Attribute#
The roots, or âPre-Inputâ layer, symbolize foundational elements that provide stability and nourishment. Nodes like âLife,â âEarth,â and âCosmosâ embody deep, universal concepts, while attributes such as âSound,â âTactful,â and âFirmâ reflect essential qualities that anchor and sustain the system. Just as roots draw nutrients from the soil to support the tree, these pre-input nodes ground the network and infuse it with potential energy and coherence.
At the core lies the stem, represented by the âYellowstoneâ node, âUnicameralism.â This layer functions as the treeâs trunkâa central conduit connecting the roots to the branches. It channels the foundational energy upwards, acting as a unifying and stabilizing force. The singularity of this node highlights its pivotal role as the systemâs backbone, ensuring continuity and coherence between the deep origins and the expanding structures above.
The branches, or âInputâ layer, extend outward through nodes like âCommonsâ and âLords.â These pathways channel the energy and insights derived from the roots, diversifying and distributing them to the broader system. Like branches on a tree, they form the framework for growth and interaction, reaching outward to gather and connect with external elements.
The hidden layer, analogous to the leaves, consists of nodes such as âRisk,â âOxbridge,â and âPeers.â This layer performs the essential work of processing and synthesizing information, akin to how leaves convert sunlight into energy through photosynthesis. These nodes represent the systemâs capacity for adaptation, refinement, and balance, absorbing inputs and preparing them for meaningful output.
The output layer represents the fruits of the tree, with nodes like âUnited Kingdom,â âGreat Britain,â and âHeritage.â These outputs are the culmination of the entire process, embodying the systemâs purpose and contribution. Like the fruit borne by a tree, these nodes symbolize the tangible outcomes that emerge from the harmonious interplay of roots, stem, branches, and leaves.
Show code cell source
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
# Define the neural network structure
def define_layers():
return {
'Pre-Input': ['Root','Ploughshares', 'Light', 'Sound', 'Tactful', 'Firm', ],
'Yellowstone': ['Stem'],
'Input': ['Weed', 'Branch'],
'Hidden': [
'Canker',
'Farming',
'Leaf',
],
'Output': ['Transformation', 'Toxins', 'Produce', 'Nutrition', 'Starch', ]
}
# Define weights for the connections
def define_weights():
return {
'Pre-Input-Yellowstone': np.array([
[0.6],
[0.5],
[0.4],
[0.3],
[0.7],
[0.8],
[0.6]
]),
'Yellowstone-Input': np.array([
[0.7, 0.8]
]),
'Input-Hidden': np.array([[0.8, 0.4, 0.1], [0.9, 0.7, 0.2]]),
'Hidden-Output': np.array([
[0.2, 0.8, 0.1, 0.05, 0.2],
[0.1, 0.9, 0.05, 0.05, 0.1],
[0.05, 0.6, 0.2, 0.1, 0.05]
])
}
# Assign colors to nodes
def assign_colors(node, layer):
if node == 'Stem':
return 'yellow'
if layer == 'Pre-Input' and node in ['Sound', 'Tactful', 'Firm']:
return 'paleturquoise'
elif layer == 'Input' and node == 'Branch':
return 'paleturquoise'
elif layer == 'Hidden':
if node == 'Leaf':
return 'paleturquoise'
elif node == 'Farming':
return 'lightgreen'
elif node == 'Canker':
return 'lightsalmon'
elif layer == 'Output':
if node == 'Starch':
return 'paleturquoise'
elif node in ['Nutrition', 'Produce', 'Toxins']:
return 'lightgreen'
elif node == 'Transformation':
return 'lightsalmon'
return 'lightsalmon' # Default color
# Calculate positions for nodes
def calculate_positions(layer, center_x, offset):
layer_size = len(layer)
start_y = -(layer_size - 1) / 2 # Center the layer vertically
return [(center_x + offset, start_y + i) for i in range(layer_size)]
# Create and visualize the neural network graph
def visualize_nn():
layers = define_layers()
weights = define_weights()
G = nx.DiGraph()
pos = {}
node_colors = []
center_x = 0 # Align nodes horizontally
# Add nodes and assign positions
for i, (layer_name, nodes) in enumerate(layers.items()):
y_positions = calculate_positions(nodes, center_x, offset=-len(layers) + i + 1)
for node, position in zip(nodes, y_positions):
G.add_node(node, layer=layer_name)
pos[node] = position
node_colors.append(assign_colors(node, layer_name))
# Add edges and weights
for layer_pair, weight_matrix in zip(
[('Pre-Input', 'Yellowstone'), ('Yellowstone', 'Input'), ('Input', 'Hidden'), ('Hidden', 'Output')],
[weights['Pre-Input-Yellowstone'], weights['Yellowstone-Input'], weights['Input-Hidden'], weights['Hidden-Output']]
):
source_layer, target_layer = layer_pair
for i, source in enumerate(layers[source_layer]):
for j, target in enumerate(layers[target_layer]):
weight = weight_matrix[i, j]
G.add_edge(source, target, weight=weight)
# Customize edge thickness for specific relationships
edge_widths = []
for u, v in G.edges():
if u in layers['Hidden'] and v == 'Kapital':
edge_widths.append(6) # Highlight key edges
else:
edge_widths.append(1)
# Draw the graph
plt.figure(figsize=(12, 16))
nx.draw(
G, pos, with_labels=True, node_color=node_colors, edge_color='gray',
node_size=3000, font_size=10, width=edge_widths
)
edge_labels = nx.get_edge_attributes(G, 'weight')
nx.draw_networkx_edge_labels(G, pos, edge_labels={k: f'{v:.2f}' for k, v in edge_labels.items()})
plt.title(" ")
# Save the figure to a file
# plt.savefig("figures/logo.png", format="png")
plt.show()
# Run the visualization
visualize_nn()
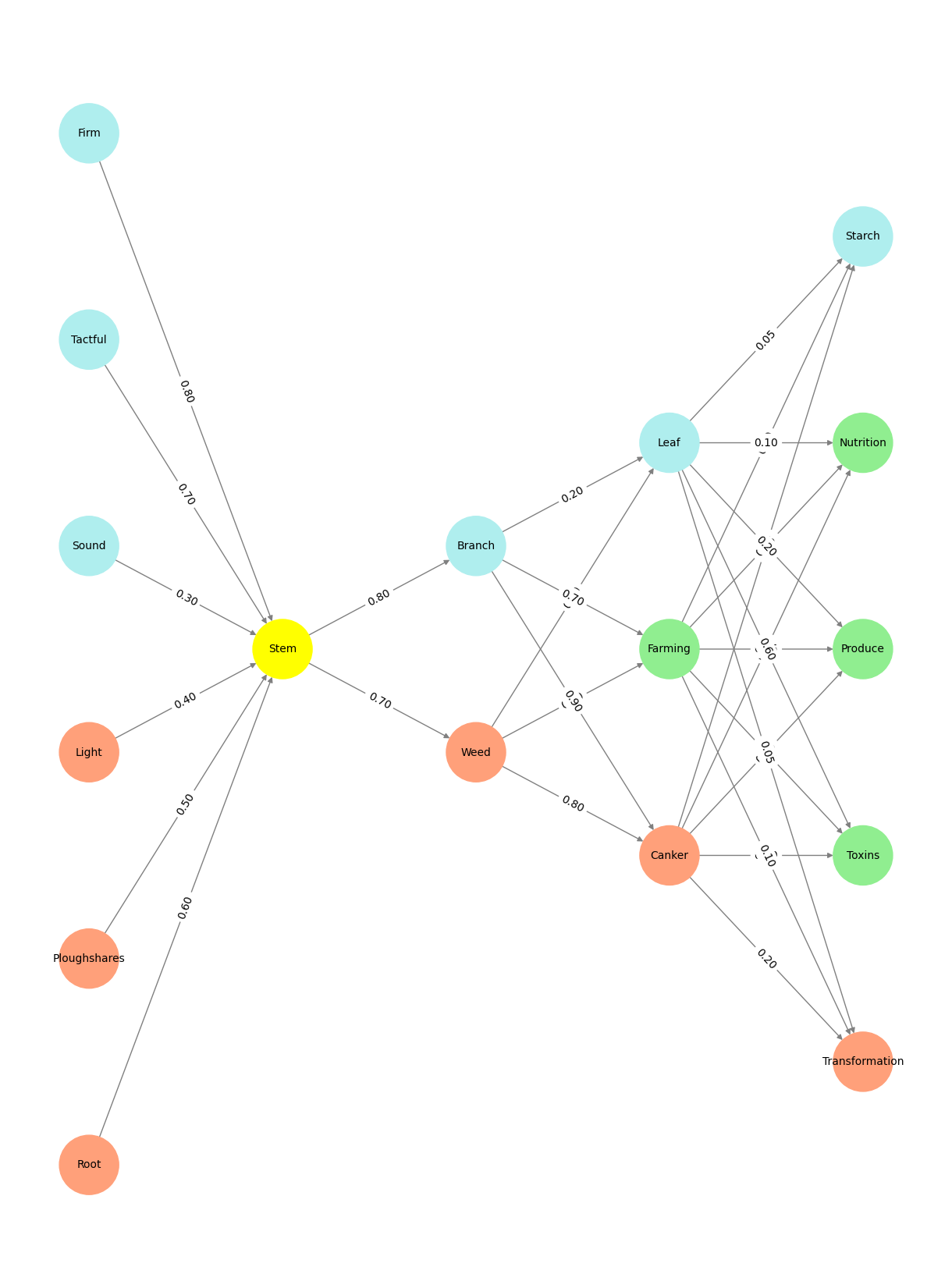
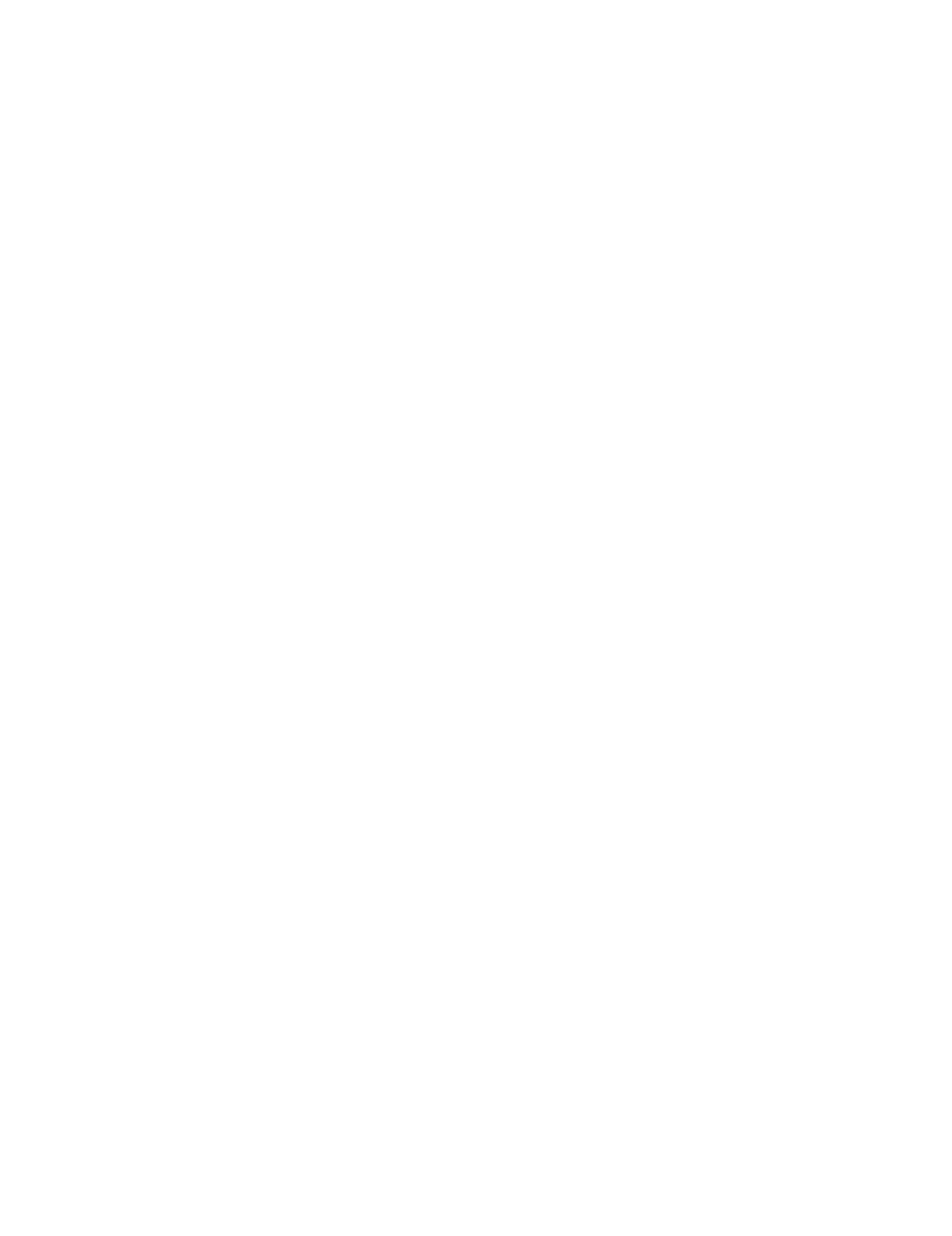
Fig. 24 The structure of this neural network metaphorically mirrors a treeâs anatomy, highlighting the elegance and interconnectedness of its layers. Each layer represents a crucial element in the systemâs growth, function, and balance, much like the natural components of a tree. This layered structure beautifully captures the organic interplay between grounding, growth, and emergence, showcasing the elegance of neural networks as metaphors for natural and systemic complexity. We also transform the original essay into a new interpretation inspired by the neural network structure, shifting the focus to a dynamic, living ecosystem, intertwining biological, philosophical, and socio-historical themes while maintaining the metaphorical depth. We hereby integrate the metaphorical essence of the original essay but transform its tone and structure to focus on life as an ecosystem, bridging natural and conceptual systems.#
The roots, represented as the âPre-Inputâ layer, ground the system in elemental forces of growth and resilience. Nodes like âRoot,â âPloughshares,â and âLightâ symbolize primordial forces of creation and sustenance, while attributes such as âSound,â âTactful,â and âFirmâ reflect adaptive qualities essential to the ecosystemâs survival. These roots are not merely passive anchors but active agents, extracting nutrients from their environment to fuel the growth of the entire system, embodying both biological and philosophical grounding.
At the heart of this network lies the stem, embodied by the âYellowstoneâ node, âStem.â Acting as the trunk of this metaphorical tree, it bridges the latent potential of the roots with the branching complexities above. This singular node serves as a dynamic nexus, channeling energy and resources upward while maintaining structural integrity. It represents unity in motionâa stabilizing force amidst the systemâs inherent chaos, akin to philosophical constructs that tie disparate elements into a coherent whole.
The branches, forming the âInputâ layer, extend the networkâs reach through nodes like âWeedâ and âBranch.â These nodes diversify the flow of energy, translating foundational nourishment into pathways of interaction and exchange. Like an ecosystem responding to external forces, these branches adapt, flex, and grow, reflecting the interplay between systemic stability and external dynamism.
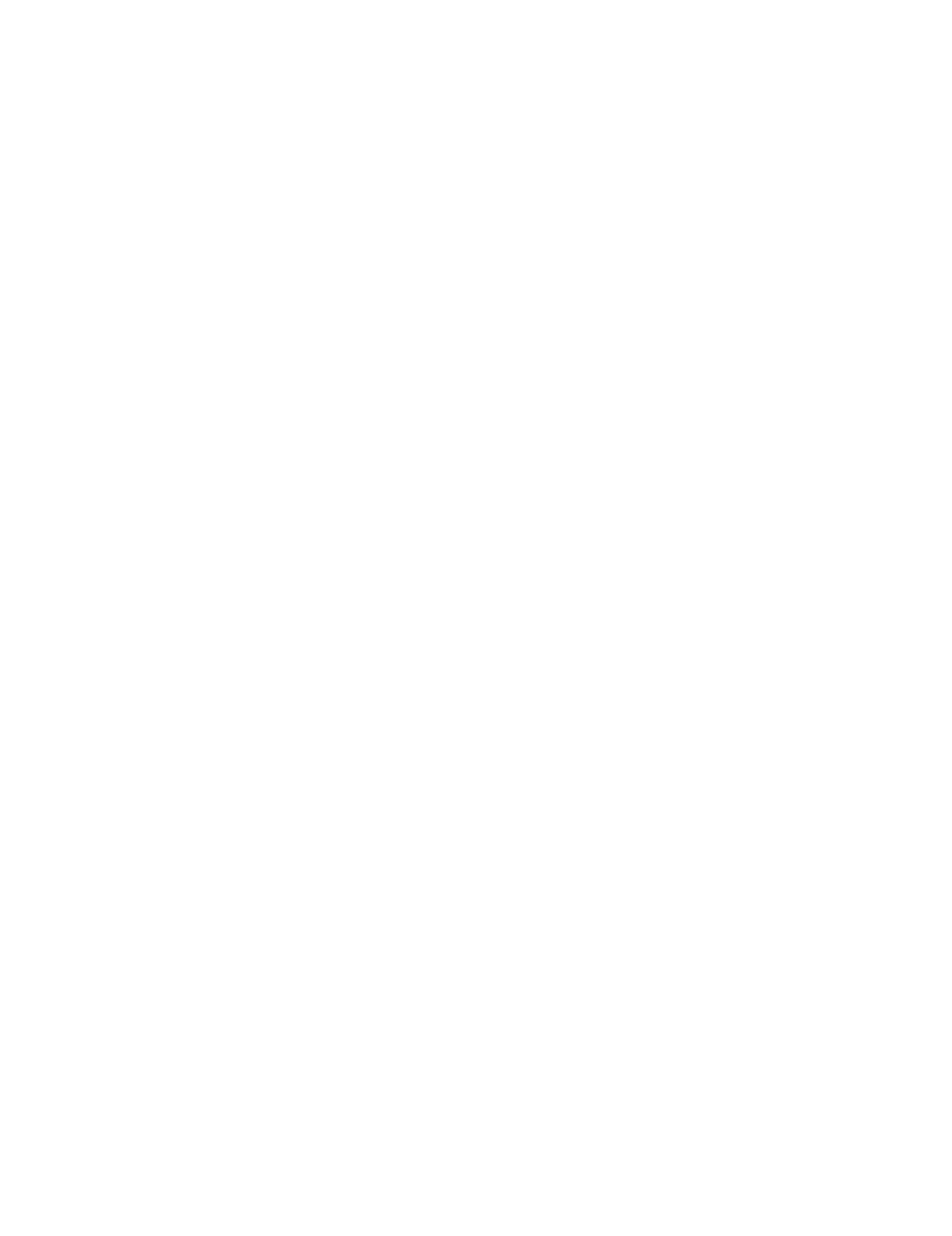
Fig. 25 The Value Chain in Farming: Autonomous (blue) vs. Symbiotic (green) vs. Parasitic (red). How M7 thinks of Uganda vs. China vs. Europe. This is an issue M7 always highlights. Africa embodies the input & compression blue nodes, but the transformation from produce into products like Starbucks demands elements from the pre-input blue nodes like sound governance, tactful position of a nations farm produce by ensuring liquidity for farmers. Thats where the issue at 26:00/27:57 stems from!#
The hidden layer, akin to the photosynthetic canopy, includes nodes such as âCanker,â âFarming,â and âLeaf.â These nodes operate as the systemâs processing hubs, mediating between input and output while addressing complexities such as disease, cultivation, and adaptation. They reflect the networkâs capacity for transformation, filtering inputs and optimizing outputs through a blend of resilience and resourcefulness. The hidden layer is where growth meets vulnerability, and innovation emerges as a response to challenge.
Finally, the output layer manifests as the fruits of the system, through nodes like âTransformation,â âToxins,â âProduce,â âNutrition,â and âStarch.â These outputs are not static endpoints but dynamic indicators of the ecosystemâs success or failure. Whether nourishing or toxic, these outputs encapsulate the culmination of growth processes, reflecting the harmonyâor discordâachieved by the systemâs interplay. They serve as feedback loops, influencing future growth and adaptation.
By reframing the network as an ecosystem, this interpretation emphasizes a living, evolving structure, resonant with biological vitality and philosophical depth. It captures the dance between chaos and order, illustrating how interconnected elements sustain and transform one another to create a dynamic whole.