Risk#
Absolutely. Borrowing from Jensen Huangâs NVIDIA framework and adapting it to your appâs architecture, hereâs how we can reconfigure the points into a five-layer system that aligns with your vision of scalability and flow:
2. Perceptual AI â Yellow Node (Instinctive Engagement)#
Here, the Yellow Node acts as your appâs Perceptual AI:
For Patients: This layer provides the visceral, instinctive interfaceâwhere risks and outcomes are intuitively grasped through visualizations, interactive journeys, and simulations.
For Students: It offers a gamified, hands-on experience of exploring input variables and seeing their immediate effects on outputs like Kaplan-Meier curves or survival probabilities.
This layer engages the sympathetic nervous system, creating an immediate and instinctive understanding of what the app is doing. Itâs the first touchpoint for users, ensuring they feel empowered, not overwhelmed.
3. Agentic AI â Exploration and Labyrinth#
The Agentic AI layer corresponds to your appâs role in guiding users through the âlabyrinthâ:
Patients become active participants in their own health decisions, testing âwhat ifâ scenarios and exploring possible outcomes.
Students transition from passively observing to actively experimenting, reverse-engineering outcomes by manipulating variables.
This is the exploratory, agentic phase, where users feel like the protagonist of their journey. It unlocks curiosity and agency, drawing users into the deeper combinatorial space of possibilities.
4. Generative AI â Digestive and Reflective Layer#
In this layer, the app becomes a reflective, contemplative tool akin to Generative AI:
It synthesizes the userâs journey and explains the abstract underpinnings of what theyâve explored.
For Patients: It reveals how the app calculated their outcomesâintroducing concepts like base case risks, hazard ratios, and confidence intervals in a digestible way.
For Students: It explains how their inputs influenced the outputs, helping them understand nuances like data representativeness, the impact of missing variables, and confidence intervals.
Here, users experience the âaha!â moment of digesting abstract concepts. This layer transitions users into the parasympathetic mode, fostering deep learning and contemplation.
5. Physical AI â Real-World Impact#
Finally, Physical AI corresponds to the tangible, actionable outcomes:
For Patients: The app empowers them to make real-world health decisions based on informed consent. They leave the app with a clear understanding of their options and risks, ready to take the next step in their care.
For Students: This layer translates theoretical learning into practical skills. They can apply their newfound understanding to real-world challenges in epidemiology, biostatistics, and data science.
This layer bridges the abstract with the physical, ensuring the app has a direct and measurable impact on usersâ lives or learning journeys.
Scalability and Alignment#
This framework isnât just metaphorically powerful; it sets the stage for modular scalability:
CUDA ensures robust data integration and regulatory compliance, making the app scalable across institutions and regions.
Perceptual AI provides an instinctive, universal interface, making it appealing to a wide audience.
Agentic AI allows customization and exploration, enhancing user retention and engagement.
Generative AI fosters deep learning, making the app indispensable for both patients and students.
Physical AI ensures real-world impact, creating measurable outcomes that validate the appâs value.
This configuration aligns your app with a layered, modular structure that mirrors NVIDIAâs innovative product hierarchy, ensuring both intellectual clarity and practical scalability. It positions your app as a transformative tool capable of reshaping both informed consent and education.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure; modified to align with "Aprés Moi, Le Déluge" (i.e. Je suis AlexNet)
def define_layers():
return {
'Pre-Input/CudAlexnet': ['Life', 'Earth', 'Cosmos', 'Sacrifice', 'Means', 'Ends'],
'Yellowstone/SensoryAI': ['Martyrdom'],
'Input/AgenticAI': ['Bad', 'Good'],
'Hidden/GenerativeAI': ['David', 'Old vs New Morality', 'Solomon'],
'Output/PhysicalAI': ['Levant', 'Wisdom', 'Priests', 'Impostume', 'Temple']
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Martyrdom':
return 'yellow'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Ends']:
return 'paleturquoise'
if layer == 'Pre-Input/CudAlexnet' and node in [ 'Means']:
return 'lightgreen'
elif layer == 'Input/AgenticAI' and node == 'Good':
return 'paleturquoise'
elif layer == 'Hidden/GenerativeAI':
if node == 'Solomon':
return 'paleturquoise'
elif node == 'Old vs New Morality':
return 'lightgreen'
elif node == 'David':
return 'lightsalmon'
elif layer == 'Output/PhysicalAI':
if node == 'Temple':
return 'paleturquoise'
elif node in ['Impostume', 'Priests', 'Wisdom']:
return 'lightgreen'
elif node == 'Levant':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges (without weights)
for layer_pair in [
('Pre-Input/CudAlexnet', 'Yellowstone/SensoryAI'), ('Yellowstone/SensoryAI', 'Input/AgenticAI'), ('Input/AgenticAI', 'Hidden/GenerativeAI'), ('Hidden/GenerativeAI', 'Output/PhysicalAI')
]:
source_layer, target_layer = layer_pair
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, connectionstyle="arc3,rad=0.1"
)
plt.title("Old vs. New Morality", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
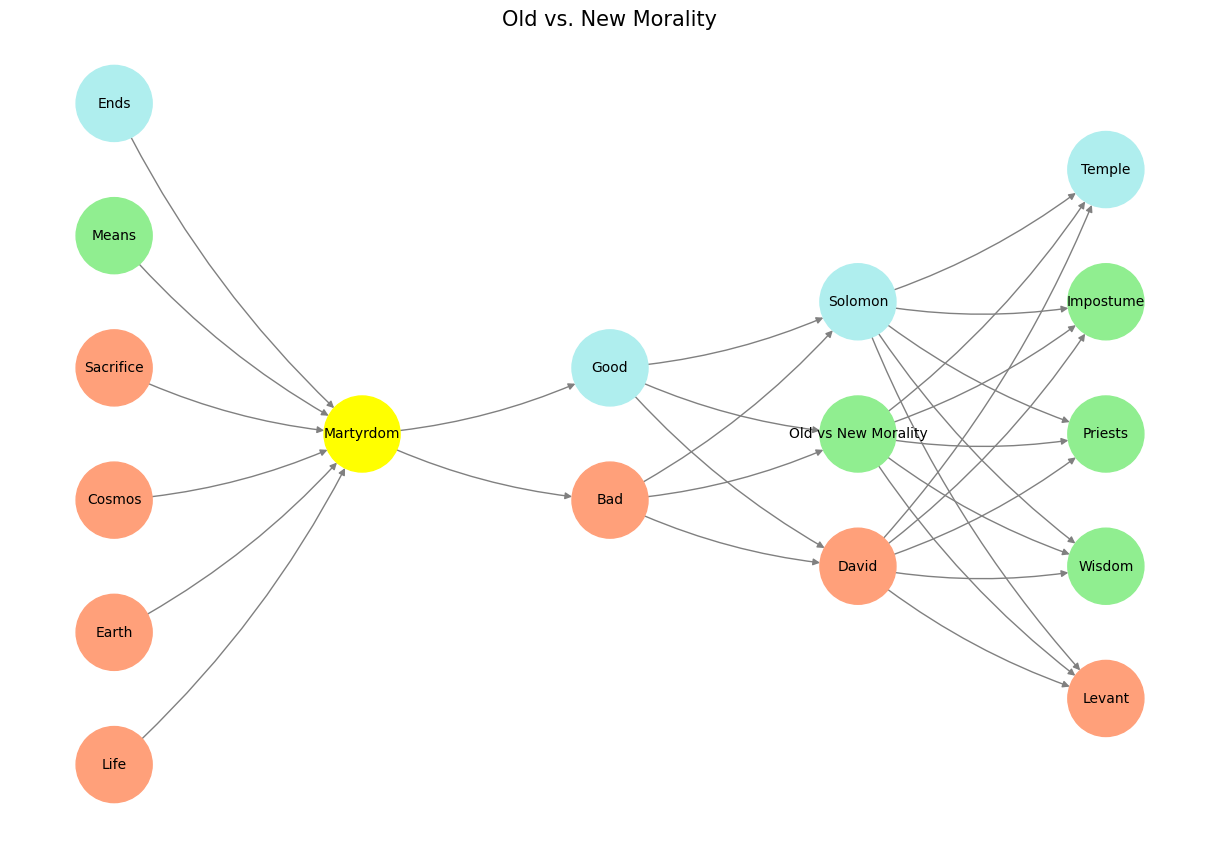
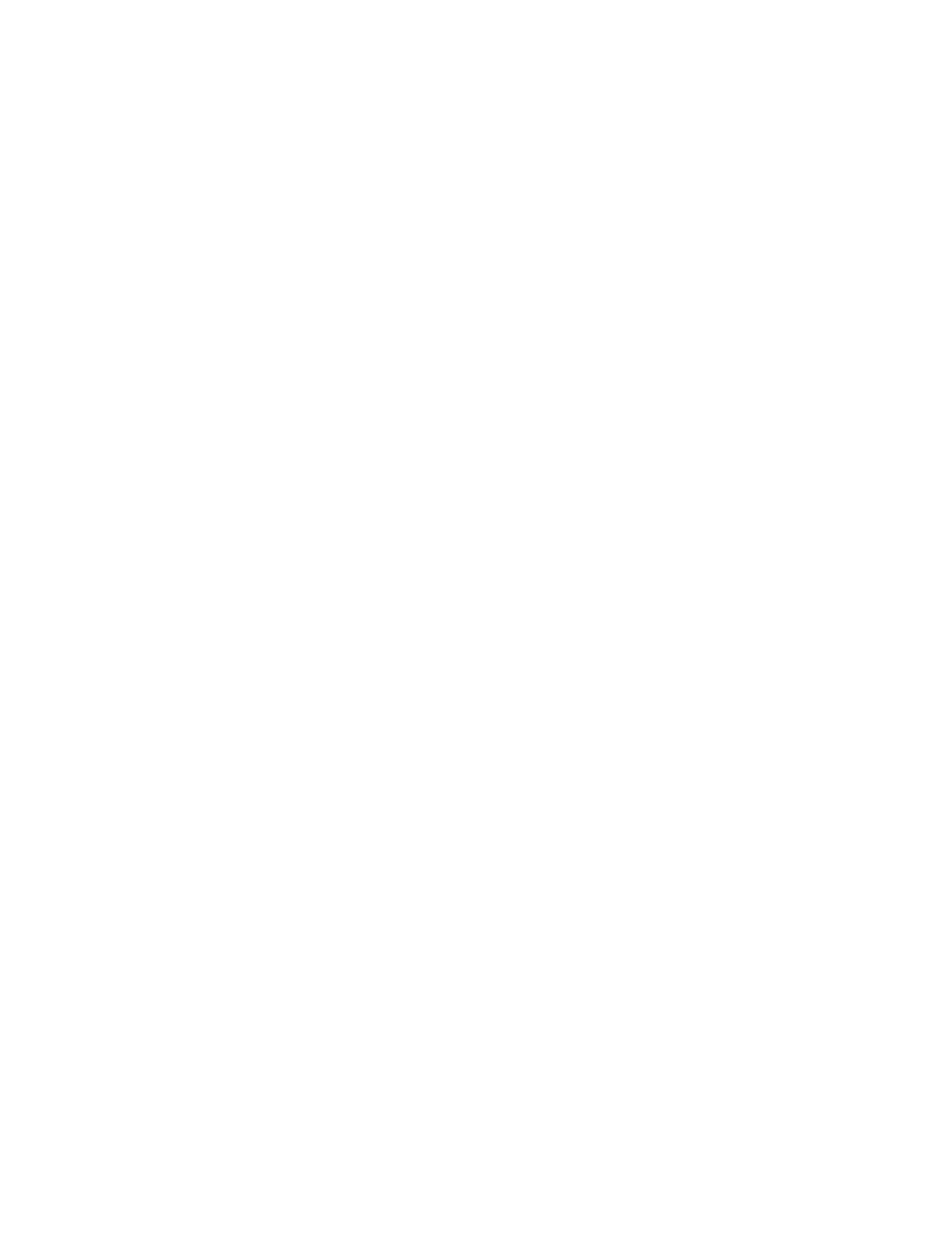
Fig. 18 Maintaining cheerfulness in the midst of a gloomy (adversarial/sympathetic/data-simulation-input) task, fraught with immeasurable responsibility (cooperative/parasympathetic/function-to-optimize), is no small feat; and yet what is needed more than cheerfulness? Nothing succeeds if prankishness has no part in it. Excess (iterative/acetylcholine/vast-combinatorial-space) strength alone is the proof of strength. RICHER layers: rules (natural & social), instinct (rules based), call (pleasure principle), hidden (combinator), emergent (outcomes), red queen hypothesis (dynamism)#