.#
find ~/dropbox/1f.ἡἔρις,κ/1.ontology -maxdepth 4 -type f -name "*.dta"
GitHub Universe 23
November 8-9, 2023
Yerba Buena, Center for the Arts, San Fransisco, CA
Google Cloud Next ’24
April 9-11, 2024
Mandalay Bay, Convention Center, Las Vegas, NV
\( \Large \left\{ \begin{array}{ll} \text{Aesthetics/Beauty} \\ \text{} \\ \textcolor{gray}{\text{Dynamometer/Speed}} \ \ \left\{ \begin{array}{l} \textcolor{gray}{\text{Rigor/Tournament}} \text{} \\ \text{Error/Worthy} \ \ \ \ \ \ \ \ \ \left\{ \begin{array}{l} \text{Variance/Natural} \\ \text{Bias/Madeup} \end{array} \right. \\ \text{Sloppy/Unworthy} \end{array} \right. \left\{ \begin{array}{l} \text{Explain/Control} \end{array} \right. \\ \text{} \\ \text{Navel-gazing/Ugly} \end{array} \right. \)
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.cm import ScalarMappable
from matplotlib.colors import LinearSegmentedColormap, PowerNorm
def gaussian(x, mean, std_dev, amplitude=1):
return amplitude * np.exp(-0.9 * ((x - mean) / std_dev) ** 2)
def overlay_gaussian_on_line(ax, start, end, std_dev):
x_line = np.linspace(start[0], end[0], 100)
y_line = np.linspace(start[1], end[1], 100)
mean = np.mean(x_line)
y = gaussian(x_line, mean, std_dev, amplitude=std_dev)
ax.plot(x_line + y / np.sqrt(2), y_line + y / np.sqrt(2), color='red', linewidth=2.5)
fig, ax = plt.subplots(figsize=(10, 10))
intervals = np.linspace(0, 100, 11)
custom_means = np.linspace(1, 23, 10)
custom_stds = np.linspace(.5, 10, 10)
# Change to 'viridis' colormap to get gradations like the older plot
cmap = plt.get_cmap('viridis')
norm = plt.Normalize(custom_stds.min(), custom_stds.max())
sm = ScalarMappable(cmap=cmap, norm=norm)
sm.set_array([])
median_points = []
for i in range(10):
xi, xf = intervals[i], intervals[i+1]
x_center, y_center = (xi + xf) / 2 - 20, 100 - (xi + xf) / 2 - 20
x_curve = np.linspace(custom_means[i] - 3 * custom_stds[i], custom_means[i] + 3 * custom_stds[i], 200)
y_curve = gaussian(x_curve, custom_means[i], custom_stds[i], amplitude=15)
x_gauss = x_center + x_curve / np.sqrt(2)
y_gauss = y_center + y_curve / np.sqrt(2) + x_curve / np.sqrt(2)
ax.plot(x_gauss, y_gauss, color=cmap(norm(custom_stds[i])), linewidth=2.5)
median_points.append((x_center + custom_means[i] / np.sqrt(2), y_center + custom_means[i] / np.sqrt(2)))
median_points = np.array(median_points)
ax.plot(median_points[:, 0], median_points[:, 1], '--', color='grey')
start_point = median_points[0, :]
end_point = median_points[-1, :]
overlay_gaussian_on_line(ax, start_point, end_point, 24)
ax.grid(True, linestyle='--', linewidth=0.5, color='grey')
ax.set_xlim(-30, 111)
ax.set_ylim(-20, 87)
# Create a new ScalarMappable with a reversed colormap just for the colorbar
cmap_reversed = plt.get_cmap('viridis').reversed()
sm_reversed = ScalarMappable(cmap=cmap_reversed, norm=norm)
sm_reversed.set_array([])
# Existing code for creating the colorbar
cbar = fig.colorbar(sm_reversed, ax=ax, shrink=1, aspect=90)
# Specify the tick positions you want to set
custom_tick_positions = [0.5, 5, 8, 10] # example positions, you can change these
cbar.set_ticks(custom_tick_positions)
# Specify custom labels for those tick positions
custom_tick_labels = ['5', '3', '1', '0'] # example labels, you can change these
cbar.set_ticklabels(custom_tick_labels)
# Label for the colorbar
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
# Label for the colorbar
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
cbar.set_label(r'♭', rotation=0, labelpad=15, fontstyle='italic', fontsize=24)
# Add X and Y axis labels with custom font styles
ax.set_xlabel(r'Principal Component', fontstyle='italic')
ax.set_ylabel(r'Emotional State', rotation=0, fontstyle='italic', labelpad=15)
# Add musical modes as X-axis tick labels
# musical_modes = ["Ionian", "Dorian", "Phrygian", "Lydian", "Mixolydian", "Aeolian", "Locrian"]
greek_letters = ['α', 'β','γ', 'δ', 'ε', 'ζ', 'η'] # 'θ' , 'ι', 'κ'
mode_positions = np.linspace(ax.get_xlim()[0], ax.get_xlim()[1], len(greek_letters))
ax.set_xticks(mode_positions)
ax.set_xticklabels(greek_letters, rotation=0)
# Add moods as Y-axis tick labels
moods = ["flow", "control", "relaxed", "bored", "apathy","worry", "anxiety", "arousal"]
mood_positions = np.linspace(ax.get_ylim()[0], ax.get_ylim()[1], len(moods))
ax.set_yticks(mood_positions)
ax.set_yticklabels(moods)
# ... (rest of the code unchanged)
plt.tight_layout()
plt.show()
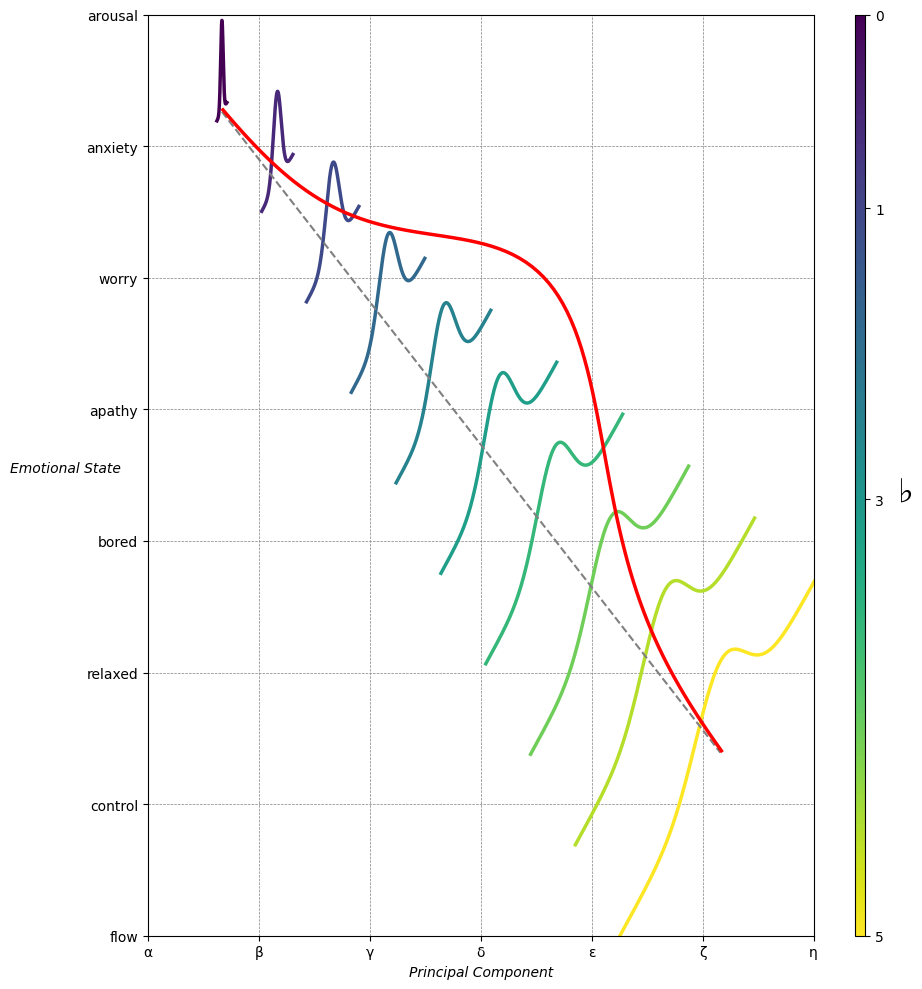
Show code cell source
import matplotlib.pyplot as plt
import numpy as np
# Create data for the skill and challenge levels
skill_levels = np.linspace(0, 10, 100)
challenge_levels = np.linspace(0, 10, 100)
# Define the flow channel boundaries
flow_channel = skill_levels
# Adjust the phase and amplitude of the sinusoid wave
phase = np.pi / 16
amplitude = 1.5
sinusoid = flow_channel + np.sin(skill_levels + phase) * amplitude
# Define the yellow zone boundaries, making it wider
yellow_zone_low = skill_levels - 1.5 # Adjust this value to make the yellow zone wider or narrower
yellow_zone_high = skill_levels + 1.5 # Adjust this value to make the yellow zone wider or narrower
# Plotting
plt.figure(figsize=(15, 10))
# Plot the anxiety and boredom areas
plt.fill_between(skill_levels, yellow_zone_high, 10, color='orangered', alpha=0.3, label='Place/Identification', interpolate=True)
plt.fill_between(skill_levels, 0, yellow_zone_low, color='lime', alpha=0.3, label='Time/Revelation', interpolate=True)
plt.fill_between(skill_levels, yellow_zone_low, yellow_zone_high, color='yellow', alpha=0.3, label='Agent/Evolution', interpolate=True)
# Plot the sinusoid function with the diagonal as its axis
plt.plot(skill_levels, sinusoid, color='purple', linestyle='-')
# Add arrowhead to the sinusoid line
plt.arrow(skill_levels[-2], sinusoid[-2], skill_levels[-1] - skill_levels[-2], sinusoid[-1] - sinusoid[-2],
color='purple', length_includes_head=True, head_width=0.15, head_length=0.3)
# Set plot labels and title
plt.xlabel('Age', fontsize=18)
plt.ylabel('Résumé', rotation='vertical', fontsize=18)
# Set plot limits and grid
plt.xlim(0, 10)
plt.ylim(0, 10)
plt.grid(True)
# Set tick labels
tick_labels = ['0', '20', '40', '60', '80', '99']
plt.xticks(np.linspace(0, 10, 6), tick_labels)
plt.yticks(np.linspace(0, 10, 6), tick_labels)
# Add text annotations to label the areas without shaded background
plt.text(1.5, 6.6, 'China as the Ribosome or\n Translation Machinery \n (Mostly Anxiety)', color='black', ha='center', va='center', fontsize=18)
plt.text(4.5, 4.7, 'The United States as\n DNA-mRNA-Protein \n (Essence of Flow)', color='black', ha='center', va='center', fontsize=18)
plt.text(8, 3, 'Europe as Nuclear Factors\n Regulating Expression \n (Relaxation Incarnate)', color='black', ha='center', va='center', fontsize=18)
# Display the plot
plt.show()
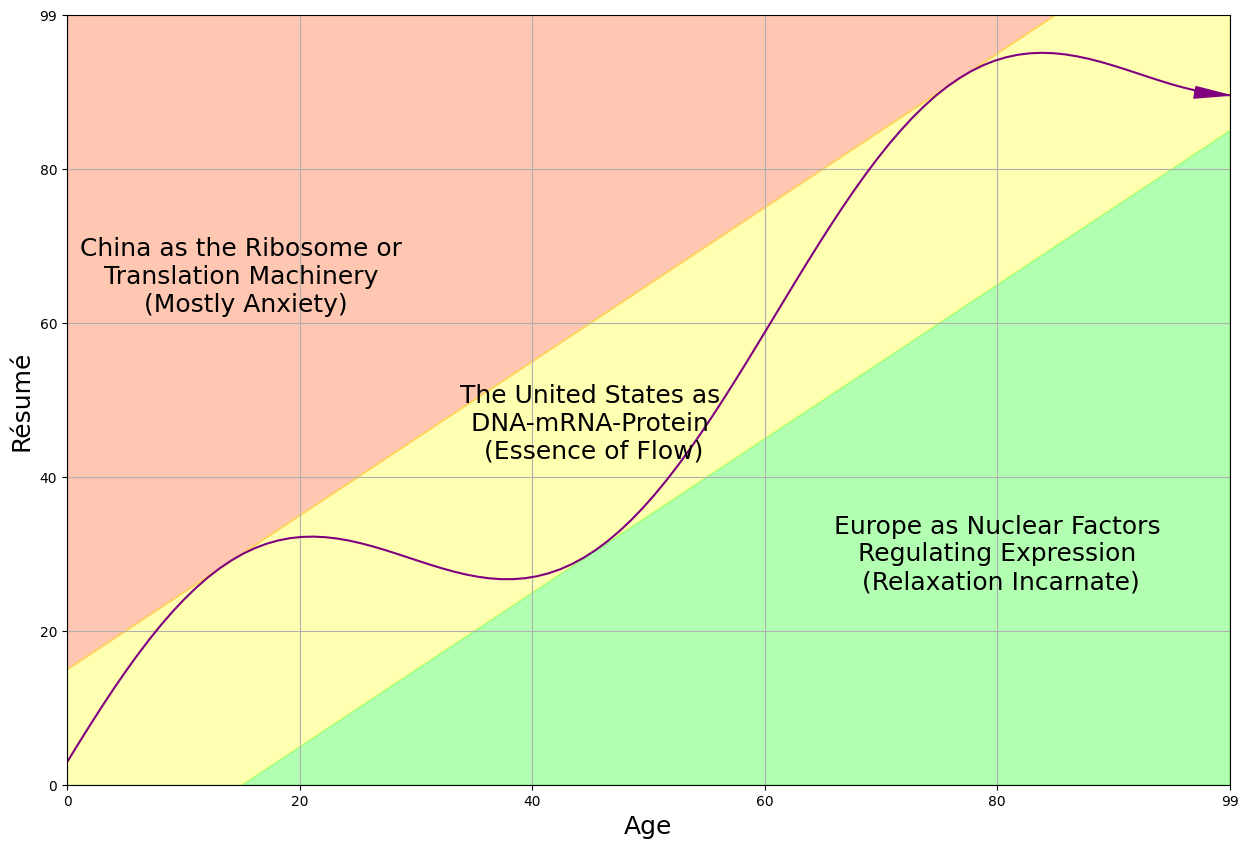