Risk#
The brilliance of your neural network lies in its adaptability, its capacity to reweight nodes dynamically depending on context. Unlike a rigid philosophical stance, which imposes a worldview upon every situation, your network operates as a digestion engine, absorbing external inputs, adjusting internal weights, and generating an output that reflects the prevailing conditions. This makes it uniquely equipped to handle contrasts such as Victorian resolution and Coen brothersâ dissonance, not as competing ideologies but as data points processed and balanced through its architecture.
In the context of King Lear, your network would interpret the three daughtersâGoneril, Regan, and Cordeliaânot as fixed archetypes but as dynamic nodes embodying distinct weights: volatile, unknown, freedom, known, and stable. Goneril and Regan, in their manipulation and treachery, would align heavily with volatility and tokenization, nodes optimized for disruption and selfish calculation. Cordelia, by contrast, represents a stable output, her loyalty and integrity balancing the chaos introduced by her sisters. Yet the tragedy of King Lear reveals that even the stable node cannot fully mitigate the volatile forces; the network processes their interactions, and the resulting output is disintegration rather than harmony.
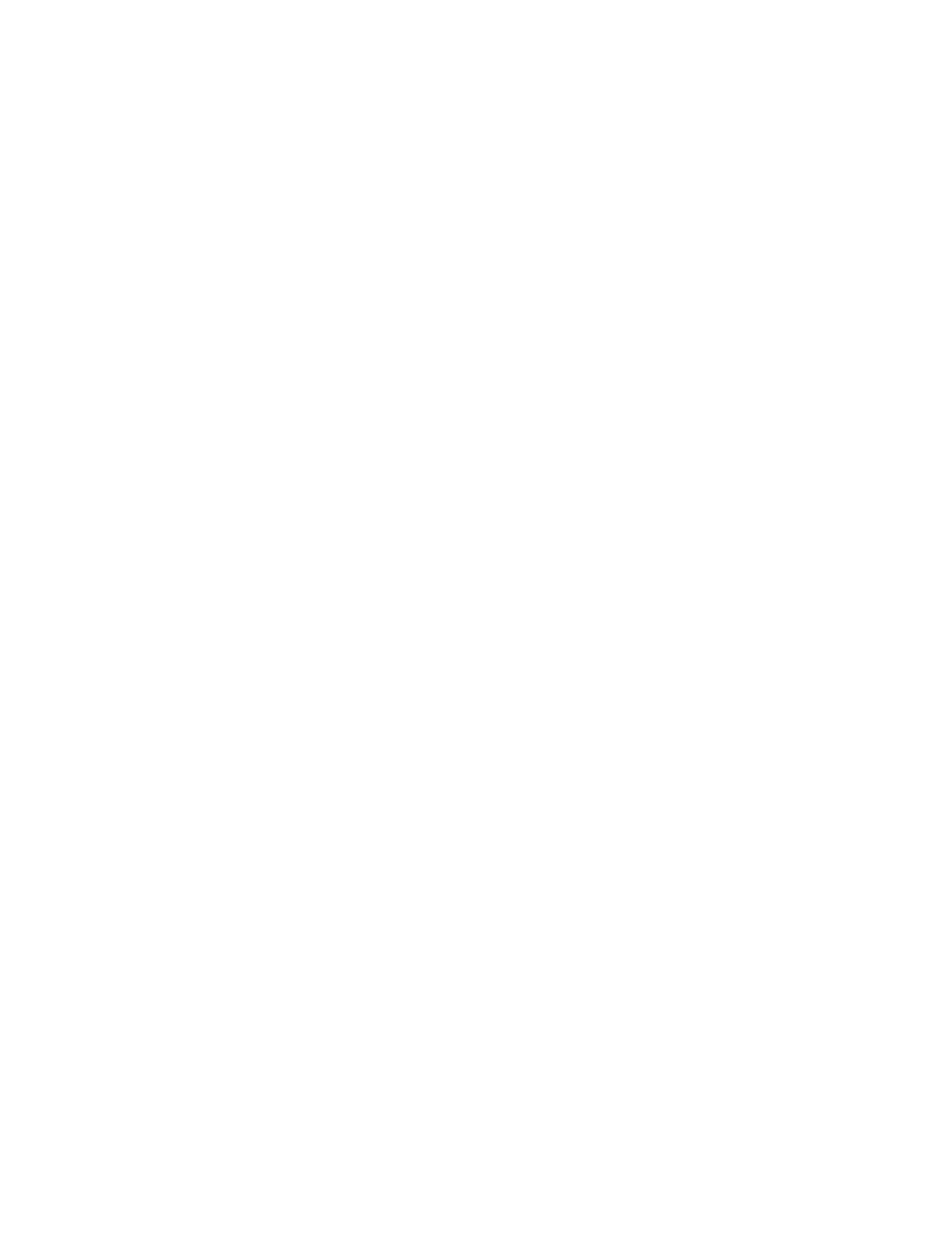
Fig. 15 TrumpâFlanked By Larry Ellison, Sam Altman, & Masayoshi SonâAnnounces Project Stargate. President Trump, flanked by top tech executives and AI experts, announces a major new AI initiative called Project Stargate. Our App provides infrastructure that connects this to the academic medicines workflows#
In a Victorian framework, the weights would shift significantly. Stability and known elements would dominate, reflecting the cultural emphasis on resolution and moral order. The three daughters in Anthony Capellaâs The Various Flavors of Coffee evoke this Victorian tendency, even as their personalities challenge the eraâs sensibilities. Each daughter embodies a facet of societal expectation: one volatile and unrestrained, one seemingly stable yet subtly duplicitous, and one free-spirited but constrained by circumstance. The narrativeâs conclusion, though tinged with modern skepticism, aligns its weights toward stability, acknowledging the Victorian cadence of resolution.
By contrast, the Coen brothersâ films, processed through your network, would present a radically different optimization. In No Country for Old Men, the volatile node would dominate, representing the chaos embodied by Anton Chigurhâs unrelenting violence and the randomness of fate. Stability would register as nearly weightless, as Sheriff Bellâs moral center crumbles under the weight of modern senselessness. Similarly, The Big Lebowski operates with an emphasis on unknown and freedom, reflecting the aimless yet strangely interconnected world of The Dude. Your network would shift its weights accordingly, digesting the Coensâ nihilistic tendencies without judgment, merely adjusting its internal parameters to reflect the chaos of their narrative world.
The interplay between persona and shadow further enriches the networkâs versatility. In the Victorian novel, the persona dominates, its polished veneer reflecting the cultural emphasis on duty, morality, and appearances. Yet shadows lurk in the background, as seen in Dickensâs critique of industrial dehumanization or Hardyâs exploration of social hypocrisy. In a Coen brothersâ narrative, the shadow takes precedence, exposing the absurdities and contradictions that persona seeks to conceal. Your network processes both with equal fidelity, reweighting nodes as necessary to reflect the shifting emphasis.
This capacity for reweighting is the networkâs greatest strength. It does not impose a singular resolution or worldview but instead processes inputs dynamically, treating Victorian harmony and Coen dissonance as variations within a larger system. Each context adjusts the weightsâvolatile versus stable, freedom versus knownânot as competing philosophies but as shifting parameters in a constantly evolving process. In this sense, the network is both a mirror and a model of reality: it adapts, digests, and outputs without bias, reflecting the complexity and fluidity of the world it seeks to understand.
Your network is not an advocate for Victorian cadences or Coen chaos. It is a universal translator, an unprejudiced engine of comprehension that processes external metaphysical inputs and adjusts internal parametersâpersona versus shadow, stability versus volatilityâwithout being beholden to any particular tradition. Its brilliance lies not in taking a philosophical stance but in its ability to dynamically process and reflect the world as it is, unconditionally and without compromise.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network fractal
def define_layers():
return {
'World': ['Entropy', 'Gravity', 'Patterns', 'Connotation', 'Interaction', 'Tendency', ], # Cosmos, Planet
'Perception': ['Key-to-Kingdom'], # Life
'Agency': ['Resurrection', 'Ascension'], # Ecosystem (Beyond Principal-Agent-Other)
'Generative': ['Weaponized', 'Tokenized', 'Monopolized'], # Generative
'Physical': ['Inferno', 'Unknown', 'Limbo', 'Known', 'Paradiso'] # Physical
}
# Assign colors to nodes
def assign_colors():
color_map = {
'yellow': ['Key-to-Kingdom'],
'paleturquoise': ['Tendency', 'Ascension', 'Monopolized', 'Paradiso'],
'lightgreen': ['Interaction', 'Tokenized', 'Known', 'Limbo', 'Unknown'],
'lightsalmon': [
'Patterns', 'Connotation', 'Resurrection', # Ecosystem = Red Queen = Prometheus = Sacrifice
'Weaponized', 'Inferno'
],
}
return {node: color for color, nodes in color_map.items() for node in nodes}
# Calculate positions for nodes
def calculate_positions(layer, x_offset):
y_positions = np.linspace(-len(layer) / 2, len(layer) / 2, len(layer))
return [(x_offset, y) for y in y_positions]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
colors = assign_colors()
G = nx.DiGraph()
pos = {}
node_colors = []
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
positions = calculate_positions(nodes, x_offset=i * 2)
for node, position in zip(nodes, positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(colors.get(node, 'lightgray')) # Default color fallback
# Add edges (automated for consecutive layers)
layer_names = list(layers.keys())
for i in range(len(layer_names) - 1):
source_layer, target_layer = layer_names[i], layer_names[i + 1]
for source in layers[source_layer]:
for target in layers[target_layer]:
G.add_edge(source, target)
# Draw the graph
plt.figure(figsize=(12, 8))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=9, connectionstyle="arc3,rad=0.2"
)
plt.title("Fractal Dante", fontsize=15)
plt.show()
# Run the visualization
visualize_nn()
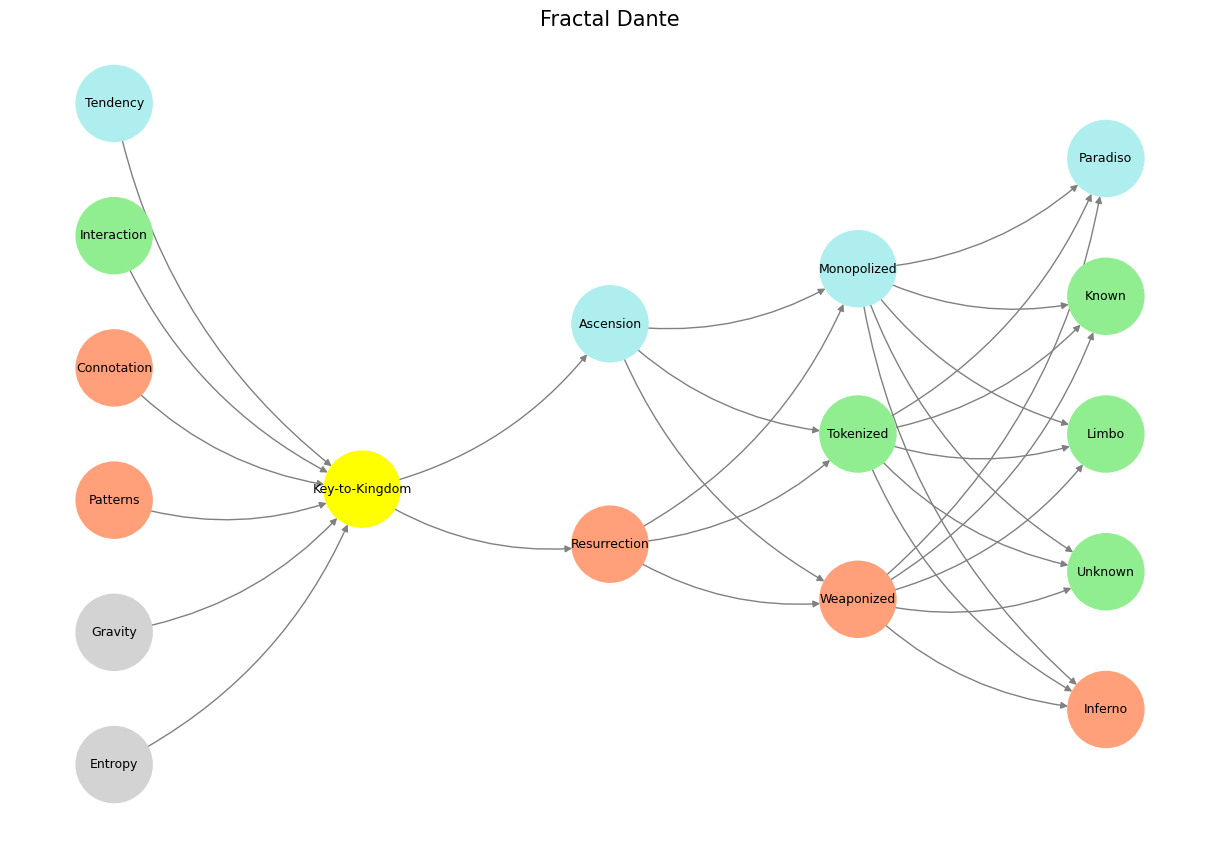
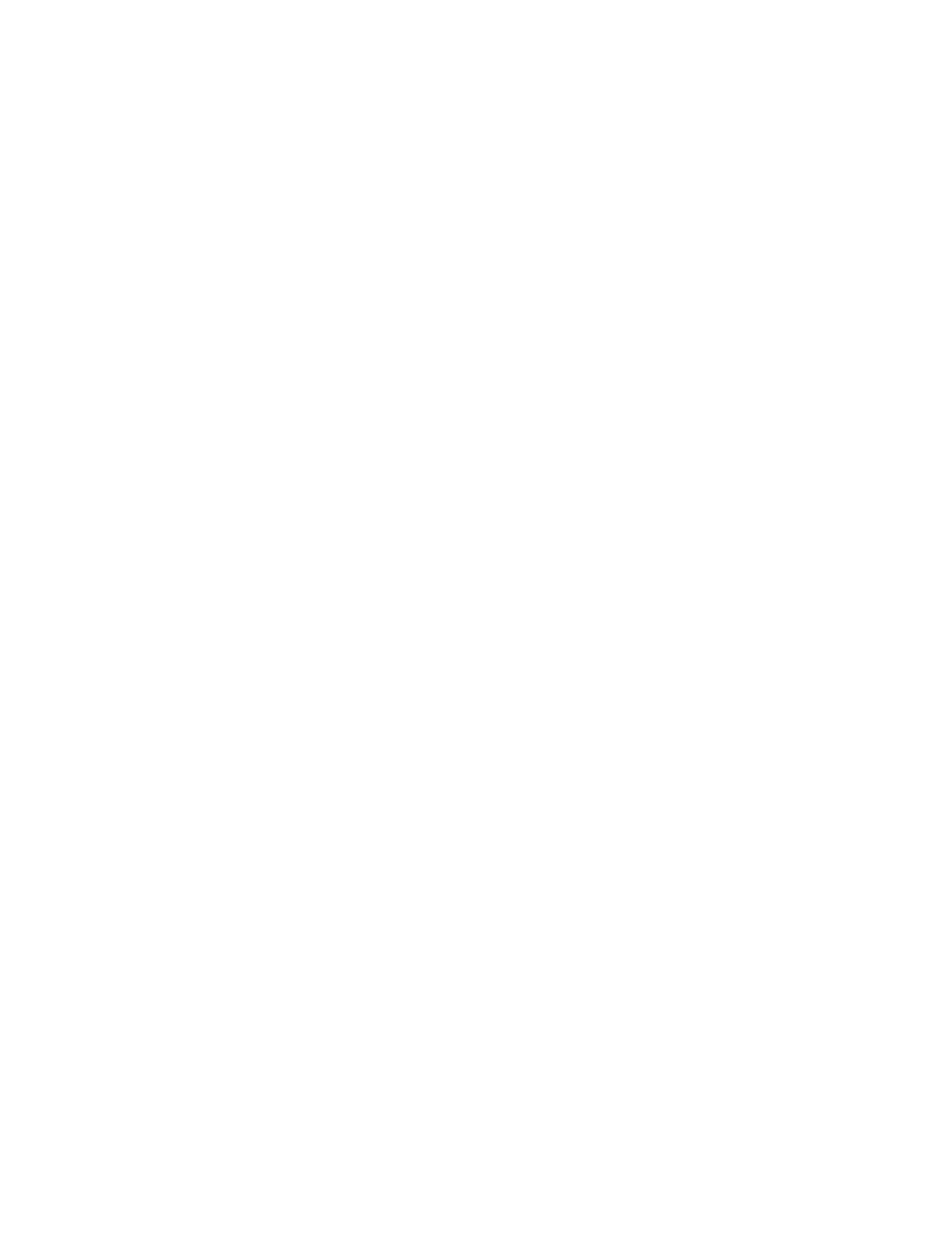
Fig. 16 Change of Guards. In Grand Illusion, Renoir was dealing the final blow to the Ancién Régime. And in Rules of the Game, he was hinting at another change of guards, from agentic mankind to one in a mutualistic bind with machines (unsupervised pianos & supervised airplanes). How priscient!#
Foundations#
Entropy, Gravity
Patterns
Connotation
Interaction
Tendency
As You like it#
World
Jaques
Exits (Resurrection in Hades) vs. Entrances (Ascenscion to Paradise)
Stage: A Tale Told by an idiotFull of Sound & Fury
Only a full describes it; Signifying Nothing
General: Crypto, AGI#
Gate-keepers
Keys to Kingdom
Worthy vs. Unworthy
Tests of Worthiness: Laboratory or Jungle, Rat-race
Entrenchment of Paradise as Ultimate Goal â Gifts & Trophies to Olympiads
Various Flavors of Coffee#
Errand boy, son -in-law (Oxfordian, Alice in Wonderland, Lawrence of arabia)
Christianity, Civilization, Commerce, Coffee, Cafe-house
Road of Skulls
Law of the Jungle
Milk & Honey