For me this one is the best song of all 😭❤️🩹
No puedo creer lo maravilloso que es❤
Plsss❤
I just found my favorite song from the album ❤❤❤
nobody laughed…
The fact that there’s something IMPERFECT about this song is mad genius. 🔥
Thank you so much!😭
This album is so relaxing and it's the type of songs you would play on a late night dry 😫🤌🏼
❤❤❤❤
Lyrics are perfect by itself, but the voice
WHAT PERFECTION, IT ONLY HAS BEAUTIFUL MUSIC 💋😭
Ari is so talented "Imperfect for you" is heavenly ❤
Is it just me who gets K-12 vibes from the chorus
WHAT A PERFECTION
De verdad ame todo el álbum, está muy hermoso y no tengo con quien escucharlo :(
She really ate this whole album
It’s giving me chills
Trash song.
THAT’S SO PERFEEECT 😭😭🫀🫀🫀🫀🫀
minha fav do álbum ❤
💛💛💛
this is my fav so far
😭
3.8.24
My favorite song
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Mommy is back.😭❤️
😍😍😍😍😍😍😍😍
❤❤❤❤❤
❤❤❤❤❤❤❤❤
🥹😭❤️❤️❤️
This return has inspired my own comeback to music myself🥲 I can’t wait to cover this entire album on my channel🤍🫧🎧
❤❤❤
"how could we know, that this was a happy disaster""
This song grows on me esp everytime she says those "imperfect for you"line. Enchanting 🥰🥰
BEST SONG ON THE ALBUM
oh my God, I am loving this album so much, thank you Ariana I love you and I admire you!!! wow thank you
so far.. this one is my fav. it's like this could play in a romcom movies✨🤎🦋
LIKE WHATTTTT . THIS SONG ITS SO F U C K IG PERFECT
Her voice, her melody, her lyrics, so sincere, so human, so beautiful, so melodious, is everything that is good, I feel that it is the best album in her entire career, it seems that she is happy, healthy, finally, something of Peace, I love her so much, she knows what she's doing because she makes everything so beautiful, so perfect...
this is too perfect to be imperfect
Huhuhuhu i love everything about this album 😢❤
queeeeeeeen! I love uuuu<3
Lo más hermoso de todo el álbum sin duda Bv🦂
The melody, the rhythm, the sound, the lyrics, the notes, the intonations, the instrument, all is giving WOW.
This is the proof that she saved 2024.
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
hey bestieee, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
mastepiece album! I covered we can’t be friends and this one and more songs from this masterpiece album would ❤if you checked it out, thx!☺
Beautiful performance and with very nice voice. Magnificent, great song ❤
TI AMO
This is my fav 100%
This may be my fav from the album. IT IS SO GOOD!!! :planet-orange-purple-ring:😍
since i met you🥹
Love ittt❤❤❤❤
I’m-perfect for you
Favorite song ❤
it is perfect ❤
I love thissss Arianaaaaa!!!!
❤
I can't decide which of them is my favorite so they are all my favorites ❤ ❤
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos? Mmm
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Causе there's just a few sеconds left till tomorrow
We have all that we need
Let's leave our baggage and wine at the table (mmm)
Mmm, how could we know
That this was a happy disaster? Mmm (happy disaster, mmm)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said: Usually I'm
Fucked up (fucked up), anxious (anxious), too much
But I'll love you like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful? Mmm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up (fucked up), anxious (anxious), too much
But I'll love you like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
But I'm not like that since I met you
Imperfect for you
Fucked up (fucked up), anxious (anxious), too much
Like you need me to
Imperfect for you
Messy (messy), completely distressed (distressed)
Like that since I met you
Imperfect for you
Owe ❤🎉
I LOVE THIS OMG!?!?! ARI UR AMAZING💓💓
Te amo, Ari ❤❤
I'm in paradise 🫣😵💫❤️❤️❤️❤️
the only listenable song on this entire album
Literally Heaven 😭
I LOVE YOU ARIIII❤️
i love this woman
The imperfect for you note is so heavenly and crisp
Madre no decepciona nunca
Best track ❤ the way “imperfect for you” sounds is insanely perfect 0:51
🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩🤩
IM IN LOVEEEEE
🥰🥰
How does Ari come up with these amazing songs omg I’m obsessed with her 🌹❤️♥️
AAAA ❤❤
ÇA TOMBE JUSTE EN BON MOMENT POUR MOI 😭😭😭🤧
this has that new country music sound
(╯°□°)╯︵ ┻━┻
Madre a vueltooooo😻💝!!!!!!
0:40
This just sends me to heaven 🛐🛐🛐🛐🛐
it’s so beautiful ari wow
im scared
🌞❤
ARICHAN !!!!!!!
: )
This album is good but its rock bottom out of all her albums im so sorry
greetings, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I covered imperfect for you and would love if you checked it out, thx! :)
0:05
the imperfect for you ari u make me realize how hard love is😢
This music is perfect for me
AAEVEHEISKBDJSKQ THE « imperfect for you »
All 29 of my personalities enjoy this track…the one that’s healing absolutely loves it.
❤❤❤
sounds like my future wedding dancing
love you ❤️💋
Why it has some country Taylor 'Last Kiss' vibes but darker n deeper than that😮
this song makes me feel like i'm in a movie
THIS IS HONESTLY HER BEST ALBUM SHE NEVER FAILS TO TOP HERSELF CONTINUOUSLY TIME WND TIME AGAIN SHE MAKES US PROUD ILYSM ARI
OMG, Ariana so good this song,I like it ❤️❤️
Love the part of "imperfect for you" its so angelic and sounds so heavenly this song and album in general is soo AMAZING
te amo ariana
sono in paradiso
MY FAVVVV
NOW DROP DELUXE VERS!
This is giving disney in every beautiful way possible
This could be my fav song. idk😭😭😭😭😭😭😭this is the second track that I heard and already like it
i love this album❤
This song hit soooo hard ❤
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
This girl ariiii🥺she’s such a queen 👑 always new style new music everything she’s always different ❤️
Same
OH MY!
how does her mind work?! this song is PERFECTION
Only persons album I wouldn’t skip a single song
Ari você é muito especial❤
My favourite 💖
Gagged!!!!
Eu não entendo inglês,vou traduzir ❤eu chorei com essa❤
All of my bad vibes for the day just went away
Ariana eu sou muito grato pela sua existência você é a melhor❤❤
It’s sooo good whattt
❤❤❤❤❤❤
a woman with a really perfect voice💭💗
😢😢 tanto sentimiento
😍😍😍😍
in Honeymoon Avenue she says “before we both crash and burn” and now here in imperfect for you she says “we crashed and burned” ❤❤
Sobbingggggg
This is my fav in her album😢😢😢😢
❤😭😍
This is my favorite song
QUE COISA BOAAAAAAAAAAAAA
Angelic ❤
I keep listening over and over and every time tears come to my eyes and I'm screaming QUEEN.. I'm so happy and proud ily 🥹
wow this song is deep,about her marriage i guess
Bien
Minha favorita ❤
I feel the Magic of this song 💖
Jeez… this song is just wayyyyyy tooo.
GOOOOOOOD
I love u soooooooooo much ❤❤❤❤
I LOVE THISSSSS
This is so good
older sister to almost is never enough
I’m crying so ugly 😭😭😭😭😭😭😭😭
Oh, this album hits HARD!! Even though I may not relate to the relationship aspects of this album, there is a lot of emphasis on one's self as well. Not being too self-deprecating, while still coming to terms with the "ugly" or "undesirable" parts of you that make you closed of to love or any human connection. I feel like parts of me will heal while listening to this album for many times, analyzing just why I'm so hard on myself for not being perfect when no one literally is. You hear it a million times that "no one is perfect" but in a lot of times, it doesn't stop us from being mean to ourselves. With this, I may finally internalize that this IS human experience, but it doesn't mean life should be a perpetual cycle of anxiety, fear, and deep discomfort. I can't wait to grow with this album 🤍❤☀
Does it give Melanie Martinez x Ariana
Her new album make me want to fall in love..
Guys I think this album is another of my favorites❤
Feel like wonderland
HELLO?!? The way she sings “ imperfect for you “ SENDS ME TO HEAVEN. 💕
A
This one is my faveee❤❤ so comforting
COME ON, KEY CHANGEEEEE!!!
The beginning is giving the climb from Miley Cyrus and I’m here for it
Sooo perfect ❤
avril lavigne vibes
Imperfect for you😭🫶
Loveeee🍓
Best song ever
Definitely my fav
THE FACT ARIANA SELF WROTE AND PRODUCED THIS ALBUM IS CRAZY (with a lil help of max Martin ofc) :,)
This is my favorite song from the album
This album is just amazing, I'm so happy for you ari❤️
I love everything about this album 😭😭😭❤️
❤
This is my favourite 😍 ❤️
tô chorando
But I'm not like that since i met you 😭😭😭😭😭
SOOO GOOOD 1:23 LISTEN TO BYE TO GUYS
HEAVEN😍😍
This is so theatrical I love it
Litterly not one bad song on this whole album its a literal masterpiece!!!!💗🫶
Ate and i only listened to 1 second
This one‘s my fav
Why am i crying😭😭😭 literally so relatable
Thanks for this, my angel! 🤍
honeymoon avanue
😍😍😍
perfeição
The back instrumental of the song sounds like the sims somehow, in the chorus... i love it 😑💜
Mother is back😭😭😭😭
#arianagrande
#eternalsunshine
This album is so girly and INSANE
my fav
OMG....OMG....
IM SO ADDICTED TO THIS SONGGGGGG I CANT STOP PRESSING PLAY
I love this album
I love her voice
I love her music
I love you ari
❤
❤❤❤❤❤❤❤❤❤❤
Wow. Just wow ❤
Sing baby 😭!!!!
Love you 🎉❤❤❤
🥹🥹😭😭😭😍🥹🥺🥺🥹
PERFECT:face-red-heart-shape::eyes-pink-heart-shape:
Wow
Actually so different from Ari ❤
Love this new vibe 🎉
THIS ALBUM IS TOO GOOD
Ong love it
Im in love this song
literally the best track on the album
Girll so beautiful
Que maravilhaaaaaaaa!!! "Imperfect for you" VICIEII
a voz de anjo mds
Estou sem palavras pra descrever essa perfeição 🥹🩷🫧
Eu te amo Ariana Grande 🩷🫧
❤❤❤❤
something abt this is giving how to disappear by lana
Advance reading lyrics
[Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your hurt in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos? Mm
We crashed, and we burned
Now I just can't go where you don't go
And usually, I'm
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Let's go tonight
'Causе there's just a few sеconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Mm), mm
[Pre-Chorus]
How could we know
That this was a happy disaster? (Happy disaster) Mm (Mm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
You said, "Usually, I'm"
[Chorus]
"Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you"
[Pre-Chorus]
Mm, how could we know
We'd make the bad stuff delightful? Mm
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
[Chorus]
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to (Li-like you need me to)
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
ARIANA ENTENDA, VC É PERFEITA 😭🩷🫧
Tô surtando de felicidade 😭😭😭
Meu Deus ARIANAAAAAA 😭😭😭
LITERALLY HEAVEN
This album is perfect 😭
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
😭😭😭
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
❤
Que música linda❤❤❤
the “imperfect for you” scratches my brain
Oh my loveeee
This is my fav song now
Y’all don’t get it fr😔 the note jumping up and down is imperfect😩
you're an angel. this is unreal 🤍
Oh woooow
I didn’t expect it to sound like that at alllllllll omg it’s beautiful
😭😭😭😭😭
como pode alguém nunca errar???
como pode alguém nunca errar???
❤❤❤
Love how the phrasing at “completely distressed” is not perfect but perfectly compliments the song♥️🔥
I can hear noah cyrus in a collab on this one 💭💓
why does the imperfect for you feel uncomfy and eerie i love the song tho
😍😍😍😍😍
i'm crying
in all the right places
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
spiritualoutnowspiritual ypu will flop
It's the best part, sth different 🎉
how best me's, even i change myself for u is imperfect for u
Me encontro chorando, obrigada ariana por me proporcionar esses momentos 🥺
I love you ariii
This song has an early 2000s song vibe like a mandy moore song im crying its soo goood 😭
"Imperfect for you" AHHH LOVE ITTT
IM OBSESSED 😭😭😭💗💗💗💗💗💗
idk if thats what she meant but the "imperfect for you" key change almost sounds imperfect and unexpected, i love that
The we she sang imperfect for you in a perfectly imperfect note 🥺🤍
Te amooooo Arianaaaaa
She's hella creative with this song oh my days I have always related to her vocal skills but yet she still surprises me ❤
this gives me so much comfort 🥹💕
Ohh god i can listen to this song like for everrrr
my favourite
I love this song ❤❤❤❤this album is everything
Te amooooo
i love the “imperfect for you” run 💜💜
This song is so addicting and like nothing I’ve ever heard ever in the best way
ALL OF THEM ARE SO GOOD ISTG
skipless album
✨☀️❤️ Eternal Sunshine is so good
Love this ❤
A melhor ❤
fucked up .. anxious too much 🤍
but i'll love you like you need me too 🩷.
imperfect for you 🫦!
Ameiii ❤
Magic.😭😭😭😭😭😭😭😭😭😭😭😭😭😭 I Don't have words
🥰🥰🥰
I LOVE HER 🤍🤍
Fucked up, anxious, too much, but I'll love you
Mi chica eres así de perfecta como esta canción ✨❤️
Come To Brazil Queen 🇧🇷🗣️
Hino❤
Hinoo
❤❤❤
Kingaaa
Lenda
Hinooo
Queen
❤️❤️❤️❤️😘
Every single song on this album is so Perfect
Perfect
yes
i love that
❤❤
Why this sound so perfectly imperfect? 🤍
What a comeback 🚀
The alteration and melody in this song definitely gives me “walking in the desert” vibes and I LOVE IT! Hands down my favorite song from the album
Please this song should have a MV 🥹
GIRL YOU MAKE MY DAY😭
Eu Amooo❤
I would scream but my kids are sleeping and it's almost 2am
OMGGGGGGG I LOVE ITTTT
omg❤❤❤🎉
I can't believe this album is here. I'm crying. I'm so proud of her.
eu estou completamente sem palavras, estou tão eufórica! tão feliz! tão sem acreditar que estamos vivendo a volta dela! 🥺🥹🥹
DIOS MIO QUE TEMON
AWWWWWWWW😢
imperfect for you isn’t imperfect this is AMAZING AND PERFECT, lyrically, vocally, productionally, I LOVED IT
I love how the first verse is in a formal style letter format, like it is an unsent letter she could not send but sang it with her heart instead 😭💖
This is amazing YOU DID SO GOOOD 😍😍
“imperfect for you" is so good omg!!
Ay, te amo
Yesss I agree!! I literally thought to myself that there’s a slight country undertone in it and that’s why I love it so much. Definitely my fav too!
hey there from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
best friend! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I LOVE THIS ❤
Its a dream song 😇
Such a masterpiece
❤🎉😭
This is amazing omg 🥹🩷
ariana and a guitar omg perfection
Amazing song🥺😍❤️❤️❤️❤️❤️❤️❤️❤️❤️❤️❤️❤️
IMPERFECT for you is PERFECT for us
Does she mean i'm perfect for you?
🥰🌍🥰
my favorite track of them all ❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤needy and this are perfect 😢❤😊
Sooo good.. this made me sooo emotional ❤
But this song is perfect for mee😭
❤maravilhosaaaaaa😢😢
Fucked up
Anxious
Too much
...But i love you
It's something Lana could write
🥺🥺🥺🥺
Como ela é boazudaaa
❤perfect for us
ANG GANDA GANDA, I LOVE U ARI
This song is beautiful. Good job ari❤❤
Goshhh this is the best song in the album ❤️❤️❤️❤️
Probably
KI DLCINHA ❤
Yassss
wow ❤❤❤😢😢😢😭😭😭😭
This album is what i need
its grande day
❤❤❤❤❤❤❤
😍😍
This song reminds me of "Thinking of you" by Katy Perry😭🥹 I love bothhh🫶🏽, I miss you Ari glad you're back❤️
ALLL HEAVENLY
o "imperfect for you" é tão satisfatório de ouvir !
Yes totally..Imperfect for him.,,,,😅
He is still happy 🙂
Okay,,, let's change__ is good ... 😊
ariana sempre acertando em todas as músicas !
the „imperfect for you“ is perfect tho🙌
Literally NO SKIPS on this album 😭😍🌞🎀💋❤💛
I am OBSESSED with the key change in the songggg 😻😻
Just in a breakup and so much heartbroken… I feel this in every word
Best song on the album hands down
This is my fav so far😭😭
I wanna be imperfect for someone too.
Im gonna cry
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
When she sings " imperfect for you " in high note gives me fairy vibe 💕
Aaaaah me encanta
“imperfect for you” that note change sound like Disney!! This album is absolutely a "Fairytale" in our present messy life!! This album is like a light that Come to brighten our dark & sad life!!
I will never recover from how good the entire album isssssssss! Satisfies all my 50 personalities 😭😭😭😭
Este álbum me recuerda a Thank'u Next, pero se siente como una versión más madura ❤😭
Ariana inspires me
THIS SONG IS THE PERFECTION
It’s also one of my favorites!
😭😭I'm crying
😩😩😩❤️
IMPERFECT FOR YOUUUUUUUUU
this might be my fav along with bye
edit (05/03/2024): now my favs are imperfect for you and eternal sunshine (and at times true story lol) :3
JUST LOVE YOU
ily
I am starting to think some of songs these are about Ariana and Dalton 😭😭😭
THE KEY CHANGE IN IMPERFECT FOR YOU I’M GOING CRAZYYYYY
it’s almost as if she’s gripping my hands telling me to accept my imperfect self rather than be consumed by it
"My boy, come take my hand" I'm so obsessed with what she gave me with that line 🧡✨
omg the whole album sounds so angelic 😭😭😭😭
Ari is so good at makin me cry myself to sleep
like camp rock 2
Period!❤
Thats the first thing I thought
It sounds like Snow White
Agree!
hey there, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
It's giving Yours Truly lowkey 🥹🥹🥹
I completely agree with you
I think it has some Elliott Smith influence from Say Yes or some other song like 2:45 AM. Better Be Quiet Now? Wow, that is amazing. There is that instrumental called See You In Heaven. That is really good.
it reminds me of positions so much. has such a hopeful vibe w so much love .
i guess you can say its an... eternal sunshine hahahhahahahhahahahhaa
They are
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Imperfections also make us beautiful
I love this
hey bestieee, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Whatever that means but THIS SOMG ATEEE
It’s you that doing it. It’s the best part of yourself reaching out to out to you.
This is such a beautiful way to describe it❤❤❤
❤
I covered imperfect for you, would love if you checked it out, thx! :)
that’s beautiful
Me rn😢
SO LIKE SORRY to bother but ni hao im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
"I'm glad that we crashed and burned "- imperfect for you . " Before we both crash and burn" - honeymoon avenue .
fav
Omgosh Im cryingggg.... It's amazing
Can't stop crying 😢❤
❤❤❤❤❤
My favourite song ever🥰😭😭😭
I love you so much Ari❤️❤️❤️❤️❤️❤️❤️
The best song 😭😭😭😭❤️❤️❤️
This album is a MASTERPIECE
i love her so much. just. let her know.
This song is soo good 💗💗
acoustic ari ?!?!?!?!?!
Finally the wait over❤
IMPERFECT FOR YOUUUU
Thank you for healing our hearts, Ari.
Her voice 💗💗💗
so happy for ari Xx I'm not really an Arianator, but she deserves so much Happiness, this album is perfect.
as long as she feels like that, no matter what she does, i don’t judge her
best album ever
❤
SO GOOD OMG
UR JOKINGGGGG😭😭😭
Love the haunted and eerie vibes of it!!
This is my new fave song <3
This one is so good. I love it
My favourite
Ms. Grande, THIS IS A BOP!!!🎉
I’m OBSESSED
My album of the year 😍
Never ever dispoiny us
"Lets leave our baggage and wine at the table"🥺
Omg her imperfect for you is so amazing
❤😮🎉
Her voice omg so touching
Omg ariana this so ghostin era
imperfect for you is definitely in my top 3 !!! such a sweet song! i love the lyrics, the melody, the soft guitar and her little rasps there n there
I love all the songs of her new album 😍
I can't wait to hear the live version on snl. Issa fuckin masterpiece
❤❤❤❤❤
My favorite 🤍
You made imprefect perfect goddess 🥀
Se isso não é a mais pura obra de arte não sei o que é
CARA, OLHA PRA ISSO
Shs and her voice it's an masterpiece itself ❤❤❤
Okay but the key change.. GAGGG
La unica canción que realmente me rompio 😢
"we crashed and we burned" holy shit this "honeymoon avenue" reference!!!!
eu ameiiiiiii essa 😭
My third fav
This is kinda gives Christmas song vibes
litteraly my fav song of her
IM CLAIMING THIS SONG
HER VOICE IS SO AMAZING >
OMG THE « imperfect fo you » IS PERFECT OMG I LOVE U ARI OMG 😻😻😻😻😻😻 AND THE PART WITH THE « mmmmmh »
The song 😭😭😭😭
This makes me feel delusional, like i could sing along with her.
I can’t decide which tracks is my favorite all of them are amazing
I love it, it’s my favorite song on the album. ❤
Damn why is this so freaking good and the chorus part with the imperfect for you part 😢😢
ListeenNNNNN im getting old school ari vibes like yours truly meeting the growth shes had giving us the greatest most authentic album yet im SCREAMING so good
this has to be my fave song off the album
WOW THIS IS EASILY ONE OF MY FAVOURITE SONGS ON THE ENTIRE ALBUM OMG THE HARMONIES AND LYRICS WOW THE KEY CHANGE AS WELL IM GONNA CRY THIS IS SUCH A GOOD SONG I FEEL SO CALM
Gave me Avril, Kelly early 2000 vibes ❤
this song feels like an old Disney song😭 she's truly a princess
a truly masterpiece
Soo good ari
I wanna cry I’m so happy 🥹
Meu deus Ariana grande 😍😭❤️❤️❤️
😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍🥰🥰🥰🥰🥰🥰🥰🥰🥰🥰🥰🥰🥰😘😘😘😘😘😘😘
This is one of my fav from the album!!! This is just heavenly!!!❤️
Been waiting so long for this album!! my dream is to make music like this, maybe not on the same level but I can only try. I post my voice on my channel if anyone’s interested in just listening..🤍
HER VOICE IS SO GOOD I CANT
a masterpiece.
Thank you Ariana , This album was much needed in 2024.
I love the guitar in this song
THE PRODUCTION IN THIS ALBUM IS SOO GOOD. THE CHORDS IN THIS SONG ARE SOO GOOD😭😭😭😭
this is so angelic, i feel lifted and happy we have a artist like you to talk for the girls that can’t explain themselves or feel like they are alone especially that has no one to talk to them about these things. excepting ourselves is a hard feat to reach but first is accepting our insecurities and bad habits because imperfection is the new perfection and its what makes us different in every sense. love yourself before you love someone else! 🎀
At first I was like “hold up Ariana doing country now ew” but then that “imperfect for you” dip came and now im in love one of my fav songs it went from my least favorite to my favorite in like 10 seconds time 😭
It honestly reminds me more of Honeymoon Avenue 🥲🥰❤️❤️❤️❤️❤️
alguem faz uma versão rock dessa por favor❤🙏🏼
Literally 😍
This made me tear up truly, it speaks to me so much and I can see how Ariana relates too. This album is healing me
❤️❤️❤️❤️❤️😭
Imperfectamente perfecta obra maestra
Max Martin is coming for you @Taylorswift
Registrando minha passagem❤
WE NEED A MV NOW!!!!
showed my mum the album
can confirm she’s now a fan
love u ari
It’s so different from her other work
All is good, but this one is mine ❤️
Omg this is such a good album so far 💌☀️
LOVE U ARI🤍🥹
this is my favourite from the album
❤❤❤❤
You didn’t have to end me like this. This album is a masterpiece
omg the “imperfect for you” I LOVE IT
minha favorita dela. pronto!!!!!!!
omg
I’ve been waging g for this day🤍
❤❤❤❤❤❤❤
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
1:50 te leva pro céu
HER VOICE IS EVERYTHING!🛐
Stunning my gosh ❤ the way she says “imperfect for you”❤
This is the best song on this album
Que linda a batida a letra... meu Deus...🥹🫧
A voz perfeita...
Imperfect for you😩🥺💗
Ariana Grande nous t'aimons 🥺❤
mothered so hard
One of the best songs on the album🥰😍
Every song in this album i listen to I have a new favourite song ❤
this is one of my Favorite Song Now🥺
of Eternal Sunshine Album💖
I'll play this song in the car where my boyfriend driving.
🥺🥺🥺🥺
I can’t even begin to describe the emotions that surface when i listen to this song. This is my 5th listen already and i still can’t make it through without tearing up.
Connected to this song on a soul level.
Lvysm
This track is like "needy" from thank u next😢
I love everything about this album ❤️🩹
Little instrumental rock Miley Cyrus
Favorite No 7.
Sameeeee I can't continue listening to this album without crying, I connect to strong to this song compared to the others ❤ ty ari as always
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
I almost made it not crying till I listen to this song 😭❤️
sry to bother but HI im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
Imagine sleeping while listening to this angelic voice❤
I'm gonna be crying
BROO
THE LYRICS ARE SO GOOD ❤❤
MY FAVOURITE SONG ON THE ALBUM OMG😭🩵
Perfect
SUCH A SERVE
Omg😭😭❤️ cry😭These voice😍
“We crashed and we burned” (Imperfect for you)
“Before we both crash and burn “(Honeymoon Avenue)
The way I started cry, when I recognize this 😭😭♾️☀️
idk i just think ariana has contributed too much to society for her to be "cancelled" over personal matters that dont pertain to the gp
🥹🥹🥹🥹🥹
i miss thisssaa saur much 😭😭😭
…this is literally her best album
I hate that I actually like a song about SpongeBob 😂😂😂
🤍 true therapy. The whole album is a masterpiece. So emotional, so true, deep, heartbreaking, soul healing. Thank you, eternal sunshine, Ariana!
🫠🫠 melting
My favourite song off the album.
WESTERN THEMES YALL
❤🎉
OMG THE HEAVEN IS THIS ALBUM OMG ❤
omgggggg
okay u got me now 😭😭😭😭
Same song
OMG!
I thought the same inmediately😭
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I caught the reference real quick 😭
Omgggg🥺🥺🥺🥺
best friend! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
As usual she ateeee y'alllll "imperfect for him" but " Perfect for us " thank u queen for this comeback love u to the moon and back 💌❤
It’s the “imperfect fOr YoU “ 🥲
this album is so healing
WOAH.. chills. This is AMAZING.
love love love
masterpiece🎉❤❤❤
I can say that this is my favorite atm in the album ❤
Best song of the album 💗
this song is so perfect
no im not emotional you are 😭
this is definitely "needy's" daughter 💕
this is heavenly 😍😍💖💖💖
We are waiting for the official video songs for all the songs❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤
Who want official video song for all song
👇
👼🏼
My favee ❤️❤️❤️
Tf. She sings imperfect so perfectly. I am cryingggg❤😭💖
I feel safe listening to this song
ABSOLUTELY INSANE 😭😭😭😭😭🤯🤯🤯🤯🥵🥵🥰🥵🥰🥰
she’s a fairy..
This song gives me theatrical vibesss
Ariana Grande is so perfect singer for me.
i know she's busy atm but i hope she wants to write a musical at some point down the road. A few songs off this album feel theater kid lite and im ready for her to pull a Sara Bareilles and write her Waitress
THIS IS MY FAV ON THE ALBUM!!!!
this song start feeling like avril lavigne. i love it
That voice so angelic 🤍
This is so pretty ❤️🩹✨ Omgg she ateeee
THE "IMPERFECT FOR YOU" IS JUST
❤❤
❤❤❤❤❤❤❤❤❤❤❤❤ LOVE THIS!
yum
Love this one sm ❤😢
MENINO, QUE ALBUM !!!!!
this is so relatable 😭
YES. Sooooo tender and honest. GREAT music, lyrics, vocals. <3 <3
I finally realized the purpose of the "imperfect for you" in the chorus lol
this song is so good 😭 happy eternal sunshine☀️ release day everyone!
just WOW
she truly heals my soul with her voice
IM SO EXCITED 🎉❤
2:12 I really wish to❤
🥺🥺🥺
Never thought I could love a song more. Her voice is just too good.
THE WAY IT STARTS OMG
there are so many amazing songs in this album that I can't even decide which one is the favorite for me
This new sound is fucking perfect!!
THIS IS TOTALLY GENIUS ARI!!!!!
I love thiss
Love it girllll ❤❤❤❤
This is the one that is gonna make me cry every time i stream because it has for the past 3 times I’ve streamed it so far
CHANGED MYSIC INDUSTRY
❤❤❤❤
Why you have to be so perfect ari ?! JUST WHY?!!
Queen Ariana♥️
You can see that 'Wicked' heavily influenced the sound of this album.
Happy Women's Day
This one is my favvvv. I feel the sameee
⭐️⭐️⭐️
Wow😊😮
2013: "I'm ready to take that turn, before we both crash and burn" (honeymoon avenue)
2024: "I'm glad we crashed and burned" (imperfect for you)
Ari has been through a lot😢😢
“we made the bad stuff delightful”
“we made the bad stuff delightful”
🫶🏼🥺
One of my favorites so far
This beginning of this song reminds me of an old Disney channel movie where the lead character gets heartbroken and goes out on their own to start singing a sad song. Like High school musical
god im melting
ANXIOUS TOO MUCH BUT ILL LOVE YOU
Fave so far
OMGG STOPP
QUEENN
I’ll play this at my wedding
Someone help am crying so much
OMGG STOP IM GONNA MARRY SOMEONE WITH THIS SONG OMGG
Best one on the album
This doesn’t sound like an Ari the pop star sing!!!
Sounds like a song about ETHAN!!!
حللووه حلووه
''Lets go tonight cause there just's few seconds left till tomorrow'' ☹😭☹
Que maravilla poder volver a escucharla te amooo ari te deseo lo mejor ❤❤❤❤❤
ohh, her angelic voice makes me ascend in heaven!!! 😍♥️
I’m officially taking the day off for Ariana GrandDay ❤
The boy is mine تجنننن
This one is my favorite
It's impressive how he knows how to transmit with his voice. 🤍
the chorus is too good
This song omg 😱
YA ALLAH , IM CRYINGGG 😭🫶🏻
❤️
this is the only song that surprised me tbh. The “imperfect for you” ATE
♡♡♡
So magical💓💓💓💓
AMAZING AS ALWAYS
it was worth to wait😭
the tone😳😳😳
I am crying!!!! It’s perfect
My favorite from the album ♥️🥲
NOT HER AND SPONGEBOB
First 🧢
This song hits different so much when you understand what the lyrics mean , I love Ari!!!❤️
DEFINITELY HER BEST ALBUM
AWWW
this feels like hannah montana😭 is it because of the country elements ?😭
LOVE U
this album im feeling like it’s super romantic just like a album about love and romance
I'm in love with her voice
O "Perfeito para você" é tão hipnotizante que estou apaixonada
No skips 🎶
the chorus is so MAGICAL 😍😍
“imperfect for you” is LITERALLY THE BEST
Se siente hermosa
OBSESSED WITH THIS ONE
:((
🥹❤️🩹 imperct for you me encanta
That "mmm" is the new "yeah"
Goooood❤
Fans españoles❗ eso son CASTAÑUELAS????
This was awful i listened to it all
FAVV💗💗
Me encanta ❤
She sounds like a baby in this song
I can't stop listening to THIS song! It's my favorite on the album 😌
okay, enternal sunshine album really her best album
Righttt it’s UNDERRATED
My sis said this album was gonna be trash bc Ari is not in her thank you next era but this is the BOMB
Imperfect for you, true story, and eternal sunshine are absolutely perfect 😭🫶🏻 this whole album is stunning
@angelgtorres186 L sis
hey bestieee, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
It reminds me of one last time.
@Sandrinarhonda ordinary things is underrated
@AnnaF33 nooo
Yes sure
It's beautiful
@angelgtorres18 6 this is ari best work
YESSSSS OMFG
Exactly and the most relatable one tbh
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
the outro wow
Imperfect for you is one of the most perfect song
this song is stuck in my head
"Imperfect for you" INLOVE ♡
How she sped up
Ok I have my favorite ❤ but at the same time I can't choose 🥺
Soo good 🤌🏻
"Into you" 2.0🥰✨
❤❤❤
can't wait for the live performance!
similar storyline to needy ! 🥺
esta me llegó ariana :'(
AAAAAAAAAAAAAA ❤️❤️❤️❤️
Yeah
this is so pixie hollow coded idk how to explain it
Fucked up
Anxious
Too much.....
la amoooo
Big sister of imagine
Rainha 😢❤
OMG😍😍 the vocal💥💥💥
This makes a great slow dance song
The imperfect for you part is so addicting im quite literally getting chills
Will I always be alone ?💔
Imperfect for you
the production of this album is INSANE
Yeah this song goes hard! I love it!❤already added to my fav songs of 2024!
best song on the album
OH MY GOD???
Aaaaaaa ❤
Crossfaded asf but this song.. idk if sober me will like of
Crossfaded asf but this song.. idk if sober me will like of
God bless Ariana Grande
In my opinion, This is her best written song on this album. “ Ill never forget when you told me I was fvcked up, anxious, too much, but I Love you like you need me to, imperfect for you” WOW JUST WOW. Hits my core so much. Also describing how she’s messy completely and distressed but she was never like that until she met the person. No matter what she always wanted to give her best to this relationship and doesn’t wanna make it fail, hence ‘imperfect for you”. Lyrically explaining how this relationship has taken its tole on her while she was in it. I love how intimate, straight forward, and honest Ariana is in this album. She’s really connecting and letting us know everything she’s feeling mentally, & physically, and that is so human of her❤
omg :face-fuchsia-tongue-out:🤩
Her voice is so mesmerizing like you're just into a whole another world!❤
imperfecto para ti
This song is so amazing it's calm gentle and just beautiful
All her songs except Yes, and are a bit quieter from this album but I love them 💓🌸💖
Bem numa!😭🎶
ay no 🥺🥺🥺🥺ಥ‿ಥ
This ❤
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
I’m not sure about insane 🙁
Meh
Real
kind of like sweetener production better
SERIOUSLY
could NOT agree more
not really though lol you fans are reaching
Yess it's amazing ❤
Yall are really overhyping the hell out of the album if you think it’s “insane” lol
@topbackpodcast2615 Damn sorry your having a bad day it will get better just listen to this album again 😁❤️ or just go fuck yourself you pick
@topbackpodcast2615 actually each song in this album is better and better than each other and have things that a song really needs like perfect prechorus and chorus and bridges
greetings, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
It’s Max Martin. The genius, the God, the Master, the most talented music producer and songwriter there is… He’s something else ⚡️
I covered imperfect for you and would love if you checked it out, thx! :)
@szactrl he meant in a good way
@sergiovilcu ari also produced :) and yes he's really good!
Max Martin is a musical genius!
@tiana1420 RIGHTTT
Sweetener 2.0
i'm super curious about how they made her voice sound so light/delicate/high compared to the live snl version. at first i thought this was pitched up but she sings in the same key as the live video. they definitely messed with EQ to reduce lower frequencies/add air but the change is just so drastic like HOW
@AchlysMaru i hope she makes a lower version cuz i LOVE this version but her lower range is just so beautiful and fits this song sm, ive been listening to the SNL version so much😫😫
all these bum bitches hating in the replies, but they don’t know how to appreciate real good music. the production on this album is quite literally insane, crisp, and the best from her entire discography.
I think you got it wrong bud
no one is wrong totally ari said it is different for every1 @pabawoode2319
it says "i'm NOT like that SINCE i met you"
Omggg so good
this might be my new favourite😍
Me encanta 😭
❤❤❤
This is the one
Oh my god
LYRICS
My boy
Come take my hand
Throw your guitar and your clothes in the back seat
My love
They don't understand
But I'll hold your hurt in the box here beside me
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
And usually I'm
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Let's go tonight
'Cause there's just a few seconds left till tomorrow
We have
All that we need
Let's leave our baggage and wine at the table
Mmm, how could we know
That this was a happy disaster, mmm? (happy disaster)
I'm glad we crashed and burned (we crashed and burned)
I'll never forget when you told me
You said, "usually I'm
"Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you"
Messy
Completely
Distressed
But I'm not like that since I met you
Mmm, how could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
When we're feeling
Fucked up
Anxious
Too much
But I'll love you like you need me to
Imperfect for you
Messy
Completely
Distressed
But I'm not like that since I met you
Imperfect for you
Fucked up
Anxious
Too much
Like you need me to
Imperfect for you
Messy
Completely
Distressed
Not like that since I met you
Imperfect for you
"imperfect for you" IS SO MESMERIZING OMG
This song has my heart❤️
This is perfect oh my
This is Hannah Montana/miley Cyrus coded
Adoro!!! ❤
Me encanto
Gracias Ariana por este album tan hemoso❤❤❤
MEU DEUS ESSA MÚSICA É PERFEITA, ELA É MUITO ARTISTA MEU PAI
so magical 💓💓💓🌙
OMG😭🤌🤌
My fav song so far of the album ❤
Sounds like I'm in love 🫧n I'm single
No importa por donde lo veas, ella te puede teletransportar a otra dimensión sin esforzarse tanto 💖 escucharla cantar es como un sueño del cual no quiero despertar nunca💖 sos la mejor ari 🫂
My soulmate
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
So in love with this album
woa the instrument is so hypnotising
crying. shaking. LOVE THIS ALBUM
that album is aoty since now
Omg i love this one so much
What a beautiful song.
THIS IS MY FUCKING FAVORITE FROM THE ALBUM
SHE ATE!
spooky x sexy sounds
Ari you are one special girl
HER VOICE
this songs give last ever night with THAT person, it's SO GOOD and new. Love the "Imperfect for you" that sounds like a P.A message or a notification. So stunning
Queeeeeeeen
when she says imperfect for you, chills run down my spiiinneee, IT'S HERE PEOPLE
Wonderful
So far it's my favorite song from the album 🥰
This song is on repeat. Totally in love.
U always made my day❤❤❤
I love this song!! I wish it was longer! This genre suits Ari so much!
❤❤❤❤❤❤❤
Finallyyyy, the whole music industry was waiting for this masterpiece
This is my favourite one in the album❤
Your voice made me happy and comfortable
This is definitely my favorite 👏👏
Ateeee
Mi canción favorita ❤💄
im OBSESSED
U slay it as usual❤❤❤❤❤
🥺💝
this is my favourite song on the album ari cooked and ate down!
Best song of the album
This is so pretty and so sad at the same time 🥲
Aaaaaaa❤🎉
Yes and: Boy, come on, put your lipstick on (No one can tell you nothin')
Come on and walk this way through the fire (Don't care what's on their mind)
And if you find yourself in a dark situation
Just turn on your light and be like
Imperfect For You: How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
How could we know
That this was a happy disaster, mmm? (Happy disaster, mmm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
Ur the bestttttt❤
ARI ILYSM
It's a very beautiful song, thank you Ari 💗💗
Imperfectly perfect 🥺❤
I’m so happy you’re singing again!!!❤♥️💋☀️
โอ้ยย เศร้ามากกก😢😭
Mi reina esta de vuelta :)
Playing this one at my wedding ceremony this year
best song in the whole album🙌🏻
You are perfect, I love you with my life ❤️🩹🕯️
Really cute and relatable song ♥
Im crying
Omg I love it the best song in the album 😭😭😭
The “imperfect for you” is so intentional 😩🫶🏾
This lyrics are too real help
Fav trackkkkkkkkkkkkkkk
Oh my god it never happens that I love an album at the first listen… but it’s happening rn
Это пипяо товарищи
0:35
This does not feel real. Thank you so much ariana for making gorgeous songs and making our day with your voice. We are so lucky to have you in our generation 💋
The “imperfect for you” is SOOOOOO satisfying like almost eerie and creepy
MY FAVVV
❤❤
Who's Listening in 2024?💯
Loveeeeee ❤❤❤❤
I LOVE THIS
Omg I loved and enjoyed so much!! Love U Ari!!💞
This album definitely gives calm
Omg llegue a esta parte del álbum para llorar 😢 oh Ariana i love so much
Eres la mejorrrr ❤️
This gonna be my comfort song im sure
Imperfect for you is perfect for me ❤
Loveee love love, is perfect😭😭😭❤❤
Keeping this on repeat. It’s so addicting and the subtle harmonies when she says “imperfect for you” SENDS ME TO SATURN!
This song is sooo good!! Sounds like running through a field with a guy you fell in love with and are running away to better things but also yall are scared and its unknown HAHAHA
El "imperfect for you" suena tan genial y da escalofrios
yours truly: honeymoon avenue: I really wanna take that turn, before we both crush and burn
eternal sunshine: imperfect for you: im glad we crushed and burn
the referenceeee
Best song I’m sorry I had to say it
Very very… MAGICAL, IS SO PERFECT
ARI U GOT ME GOING THROUGH AN EMOTIONAL ROLLERCOASTER 😭😭😭
OMG THIS IS MY NEW FAV
WHY????IS?????THIS????SOUNDS?????SO????GOOD???!?!!!
DESERVES A MUSIC VIDEOOOOO
Awww 🥰😢
Is so good, ARIANA THANKS FOR WORKING ON THIS BEAUTIFUL ALBUM FOR US, WE LOVE YOU ❤
I'm dying
This gives me chills
ARI I'M CRYINGG
Te amooooo Ari 😭💗💗💗
Women never forget words!
i loved you since you started your music and i love you now thank god you exist; i love you with my whole heart and always will
🩵🫧🫶🏻
Best album
Imperfect for you
imperfect for you, heavenly
Queen of pop 👑
A melhor música do album
O Album esta tão delicado , tão doce, emocional e tão verdadeiro. Essas musicas poderiam ser facilmente um filme lindo de um musical. ❤
Not me tearing up
Oh this makes me SOB bro. Best album yet 🔥
OMGGGGGG 💔=❤️🩹=❤️ my heart after listening to your songs
So chills 🎧
I feel like she is trying to tell us that she has been so misunderstood, I hope she tells her side of the story at her own time.........
this should be the third single! or fourth with the boy is mine as the third
😍😍😍
1:11
I'm in love OMG
Perfect❤
best song on the album
“I know we’ll be there for each other…” 😭
esto me dio vibes de glinda, que hermoso❤️🩹
TE AMO COMO NO TIENES IDEA
I’VE GOT CHILLS. THIS IS SO GOOD !!! 🥹
❤❤❤
💕🌙
Realmente estoy tan feliz por tener de nuevo la oportunidad de escucharla cantar un nuevo album, sin duda alguna Ari siempre va a seguir haciendo música demasiada buenaa! ♡
❤️☀️
this album just feels so safe. I can’t explain it.
Music perfect ❤❤❤❤❤❤❤❤
My claimed track !!😋
DIOS MÍO ESTA CANCIÓN ES DE MIS FAV😭💘
I love it♡♡♡♡♡♡♡♡
I love how the “imperfect for you” sounds! I’m not really into all the musical notes and keys and whatever so I don’t know what to call it. But I love how it’s different from the rest of the song! It’s so beautiful
Amo el "imperfect for You" 🥹🥹❤️
❤
i love this fucking song.
54 mins!!! early 🤭✨
I love the "imperfect for You" 🥹🥹
LETSSSS GOOOOOOOOOOOOO
she’s so angelic
New fav catch phrase: I’m just being Imperfect for u 😩
Ari did her THANG on this album❤️✨
the best song on the album
The "imperfect for you" sends me to heaven fr
Shes so back
Love you ariana ♥️
brazil loves uuuu
THE WAY SHE SAYS IMPERFECT FOR YOU ACTIVATES CERTAIN CORNERS OF MY BRAIN.
i’ve missed her soft and delicate songs
Brasil em peso
THE WAY SHE SAYS IMPERFECT FOR YOUUUUU JUST SCRATCHES MY HEAD SO GOOD 😍😍
“ how could we know
we’d rearrange all the cosmos, mmm?
we crashed and we burned
now i just can’t go where you don’t go” sounds really familiar but i can’t figure out what song it is 😭
Ariana esta en el puesto número uno de mis cantantes favoritos por que su voz y su música es lo mejor
This shi got me feeling some kind of way 😢
This one gonna be definitely one of my fav
HOW BEAUTIFUL🥹🥹🥹💕
THIS SONG IS SOO FUCKING BEAUTIFULL
I’m gonna tattoo this someday
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
may ik what that means ?
@jhunricvillordon3488 ???
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
omg is your pfp Clouds by Before You Exit
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
Omg for real like ie sounds so heavenly
Seriously, it's that push from the edge.❤❤❤
FRRRR
Right?!
greetings, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
YESSSSYESSSS
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
REAL! Best part hands down
😢😢😢😢😢😢😢😢😢😢😢😅😢😢😢😢😢😢😮😢😢😢😢😢😢😢😢😢😅😮😮🎉😢😢😢😢😮😢😢😢😢😅😢😅😂😢😢😅😢😢😢😢😢😅😢😢😢😢😅😢😅😢😢😅😢😅😅😢🎉😢😢😢😮😢😮😢😢😮😅😢😅😢😢😮😢😮😢😅😢🎉🎉😮😮🎉😮😮😮😮 @laiya2904
SAMEEEE
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
REAL
best friend! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
Não faz isso comigo :(
Esto se siente tam nostálgico
❤❤❤❤❤❤❤❤❤❤
No this one is actually the best omg
I'm surprised!!!! 😢
Noooooo que canción tan... simplemente es perfecta
QUE PRECIOSIDAD 😭😭😭😭
1:00
i love being a child of ariana grande.
It seems like the word ‘rearrange’ comes up in every song. Nothing wrong with it I just found it cool
❤
I feel like the music video for this could include dolls 🫣❤️❤️❤️❤️
ok i think it’s my fav with bye and supernatural
WHEN SHE SAYS IMPERFECT FOR YOU, MY BODY GETS THIS FEELING WITH CHILLS LIKE HOW DOES SHE COME UP WITH THESE NOTE CHANGES. DEFINETLY in my top 3
THE WHOLE ALBUM SLAYYYYS AND I AM SO SO SO IN LOVE ❤❤❤
INLOVE AT FIRST LISTEN!!
i love the red
It doesn’t make sense to me yet
but it will and I can’t wait
IM CRYING PLEASE ARIANA I LOVE YOU SOOO MUCHO IM CRYING IM SO PROUD OF YOU
Masterpiece but fun fact I think ariana singing about SpongeBob!!
Missing old Ariana's songs like from dangerous woman era and my everything era
I hope we get a mv 😭
I agree this made me emotional
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
@Myeverythinggg nooo this gives me underrated for the general public but fan favorite vibes, i don’t want it to be a single and become mainstream😭
I say this for each song, how can they all be soooo goood ! ❤
literally
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
sorry its true story
@heyhi5503 that’s you, but I want a mv
fucked up
anxious
too much
messy
completely
distressed
i love how she says it
if somebody isn’t playing this whole album at my funeral with a red or pink casket i don’t want it 😌☝🏻
ARIII
Omggg chilllll 😭😭😭🥺🥺🥺🫶🏻🥺😭😭☀️☀️🥺😭
This song is perfect for me.
this is already my favorite
DEAR GOD IM CRYINF
without a doubt the best album I love you Ari
❤❤❤❤❤yes
Esse álbum não tem uma música ruim!
She has literally made me fly little by little over a cloud with her magical voice💫
I love her 💌
OMGAD ARIANA ILYSM UR SO GORG
This song just sends chille troughout my spine
This song does something to me
this one brought me to tears.
Here
DBHASDHA THIS IS SO BEAUTIFUL
❤❤❤❤❤❤
Me encantó la canción😍
i really liked this one a lotttt😊
this song almost sounds like the aftermath of needy and imagine
Vou pra casa do krlh com essa
But I love you like you need me too 😢
🇮🇳🇮🇳🇮🇳🇮🇳❤❤❤❤
Reminds me of tightrope from the greatest showman ❤ love this song smmm
imperfect for you reminds me of 13 going on 30, idk why
I feel I'm hearing a little bit of country in this song and it's very calming...🫧
Te amo
Thanks Ari, this album makes me very happy, I'm so excited to sing this when I learn to read ❤
THIS ONE IS PERFECT FOR ME!!!❤
❤❤❤❤
This is already my favorite song the album
Streams
My favorite song from this album IS FOR SURE THIS ONE when I tell you CHILLLS down my spine listening to this
“I’m a girl with a whole lot of baggage” - ghostin
“Let’s leave our baggage at the table” - imperfect for you 😭😭😭
This is so different! I love it!
🤗
Obrigada por tudo ❤
♡
CRYING OVER THIS WHYYYYYY MY GIRL WENT THROUGH SO MUCHHH ILYSB ARI
MY FAVE SONG OFF OF ETERNAL SUNSHINE❤❤❤❤❤
te amooooo ariii, te amooooooo ❤
YAYYYYYY ETERNAL SUNSHINEEE
She sounds like 2016 ari
✨I’ll hurt your hurt in a box here beside me✨& ✨now I just can’t go where you don’t go✨
Hits hard, dude 😢
I LOVE !!!
🪽”imperfect for you”🪽
😭😭😭
Mi favorita desde ya, la reclamo como mía
HOW IS THIS SO PERFECT ARIANA! THE VOCALS, THE LYRICS LITERALLY EVERYTHING KILLS
Te amo Ariana
"Imperfect for you" ✨ 🌸 💞✨️
Ringing in me! ♡♡♡๑๑♡
This song gives me chills 🥹
❤❤❤❤
Me hace acordar a mendy moore 😅
🤍🤍🤍
Wait…this is so nobody else coded 😭😭
THIS IS TOO GOOD
This is my fav on the album
Your vulnerability is so so soooo respected please know this
Omg! This is a complete masterpiece 🥹🥺
MEU DEUSSSS, EU AMEI ESSAAAA
I wish you the best ☀️
Después de escuchar todo este arte en Spotify lo tengo que hacer en Youtube, gracias reina ❤
After listening to all this art on Spotify I have to do it on Youtube, thank you queen ❤️
BEAUTIFUL
gracias por tantoooo! 💗💗
Wow❤
The cosmos were arranged for me to hear these beautiful songs
Verse 1]
My boy, come take my hand
Throw your guitar and your clothes in the back seat
My love, they don't understand
But I'll hold your heart in the box here beside me
[Pre-Chorus]
How could we know
We'd rearrange all the cosmos, mmm?
We crashed and we burned
Now I just can't go where you don't go
Chorus]
And usually I'm
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Verse 2]
Lеt's go tonight
'Cause there's just a fеw seconds left 'til tomorrow
We have all that we need
Let's leave our baggage and wine at the table (Ooh), mmm
[Pre-Chorus]
How could we know
That this was a happy disaster, mmm? (Happy disaster, mmm)
I'm glad we crashed and burned (We crashed and burned)
I'll never forget when you told me
[Chorus]
You said, "Usually I'm
Fucked up, anxious, too much"
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you, mmm
Pre-Chorus]
How could we know
We'd make the bad stuff delightful, mmm?
I'm glad we crashed and burned
I know we'll be there for each other
[Chorus]
When we're feelin'
Fucked up, anxious, too much
But I'll love you like you need me to
Imperfect for you
Messy, completely distressed
But I'm not like that since I met you
Imperfect for you
[Outro]
Fucked up, anxious, too much
Like you need me to
Imperfect for you
Messy, completely distressed
Not like that since I met you
Imperfect for you
estoy enamorada de todas las canciones
Imperfect for you ❤
I think this its my fav
pero queeeee
I can’t even she’s soooooo good at what she does
OH THIS IS SO GOOD AAAAAH
THE BAGGAGES OMGGG
Sounding like a Country Hit Ari 💯😁
sounds so ethereal
Happy internal sunshine everyone!! <333
Pleasure and Pain together 😭🤍
❤❤❤ I Love You
This song gives me the chills
Después de tanta espera, todo ha valido la pena, te amo Ari, gracias por tanto, gracias por tanta felicidad❤
AAAAAHHH ❤
Omg i love this
Esse álbum nn tem uma música ruim
que temazo 😭💗
😢❤me encanta
UN FEELING 😭😭😭
😭😭😭
😭😭😭
Después de tanta espera, todo ha valido la pena, te amo Ari, gracias por tanto, gracias por tanta felicidad❤
❤❤❤❤❤❤❤
best song
Walk with me...
Remix this with Beyoncé and then Ari on a Plastic Off the Sofa remix.
Te amo más que a nada💗
💗 hermosa canción
that’s sooo good😍😍
Wow, qué hermosa canción!! La amo 🥺❤️
Ngl true
🫂🛐
the calm in my storm 🤞🏼☁️
The best song on the album 😫
I need Madison Beer to hop on this.
<3
I don’t think I’m alive omgg
The best of the album. Even, made me cry sometines when she said "imperfect for you". The way of how melody sounds like out tune in that part, fits perfect with the song. Ari, you are one my biggest inspiration in my life, even i get emotional writing this again hahah
☀️☀️☀️☀️
Best song on the album in my opinion along with supernatural
☀️☀️☀️
te amo💘
OH MY GOD
❤️🩹
Diosaaa
TEMAZO 😍😍😍😍 OMGGGG
“imperfect for you” is PERFECT🤩🤩
I think this is my favorite song with the other song bye
🎉🎉🎉🎉
Primera escucha te amo 💗😭
La melodía y la voz de ariana, todo lo que quería escuchar 😭
SAME
Real
Real!
Fr. I love it😭
ITS ON REPEAT
Wtf ThatBacon_Shorts
It’s so perfect wtf
Totally agree !
Frrr
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
Yes
Amen
bestie bestie! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
Chills 😭💕😍
No sabes cuanta falta me hacías😭
DIOS MÍO T AMO
😭😭😭
Your Voice is perfect❤
<3
ÉSTO ES HERMOSO 😭😭😭😭😭
ARE U KIDDING
This is one of my favorites!!!
this might be one of my favourites
💔💔
Simplemente te amo estás canciones me llegan al corazón y el alma🩷❤️
SHE LITERALLY CHANGED HER PFP LIKE 30 SECONDS AGO I THINK IM THE FIRST PERSON ON EARTH TO SEE
:')
IMPERFECT FOR YOU !
Alguien sabe a que hora la traducen? Jajaja
i love Ariana grande mi everytime ❤❤❤❤❤❤
https://youtu.be/WkkfKSKJ6TI?
te amo Ariana 😭
Ur so talented Ari ur music just makes my day😊
you were right
Thanks thanks thanks Ariana 🌞💛
I think so far I love these songs! All of them. But this is #1 for me! On to the last track ❤️
Amazing
Wow what a fucking BOP
I love your music Ariana
Oh my Goshhhh😭😭😭
BEST SONG
Good vocals, good songs, good messages... Love it
I swear Ariana hasn’t made one bad song her voice is just so beautiful and good
This album is amazing 🧡
Omg this melody is haunting as hell 😮 Lana Del Rey vibes !! ❤
CHORANDO
Every song is about her leaving her man for another man lol any one else picking up on that through these lyrics??
The melody gives me vibes of Swettener ✨❤
the melody the vocals this entire song is just everything.
We love this girlllll
let's take this album to n1 you know it deserves it
This is so gooddd
I get such a weird funny joyful scary feeling when she says “imperfect for you”
She did just rearrange the cosmos
Haunting like in a good way btw holy shit chills
no it’s not
Definitely
She is so vulnerable with this one
Here before the internet ruins the album for me ❤️❤️
THE DEFINITION OF MUSIC AND ARTIST MY FRIENDS, THIS IS AWESOME:,)
OMG IM SO HAPPY I THINK THAT BETTER THAN POSITIONS
I love itttt
😍
This album is everything
THIS SONG.
😍😍😍
La amo 😭
THIS SONG IS TRULY PERFECT
Ariana vamos comemorar tomando um Açaí com farinha?❤❤❤❤❤
Thank you so much for this Master piece ❤❤❤❤
Her voice is so angelic 🤍
This song is crazy huhuhuhu
AMOOOOOO
stanning ariana grande isn't just a hobby, it's a lifestyle, a reason to breathe, an escape from this cruel world filled with thieves. it's art <3
this is soooo good 😭😭😭
Keeping this on repeat..I don't normally listen to ariana but wow. She killed it with this...sounds so free ..angelic. she sounds happy
fav so far this one is beautiful ariana 🥹
Because she is an angel 😇 ✨🌙💫
Frr
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
totally! I covered imperfect for you, would love if you checked it out, thx! :)
Without having to be extravagant, Ariana gave us a simple and beautiful album, this great artist is worthy of admiration.🥰💋
❤
Imperfect for you, but you are all the good thing in the world🤍
The line "crushed and burned" reminds me of 'Honeymoon Avenue' 🥹🫶
Ayyy te amo mil 😢❤
Sounds like a princess
😢😢😢 love
Arte ❤❤❤❤
Her voice is heaven
My top 5:
Imperfect for you
Supernatural
don't wanna break up again
Yes, and?
Bye
Eternal sunshine is just wow
Llorando
❤❤❤❤
That one person @jordysan commenting the same thing on every song 😭
crying
OMG ONE OF THE BEST SONGS OF THE ALBUM FOR ME
Es increíble ❤
This song just tingles my brain
i think I'm getting a heart attack someone help it's too beautiful
The chorus is just wow
That’s exactly what I was thinking 🥹
me too. ❤❤
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
Sameeee
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
yes imperfect for you and super natural are def my fav toooo
this album saved so many of us
Screaming, crying 🥲
This song makes me feel like I'm at a Disney princess ball
you are perfect god I will die with your voice te amo ari!! 💗✨💋
I’m sobbing this song is heaven sent it’s so perfect
Her voice is so beautiful😭😭I’m crying
ahh my heart this is perfect for me
omg i love thiss
😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍😍🥺
This reminds me of one song in Disney or in a movie which I love from the melody but can’t remember 😭😭
I’m feeling that I’m in heaven
i am crying
Thank youuu so much,❤❤❤❤❤
I love u ari
OH THIS BOP. OMG.
❤️🩹☀️🥹
The dissonce is crazy ❤
Wish this has a music video too 😫😫😫
Im in love with this one
❤
❤❤❤❤❤❤❤❤❤❤
ESTO ME DESTROZA, ERWS LA MEJOR ARIANA GRANDE
IMPERFECT FOR YOU ✨
I listened to all of the songs and this one just hits different than the other ones. ❤❤❤
SHE IS ICONIC 😭💋
That's because I am an Arianator
imperfect for me
EL MEJOR TEEMAAAAAAA TE AMOOOO ARI
OH this songs taking it
I'm not feeling anxious at all with this masterpiece
Eternal sunshine 😭😭❤️
Ari i love u 🥹💋A caring mother
Que letra!!! Que vocais!!! Que instrumentais!!! Que álbum!!!!
🥺🤍
You are a true healerr❤
i better see her album at the grammys. periodt.
when i heard that first “imperfect for you”???? wow
ari i'm so proud
THE “IMPERFECT FOR YOU” HITS EVERYTIME
EL IMPERFECT FOR YOU OMG
So much in loveeeeeweeew❤❤❤❤❤
im so happy right now
This is very beautiful, waiting was worth it, I LOVE THIS ALBUM 😩
I immediately said, okay guitar! this is a totally different instrumental sound for her, ICONNNN, the change in note for imperfect for you, her mind!! perfectly imperfect
This song hits diff
The “imperfect for you” key change is so GOOODDD
THESEEEEE AREEE SOO GOODDD😭😭😭😭😭😭😭😭
I fckin live this 😍😍😍
My last song to hear, best one I saved and overall all these songs I don’t hate, I adore them already and will forever
♡˖꒰ᵕ༚ᵕ⑅꒱
this album is like medicine
it feels unreal, happy release day for every fan that never stopped supporting ari
Mother😢❤
🇧🇷🤍
Te amo y te amaré hasta la eternidad ❤🫂
Este album sin duda es hermosoooo
WHAT IS THIS FEELING 😩😩😩
Perfecto total
This album is for sure my favourite 🫧
Todo el album es sobre mac 🥺😭
FRRRRR I KEEP REPEATING
There are no key changes in the song, what you're hearing is dissonance, which is technically not a key change but sounds similar due to being slightly unpleasant to the ear
It’s the definition tbh
She’s a GENIUS!❤
I didnt expect it either.
@noamchemla thank you! also very smart bc of the theme of the song:) love it sm
HER MIND.
Frfrfrfrf
IKR! it was unexpected but it’s gorgeous
frr
Typically a modulation but yeah it's great
It's technically a modulation but yeah it's great
ThatBacon_Shorts SYM
purrr
Frrrr
it’s not a key change, it’s an alteration🩷
@noamchemla i don’t think there’s dissonance - isn’t it just a chromatic thing?
@noamchemla like it’s just each note of the tonic major chord raised a half step to return back to tonic. like a neighboring chromatic chord.
나도 이런 변형 코드 쓰고 싶었거든 유명 팝가수한테 ㅋㅋ ㅡㅠ
수학 불협화음 쓰기 개쩌누ㅋㅋ
Arianators panic after Dalton runs Ariana over with a car after album release https://youtu.be/FXRogf04_x4?si=wJBh1yu7XzzjZ3Qc
I’m a 13 year old boy artist, Please check out my track ‘angel’, it’s inspired by ‘yes, and’ and it’s kinda similar to this song (I’m not joking I’m serious) I have a 5 octave range from whistles to baritone notes and hope you guys can support my dreams of being a singer lol
There is no key change. It’s just the Neapolitan chord of that key 🫶🏻
I just know it sounds like “🧙♀️🌸”
Thnks for spoiling
@musicnstuff_22 listening back i think youre right, but i was just trying to break it down barney style since i imagine the original commenter isnt music theory adept
@noamchemla true true ! and since the harmony kind of blends over into the next chord, it might sound dissonant a little too! :)
@musicnstuff_22 yeah my main point was that they were conflating "odd sounding" with "key change"
it's not a key change! it's dissonance
@joliejenn9537 oh my god you're righttt
Just heard it cute
@noamchemla lol sorry but what’s key change? Also dissonance? 😭
hey there, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
@LOML423 a key is essentially a group of notes that a song "centers" around and sound good together. so a key change is going from one key to another. dissonance is something that doesnt sound naturally good to your ears due to either musical expectations or the physical properties of sound waves.
@noamchemla it’s actually smart since it’s “imperfect”
@noamchemla ahhh that is why only that part is dissonance, because it's "imperfect"
well i cant speak on the intention behind the track because im not the artist, it could be that the production was handed to ariana and she came up with a melody before writing lyrics to it, or maybe she already had lyrics and chords and it came about more naturally. you never know! @Cindy12345ful
Duuuude I love it but everyone I know seems to be torn. Some love it some hate it.
Legit the part that has me listing again. Love it!
LITERALLY, my heart js melted
YESSSS
W O W lol just wow
It's not a key change that is a chromatic slide. The F major is not in the songs key and it's a weird borrowed chord that creates this exotic effect.
Modal interchange *
Not a key change❤
yesssss, i love this <3
@noamchemla It's an "Imperfect Melody" 🎶🙃 Ffff For THAT! 😏
I covered imperfect for you would love if you checked it out, thx!
Yup I just listen for the key change ❤
It's a "chromatic embellishment,"in the manner of Schubert, maybe not really a key change, the little dissonant, or "clashing" arpeggio there, a strand for the sung word "imperfect,." So, idk,"perfect," but the musical formation mirrors the lyric there and that's a nifty element in this song.
ItzRileyShorts 🎉😢🎉😢🎉😢o🎉😢🎉😢🎉o😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢🎉😢🎉😢🎉😢🎉😢🎉😢o🎉😢🎉😢😢🎉🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢🎉🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉o😢🎉🎉😢🎉😢🎉😢🎉o😢🎉🎉😢🎉😢🎉😢o🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢😢🎉🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉o😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢🎉😢😢🎉😢🎉
Damn you are right bestie!!!
I think it’s a major 2nd chord which is soo dope ❤❤❤❤
FR
Yeah right? I'm going to the piano to figure out what she did.
I said the same
frrr it's so magical 😭❤ feels like a movie
Its not a key change, she's using notes that are not in the scale that she is singing in for most of the song, hence the "imperfect" sound and the adlib for that
yes
Hii guys , me and my cousin have a music covers channel can u please check this Ariana song cover we made , Tysm ❤ https://youtu.be/UqmQLv14f4k?si=U8c5l-MRanlnhM-H
Frl ❤️🔥😍
FRRRR IT GIVES ME CHILLS ITS JUSY WOWW
LITERALLY I KEEP GETTING CHILLS
So true
Internet baiter detected.
@shamron0728 No one expected.
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु. .
@joanneweavermusic used this exact same chord progression in her album interstellar few years ago ❤
Kuncinya di ganti jadi C Eminor Gminor D C or Eminor C Gminor Eminor or E C G D or E Aminor C F G D Dminor C F or F#mayor G7 D4 Bes !!! 👍🤣
Oh, salah kuncinya di ganti dengan kunci Inggris !!! 👍🤣
Oh, salah lagi !!! 👍🤣
Ini baru bener ni ganti dengan "I'm Falling in love with you" !!! 👰🤵
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
Who actually wrote the music? I’m not usually a fan, but I saw this on Saturday Night Live and I thought the musicality of it was stunning.
@musicnstuff_22 . I have some basic music theory, but not much but I thought this was really gorgeous. I’m not even usually into this kind of music, but I saw it on Saturday Night live.
it’s not a key change she’s just hitting the tritone
There's so much confusion in the comments, I'll clarify it harmonically.
It's a wonderful passage that create ambiguity because of what happens before that, the progression goes E - F#4 (this is already dissonant in E major) - A - E and then it hits F major. Now F#m is in the key of E major, but already they had a surprising F#4 the first time, then the second time it's F natural, which is not part of E major tonality, and it's even more ambiguous tonally. And beautiful. It's just a chromatic movement from a major chord to another, it's no modulation, no N6 nor anything else as many said.
@noamchemla sounds hauntingly beautiful than unpleasant to the ears mmmm yes
Girl..
Tllllllllm ❤❤
I feel like this could also be a Miley song. Love the crossover vibes. Almost country trap. I’m in love
"Imperfect for you", cryingggg 🥹🥹
Su voz suena hermosa ✨🥺
❤❤❤
Yes.
sounds like a princess
OoOooooOoooOOOooo! I love this 🙌🏼🙌🏼. Especially the minor notes!
imperfect for you🥹❤
Mother won the best album of the year ❤❤
Jod3r que bueno álbum.😭💕🧸
I LOVE THIS
Que joyaaaaaaaaa ❤️❤️❤️💋💋💋
THE QUEEN IS BACK ❤
How many subscribers will i get from here
Current: 811
GOAL : 1K
‘before we crash and burn’ - honeymoon avenue
‘i’m glad we crashed and burned’ - imperfect for you
meudeus ari, está tudo tão lindo, a voz, os instrumentais, tudo está impecável, essa era é linda. álbum da carreira.
Calma Ari, eu preciso dormir hj😅❤
Pensando bem ,nem sei se consigo dormir 😮
Wow, This Song is Sad.
WHAT BEAUTIFUL ÁLBUM 🥺🥺🤎🤎☀️☀️
ARIANA GRANDE SAVED MY LIFE WHEN I WAS ONLY 14 ARIANA IS MY EVERYTHING IM A FAN SINCE 2015
So bitter sweet ❤
Magic🎉❤
It's feel like heaven💕
I knew this song would be a bop
She’s a literal sweetheart
if you didn't come from TikTok, you are allowed to like this comment 🤝
Andre 💓🦆
love this song so much 🥹
NO QUINTO SEGUNDO EU JA ESTAVA ARREPIADO, EU N TENHO PALAVRAS PRA DESCREVER O QUANTO ELA SE SAIU PERFEITAMENTE NESSE ALBUM
Diosssssss que perfección ⭐️✨️✨️✨️❤️❤️❤️❤️
the most comfort song from u ari😂😂😂😂 i like this mooment when i think omg this is so comfoty song but u say fucked up i love uu ❤❤❤
Te amo Ari me encantó ❤️
AAHAHHAHhaha
Que emoção Ariana grande Nós te amamos 🇧🇷🇧🇷🇧🇷
Que arteeeeee me encanta ❤❤❤❤❤❤
I was waiting so long thank, u ❤🥹
❤❤❤❤❤❤❤❤❤
I lov this❤
Eterno amor. 🔥
Te amo tanto veyy
This song makes me cry ♥️ so so good
I love you my queen ❤❤❤❤❤
La amoooo...cada like que me den recordaré su música
I LOVE THIS!!!!!!!!!!!!!!!!!!!!!!
SO BEAUTIFUL HER VOICE 😭😭😭❤️❤️❤️
OMGGGGGG YES 😭 WHY IS IT THISSSSS GOOD🥲🩷
❤️❤️❤️❤️❤️❤️
Imperfectly good!!!
THE WHOLE ALBUM MAKES ME CRY 😭😭😭
so great😭❤️
Salvaste el pop reina 😮💨👌🏻👌🏻😩😩😩
The " imperfect for you" gives me chills 😍
Ure my muse my goddes ure all for me ari i love soooo much in uncomparable
🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞🌞
❤💋💞
te amo
Hermosooooo
this is what heaven sounds like
FAVORITE.
😢❤❤❤❤❤❤❤
my everything
Her voice is so good
Ariana and Ethan are couples goals.
If u are a die hearted fan of ari u can love this cmnt
I LOVE
Esta canción me llegó al corazón
I love you Just like I have loved no one 🥹💕😭
Her voice is so pretty ♡
Ari sin ti no vivoooo !
I'm cryinggggg 🥹
I found my favorite guys 🥹🥹
❤❤❤❤❤❤
the princess is back
I love this girl
Eres la mejor, te amo muchísimo 🩷🩷😭😭😭😭
This is my fav song
I can kiss your feets if you wanna, why? The real question is, why not? Youre a queen
I want to cry ❤❤❤
fucked upp💕💕💕💕💕
Me hiciste tanta falta
QUEEEN,QUEEEN,QUEEEN ❤❤❤
Love you forever 💜
😢
Tan angelical que es su voz, me hipnotiza!!
the type of song u dance too in a elegant ball
Eternal sunshine is Eternally good
Nos hiciste mucha falta :(
“we crashed and we burned” HONEYMOON AVENUE
Owemji🥺😍
حبيت ذي الاغنيه😩💗💗
this is just perfect 😭
❤️❤️❤️❤️❤️
Es tan triste y hermosa esta canción 💖
A complicated masterpiece
Love this one
My favorite so far 😭🩷
the melody of “imperfect for you” is just perfect and giving me chills and chicken skin
heather ???
best song for the album❤😍😍😍
The best 😭🥰
Favorite song on the album
Your voice so pretty 💗baby
Love the vibes of this album so far, I think it could surpass TUN in my Ariana ratings 😳😳
I’m so happy about the drop but then can’t help but overthink of when the next album will be 😭 she will leave us to starve for so long again . I can’t help but want more
If you didnt come from tiktok, you are allowed to like this comment
OMG MY #1
💧
ESTO ES ARTEEEE AAAAAAAAA
NO PUEDO DESCRIBIR COMO ME SIENTO EN ESTE MOMENTO, I LOVE YOU ARIANA 💗💗💗💗💗
AHHHH LOVE SO BEAUTIFUL.
This song is the best one in the album in my opinion. It literally gives me chills. SO BEAUTIFUL❤
Aghhhhsjsksbsisb
im so glad i didnt have to wait until 5 am for this
ARIANA EU TO CHORANDO
IS THIS FOR ME 😭
THE BEST SONG
Alguém do Brasil?🇧🇷🇧🇷🇧🇷
🩷
THIS is wow.
@arianagrande we love you so much❤❤❤
@arianators❤❤❤
i will ALWAYS fucking ADORE this woman’s lower range
Ariana Grande
comes back to Japan😢
Ariana, I know you won’t see this. But I made my most recent song on my channel about you. I know you haven’t had much luck with guys, but with me you wouldn’t need it. 🍀
Completamente harté Ari es la mejor ❤🎉😍👑🫂
These lyrics, this melody, thie voice . Am i in Heaven ngl?
I mean it’s diffrent???
She Will save our 2024!!!! Every son just become better and better OMG 🤍🤍🤍
IM SCREAMING🥰🥰🥰🥹🥹🥹
I love you Ariana 🌷👑
Euphonious, so soothing. Ariana melodizes! 💖
AMAZING SONG ✨️❤️🔥
OOOOOOHHH THE CHILLS IN THIS SONG
Best in the album
it kinda sounds like imagine 😭💖
❤❤❤
„Crashed & burned“ yea.. Leon Thomas we heard ya😂
Es que es una joyaaa👌🥹🥹
Amooo, es que... es arte, te amo Ari❤
Listening to this while walking outside, it’s raining, & your makeup is fading, it’s 7pm, & you got stood up idk
I love you mommy for this album and all your upcoming ones❤🥰
La voz más perfecta del mundo ❤
MI SEÑORA 😍
is that a good or bad thing
Omg 🥹❤️
Voy a llorar , es demasiado hermoso 😭🥹🥹💗💗🥰
Oh oh oh that "imperfect for you" a princess show up from nowhere and sing it for her❤️
„We crashed & burned“ yea.. Leon Thomas III was here 😂
Obrigada ari ❤
This song is so good
😭😭😭😭😭😭
😍😍😍😍😍😍😍
I love this song ❤️🩹
😭💗
This needs to be like in a Disney movie or something
Love you Ariiii! This song is beautiful!!! I love it❤
This album broke me ilsym ❤
Esté álbum es el mas corto, pero el mas sandor❤😢
This is like better off 2.0
Lindo lindo lindo lindo lindo, sem palavras 😭😭😭😭
BYE I CANNOT FIND A SONG THAT I HAVENT CRIED TOO😭💓
i love you so much really u can’t believe how i love you, my therapy since 2014 ❤
My Favoriteeee😍😭
WOWWWW!!!!!! 🎉🎉♥️
Ikr
Same seriously same, I was not even prepared for the intro
Sheeeet so gandaaa!!! Kilig werut ko
My album “Eclipse” came out 2.23. Ariana you inspire me to come out with another album 😭⭐️❤️
Ariiiii es perfección ❤❤❤❤
Diva nunca erra ❤
so beatifulll song ❤❤❤❤
MY FAVORITE
❤❤❤❤❤🇬🇦🇬🇦🇬🇦🇬🇦🇬🇦🇬🇦
My fav 😭
omggggggggggggggggggggggg
you're imperfectly perfect
Beautiful 😭😭
I’m gonna cry !
screaming crying throwing up
“We crashed and we burned” PARTIAL YOURS TRULY REFERENCE
This song is beautiful I love it
One of the best for me
IT GIVES ME THE CHILLS WTF
She killed it with this album it gives me chills, i feel so bad we get this and meanwhile she was making this some of her old songs were getting leaked, besides that I am so happy the album is out go stream for ari!!!
Those who love Ariana Grande like my comment
ME ENCANTÓ ❤
"Fucked up, anxious, too mich..." this is all so relatable
🔥🔥🔥🔥
MY GIRL IS BACK 🥹❤️
te extrañe madresita mía 😭😭🩷🩷
I Gonçalves cry, thanks ariana this is so perfect❤❤
I love you ari, can feel how low u had gone through, love you my GLINDA, stay awesome and amazing always
OH MY GODDDD ❤😭
😭😭🩷🩷🩷🩷🩷amoo
thissss❤️❤️
Gracias ari por llegar a salvar el pop😿💘
I love you Ariana Grande ❤️
She knows how to make a person feel better 💐 💔❤️🩹
te amo ariana desde chiquita me vestía como tú y hablaba como tú, hoy cumplo 8 años escuchándote 😭😭💞💞💞💞💞💞
🥺🥺🥺
ARIANATORS ARE WE BREATHING ??
❤❤❤
I feel bad for dalton, lily and her kid. They deserve so much bro.
Flores no campo, uma cesta de piquenique, cheirinho de natureza, sensação de infância, obrigado Ariana ❤
yayyyyy best day
La amoo
no she does too
@cloudsgirlie no just them. Ariana is happy now with her spongebob. What more could she ask for?
This>>>>>> 😍
❤❤
“ we crashed and we burned” oh mannn I love ari she better get album of the year this a dam masterpiece right here this the 3rd song I’m listening to in the album arianator forever 💕
💞✨️🫶🏻😭
I need traduction In spanish PLEASE
This song is life ❤
This is officially the best day of my life 🥹
Mi novia se siente mal por una cirujia que tuvo que hacerse hoy y no pudo escuchar primera todas estas canciones, pero, en su honor me pidio que escuchara el regreso de Ariana ya que ella desde pequeña es una gran fan de ella y se que le emociona demasiado su regreso, aqui estoy mi amor escuchando esta bella cancion por ti y por el enorme cariño que sientes por Ariana que te hace sentir muy feliz y eso a mi me encanta demasiado ❤. Por ti Nicolle Alexia, el regreso de tu artista favorita ❤️.
díganmela en español porfavor😢😢😢
Just listened to the second song on your new album I like it so far up next to the other songs!
The peace she projects with her vocal ❤
❤❤
Este album es perfecto 😭
This song is life! ❤❤❤
U know , i love you
Serene vibes❤
Happy eternal sunshine
I'm telling y'all that's not a human being it's an angel send to us from heaven
Quee0n come back for
love and lovers 0❤
SHE'S NEVER A DISAPPOINRMENT GET YOUR GAME ARIIIII❤❤❤❤
MISS GRANDE YOU SERVED
SO LIKE SORRY to bother but ni hao im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
❤❤❤
this whole album is already giving me chills. CHILLS!!💘
Queen ❤❤❤❤🎉🎉🎉🎉🎉
Fuck, I cry all the album jajajaja
QUERÍAMOS MÚSICA, A REPRODUCIR!!
THIS IS MY FAV ONE OMG!!!!!
this is real😭😭😭😭
Ari for president
SWEETENER SUNSHINE 🧁🤍
''How could we know we'd rearrange all the cosmos''
Omg ariii 😭❤️❤️
This was my favorite without a doubt.
the queen is back
Vamos arianators
LLORANDOOOOOO😭😭😭
ayyyyyyyyy😢😢😢
Te amo mucho Ariana Grande 🩷✨💜
Art❤
❤@ri❤
You are perfect my queen
Llore ok
❤❤❤❤❤❤
A REAL MASTERPIECE
ONE OF MY FAVS
arianators let’s stream
ASÍ SE OYE LA PERFECCIÓN GENTE
THE QUEEN IS BACK BABY WOOO😊😊😊😊!!!! ARINAITORS FOR EVER❤❤❤❤❤❤
Te amo Ariana 😭
❤❤❤
A fan wasn't lying when he/she said that this album was a mix of emotions. I'm very nostalgic and happy for the release of ES at the same time 😢😢❤
mi psicólogo sabrá de esto
las lagrimas no paran, y solo tenemos 3, y este track por alguna razon saco mas emociones q ni sabia q existian en mi, todo a la vez
me encanta aunq lloré, aqui el culpable, gracias 2:00
THE ANGELLLLSSSSS 😭😭😭😭
Ariiiiiii
i might cry, this is too much 😭❤️
❤❤❤❤❤❤❤❤
SHAKING SCRWAMING CRYINF IM SO GRATEFUL I WAITED FOR THISS❤❤❤❤
Mother Earth here she come welcome 🙏
Oh Ariana this is my top 5
USE HEADPHONES EVERYONEHUHHUUHU
This album is super emotional and every song has a deep meaning
NI HAO from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Agreed
Omfg I just realized the initials of her album title are the initials of Ethan Slater
@Empressco nah man the initials are for eternal sunshine like wth
Estoy llorando 😭
My favorite ❤🎉😊
Amoooo ❤❤❤ Ariana ya lo esperaba
GUATEMALA LOVE YOU 😻❤️🔥
ES LA PRIMERA QUE ESCUCHO PERO YA ES MI FAVORITA, YA LA PEDÍ BAI
❤❤❤❤❤
She ate that
Alguien más está llorando ?
TE AMO ARIANA DIOS MIO, QUE OBRA DE ARTE
I think this is my fav onee
❤❤❤❤❤❤❤❤
In love with this song 😩
😭😭😭😭 ANG GANDAA❤❤❤
I remember my grandpa singing this song to me before he passed in 1987 this song brings tears of joy whenever i hear it ❤
Siento tan asdfghjklñ volverte a escuchar 🥹
I LOVE THIS ❤
te amo diosa
I love this sm
This will be number 1
My fav ❤❤❤😢😢😢
Я найду этот комментарий спустя 10 лет
Omg stop
Ari sings the song and Arianators feels the song, Ari raises her vocals and arianators levitate in the vastness of vocals, Ari laughs and Arianators Smile and giggle, Ari joys and Arianators enjoys 🥹❤️ Ari this is amazing, thank u from my bottom of my heart 💋🌞☁️🫧
Es que la hermosa voz de Ari 😻💗
MI CANCIÓN 😭😭😭
Amo como suena su voz en esta cancion💕
I can’t breatheeeeeeeeeee
I know her since I was 12 years old, now I’m 22 and I’m feeling like a child rn while hearing these masterpiece, literally I feel like I’m aliveeeeeee
Such an amazing feeling just by how her art has beeen present troughout my lifeeeeee😢😢😢❤❤❤
His voice is so angelic that it gives me so much peace. I LOVE HER❤❤
Wow wow awesome job again ariana grande wow
Si vooozzz❤❤
GOOOOO ARIIIIII
AHHHH LOVE YOU ARI
esse álbum não tem UMA música ruim, bom, a ariana n tem nem uma música ruim, simplesmente perfeita
IM CRYING😭😭😭😭
GOD OH MY GODDDDD❤❤❤
MY GHADDDDDDD I LUV IT
Love u ❤
El "imperfect for you" dios te amo eternamente ariana.
Ang gandaaa. 😭😭😭😭
❤️
MOTHER IS BACK
xDios ari, haces arte 😭
Es la hermana de pov?
Her vocals are heavenly❤
stream and buy the album ᡣ𐭩
i love u
Why these songs do short 😭
Ariana este álbum es lo mejor que me pudo pasar en la vida.
💋💋💋
😭❤️❤️
😭😭😭😭😭ahhaaaaa i love it ari
Weeeey 😭😭😭😭❤❤
Tô apaixonada
Ari❤
😔😭
Que bonito ❤️
Im in love with this song 💕😭
Me encantó muchisimo este tema!
my new faveeee! 😭🩷🩷🩷🩷
❤
indeed a masterpiece 🥹🩷
this is her speak up era ✨🔥
Awww
This song calms me so much🥹🤍
❤❤❤❤
i love this kind of soundddd sheesh 💋🥵
Ariana will forever remain a legend
GRACIAS POR DARLE SENTIDO A MI VIDA
❤
Preciosa Lovely
this is life
I love you
Öldüm ulan çok güzel
This is perfect
Gracias por tanto Ari, sos lo único que amo. Tu música me ayuda a superar todo, gracias por todo y perdón por tan poco... Te adoro Ariana Grandeeeee 😭💓💓💓
MULHER😭😭😭😭
I love it 💕
Infartando completamente 😢❤
FELIZ DIA DE ETERNAL SUNSHINE
Ariana slay ❤️🔥❤️🔥❤️🔥
Lenda
Omg 😲 this is it 😍
I love it
YALL THE VOCALS??? THE INSTRUMENTALS???? THE LYRICS??? GOD THIS GIVE ME THE CHILLS
Aún no empieza el segundo 1 y ya estoy llorando !!!
Avisa que Imperfect For You já é Hit
❤❤❤
QUE LINDO
Imperfectamente perfectos ❤️🔥
this album is making me lose my mind-
Perfectly imperfect ❤
The best album queen
AAAA ARI I CANNNTTNTNTNMT
😭😭😭😭😭😭
beautiful song❤
It was worth the wait, and I would wait for you all my life.
I'll hold your hurt in a box beside me just so sweet
❤️❤️❤️
I loved Ariana Grande 🫶🏻
I LOVE YOU ARIANA
It's perfect
I loved the way and the sound of the songs, she definitely wanted this album to be lighter in some way so the songs have a calmer feel
This is giving that “fuck you” song by eamon and frankee. When I listened I instantly tried to figure out the tune this song was giving. Correct me if I’m wrong please💜
There’s another song I’m thinking of that’s at the tip of my tongue but can’t put my finger on it and it’s killing me!
WAIT, hear me out, maybe a smidge of Katy Perry her Vegas song?!
bestie bestie! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Dude, same here… holy shit. This is already perfect but MY GOD her SNL performance of this song was phenomenal.
YESSSS
@stev6963 OMG FRRR
I covered imperfect for you, would love if you checked it out, thx! :)
I LOVE THIS
Love u ari 😢😢😢😢❤❤❤❤❤❤❤
Heyyyy
My godness shes really incredible, i was diynd for hear her álbum
This Is magiccccccc
😻😻
🥰🥰🥰🥰🥰🥰🥰🥰
Omggggggg
Te amoooo Ariii❤❤❤❤
QUE LINDA CANCIÓN MORI
I loved this one so much
Aaaaa!!
Te amooooo 🥹✨☀️
Sali llorando pq volviste mi ari pero ahora por mi ex ariana NAAAAUE TE AMO MI ARIANAAAA 😭😭😭
love you ari ❤
Voice as perfect 🥺❤
The beat in this is so beautiful it kinda country but lofi and pop at same time I love it and the lyrics are so true ❤
Por que isso me lembrou a Taylor?
Status: living to Ariana's songs
THIS IS SO PRETTY BRO😭🫶🏻🫶🏻
YOU ARE MY HOBBY
The chills😭😭!
AAAAAAAAAAAAAAAAAAAAAAAAAAAAAA
NEM ACREDITO Q TÔ VENDO ISSO
That "imperfect for you" was perfect for me 😭
Oh my good❤❤❤
sounds magical 😭😭🤍
ohhh this is so late night drive coded🥲
🥺❤️
I'M SHAKING TO THE BONES 😭😭
😭
OMG!!!! ARIANA PLEASE 7-7
Me encantaaaaa🥺🩷💋
TE AMOOOOOOOOOOOOOOOOOOOOOOOOOOOO
I LOVE YOUUU❤
YOU MADE MY GOT SENSE
Wowoowo❤dios
😭😭
This touch my soul
The Best❤️!
Iloveyouuuuuuuuu
After a drought, finally 😭💗
I love youu Ari 🤍✨
It gives me feel more chill. Hows beautiful 🩷
Oh myyy godd Ariana your's songs is so perfect and you
🩶🩶
THIS SONG HITS OMYGOD
OMG THIS SONG IS LITERALLY PERFECT and the “Imperfect for you” is just so clean and pretty
Wooow ❤ es es woooow ❤
I like this one ❤
Oh god this is so Beauty
A maior depois de yes, and!! Sim com certezaaaaaaa
I love this woman so much ❤❤❤❤
Couldn't of asked for better <3
🤍✨✨
CRYING RNNNN
Thank u ♥️ love u ♥️
needy but healed
I've been waiting for this album for a long time, I'm very sensitive, I love you, Ari. 🥹❤️
I can't believe It's actually happening rn
MOTHER SERVE
THIS IS PERFECT IM CRYING
Canciones muy tranquilas que dan mucha paz!! 🥺🥺💖🥰
oh hell yea MOTHER ate with this one 🫶🏼
Ya necesito la traduccion en español 😭😭
Okay but the ✨imperfect for you✨ just HEALED ME?!
3:01 te amo ariana y te apoyo toda mi vida
art❤
I love you. A+ your as fine as 999. Thank you 4 the music.
Como é bom ter ela novamente ❤
❤❤❤❤
Album of the year
THE MOTHER IS BACK 👑✨🖤 TE AMOOOOOO 🖤
Ariana is the best ♥️ with an angelic voice 🥲
Te amoooooo Ariana
guys i was literally refreshing the page as soon as the clock struck midnight 😭
Okyyy yess girll slayyy i love this songg
SHES BACKKK ❤
The “imperfect for you” sounds so hypnotising I’m in love
BEAUTIFUL
OMG🤌🏻🫂❤️🩹
Her Voice is so calming 😊😊😊❤❤❤
GATAUU SUMPAU BINGUNG, BAGUS BAGUSSS
GregaJohn-gb6ev ☠️☠️
Totally agree
Is this what they called butterflies in your stomach 🥰🤩
I feel so bad for you, two like beggers replied to you.
i know right??? her use of dissonance in this song is so beautiful
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
spiritualoutnowspiritual 5 OCTAVES??? WHATS UR RANGE OMG
@juliarolens it’s a bot
@thislovesposessingme oh 😔
I hate it so much, to the point that I can’t listen to it without being nervous or anxious. I need someone to fix it
I covered imperfect for you and would love if you checked it out, thx! :)
Hii guys , me and my cousin have a music covers channel can u please check this Ariana song cover we made , Tysm ❤ https://youtu.be/UqmQLv14f4k?si=U8c5l-MRanlnhM-H
Maybe
you use my voice and my mastering for the distributed version without responding to my hello on insta. You know only my friends can make money from my job but you ghost my hello and happy birthday after 6 years you ghost me you continue to hack my pc for robbed my voice and my mastering without hello and contract signed to do business. Please delete all my work on 7rings/stuck with you/34+35/positions/santa tell me/all tracks eternal sunshine Dj scrog remix to help you get back. now you and Mariah Carey are my enemy, you are not allowed to use my voice and my mastering for the buisness version because you never respond to my hello. you know I'm not interested by your money after 6 years, I help you for you Mariah's feat and your musical arrangement. you are killing our love for no, hello now i hate you. you kill my love for you goodbye forever. eternal goodbye
Yesss🥺
The way the chord changes itches my brain just right
I WAITED 3 YEARS FOR THIS 3 YEARS I LOVE HER SM
😢❤
❤❤❤
🎶🎶🎶
so beautiful....oh my gosh and the enunciation is so muchhh clearrrr
Te amo
✨✨✨
❤
:)
my new fav song
I am literally crying 😭 still can't believe it's that real ❤ thanks so much for this my dear Ari ❤❤❤❤
México te ama mucho ari ✨😭💗
Slightly eerie 🤩🔥🥴
AMEI ESSA ATE AGORA
💋💋💋💋💋💋
😭❤️
THIS SONG IS SO GOOD
this is definitely a masterpiece😩😩
REINAA! 😍
Te amo adriana grande
❤❤❤
Estoy en el cielo y un ángel me canta
STANNING ARIANA BEST CHOICE
She in her country bag omg
No puedo con lo feliz que me haces 😭😭
Ariana Grande's music is my therapy ♥️
TE AMOOOOOOOO GRACIAS POR VOLVERRRR
🥹🥹🤍
Ariana does not deserve hate at all
♡♡♡♡♡😍😍😍😍
😢llorando de emoción 😍
ariana fucking grande really did not come to play around this year !
Wahou this voice😢❤
🥹🥹🤍🤍🇧🇷🇧🇷🇧🇷
❤❤
Te extrañeeeee tantooo😢
THIS IS MUSIC, ART, HAPPINESS, EVERYTHING!!!!
The vibe is so her.
💫🩷
Me encata esta cancion
Omg 😭😭🫶🏻
Dolly Parton vibes.
❤❤❤❤
If you want real entertainment, Ariana's Youtube page is the place.
ARII
shet ❤❤❤❤
I love
Thank you Ariana Grande for this beautiful song
Omg I love this SONG
I ´M SO HAPPY
Ariana is the princess and she came to revolutionize pop with this new era❤❤❤❤❤
This is definitely one of my FAVORYIES
Finally new jewels to listen.
TE AMO TE AMO TE AML TE REAMOOOO
❤❤❤❤
BOTÓN PARA DECIRLE TE AMO A ARI 🩷
IM CRYING
I love You Ariana 😭❤
eu te amo
real
SO LIKE SORRY to bother but ni hao im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by THE ONE AND ONLY eternal sunshine and please support me in my music career xoxo
❤❤❤❤❤❤❤❤❤❤❤❤
I can't believe how beautiful is this😢
como vas a tener una voz tan linda te amo
I love you always forever Ari❤️
Omg I love this❤
Que bonita voz 🫵🏻
Te amo ariana grande ❤
IM CRYING HOW IS THIS SO GOOD
imperfect for you had to be one of Ariana's most delicate and most emotional songs to date. Her vocals perfectly accentuate the lyrics that run deep within her soul.
Comentários brasileiros aqui (o que acharam, amorecos?):
ariana is so talented! this album is perfect!
😍😍
It’s so interesting seeing her use her voice in different genres. Her voice can seriously work with anything
I love youuu ARIIIIII 💗💗💗💗
😭😭❤️
No wayyyyy! I'm just sobbing this is so good ❤🥹 This is the moment :-) Ari is the best, so amazing, so beautiful, so lovely 🌹
8 mars 2024🤘🏽😜😜😜
What a beautiful song, I love you so much. ❤❤
Mi amor ❤
THIS IS PURE GOLD OMF
Ariana Grande Will win world war three with this song
Ur pfp..... ITS AWESOME
r the different genres in the room with us?
ThatBacon_Shorts shut up
@allace7855 bahahaha I know they always say that lol people please learnt what difference between different sound and a whole GENRE
@allace7855 do I really have to explain to you lmao? Her last album was r&b and this song is completely different stupid
I lowkey she was gonna go rock for a second
@allace7855 listen to 34+35 or 7 rings and tell me if you hear the same genre as this song 😭
greetings from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
@allace7855 you dont hear the country and rock influences 😐
@TryingtoreachsubswithNovideos this album had pop, house and R&B lol what are you talking about
@boopboop7208 that’s literally the genres she’s been doing 😭😭
@k.c.4384 COUNTRY naw get out
@TryingtoreachsubswithNovideos different genres as in different types of genres… what’s not clicking
true!
I covered imperfect for you and more songs from this masterpiece album, would love if you checked it out, thx!😊
❤
6 minutes agooo!!!🎉
Ariana the best I love you 🤍
I swear ARIANA better win every award, every category, everything. She DESERVES THIS. This is Ariana' s year
La reina está de regreso
Her voice 😍😍😍😍😍😍
(´▽`).。o♡
Ariana will always be my queen
holy shit.
MY QUEEN IS BACKKKKK
Again I’m here cuz I used all my skips already
Omg, i'am cry ari
Literally I’m crying😭😭😭💖💖💖💖
😍😍😍😍😍😍😍
Dude this should be lead single
Omggg wawwww❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤❤
♥️♥️♥️♥️
WOWWWW
Siempre sirviendo arte❤
Ariana did it perfect!!
te amo más que a mi vida😭💘
Her voice is as angelic as Los Angeles 🤍🫧 she is totally beautiful
Omggg Ari 😭❤️
Hermosaaa❤️❤️
OMGGGGGGG ARIANAAAAAAA❤️🩹🫧😭💗
Mi reina
She is the best ❤
Save 2024 queen 👑
Teamooooooo
CRYING LITERAL TEARS
GRACIAS POR ESTO ARIANA TE AMO DEMASIADO ✨️✨️✨️✨️
Love her sm 😭
COUNTRY GURLSS
Omg, I can’t 🥹
I really have no words
I am crying this song is the best😭
I KOVE YOU ARIANA THANKS FOR COME BACK😭😭😭😭
WAITED SO LONG FOR THIS MOMENT!!🥹♥️
SHES BACK TO SAVE US😩❤️
Te amo Ariana Grande!! 🫧 ♡ desde 2020 empecé a escuchar tu música, cuando todo el mundo estaba paralizado, y escuchar tu voz era la única conpañia que tenía. cambiaste mi vida completamente y te lo agradezco. Atte: Mateo del año 2020 ♡🥹
¿quien más ama tanto a Ariana Como lo hago yo?
Omg yes she has! Her and Taylor r both slaying this year
she already did! I covered imperfect for you, would love if you checked it out, thx! :)
Agree
GregaJohn-gb6ev I DON’T CARE
GregaJohn-gb6ev FODASE 💕🇧🇷
yeah , it's the best of her on the album after true story .
i just cried too. i love this one.
she got me emotional
❤
❤
omg
Love u Ari💋
im crying rn
Im about to cry
are the intro vocals a mash up of her young vocals and current vocals or am i hearing things
My jaw is on the floorrrr this album is incredible im gonna cry the melodies are everythingg
🥺🥺🥺 ❤ Amooo✨
the best song off the album
POSITION ERA IS OVER
back from 4 years💛💛
❤❤❤❤
Jaw dropped from the first note. Holy fucking shit
ARIIII :(((❤❤❤❤
Nada é igual a ti
Amo esto 🤍
Soy unos de los primeros en hacer historia de eternal sunshine
❤❤❤❤😭😭😭
Te amo Ariana, fan desde la canción Love Me Harder. ❤
AHHHHH
Hello From Latam 🌎 ❤
It's giving somewhat alternate of nostalgia
I covered imperfect for you, would love if you checked it out, thx! :)
@arianzidaneofficial yesss
@RSpark awe sure I will!
AHHHH LOSING MY SHITTT
❤
POR DIOS 😭😭
Thank ariana ❤🎉
I'm crying 😭
happy eternal sunshine 😭😭😭😭
TE AMO TANTOOO😭😭😭😭
Beautiful asf 🔥
Ahhhh😊
Mmmhmmm
This feels like when a candle lights up the setting
Ariana grande, ahora que tendremos que decir gracias por darnos la oportunidad de volverte a escuchar 🎧
Im a big fan
Gracias ariana ❤
YALL WE MADE IT THROUGH THE DROUGHT
SO EXCITED 🧚🏻♀️🧚🏻♀️🧚🏻♀️🧚🏻♀️
SHE NEVER DISAPPOINTS!!!!
Es bellísimo
im love it❤❤❤❤
I love you ariana grande ❤
🎉
🎉🎉🎉
Yesss
SKIPLESS ALBUM!!
and also to you 🙏
0:24 I love thatttt! ♥️😭
sry to bother but heyyy im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
I covered imperfect for you, would love if you checked it out, thx! :)
I love you 🥹
Tour time!
Eres la razón de mi vida ❤🌈🇪🇨
❤❤❤❤
Ari siempre dándonos Arte ❤
OMGG
ARIANA QUE ESTA PASANDO
I WAITED FOR THIS NOT DISAPPOINTED ♾️☀️✨💕
TU QUE ESTÁS LEYENDO ESTO VAS HACER EXITOSO YO CREO EN TI🙏🏾….
"We crashed and we burned
Now i just cant stop go where you go"
❤❤❤
Fooor youuuu
NUNCA ME HABIA EMOCIONADO TANTO POR DIOS AHHHHHH!!!!
i will work on a song with Ariana Grande one day, speaking it into existence 🙏🏼
❤️❤️
OMGGG
OMGGG
😭❤️❤️
Let's get this album to #1
AHHHH 11: 03❤❤❤
Thank You ✨
YESSS
LIGHT WORK
It's ari ofc it's gonna get #1😭
i like how you think :) I covered imperfect for you, would love if you checked it out, thx! :)
WOWWW
Primeiro
TE AMO
The “imperfect for you” gives me chills
was lit reseting the channel these last minutes
aaaahhhhh❤
😍😍😍😍
THIS IS AMAZING OMFGGG AHHHH
AMOOO
ARIANA THE BESTTT
Estoy llorando!!! No puedo ❤
❤❤❤
PERFEITA ❤
Amor de mi vida te amooo❤️
PROUD MOM 💗💗
Yuh
❤❤❤
lindaa!!te amo bb🌼💞💅
ГОСПОДИ ДА
OMG I LOVE YOU, ANGEL!❤️🩹
Me encantó ❤
Yess
Righttt
LITERALLY
Why does it sound SO familiar and heavenly?? 😭
@saniyaj4631 RIGHT I feel like I've heard something like this before??😭
@saniyaj4631 it's straight out of disney
OKOK HEY hey there, im a 13 year old male artist from hk with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Sounds so beautiful😍
There's not any key change. It just uses chords from other scales,
Idk why but it gives me alien abduction vibes 🛸
I covered imperfect for you and would love if you checked it out, thx! :)
That's why it's is on top of my top 3
Frrrr😊
Yeessss
how in a good way or in a bad way😡😠
@Rosie-zw6yk why are you mad
IT ACTUALLY DOES THOUGH NOT EXAGGERATING I ACTUALLY HAVE GOOSEBUMPS ITS TOO GOODDD
I love it ❤
❤
❤️
ATEEEEE
❤️🩹😭
TE AMO ARI❤❤❤
OMG I LOVE YOU SO MUCH
PERFECT ❤❤
😍😍😍😍
oh god it happened :face-red-heart-shape:
❤❤❤
AHHHHHHHHH
EU TO CHORANDO
Omg
omg ariana😭😭😭❤
queeeeeeeeeeeeeeeeeeeen
I love you Ari
LITERALLY IM DIYING
i love yooou!!
❤
❤❤❤
Slayy
te amo eres lo mejor de mi vida mi niña talentosa❤
AAHHHHHHHH
รักอาริ❤
MOTHER
I LOVE YOUUUU ARIANAAAA 😭😭💗💗💗🫧💗🫧💗😭🫧😭💍
Omggg This is perfect
LIKE IF YOU LOVE HER
❤
Love!
Omg ariiiii ahhhh❤❤
❤❤❤
♡
stanning ariana grande isn't just a hobby, it's a lifestyle, a reason to breathe, an escape from this cruel world filled with thieves. it's art <3
QUEEN
Omg
omg
Amoo
Amo tu música, Ari♥️
Don't translate...
भवतः हृदयस्य धड़कनं कतिपयेषु घण्टेषु स्थगयिष्यति, अस्य शापस्य मुक्तिं प्राप्तुं एकमात्रं मार्गं मम चैनलस्य सदस्यतां कुर्वन्तु………..
WORD
u gonna comment that thing in every damn video
Same😢 ❤
bro stop spamming dude all of u guys (original comment and first three replies) no one is buying ur "professional camera"
❤
Bro wtf??? @DJILMarioBros_
Frr❤❤❤
@DJILMarioBros_ 😂😂😂😂😂😂 LOL
Dude is tryna get likes and attention for commenting on every song she posted 😭😭😭🤦🏼🤦🏼🤦🏼😭🤦🏼
Copy
My sister literally took off work for this lol
FACTS
@physicaljenner LMAOA
Sheesh lol
Absolutamente cierto, no lo pudiste decir mejor.
Its a must
WBK
bestie bestie! hi from hk, im a 13 year old male artist with a 5 octave range including whistles and baritone notes, check out my track 'remove me' its inspired by eternal sunshine and please support me in my music career xoxo
Get a fcking life dude
she is an amazing gemini cancer cusp
@DJILMarioBros_ tf same comment in every video
get a life jesus christ
@DJILMarioBros_ ur everywhere and no one. actually. cares.
for realness
This has to be a joke
Its joblessness
THIS
@DJILMarioBros_ man
@physicaljenner right
calm down, she doesnt even know you exist lmaooo
I’ve never related to an Artists emotions like I do with Ari .. crying screaming can’t breathe obsessed can’t stop thinking about you levels of obsession.
ILYSM ARIIII
MEU DEUS
The number 1 fan here present!!!!❤❤❤
I lovedddddd❤❤❤❤❤❤❤❤❤❤❤
❤❤
I love you 💞😭
"voice as perfect as heaven and as painful as hell"
First❤❤❤
HAPPY ETERNAL SUNSHINE
ARIANA I LOVE YOU ❤️
I'm first
Beautiful
FIRST
If you ain’t here from Tiktok, you are allowed to like this comment..
😍😍
BollywoodNewzz can you stop commenting the same thing over and over
BollywoodNewzz doing that doesn’t make me want to support you
@snowome i know I didn’t tag you correctly but idc, I LOVE WHAT YOU SAID 🥺♥️
@aniyahhxoxo fr it's on all of the eternal sunshine songs that just released
@arianzidaneofficial YES
@aniyahhxoxo kiss 🥹✨💖💖
Tip
But, while knowing English is clearly an advantage to songwriters and producers seeking success in the U.S. and the U.K., a lack of facility with the finer points of the language is equally important. Swedish writers are not partial to wit, metaphor, or double entendre, songwriting staples from Tin Pan Alley through the Brill Building era. They are more inclined to fit the syllables to the sounds—a working method that Martin calls “melodic math”—and not worry too much about whether the resulting lines make sense. (The verses in “I Want It That Way,” for example, completely contradict the meaning of the chorus lines.) Fans of Cole Porter may see this development in roughly in the same spirit that “Downton Abbey” fans might view “Keeping Up with the Kardashians”—with horror—but one can argue that this very freedom from having to make sense lyrically has allowed the Swedes to soar to such melodic heights.
import requests
from bs4 import BeautifulSoup
# Replace with the URL you want to scrape
url = 'https://www.newyorker.com/culture/cultural-comment/blank-space-what-kind-of-genius-is-max-martin'
# Send a request to the website
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# Example: Find all paragraphs
paragraphs = soup.find_all('p')
for paragraph in paragraphs:
print(paragraph.text)
else:
print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
Find anything you save across the site in your account
Among the stranger aspects of recent pop music history is how so many of the biggest hits of the past twenty years—by the Backstreet Boys, ’NSync, and Britney Spears to Katy Perry, Taylor Swift, and the Weeknd—have been co-written by a forty-four-year-old Swede. His real name is Karl Martin Sandberg, but you would know him as Max Martin, if you know of him at all, which, if he can help it, you won’t. He is music’s magic melody man, the master hooksmith responsible for twenty-one No. 1 Billboard hits—five fewer than John Lennon, and eleven behind Paul McCartney, on the all-time list. But, while Lennon and McCartney are universally acknowledged as geniuses, few outside the music business have heard of Max Martin.
Presumably this is because Martin writes all of his songs for other people to sing. The fame that Lennon and McCartney achieved by performing their work will never be his, which no doubt is fine with Martin. (He still gets the publishing.) He is the Cyrano de Bergerac of today’s pop landscape, the poet hiding under the balcony of popular song, whispering the tunes that have become career-making records, such as “… Baby One More Time,” for Britney Spears, “Since U Been Gone,” for Kelly Clarkson, and “I Kissed a Girl,” for Katy Perry. The songs he co-wrote or co-produced for Taylor Swift, which include her past eight hits (three from “Red” and five from “1989”), transformed her from a popular singer-songwriter into a stadium-filling global pop star. (The “1989” tour recently passed the hundred-and-fifty-million-dollar mark.)
Martin has thrived in the ghostwriter’s milieu, where the trick is to remain as anonymous as possible, because the public likes to believe that pop artists write their own songs. That the Swede happens to bring to the table an exceptionally large dollop of Jantelagen, the Scandinavian disdain for individual celebrity, makes him especially well-suited to his vocation.
Still, even for a Nordic, it is a powerful act of self-denial to forego the pleasure (and, yes, the fame and attendant adulation) of recording your own songs, and give all your beautiful tunes to other people, who become famous instead. This path is especially difficult when you possess a beautiful singing voice of your own, which Martin does. As one of his early collaborators, the Swedish artist E-Type, says in “The Cheiron Saga,” a 2008 Radio Sweden documentary about Martin and his former colleagues at Cheiron Studios, in Stockholm, “With his own demos, Max Martin singing himself, those would have sold ten million or more, but he wasn’t an artist; he didn’t want to be an artist.”
And yet Martin is known to insist that the artists he works with sing his songs exactly the way he sings them on the demos. In a sense, Spears, Perry, and Swift are all singing covers of Max Martin recordings. They are also among the few people in the world who have actually heard the originals. Countless self-proclaimed performers on YouTube sing Max Martin songs, but there is not a single publicly available video or audio recording of Martin performing his own stuff. (In the course of researching my book “The Song Machine,” I got to hear an actual Max Martin demo, for “… Baby One More Time,” when a record man who had it on his phone played it for me. The Swede sounded exactly like Spears.) Martin’s demos are the missing originals of our musical age—the blank space at the center of the past two decades of pop music.
Sandberg was born in Stenhamra, a suburb of Stockholm, in 1971. His father was a policeman. He later recalled the handful of recordings his parents had in their collection: Elton John’s “Captain Fantastic,” the best of Queen and Creedence Clearwater Revival, “the Beatles one where they look down from the balcony,” Vivaldi’s “Four Seasons,” and Mozart’s “Eine Kleine Nachtmusik.” Put them all altogether and you've got Max Martin.
Sandberg’s older brother was a glam-rock fan, and “he brought home old Kiss cassettes,” Sandberg recalled, in a 2001 interview in Time magazine, which was the first and last time he took part in an English-language profile. Listening to those tapes made young Karl Martin Sandberg want to be a rock star. “I was a hard rocker at that time, and listened to nothing but Kiss,” he told the Swedish documentarian Fredrik Eliasson, in a follow-up to “The Cheiron Saga” that ran on Radio Sweden earlier this year. “I mean, nothing but Kiss. It was like we belonged to a cult: if you listened to anything else, then in principle you were being unfaithful.”
Sandberg learned music through Sweden’s excellent state-sponsored music-education programs, receiving free private lessons in the French horn. (Thirty per cent of Swedish schoolchildren go to publicly funded after-school music programs.) “I first began with the recorder in our community music school,” he remembered in a Radio Sweden interview. “After that I played horn, and participated in the school orchestra. I remember that I started playing brass not so much because I had a calling but because I thought it looked cool.” Eventually, he moved on to drums, then keyboard. He has credited Sweden’s musical-education system with his success, telling Eliasson, “I would not be standing in this place today if it weren’t for the public music school.”
In the mid-eighties, Sandberg became the front man and main songwriter of a glam-metal band called It’s Alive, adopting the stage name Martin White. In the video for the group’s song “Pretend I’m God,” White plays Jesus and enacts a pseudo-crucifixion, doing his best Ozzy Osbourne imitation. While the song must be considered a work of juvenilia, it does at least explain why eighties metal seems to lurk beneath the surface of so many of today’s pop hits.
But Sandberg had a terrible secret, one that he couldn’t share with the rest of the band. He loved pop music. At home, he listened to Depeche Mode’s “Just Can’t Get Enough” and the Bangles’s “Eternal Flame,” which he later told Time was an all-time favorite. “I couldn’t admit to my friends that I liked it,” he said.
In 1994, he met his mentor, a Swedish d.j. who called himself Denniz PoP and co-founded Cheiron Studios. (His real name was Dag Krister Volle; friends called him Dagge.) Denniz realized that Sandberg’s talents lay in songwriting, not performing, and showed him how to use the studio. Denniz was coming off the major hits he had produced for Ace of Base, “All That She Wants” and “The Sign”; one of Sandberg’s first production credits was on “Beautiful Life,” the group’s final hit. By then his mentor had renamed him Max Martin, a drab, forgettable disco name that is almost as bad as Denniz PoP.
Unlike Denniz, who neither wrote nor played music, Martin knew music theory and musical notation. “Martin was very schooled; he could read the notes, write partitions, and do musical arrangements,” E-Type says in “The Cheiron Saga.” “Dagge would say, ‘We need a new influence, so Martin, make us something pretty while E-Type and I run out for some sushi.’ We’d come back and find something so gorgeous that we both almost fell backwards.”
Martin worked by theory, Denniz by feel. “Dagge was driven by his instincts,” E-Type says in the documentary. “If there was something that worked, well, then, that’s what he’d do, always. Martin was the musician, and he got the principles around funk, and with those abilities was able to take it a step further.”
That next step was presented by a boy band that was unknown at the time, the Backstreet Boys. The songs Martin wrote for them, including “We’ve Got It Goin’ On,” “Show Me the Meaning of Being Lonely,” and the timeless “I Want It That Way,” made the group world-famous. They also created a template for the Max Martin sound, which combines ABBA’s pop chords and textures, Denniz PoP’s song structure and dynamics, eighties arena rock’s big choruses, and early-nineties American R. & B. grooves. On top of all that is Sandberg’s gift for melody, which owes as much to Edvard Grieg’s dark Norwegian musical fable “In the Hall of the Mountain King” (a.k.a. the “Inspector Gadget” theme song) as to any contemporary influence. Like many of ABBA’s tunes, these Backstreet Boys songs use major and minor chords in surprising combinations (going to a minor chord on the chorus, say, when you least expect it), producing happy songs that sound sad, and sad songs that make you happy—tunes that serve a wide variety of moods.
Perhaps the greatest advantage that Sandberg and his Swedish colleagues enjoyed was their relative freedom from the racial underpinnings of the long-established American distinction between R. & B. and pop. Rhythm and blues, a term coined by the Atlantic Records co-founder Jerry Wexler, back when he was a writer for Billboard, in the fifties, replaced the frankly racist category of “race records,” but the underlying race-based distinction remained: R. & B. was music by and for black people, whereas white performers were “pop,” even if their music was heavily indebted to R. & B. A white American songwriter composing R. & B. tunes was not likely to get very far on the balkanized pop-music scene in the U.S., but a Swedish writer, free of the racist legacy of the R. & B./pop dichotomy, could create music that combined both, and that is just what Martin has done. The resulting hybrid, one could argue, has become the mainstream sound on Top Forty radio today. SiriusXM’s recent rebranding of its pop channel, Venus, which now plays “rhythmic pop” (R. & P.?), is just one measure of this Swedish-led transformation.
The Backstreet Boys were on Jive Records, the label founded by the reclusive South African record man Clive Calder, who is, and for the foreseeable future will be, the richest man the record business has ever produced. (He cashed out of Jive and Zomba, his publishing company, for close to three billion dollars, in 2002.) So when, in 1997, Jive signed a young girl named Britney Spears and was looking for danceable pop songs, it naturally thought of Max Martin.
As it turned out, Martin had a song for Spears. He had composed it with Rami Yacoub, a Swedish-Moroccan beatmaker who was part of the Cheiron team. The song, initially called “Hit Me Baby (One More Time),” had been written for TLC, the three-woman R. & B. group. When Martin sent TLC a demo, which featured the Swede doing four-part harmonies all by himself, the trio had rejected it. Years later, T-Boz, the leader of the group, recalled the decision in a MTV interview: “I was like, I like the song, but do I think it’s a hit? Do I think it’s TLC? ... Was I going to say, ‘Hit me, baby, one more time’? Hell, no!”
“Max, at that point in his career, thought he was writing an R. & B. song,” Steve Lunt, the Jive A. & R. man who was assigned to the Spears project, told me. “Whereas, in reality, he was writing a Swedish pop song. It was ABBA with a groove, basically.” There is a funky bass slap in the song that sounds urban, and on the demo Martin does that cowboy-sounding “owww” made famous by Cameo and beloved by Denniz PoP. “But all those chords are so European, how could that possibly be an American R. & B. song?” Lunt continued. “No black artist was going to sing it.” He added, “But that was the genius of Max Martin. Without being fully aware of it, he’d forged a brilliant sound all his own, and within a few weeks every American producer was desperately scrambling to emulate it.”
When TLC rejected the song, Martin offered it to Robyn, the Swedish artist, but nothing came of that, either. After meeting Spears in New York, he went back to Stockholm, worked on the song a little more to tailor it for Spears, made a copy, and mailed it to Jive. (Although his career as a performer was over, Martin still looked like Martin White, the hirsute glam-metal front man, and his appearance initially alarmed Spears, who said, “I thought he was from Mötley Crüe or something.”) All the hooks in the song were worked up to their finished state, but most of the verses were unfinished, often mere vowel sounds. There was no bridge yet, because, as Lunt put it, “Max would say, ‘If you don’t like the song by then, fuck you’—in his polite Swedish way, of course.” When the demo reached Jive, everyone thought, “Holy shit, this is perfect,” according to Lunt.
“Hit Me Baby (One More Time)” is a song about obsession, and it takes all of two seconds to hook you, not once but twice, first with the swung triplet “Da nah nah” and then with that alluring growl-purr that Spears emits with her first line (following Martin’s trace vocal): “Oh, baby, bay-bee.” Then the funky Cheiron backbeat kicks in, with drums that sound like percussion grenades. Next comes Tomas Lindberg’s wah-wah guitar lines, which signal to one’s inner disco hater that it can relax: it’s a rock song, after all.
And yet the vocal hook, irresistible as it was, sounded odd. You weren’t sure it was O.K. to sing it out loud. It was hard to imagine that anyone forwhom English is a first language would write the phrase “Hit me, baby” without intending it as an allusion to domestic violence or S & M. That was the furthest thing from the minds of the gentle Swedes, who were only trying to use up-to-the-minute lingo. Jive, concerned that Americans might get the wrong idea, changed the title to “... Baby One More Time.”
The song was Martin’s first Billboard No. 1. “I don’t really think we understood what we had done,” he says in “The Cheiron Saga.” “I actually remember that specific moment; I remember sitting in the studio when they called me to let me know that my song had made No. 1 in the U.S.A. And that was incredible, but I also remember that I had so much going on with everything else right then, I didn’t really grasp the meaning of it.”
Although Martin may be sui generis, he is by no means the only disciple of Denniz PoP to go on to chart-topping success. Others, including Andreas Carlsson, Jörgen Elofsson, and Per Magnusson, also have long track records of hits; they’ve been especially successful with U.K. boy bands. According to Marie Ledin, the managing director of the Polar Music Prize, Sweden’s musical Nobel, Swedish songwriters and producers were partly responsible for a quarter of all Billboard Top Ten hits in 2014, an astonishing accomplishment for a country of fewer than ten million people. Clearly, there is more at work here than individual genius. Apart from the country’s musical-education system, what qualities and characteristics make Swedes so good at producing pop songs?
Generally speaking, there is a flowing melodic element in Swedish folk songs and hymns (the national anthem, “Thou Ancient, Thou Free,” even sounds a bit like a pop song) that has rooted itself in the sensibility of many a musical Swede. More specifically, the relative computer literacy of the population, combined with the country’s excellent broadband infrastructure, has allowed Swedes to excel at making music on computers, and collaborating with other composers over the Internet, which has become the standard method of pop songwriting today. Added to that is Sweden’s xenophilia—its love of other cultures, in particular Anglo-American cultures. In Sweden, American TV isn’t translated into the local language, as it is in France and Italy, say, and the music you hear on the radio is more likely to be sung in English than Swedish. More than ninety per cent of Swedes speak English.
But, while knowing English is clearly an advantage to songwriters and producers seeking success in the U.S. and the U.K., a lack of facility with the finer points of the language is equally important. Swedish writers are not partial to wit, metaphor, or double entendre, songwriting staples from Tin Pan Alley through the Brill Building era. They are more inclined to fit the syllables to the sounds—a working method that Martin calls “melodic math”—and not worry too much about whether the resulting lines make sense. (The verses in “I Want It That Way,” for example, completely contradict the meaning of the chorus lines.) Fans of Cole Porter may see this development in roughly in the same spirit that “Downton Abbey” fans might view “Keeping Up with the Kardashians”—with horror—but one can argue that this very freedom from having to make sense lyrically has allowed the Swedes to soar to such melodic heights.
Finally, while Sweden has a strong songwriting culture, it lacks an equally strong culture of performing. Klas Åhlund, a successful Swedish songwriter and producer in his forties, who is also a performer (in the rock band Teddybears), told me, “Swedes are very musical, and they love to write songs. But it’s a big country, and it has very few people in it. So you had these farmers out there who were good at writing songs but had no one to sing them. Songwriting was just a thing you did on your own when you were watching the cows, a kind of meditation. You didn’t focus as much on your ability as a performer as you did on the structure and craft of the songs. Which is really not the case in the U.S., where your charm and your voice and your powers as a performer come immediately into play.”
A nation of songwriters endowed with melodic gifts, meticulous about craft but reluctant to perform their own songs, is a potential goldmine for a nation of wannabe pop stars who don’t write their own material, which is often the case in the U.S. By hooking up the two countries, musically speaking, Martin and his peers changed pop songwriting.
Martin’s legacy must be measured not only by the number of hits that he and his Swedish colleagues have created but also by the songwriting methods that they have instilled around the world. (Many K-pop songs are the result of collaborations between Korean and Swedish songwriting teams.) A strong part of Denniz PoP’s vision for Cheiron was that songwriting should be a collaborative effort; no one was supposed to be proprietary about his work, and Martin has passed these principles on to two generations of songwriters. Songwriters are assigned different parts of a song to work on; choruses can be taken from one song and tried in another; a bridge might be swapped out, or a hook. Songs are written more like television shows, by teams of writers who willingly share credit. In a Swedish TV documentary called “The Nineties,” E-Type describes the working conditions at Denniz PoP’s studio. “I get this feeling of a big painter’s studio in Italy, back in the fourteen-hundreds or fifteen-hundreds. One assistant does the hands, another does the feet, and another does something else, and then Michelangelo walks in and says, ‘That’s really great; just turn it slightly. Now it’s good; put it in a golden frame, and out with it. Next!’ ” The description might apply equally well to Martin’s home studio, in Los Angeles (Frank Sinatra once lived there, and he sublet the pool house to Marilyn Monroe), where he is now the master.
Lennon and McCartney wrote almost all of their hits with each other, and while they had assistance from others in their solo careers, the level of collaboration was nothing like their former partnership. Martin, on the other hand, has consistently sought out new, fresh collaborators when the heat of a previous partnership begins to cool, which is why his magic touch has lasted longer than even Sir Paul’s. These protégés—Dr. Luke is the best known, but by no means the only one—often go on to be major hitmakers in their own right, and acquire and mentor their own protégés, who become hitmakers, too, spreading Swedish methods further and further into the songwriting mainstream. Martin is their Obi Wan.
And yet, for all his success and influence, there is something missing in Martin’s oeuvre, when compared to the Beatles’s. It’s not the quality of the songs—history will judge whether they have what it takes to endure. It’s the absence of a broader political and cultural framework in which to place the songs. The story of the Beatles, from “I Want to Hold Your Hand” to “Let It Be,” is a story of the sixties—politics, war, protest, drugs, free love, and how the songwriters responded to those forces. The hits are embedded within albums that offer rich, complex musical statements, and insights into the artists’ personal development and changes. What story does Martin’s string of No. 1s tell, from “... Baby One More Time” to “Can’t Feel My Face,” his most recent? What changes do they trace? The songs are all about the same thing, more or less, which is not the same thing at all.
Sections
More
© 2024 Condé Nast. All rights reserved. The New Yorker may earn a portion of sales from products that are purchased through our site as part of our Affiliate Partnerships with retailers. The material on this site may not be reproduced, distributed, transmitted, cached or otherwise used, except with the prior written permission of Condé Nast. Ad Choices