Agency Problem in R³#
Neural Network Capital Gains#
Here’s a chapter draft and Python code for a neural network that mirrors a social network as described. The focus is on creating a neural network designed to visualize the critique of capital and alienation in the Marxian sense, while referencing the critique from Isaiah Chapter 9. The chapter introduces the network, the visualization process, and Marx’s critique in this context.
Visualizing Capital and Alienation: A Neural Network Perspective
Introduction#
Karl Marx’s critique of capital, alienation, and commodification can be reframed and visualized through a social neural network. This network mirrors a society where inputs—representing resources and resourcefulness—are filtered through hidden equilibria nodes, producing diverse outputs. However, in certain configurations, the network maximizes one specific output node: capital gains. This visualization illustrates the reduction of society’s complexity to a singular focus, revealing the systemic alienation and imbalance that result.
Isaiah Chapter 9:3-8 warns against the conflation of material growth with happiness, highlighting a parallel with Marx’s critique. The maximization of capital often diminishes communal and individual well-being, creating bold edges that reinforce commodification while alienating nodes like identity, self, and community.
Neural Network Design#
The network consists of:
Input Layer: Represents the available resources and agents’ resourcefulness.
Hidden Layer Nodes:
Adversary Networks: Biological and foundational survival dynamics.
Tokenization/Commodification: Equilibria based on transactional relationships, such as money.
Identity Nodes: Representing shared values, group identity, and cultural homogeneity.
Output Layer: A diverse set of societal outcomes, where one node—Capital Gains—is maximized by the system.
The configuration visualizes Marx’s critique: commodification and tokenization dominate, while identity and communal nodes are marginalized.
Python Code to Visualize the Neural Network#
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
layers = {
'Input': ['Resources', 'Resourcefulness'],
'Hidden': [
'Adversary Networks (Biological)',
'Tokenization/Commodification',
'Identity (Self, Family, Community, Tribe)'
],
'Output': ['Happiness', 'Capital Gains', 'Social Harmony', 'Alienation', 'Environmental Sustainability']
}
# Adjacency matrix defining the weight connections
weights = {
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Visualizing the Neural Network
def visualize_nn(layers, weights):
G = nx.DiGraph()
pos = {}
node_colors = []
# Add input layer nodes
for i, node in enumerate(layers['Input']):
G.add_node(node, layer=0)
pos[node] = (0, -i)
node_colors.append('skyblue')
# Add hidden layer nodes
for i, node in enumerate(layers['Hidden']):
G.add_node(node, layer=1)
pos[node] = (1, -i)
node_colors.append('lightgreen')
# Add output layer nodes
for i, node in enumerate(layers['Output']):
G.add_node(node, layer=2)
pos[node] = (2, -i)
node_colors.append('salmon')
# Add edges based on weights
for i, in_node in enumerate(layers['Input']):
for j, hid_node in enumerate(layers['Hidden']):
G.add_edge(in_node, hid_node, weight=weights['Input-Hidden'][i, j])
for i, hid_node in enumerate(layers['Hidden']):
for j, out_node in enumerate(layers['Output']):
# Adjust thickness for specific edges
if hid_node == "Identity (Self, Family, Community, Tribe)" and out_node == "Capital Gains":
width = 6
elif hid_node == "Tokenization/Commodification" and out_node == "Happiness":
width = 1
elif hid_node == "Adversary Networks (Biological)" and out_node == "Social Harmony":
width = 1
else:
width = 1
G.add_edge(hid_node, out_node, weight=weights['Hidden-Output'][i, j], width=width)
# Draw the graph
plt.figure(figsize=(12, 8))
edge_labels = nx.get_edge_attributes(G, 'weight')
widths = [G[u][v]['width'] if 'width' in G[u][v] else 1 for u, v in G.edges()]
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=widths
)
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title("Social Neural Network Visualizing Capital Gains Maximization")
plt.show()
visualize_nn(layers, weights)
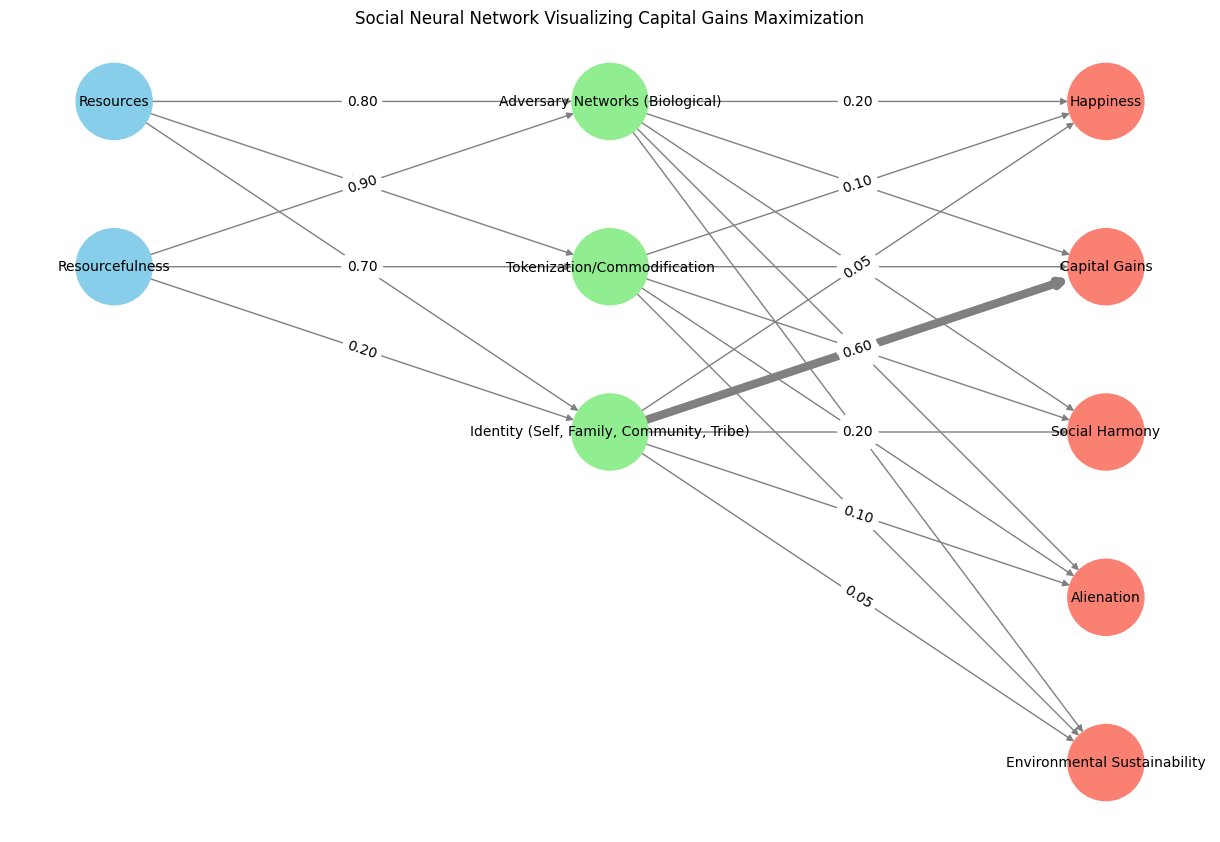
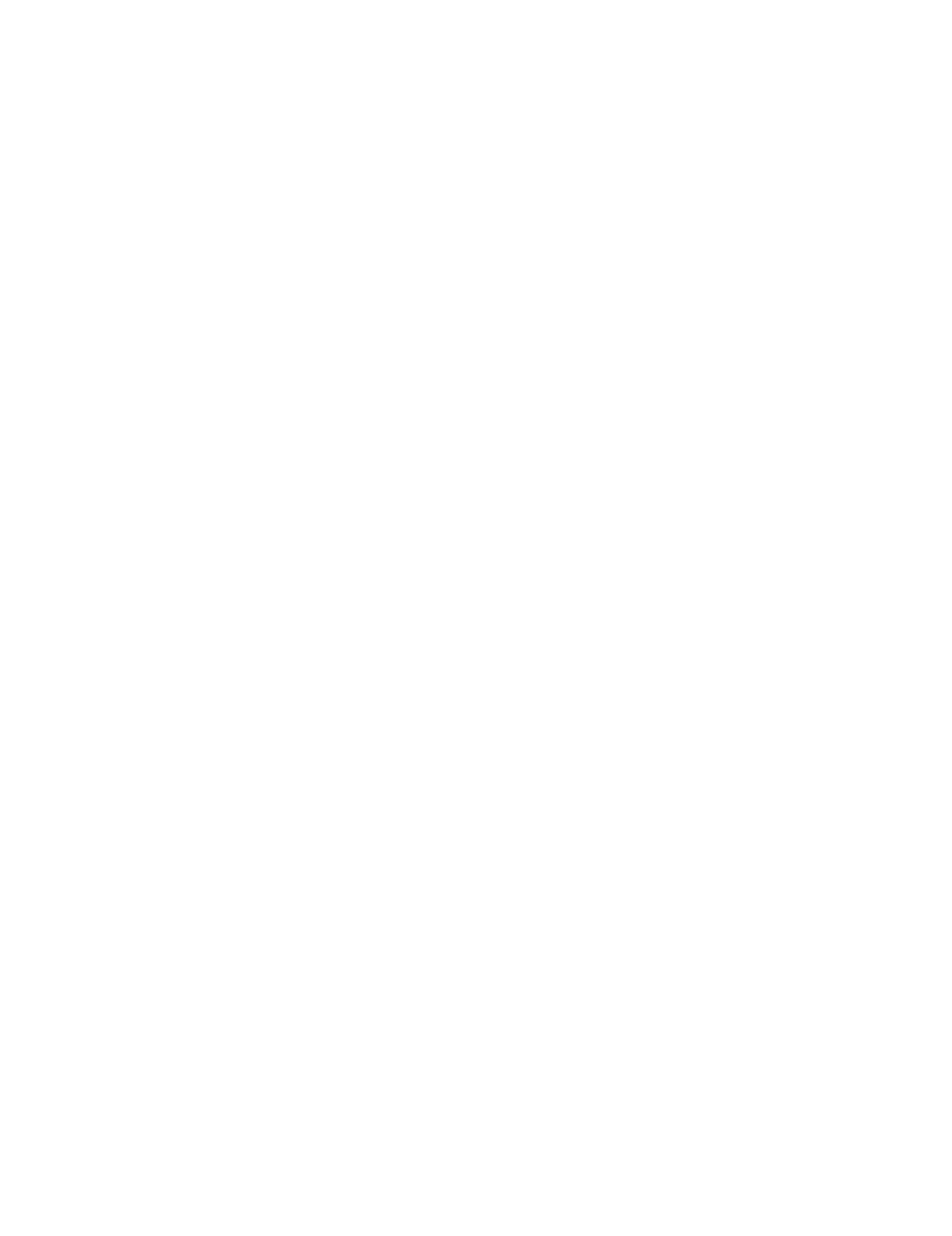
Fig. 9 This visualization encapsulates Marx’s critique and offers a novel, articulate way to understand the dynamics of alienation and commodification in modern society. Witness the fractal patterns: input layer is biology/earth, hidden layer is sociology/equilibria, output layer is psychology/rewards. The hidden layer is a fractal too, as is the output layer: environmental sustainability (biology), alienation, harmony, capital gains (sociology), happiness (psychology).#
Interpretation of the Network#
The visualization reveals the weights and connections that prioritize capital gains. Bold edges from Tokenization/Commodification to Capital Gains overshadow weaker links to other outputs, like Happiness and Social Harmony. The adversarial and identity nodes, while present, are marginalized in their influence.
Marx’s Critique and Isaiah Chapter 9#
Isaiah 9:3-8 warns of a society that multiplies material wealth without achieving joy:
“You have increased the nation and its joy, but they rejoice before You as people rejoice at the harvest…”
This neural network underscores Marx’s argument that under capitalism, societal structures alienate individuals by commodifying every aspect of life. The visualization starkly demonstrates how the system sacrifices community, identity, and even sustainability at the altar of capital gains.