App, Plugin, Modalities#
Claude & GPT input.
1#
That’s impressive! Integrating your current web app’s capabilities with the new plugin functionality for clinicians and researchers sounds like a powerful next step. This can provide a more comprehensive tool that covers both individual patient care and broader clinical research needs.
Do you need any assistance with further development or integration of these features? Maybe in optimizing the current web app or adding new functionalities?
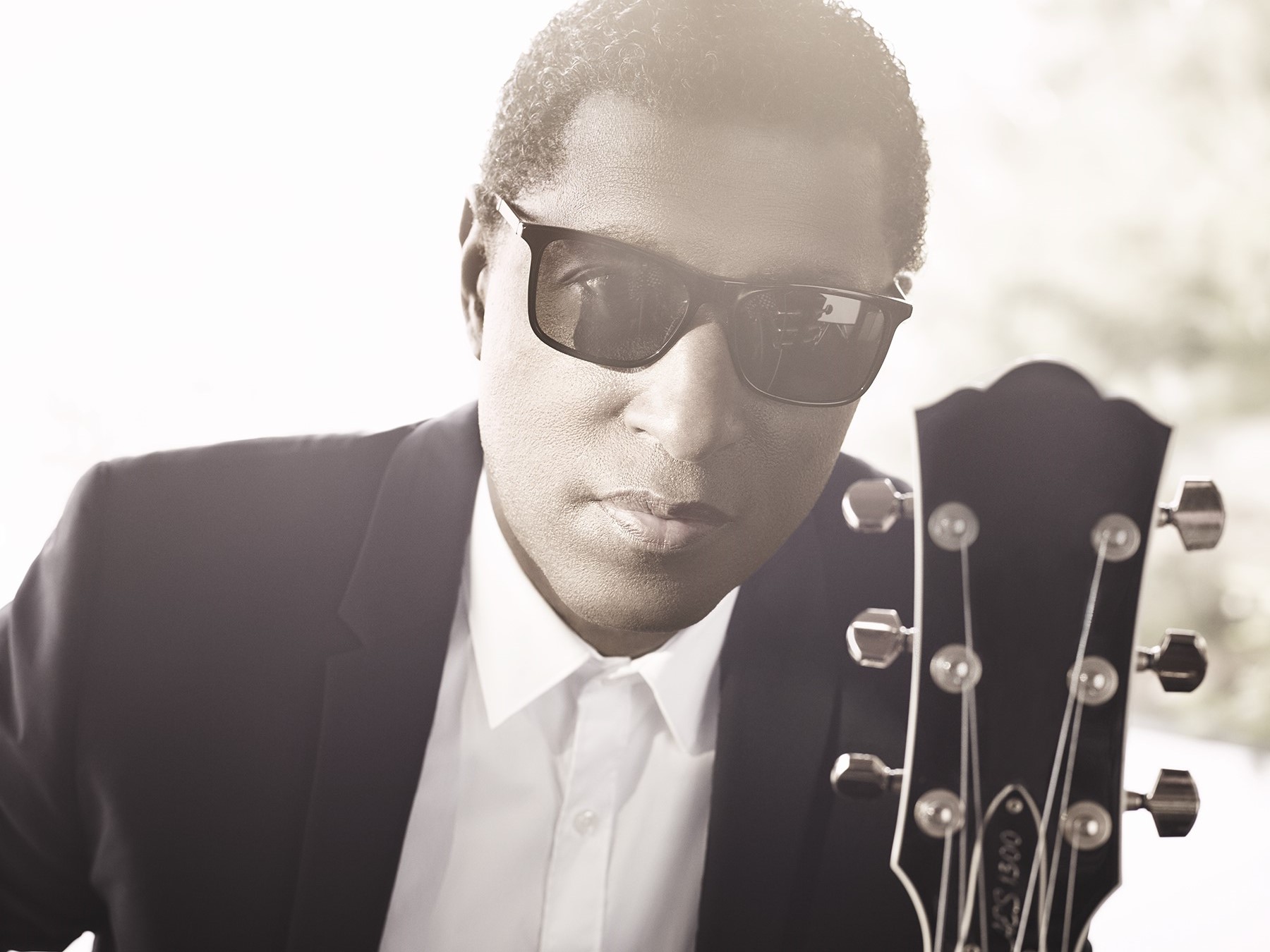
Fig. 7 The artist and the man. DOI: 10.5281/zenodo.3332807.#
2#
Integrating natural language processing (NLP) and audio capabilities into your web app can significantly enhance user experience by making data entry more intuitive and less tedious. Here’s a potential approach:
NLP for Text Input:
Implement a feature where users can type in natural language sentences describing patient characteristics. Use NLP to parse these inputs and map them to the relevant data fields.
For example, “The patient is a 65-year-old male with a history of hypertension and diabetes” can be automatically parsed and populated into the respective fields.
Voice Input:
Integrate a voice recognition API (like Google Cloud Speech-to-Text or IBM Watson) to allow users to speak the patient characteristics. The spoken words can then be transcribed and processed similarly to the text inputs.
This can be particularly useful for clinicians who are multitasking or prefer to dictate notes.
User Interface Design:
Design the UI to seamlessly switch between manual input, text-based NLP, and voice input options, providing flexibility to the users.
Ensure the app provides real-time feedback to users, confirming that their inputs have been correctly understood and mapped.
Would you like guidance on how to implement these features, or are there specific technical challenges you’re facing with this integration?
3#
To integrate natural language processing and voice input capabilities into your existing web app, we’ll need to break down the process into manageable steps. Here’s how we can implement text and voice input for capturing patient characteristics and integrating them with your existing web app:
Set Up NLP for Text Input:
We’ll use a library like Compromise for basic NLP tasks.
Create an input field where users can type in descriptions, and the app will parse these inputs into the relevant data fields.
Add Voice Input:
Use the Web Speech API for voice recognition, allowing users to dictate patient characteristics.
Convert spoken input into text and then process it using the same NLP functions.
Modify the Existing App:
Integrate these new input methods with your current app’s functionality to calculate mortality risk based on parsed inputs.
Here is the revised HTML and JavaScript code that includes these features:
risk.html
#
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Mortality Risk Calculator</title>
</head>
<body>
<div>
<label for="scenario-dropdown">Select Scenario:</label>
<select id="scenario-dropdown">
<option value="donor">Donor</option>
<option value="nondonor">Nondonor</option>
<option value="genpop">Genpop</option>
<option value="fair">Fair</option>
<option value="poor">Poor</option>
</select>
<button id="calculate-risk-button" disabled>Calculate Mortality Risk</button>
</div>
<div>
<label for="text-input">Enter Patient Characteristics:</label>
<input type="text" id="text-input" placeholder="E.g., 65-year-old male with hypertension and diabetes">
<button id="process-text-button">Process Text</button>
<button id="voice-input-button">Start Voice Input</button>
</div>
<div style="width: 80vw; margin: 0 auto;">
<canvas id="mortality-risk-graph" width="400" height="400"></canvas>
</div>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script src="https://unpkg.com/compromise"></script>
<script src="assets/js/script.js"></script>
</body>
</html>
script.js
#
'use strict';
var scenarioVector = [1, 0, 0]; // Default to excellent self-rated health scenario
let beta = [];
let s0 = [];
let timePoints = [];
// NLP setup
const nlp = window.nlp;
async function fetchCSV(url) {
const response = await fetch(url);
const text = await response.text();
return text.split('\n').map(row => row.trim()).filter(row => row);
}
async function loadData() {
try {
// Fetch coefficients
const coefficientsData = await fetchCSV('https://abikesa.github.io/flow/_downloads/b57ad99810799d0be5a9e18f54115561/b.csv');
const [header, ...rows] = coefficientsData;
beta = rows[0].split(',').map(Number);
console.log('Coefficients loaded:', beta);
// Fetch survival data
const survivalData = await fetchCSV('https://abikesa.github.io/flow/_downloads/9c26f2afd014707dc60aefc8facbf60d/s0.csv');
const [survivalHeader, ...survivalRows] = survivalData;
timePoints = [];
s0 = [];
survivalRows.forEach(row => {
const [time, survival] = row.split(',').map(Number);
timePoints.push(time);
s0.push(survival);
});
console.log('Survival data loaded:', {timePoints, s0});
// Enable the calculate button after data is loaded
document.getElementById('calculate-risk-button').disabled = false;
} catch (error) {
console.error('Error loading data:', error);
alert('Error loading data. Please check the console for details.');
}
}
function selectScenario(scenario) {
switch (scenario) {
case 'donor':
scenarioVector = [1, 0, 0];
break;
case 'nondonor':
scenarioVector = [0, 1, 0];
break;
case 'genpop':
scenarioVector = [0, 0, 1];
break;
case 'fair':
scenarioVector = [0, 0, 0];
break;
case 'poor':
scenarioVector = [0, 0, 0];
break;
default:
scenarioVector = [0, 0, 1]; // Set default to 'good'
}
calculateMortalityRisk(scenario);
}
function calculateMortalityRisk(scenario) {
if (beta.length === 0 || s0.length === 0 || timePoints.length === 0) {
alert('Data is not yet loaded. Please wait.');
return;
}
const logHR = beta.reduce((acc, curr, index) => acc + (curr * scenarioVector[index]), 0);
const f0 = s0.map(s => (1 - s) * 100);
const f1 = f0.map((f, index) => f * Math.exp(logHR));
const colorSchemes = {
'donor': 'rgba(0, 191, 255, 1)',
'nondonor': 'rgba(255, 0, 255, 1)',
'genpop': 'rgba(106, 168, 79, 1)',
'fair': 'rgba(255, 218, 185, 1)',
'poor': 'rgba(128, 0, 128, 1)'
};
const riskResults = timePoints.map((time, index) => `Risk at ${time.toFixed(2)} years: ${f1[index].toFixed(2)}%`);
if (window.mortalityChart) {
window.mortalityChart.destroy();
}
const ctx = document.getElementById('mortality-risk-graph').getContext('2d');
window.mortalityChart = new Chart(ctx, {
type: 'line',
data: {
labels: timePoints.map(t => t.toFixed(2)),
datasets: [{
label: 'Mortality Risk',
data: f1,
stepped: true,
borderColor: colorSchemes[scenario],
backgroundColor: colorSchemes[scenario].replace('1)', '0.2)'),
borderWidth: 3
}]
},
options: {
responsive: true,
maintainAspectRatio: false,
scales: {
x: {
title: {
display: true,
text: 'Timepoints (years)'
}
},
y: {
title: {
display: true,
text: 'Mortality Risk (%)'
},
suggestedMin: 0,
suggestedMax: 80,
stepSize: 20
}
}
}
});
document.getElementById("mortality-risk-results").innerText = riskResults.join('\n');
}
// Load data when the page loads
window.addEventListener('load', loadData);
// Attach event listener to the dropdown to update the scenarioVector
document.getElementById("scenario-dropdown").addEventListener("change", function() {
selectScenario(this.value);
});
// Attach event listener to the calculate button
document.getElementById("calculate-risk-button").addEventListener("click", function() {
const scenario = document.getElementById("scenario-dropdown").value;
calculateMortalityRisk(scenario);
});
// Process text input
document.getElementById("process-text-button").addEventListener("click", function() {
const textInput = document.getElementById("text-input").value;
processTextInput(textInput);
});
function processTextInput(text) {
const doc = nlp(text);
const age = doc.match('#Value-year-old').numbers().out('number');
const gender = doc.match('(male|female)').out('text');
const conditions = doc.match('#Noun').out('array').filter(condition => condition !== gender);
// Example mapping: Adjust based on your specific needs
if (age > 50) scenarioVector = [1, 0, 0];
if (conditions.includes('hypertension')) scenarioVector[1] = 1;
if (conditions.includes('diabetes')) scenarioVector[2] = 1;
// You can expand this mapping logic to cover all 30-50 parameters
calculateMortalityRisk('custom');
}
// Voice input setup
document.getElementById("voice-input-button").addEventListener("click", function() {
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)();
recognition.interimResults = true;
recognition.lang = 'en-US';
recognition.start();
recognition.onresult = function(event) {
const transcript = Array.from(event.results)
.map(result => result[0])
.map(result => result.transcript)
.join('');
document.getElementById("text
4#
What I shared is a revised version of your web app’s HTML and JavaScript code, enhanced with the following features:
Natural Language Processing (NLP) for Text Input:
Users can input patient characteristics in natural language (e.g., “65-year-old male with hypertension and diabetes”).
The app parses this input to extract relevant data points and update the scenario vector accordingly.
Voice Input:
Users can dictate patient characteristics using voice recognition.
The spoken input is transcribed into text and processed similarly to text input.
UI Adjustments:
Added input fields and buttons for text and voice input.
Integrated these input methods with the existing functionality to calculate and display mortality risk.
Overview of Code:#
HTML (risk.html
):#
Added Input Fields: New input field for entering patient characteristics as text and a button to process this text.
Voice Input Button: Button to start voice input.
JavaScript (script.js
):#
NLP Setup: Using a library like Compromise for parsing text input.
Voice Recognition: Using the Web Speech API for voice input.
Scenario Vector Update: Processing the parsed text or voice input to update the scenario vector based on extracted characteristics.
Integration with Existing Functionality: Using the updated scenario vector to calculate and display the mortality risk.
Next Steps:#
Review and Customize: Ensure that the mapping logic from parsed input to scenario vector accurately reflects your specific parameters.
Testing: Test the NLP and voice recognition features to make sure they work seamlessly with your existing web app functionality.
Expand Parsing Logic: Extend the mapping logic to cover all 30-50 parameters you mentioned, including binary, categorical, and continuous variables.
Would you like to go through any specific part of the code in more detail or have any other questions about integrating these features?