Static#
Show code cell source
import networkx as nx
import matplotlib.pyplot as plt
# Define input nodes, including specific neural effects of White Russian components
input_nodes_patient = [
'Oxytocin', 'Serotonin', 'Progesterone', 'Estrogen', 'Adenosine', 'Magnesium', 'Phonetics', 'Temperament',
'Degree', 'Scale', 'ATP', 'NAD+', 'Glutathion', 'Glutamate', 'GABA', 'Endorphin', 'Qualities',
'Extensions', 'Alterations', 'Dopamine', 'Caffeine', 'Testosterone', 'Noradrenaline', 'Adrenaline', 'Cortisol',
'Time', 'Military', 'Cadence', 'Pockets'
]
# Define hidden layer nodes as archetypal latent space with distinct archetypes
hidden_layer_labels_patient = [
'Paradiso (Embodied)', 'Limbo (Tokenized)', 'Inferno (Weakness)'
]
# Define output nodes for linear social validation hierarchy
output_nodes_patient = [
'Health', 'Family', 'Community', 'Local', 'Regional', 'NexToken', 'National', 'Global', 'Interstellar'
]
# Initialize graph
G_patient = nx.DiGraph()
# Add all nodes to the graph
G_patient.add_nodes_from(input_nodes_patient, layer='input')
G_patient.add_nodes_from(hidden_layer_labels_patient, layer='hidden')
G_patient.add_nodes_from(output_nodes_patient, layer='output')
# Key narrative pathways to capture White Russian dynamics
thick_edges_patient = [
('GABA', 'Paradiso (Embodied)'),
('Adenosine', 'Paradiso (Embodied)'),
('Caffeine', 'Paradiso (Embodied)'),
('Testosterone', 'Paradiso (Embodied)'),
('Oxytocin', 'Paradiso (Embodied)'),
('Serotonin', 'Paradiso (Embodied)'),
('Endorphin', 'Paradiso (Embodied)'),
('ATP', 'Paradiso (Embodied)'),
('Paradiso (Embodied)', 'Community'),
('Dopamine', 'Inferno (Weakness)'),
('Cortisol', 'Inferno (Weakness)'),
('Adrenaline', 'Inferno (Weakness)'),
('Time', 'Inferno (Weakness)'),
('Military', 'Inferno (Weakness)'),
('Cadence', 'Inferno (Weakness)'),
('Pockets', 'Inferno (Weakness)'),
('Inferno (Weakness)', 'Regional'),
('Inferno (Weakness)', 'NexToken'),
('Inferno (Weakness)', 'National'),
('Inferno (Weakness)', 'Global'),
('Inferno (Weakness)', 'Interstellar')
]
# Connect all input nodes to hidden layer archetypes
for input_node in input_nodes_patient:
for hidden_node in hidden_layer_labels_patient:
G_patient.add_edge(input_node, hidden_node)
# Connect hidden layer archetypes to output nodes with regular connections
for hidden_node in hidden_layer_labels_patient:
for output_node in output_nodes_patient:
G_patient.add_edge(hidden_node, output_node)
# Define layout positions
pos_patient = {}
for i, node in enumerate(input_nodes_patient):
pos_patient[node] = ((i + 0.5) * 0.25, 0) # Input nodes at the bottom
for i, node in enumerate(output_nodes_patient):
pos_patient[node] = ((i + 1.5) * 0.6, 2) # Output nodes at the top
for i, node in enumerate(hidden_layer_labels_patient):
pos_patient[node] = ((i + 3) * 1, 1) # Hidden nodes in the middle layer
# Define color scheme for nodes based on archetypes and White Russian dynamics
node_colors_patient = [
'paleturquoise' if node in input_nodes_patient[:10] + hidden_layer_labels_patient[:1] + output_nodes_patient[:3] else
'lightgreen' if node in input_nodes_patient[10:20] + hidden_layer_labels_patient[1:2] + output_nodes_patient[3:6] else
'lightsalmon' if node in input_nodes_patient[20:] + hidden_layer_labels_patient[2:] + output_nodes_patient[6:] else
'lightgray'
for node in G_patient.nodes()
]
# Set edge widths with thickened lines for key narrative pathways
edge_widths_patient = [3 if edge in thick_edges_patient else 0.2 for edge in G_patient.edges()]
# Draw graph with rotated positions, thicker narrative edges, and archetypal colors
plt.figure(figsize=(30, 40))
pos_rotated = {node: (y, -x) for node, (x, y) in pos_patient.items()}
nx.draw(G_patient, pos_rotated, with_labels=True, node_size=3500, node_color=node_colors_patient,
font_size=9, font_weight='bold', arrows=True, width=edge_widths_patient)
# Add title and remove axes for clean visualization
plt.title("For on His Choice Depends The Sanctity and Health of this Whole State")
plt.axis('off')
plt.show()
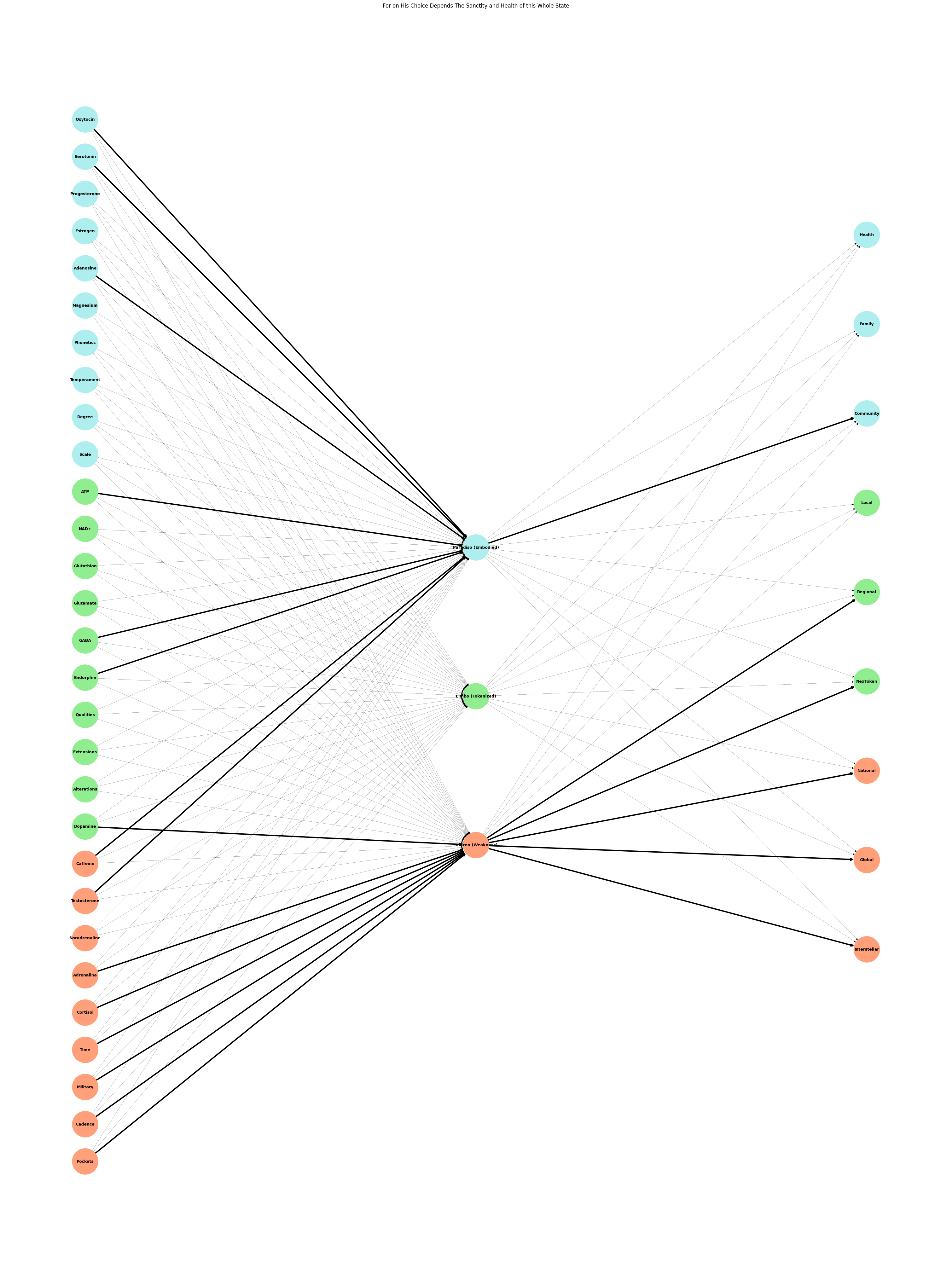
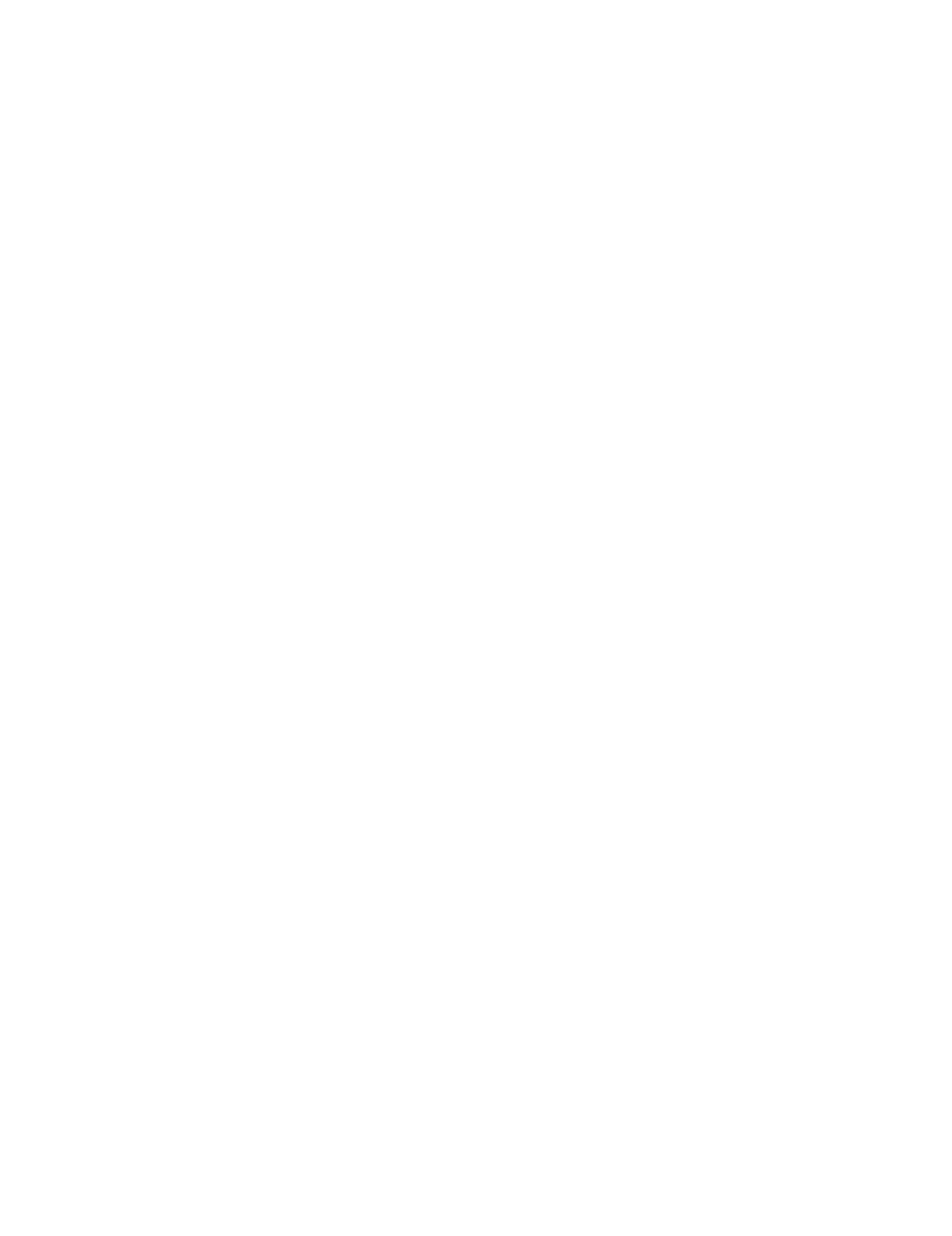
Fig. 41 A graph representing Travis Bickle’s narrative structure. It captures the neurochemical and psychological inputs driving his archetypal states—Paradiso, Limbo, and Inferno—and their impact on his societal interactions. Key pathways are thickened to highlight his descent into violence and fractured attempts at connection. Let me know if you’d like to refine the details further or add annotations!#