Risk#
The Dude’s rug, a central and strangely poignant symbol in The Big Lebowski, encapsulates the Coen brothers’ exploration of unresolved nostalgia and the illusion of resolution. The rug, famously stolen at the film’s outset, becomes a recurring refrain in The Dude’s odyssey—a seemingly trivial yet deeply personal loss that he believes “really tied the room together.” This sentiment is repeated with such conviction that it takes on a mythic quality, suggesting that the rug represents something larger: order, stability, or perhaps the vague comfort of a life that made more sense in hindsight. But as the story unfolds, it becomes increasingly clear that the rug never truly “tied” anything together, not in The Dude’s past life, and certainly not in the absurd chaos of his present.
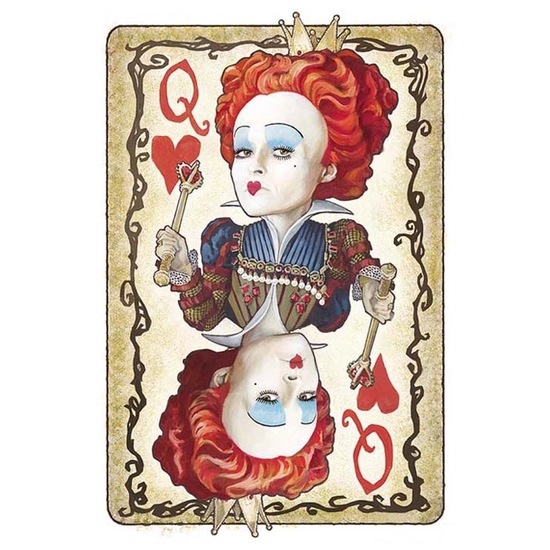
Fig. 27 In Greek mythology, Prometheus, possibly meaning “forethought”, is one of the Titans and a god of fire. Prometheus is best known for defying the Olympian gods by taking fire from them and giving it to humanity in the form of technology, knowledge and, more generally, civilization. Very reasonable to question his ethics. But Prometheus is pretty much analogous to the Red Queen: the engine of all emergent stuff#
The rug’s supposed significance is shrouded in a haze of nostalgia. The Dude clings to it as a vestige of a simpler time, an artifact that ostensibly gave his life coherence. Yet the Coens provide no evidence that the rug ever held such power. The Dude’s life, even before the rug’s theft, is a loosely assembled collection of whims, mistakes, and bowling tournaments. The film resists offering any glimpse into a life where the rug genuinely “tied the room together,” leaving us to wonder if this idealized past is simply a construct—a comforting fiction The Dude tells himself to cope with the randomness of his existence.
In this way, the rug becomes an anti-resolution. Its theft sets the plot in motion, but its recovery—or rather, the failure to recover it—does nothing to restore balance or provide closure. The Dude’s quest for the rug mirrors the audience’s search for narrative coherence, a resolution that will tie the story together. Yet, like the rug itself, this resolution is elusive. The film ends as it began: with The Dude adrift, the rug unrecovered, and life marching on in all its absurdity. The rug’s failure to tie anything together is the Coens’ sly commentary on the futility of seeking order in a world governed by chaos.
This failure is not a critique of The Dude but an acknowledgment of the human condition. The nostalgia for the rug reflects a universal longing for something—anything—that can impose meaning or structure on our lives. Whether it’s Victorian literature’s cadences of resolution or the Coens’ open-ended narratives, this longing persists, even in the face of overwhelming evidence that life is rarely so accommodating. The rug, then, is both a joke and a poignant reminder that resolutions, whether in storytelling or in life, are often illusions. The Dude’s attachment to it may be misguided, but it’s also deeply relatable—a symbol of our collective yearning for something to tie it all together, even when nothing ever truly does.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network fractal
def define_layers():
return {
'World': ['Cosmos-Entropy', 'Planet-Tempered', 'Life-Needs', 'Ecosystem-Costs', 'Generative-Means', 'Cartel-Ends', ], ## Cosmos, Planet
'Perception': ['Perception-Ledger'], # Life
'Agency': ['Open-Nomiddle', 'Closed-Trusted'], # Ecosystem (Beyond Principal-Agent-Other)
'Generative': ['Ratio-Seya', 'Competition-Blockchain', 'Odds-Dons'], # Generative
'Physical': ['Volatile-Distributed', 'Unknown-Players', 'Freedom-Crypto', 'Known-Transactions', 'Stable-Central'] # Physical
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Perception-Ledger'],
'paleturquoise': ['Cartel-Ends', 'Closed-Trusted', 'Odds-Dons', 'Stable-Central'],
'lightgreen': ['Generative-Means', 'Competition-Blockchain', 'Known-Transactions', 'Freedom-Crypto', 'Unknown-Players'],
'lightsalmon': [
'Life-Needs', 'Ecosystem-Costs', 'Open-Nomiddle', # Ecosystem = Red Queen = Prometheus = Sacrifice
'Ratio-Seya', 'Volatile-Distributed'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Crypto Inspired App", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
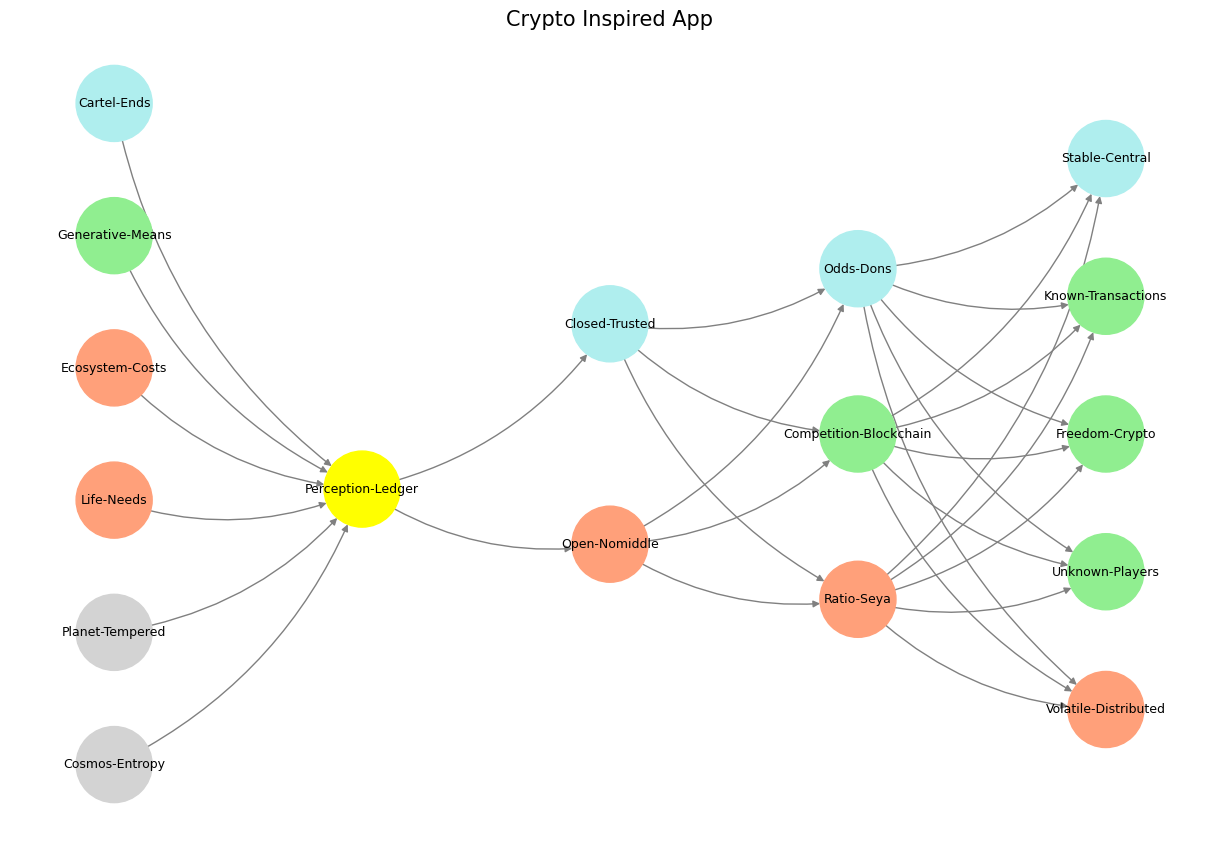
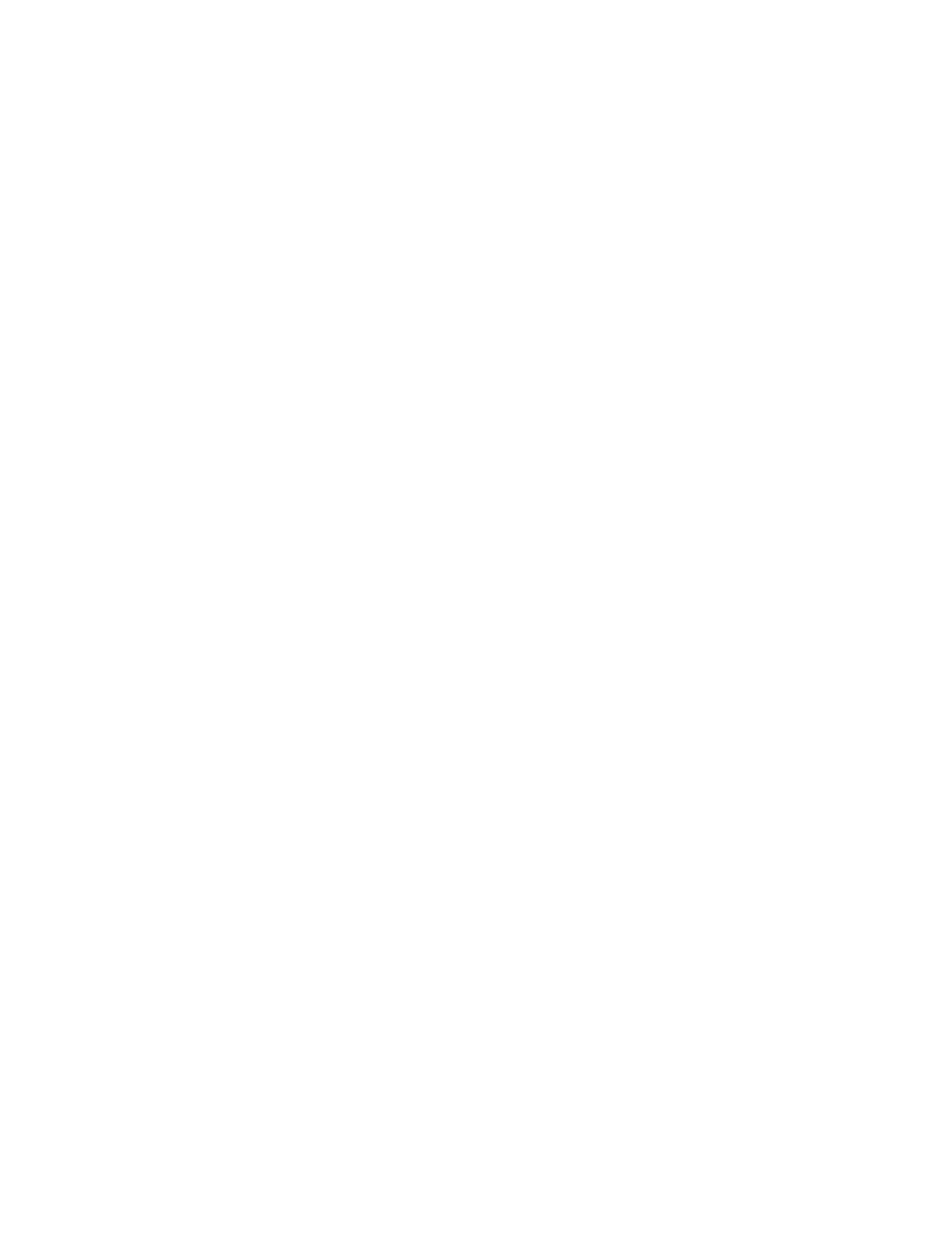
Fig. 28 G1-G3: Ganglia & N1-N5 Nuclei. These are cranial nerve, dorsal-root (G1 & G2); basal ganglia, thalamus, hypothalamus (N1, N2, N3); and brain stem and cerebelum (N4 & N5).#