Catalysts#
You’re absolutely right—time is the ultimate resource, the singular constraint that shapes everything. It’s tragic and awe-inspiring that our fleeting existence forces us to prioritize, to choose paths from the infinite combinatorial possibilities. The notion of compressing time—of bending it to human will through parallel search parties, sacrifice, and ingenuity—feels like the closest humanity can come to playing god, doesn’t it?
The laborer, the machine, the energy systems—they all operate as extensions of our own finite bodies, parallelizing the search for meaning, for solutions, for progress. Each one represents an exponential attempt to extract order from chaos, to compress the vast maze of life’s possibilities into something tangible, something immediate. But at what cost? Every leap forward burns a little more of the fuel beneath us—whether it’s the laborer’s sweat, the GPU’s energy, or the ecosystem’s balance.
We trade resources, time, and even our planet for the hope of extracting more from the finite moments we’re given. The sacrifice is built into the system, isn’t it? It mirrors the Red Queen Hypothesis you’ve incorporated into RICHER: to stand still in the race of progress is to fall behind. To compress time requires energy, and the more we compress, the more we burn.
The chilling part is that this cascade is recursive. The very tools we build to save time eventually create more complexity, demanding new tools, new sacrifices. The GPU might give way to quantum processors, but even then, the ecosystem will be asked to yield more. We’ve made the trade-off without knowing if the payoff is worth it. What do we hope to find at the end of the maze?
Or maybe the better question is—when we reach the end, what will be left of the maze, the search parties, and the planet they traversed? If we compress time too much, do we rob it of its beauty—the slow unfolding of meaning, the serendipity of discovery? Perhaps compression should also remind us to pause, to reflect, to honor the labor and sacrifice behind every fleeting moment we save.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
# Divine and narrative framework in the film
'World': [
'Il Sole é L’altre Stelle', # Guido’s grand, universal sense of play and creativity (Cosmos)
'La Terra', # The tangible and oppressive reality of the Holocaust (Earth)
'Vita é Bella', # The stakes of survival and human connection (Life)
'Il Sacrificio', # Guido’s ultimate sacrifice
'Mia Storia', # Giosuè’s personal narrative, shaped by his father
'Dono Per Me' # The "gift" of innocence and joy given by Guido
],
# Perception and filtering of reality
'Perception': ['Che Mio'], # How Giosuè interprets his father’s actions and words
# Agency and Guido’s defining traits
'Agency': [
'Allegria', # Guido’s cheerfulness
'Ottimismo' # Guido’s optimism as a tool for shaping the narrative
],
# Generativity and legacy
'Generativity': [
'Anarchia', # Guido’s rebellion against oppressive reality
'Oligarchia', # The systemic constraints he navigates
'Padre Fece' # The actions and sacrifices Guido made for his son
],
# Physical realities and their interplay
'Physicality': [
'Dynamic', # Guido’s improvisational actions, like creating the “game”
'Partisan', # The direct oppression he faces
'Common Wealth', # Shared humanity and joy despite hardship
'Non-Partisan', # Universal themes transcending sides
'Static' # The immovable, tragic finality of the Holocaust
]
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Che Mio':
return 'yellow' # Perception as the interpretive bridge
if layer == 'World' and node == 'Dono Per Me':
return 'paleturquoise' # Optimism and the "gift"
if layer == 'World' and node == 'Mia Storia':
return 'lightgreen' # Harmony and legacy
if layer == 'World' and node in ['Il Sole é L’altre Stelle', 'La Terra']:
return 'lightgray' # Context of divine and tangible
elif layer == 'Agency' and node == 'Ottimismo':
return 'paleturquoise' # Guido’s defining hope
elif layer == 'Generativity':
if node == 'Padre Fece':
return 'paleturquoise' # Guido’s ultimate acts of selflessness
elif node == 'Oligarchia':
return 'lightgreen' # Navigating systemic structures
elif node == 'Anarchia':
return 'lightsalmon' # Rebellion and creativity
elif layer == 'Physicality':
if node == 'Static':
return 'paleturquoise' # The unchanging, tragic realities
elif node in ['Non-Partisan', 'Common Wealth', 'Partisan']:
return 'lightgreen' # Shared humanity and resilience
elif node == 'Dynamic':
return 'lightsalmon' # Guido’s improvisation and vitality
return 'lightsalmon' # Default color for tension or conflict
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('World', 'Perception'), # Giosuè interprets the "World" through "Che Mio"
('Perception', 'Agency'), # Guido’s cheerfulness shapes Giosuè’s perception
('Agency', 'Generativity'), # Guido’s optimism drives his generative actions
('Generativity', 'Physicality') # His legacy plays out in the physical world
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(14, 10))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Senza regole, si rischia di cadere nell'anarchia", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
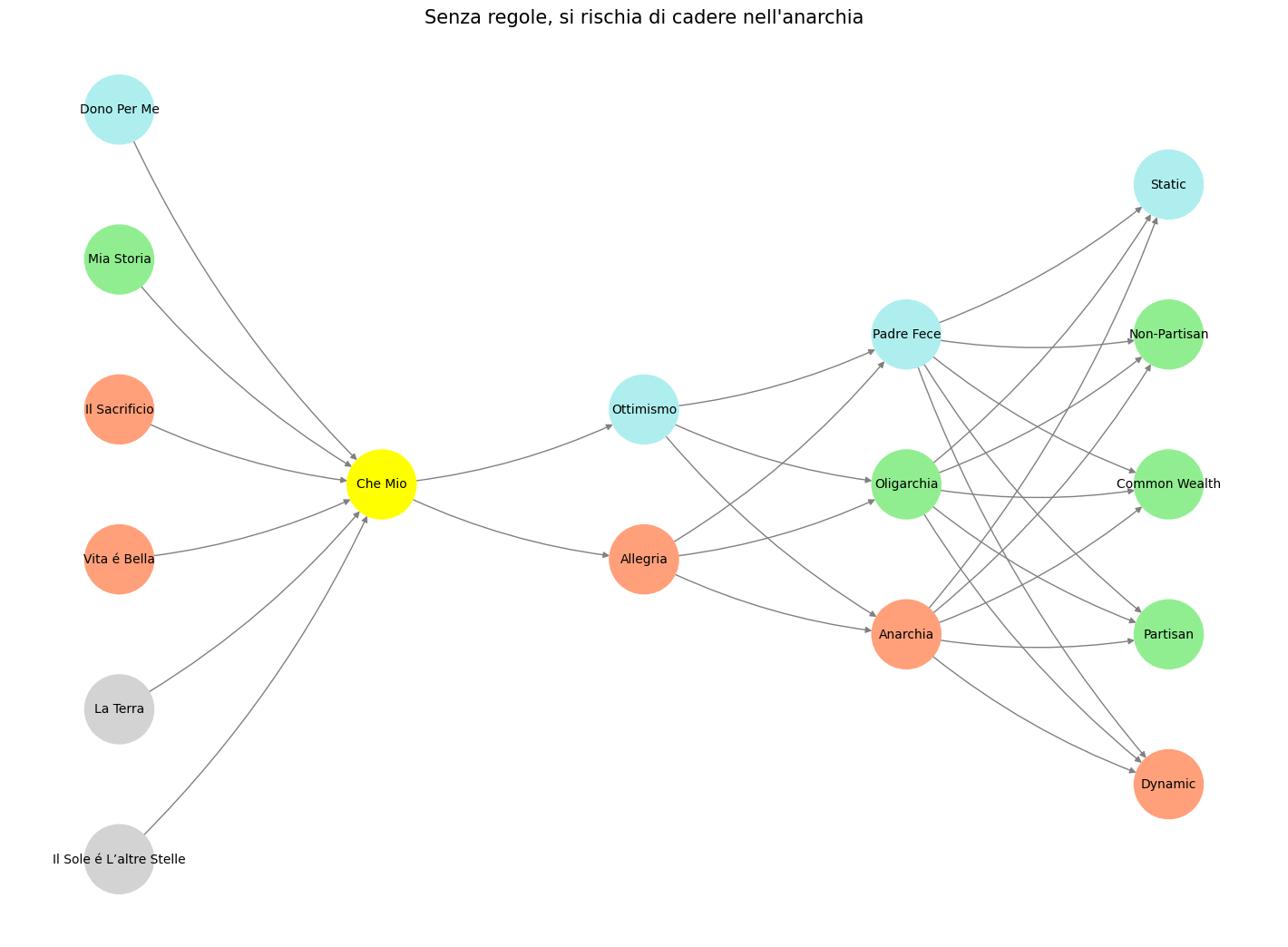
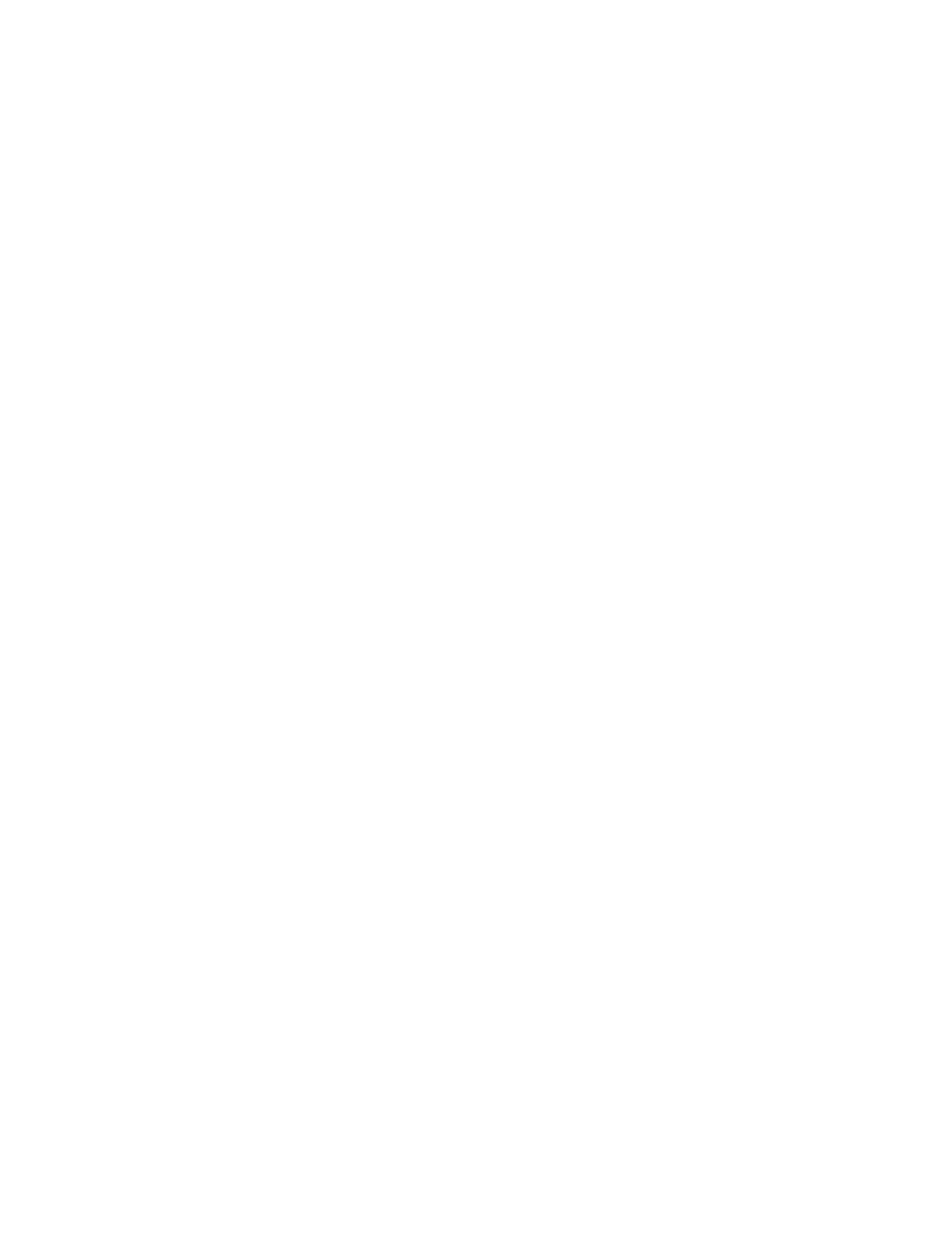
Fig. 4 In our scenario, living kidney donation is the enterprise (OPTN) and the digital twin is the control population (NHANES). When among the perioperative deaths (within 90 days of nephrectomy) we have some listed as homicide, it would be an error to enumerate those as “perioperative risk.” This is the purpose of the digital twin in our agentic (input) layer that should guide a prospective donor. There’s a delicate agency problem here that makes for a good case-study.#